kjsembed
#include <qobject_binding.h>
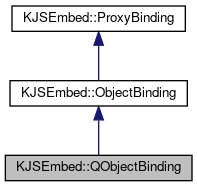
Public Types | |
enum | Access { None = 0x00, ScriptableSlots = 0x01, NonScriptableSlots = 0x02, PrivateSlots = 0x04, ProtectedSlots = 0x08, PublicSlots = 0x10, AllSlots = ScriptableSlots|NonScriptableSlots|PrivateSlots|ProtectedSlots|PublicSlots, ScriptableSignals = 0x100, NonScriptableSignals = 0x200, PrivateSignals = 0x400, ProtectedSignals = 0x800, PublicSignals = 0x1000, AllSignals = ScriptableSignals|NonScriptableSignals|PrivateSignals|ProtectedSignals|PublicSignals, ScriptableProperties = 0x10000, NonScriptableProperties = 0x20000, AllProperties = ScriptableProperties|NonScriptableProperties, GetParentObject = 0x100000, SetParentObject = 0x200000, ChildObjects = 0x400000, AllObjects = GetParentObject|SetParentObject|ChildObjects } |
![]() | |
enum | Ownership { CPPOwned, QObjOwned, JSOwned } |
Public Member Functions | |
QObjectBinding (KJS::ExecState *exec, QObject *object) | |
virtual | ~QObjectBinding () |
AccessFlags | access () const |
bool | canPut (KJS::ExecState *exec, const KJS::Identifier &propertyName) const |
KJS::UString | className () const |
bool | getOwnPropertySlot (KJS::ExecState *exec, const KJS::Identifier &propertyName, KJS::PropertySlot &slot) |
void | put (KJS::ExecState *exec, const KJS::Identifier &propertyName, KJS::JSValue *value, int attr=KJS::None) |
template<typename T > | |
T * | qobject () const |
void | setAccess (AccessFlags access) |
KJS::UString | toString (KJS::ExecState *exec) const |
void | watchObject (QObject *object) |
![]() | |
template<typename T > | |
ObjectBinding (KJS::ExecState *exec, const char *typeName, T *ptr) | |
virtual | ~ObjectBinding () |
KJS::UString | className () const |
template<typename T > | |
T * | object () const |
Ownership | ownership () const |
template<typename T > | |
void | setObject (T *ptr) |
void | setOwnership (Ownership owner) |
KJS::UString | toString (KJS::ExecState *exec) const |
KJS::JSType | type () const |
const char * | typeName () const |
void * | voidStar () const |
![]() | |
ProxyBinding (KJS::ExecState *exec) | |
virtual | ~ProxyBinding () |
bool | implementsCall () const |
bool | implementsConstruct () const |
Static Public Member Functions | |
static KJS::JSValue * | propertyGetter (KJS::ExecState *exec, KJS::JSObject *, const KJS::Identifier &name, const KJS::PropertySlot &) |
static void | publishQObject (KJS::ExecState *exec, KJS::JSObject *target, QObject *object) |
Additional Inherited Members | |
![]() | |
static const KJS::ClassInfo | info = { "ObjectBinding", 0, 0, 0 } |
Detailed Description
Definition at line 79 of file qobject_binding.h.
Member Enumeration Documentation
Enumeration of access-flags that could be OR-combined to define what parts of the QObject should be published.
Default is AllSlots|AllSignals|AllProperties|AllObjects what means that everything got published, even e.g. private slots.
Definition at line 94 of file qobject_binding.h.
Constructor & Destructor Documentation
QObjectBinding::QObjectBinding | ( | KJS::ExecState * | exec, |
QObject * | object | ||
) |
Definition at line 229 of file qobject_binding.cpp.
|
virtual |
Definition at line 253 of file qobject_binding.cpp.
Member Function Documentation
QObjectBinding::AccessFlags QObjectBinding::access | ( | ) | const |
- Returns
- the defined Access flags.
Definition at line 312 of file qobject_binding.cpp.
bool QObjectBinding::canPut | ( | KJS::ExecState * | exec, |
const KJS::Identifier & | propertyName | ||
) | const |
- Returns
- true if the property
propertyName
can be changed else false is returned.
Definition at line 371 of file qobject_binding.cpp.
KJS::UString QObjectBinding::className | ( | ) | const |
- Returns
- the QObject's classname. For example for a QWidget-instance the string "QWidget" is returned.
Definition at line 387 of file qobject_binding.cpp.
bool QObjectBinding::getOwnPropertySlot | ( | KJS::ExecState * | exec, |
const KJS::Identifier & | propertyName, | ||
KJS::PropertySlot & | slot | ||
) |
Called to ask if we have a callback for the named property.
We return the callback in the property slot.
Definition at line 281 of file qobject_binding.cpp.
|
static |
Callback used to get properties.
Definition at line 297 of file qobject_binding.cpp.
|
static |
Definition at line 174 of file qobject_binding.cpp.
void QObjectBinding::put | ( | KJS::ExecState * | exec, |
const KJS::Identifier & | propertyName, | ||
KJS::JSValue * | value, | ||
int | attr = KJS::None |
||
) |
Set the value value
of the property propertyName
.
Definition at line 322 of file qobject_binding.cpp.
|
inline |
- Returns
- the internal object as a pointer to type T to the internal object that is derived from QObject.
Definition at line 177 of file qobject_binding.h.
void QObjectBinding::setAccess | ( | AccessFlags | access | ) |
Set the defined Access flags to access
.
Definition at line 317 of file qobject_binding.cpp.
KJS::UString QObjectBinding::toString | ( | KJS::ExecState * | exec | ) | const |
- Returns
- a string-representation of the QObject. For example for a QWidget-instance that has the QObject::objectName "mywidget" the string "mywidget (QWidget)" is returned.
Definition at line 392 of file qobject_binding.cpp.
void QObjectBinding::watchObject | ( | QObject * | object | ) |
Add the QObject object
to the internal QObjectCleanupHandler to watch the lifetime of the QObject to know when the QObject got deleted.
Definition at line 276 of file qobject_binding.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:53 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.