KNewStuff
#include <downloadmanager.h>
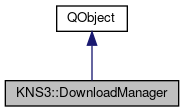
Public Types | |
enum | SortOrder { Newest, Alphabetical, Rating, Downloads } |
Signals | |
void | entryStatusChanged (const KNS3::Entry &entry) |
void | searchResult (const KNS3::Entry::List &entries) |
Public Member Functions | |
DownloadManager (QObject *parent=0) | |
DownloadManager (const QString &configFile, QObject *parent=0) | |
~DownloadManager () | |
void | checkForUpdates () |
void | installEntry (const KNS3::Entry &entry) |
void | search (int page=0, int pageSize=100) |
void | setSearchOrder (SortOrder order) |
void | setSearchTerm (const QString &searchTerm) |
void | uninstallEntry (const KNS3::Entry &entry) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
KNewStuff update checker.
This class can be used to search for KNewStuff items without using the widgets and to look for updates of already installed items without showing the dialog.
- Since
- 4.5
Definition at line 34 of file downloadmanager.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Newest | |
Alphabetical | |
Rating | |
Downloads |
Definition at line 39 of file downloadmanager.h.
Constructor & Destructor Documentation
|
explicit |
Create a DownloadManager It will try to find a appname.knsrc file (using KComponentData).
Appname is the name of your application as provided in the about data->
- Parameters
-
parent the parent of the dialog
Definition at line 61 of file downloadmanager.cpp.
Create a DownloadManager.
Manually specifying the name of the .knsrc file.
- Parameters
-
configFile the name of the configuration file parent
Definition at line 70 of file downloadmanager.cpp.
DownloadManager::~DownloadManager | ( | ) |
destructor
Definition at line 86 of file downloadmanager.cpp.
Member Function Documentation
void DownloadManager::checkForUpdates | ( | ) |
Check for available updates.
Use searchResult to get notified as soon as an update has been found.
Definition at line 102 of file downloadmanager.cpp.
|
signal |
The entry status has changed: emitted when the entry has been installed, updated or removed.
Use KNS3::Entry::status() to check the current status.
- Parameters
-
entry the item that has been updated.
void DownloadManager::installEntry | ( | const KNS3::Entry & | entry | ) |
void DownloadManager::search | ( | int | page = 0 , |
int | pageSize = 100 |
||
) |
Search for a list of entries.
searchResult will be emitted with the requested list.
Definition at line 141 of file downloadmanager.cpp.
|
signal |
Returns the search result.
This can be the list of updates after checkForUpdates or the result of a search.
- Parameters
-
entries the list of results. entries is empty when nothing was found.
void DownloadManager::setSearchOrder | ( | DownloadManager::SortOrder | order | ) |
Set the sort order of the results.
This depends on the server. Note that this function does not trigger a search. Use search after setting this.
- See also
- SortOrder
- Parameters
-
order
Definition at line 153 of file downloadmanager.cpp.
void DownloadManager::setSearchTerm | ( | const QString & | searchTerm | ) |
Sets the search term to filter the results on the server.
Note that this function does not trigger a search. Use search after setting this.
- Parameters
-
searchTerm
Definition at line 171 of file downloadmanager.cpp.
void DownloadManager::uninstallEntry | ( | const KNS3::Entry & | entry | ) |
Uninstalls the given entry.
- Parameters
-
entry The entry which will be uninstalled.
- Since
- 4.7
Definition at line 133 of file downloadmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:25:44 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.