KIO
#include <jobuidelegate.h>
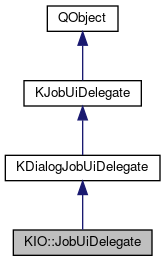
Public Types | |
enum | ConfirmationType { DefaultConfirmation, ForceConfirmation } |
enum | DeletionType { Delete, Trash, EmptyTrash } |
enum | MessageBoxType { QuestionYesNo = 1, WarningYesNo = 2, WarningContinueCancel = 3, WarningYesNoCancel = 4, Information = 5, SSLMessageBox = 6 } |
Public Member Functions | |
JobUiDelegate () | |
virtual | ~JobUiDelegate () |
bool | askDeleteConfirmation (const KUrl::List &urls, DeletionType deletionType, ConfirmationType confirmationType) |
virtual SkipDialog_Result | askSkip (KJob *job, bool multi, const QString &error_text) |
int | requestMessageBox (MessageBoxType type, const QString &text, const QString &caption, const QString &buttonYes, const QString &buttonNo, const QString &iconYes=QString(), const QString &iconNo=QString(), const QString &dontAskAgainName=QString(), const KIO::MetaData &sslMetaData=KIO::MetaData()) |
virtual void | setWindow (QWidget *window) |
![]() | |
KDialogJobUiDelegate () | |
virtual | ~KDialogJobUiDelegate () |
virtual void | showErrorMessage () |
void | updateUserTimestamp (unsigned long time) |
unsigned long | userTimestamp () const |
QWidget * | window () const |
![]() | |
KJobUiDelegate () | |
virtual | ~KJobUiDelegate () |
bool | isAutoErrorHandlingEnabled () const |
bool | isAutoWarningHandlingEnabled () const |
void | setAutoErrorHandlingEnabled (bool enable) |
void | setAutoWarningHandlingEnabled (bool enable) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Related Functions | |
(Note that these are not member functions.) | |
virtual RenameDialog_Result | askFileRename (KJob *job, const QString &caption, const QString &src, const QString &dest, KIO::RenameDialog_Mode mode, QString &newDest, KIO::filesize_t sizeSrc=KIO::filesize_t(-1), KIO::filesize_t sizeDest=KIO::filesize_t(-1), time_t ctimeSrc=time_t(-1), time_t ctimeDest=time_t(-1), time_t mtimeSrc=time_t(-1), time_t mtimeDest=time_t(-1)) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | slotWarning (KJob *job, const QString &plain, const QString &rich) |
![]() | |
virtual void | slotWarning (KJob *job, const QString &plain, const QString &rich) |
![]() | |
KJob * | job () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
A UI delegate tuned to be used with KIO Jobs.
Definition at line 39 of file jobuidelegate.h.
Member Enumeration Documentation
ForceConfirmation: always ask the user for confirmation DefaultConfirmation: don't ask the user if he/she said "don't ask again".
Used by askDeleteConfirmation.
Enumerator | |
---|---|
DefaultConfirmation | |
ForceConfirmation |
Definition at line 116 of file jobuidelegate.h.
The type of deletion: real deletion, moving the files to the trash or emptying the trash Used by askDeleteConfirmation.
Enumerator | |
---|---|
Delete | |
Trash | |
EmptyTrash |
Definition at line 109 of file jobuidelegate.h.
Message box types.
Should be kept in sync with SlaveBase::MessageBoxType.
- Since
- 4.11
Enumerator | |
---|---|
QuestionYesNo | |
WarningYesNo | |
WarningContinueCancel | |
WarningYesNoCancel | |
Information | |
SSLMessageBox |
Definition at line 141 of file jobuidelegate.h.
Constructor & Destructor Documentation
KIO::JobUiDelegate::JobUiDelegate | ( | ) |
Constructs a new KIO Job UI delegate.
Definition at line 48 of file jobuidelegate.cpp.
|
virtual |
Destroys the KIO Job UI delegate.
Definition at line 53 of file jobuidelegate.cpp.
Member Function Documentation
bool KIO::JobUiDelegate::askDeleteConfirmation | ( | const KUrl::List & | urls, |
DeletionType | deletionType, | ||
ConfirmationType | confirmationType | ||
) |
Ask for confirmation before deleting/trashing urls
.
Note that this method is not called automatically by KIO jobs. It's the application's responsibility to ask the user for confirmation before calling KIO::del() or KIO::trash().
- Parameters
-
urls the urls about to be deleted/trashed method the type of deletion (Delete for real deletion, Trash otherwise) confirmation see ConfirmationType. Normally set to DefaultConfirmation. Note: the window passed to setWindow is used as the parent for the message box.
- Returns
- true if confirmed
Definition at line 108 of file jobuidelegate.cpp.
|
virtual |
See skipdialog.h
Definition at line 97 of file jobuidelegate.cpp.
int KIO::JobUiDelegate::requestMessageBox | ( | MessageBoxType | type, |
const QString & | text, | ||
const QString & | caption, | ||
const QString & | buttonYes, | ||
const QString & | buttonNo, | ||
const QString & | iconYes = QString() , |
||
const QString & | iconNo = QString() , |
||
const QString & | dontAskAgainName = QString() , |
||
const KIO::MetaData & | sslMetaData = KIO::MetaData() |
||
) |
This function allows for the delegation user prompts from the ioslaves.
- Parameters
-
type the desired type of message box. text the message shown to the user. caption the caption of the message dialog box. buttonYes the text for the YES button. buttonNo the text for the NO button. iconYes the icon shown on the YES button. iconNo the icon shown on the NO button. dontAskAgainName the name used to store result from 'Do not ask again' checkbox. sslMetaData SSL information used by the SSLMessageBox.
- Since
- 4.11
Definition at line 200 of file jobuidelegate.cpp.
|
virtual |
Associate this job with a window given by window
.
- Parameters
-
window the window to associate to
- See also
- window()
Reimplemented from KDialogJobUiDelegate.
Definition at line 58 of file jobuidelegate.cpp.
Friends And Related Function Documentation
|
related |
Construct a modal, parent-less "rename" dialog, and return a result code, as well as the new dest. Much easier to use than the class RenameDialog directly.
- Parameters
-
caption the caption for the dialog box src the URL of the file/dir we're trying to copy, as it's part of the text message dest the URL of the destination file/dir, i.e. the one that already exists mode parameters for the dialog (which buttons to show...), see RenameDialog_Mode newDestPath the new destination path, valid if R_RENAME was returned. sizeSrc size of source file sizeDest size of destination file ctimeSrc creation time of source file ctimeDest creation time of destination file mtimeSrc modification time of source file mtimeDest modification time of destination file
- Returns
- the result
Definition at line 64 of file jobuidelegate.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.