KNewStuff
QAsyncImage Class Reference
#include <qasyncimage_p.h>
Inheritance diagram for QAsyncImage:
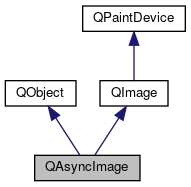
Signals | |
void | signalLoaded (const QString &url, const QImage &pix) |
Public Member Functions | |
QAsyncImage (const QString &url, QObject *parent) | |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
QImage () | |
QImage (const QSize &size, Format format) | |
QImage (uchar *data, int width, int height, Format format) | |
QImage (const char *const [] xpm) | |
QImage (const QString &fileName, const char *format) | |
QImage (const uchar *data, int width, int height, Format format) | |
QImage (const char *fileName, const char *format) | |
QImage (const QImage &image) | |
QImage (int width, int height, Format format) | |
QImage (uchar *data, int width, int height, int bytesPerLine, Format format) | |
QImage (int width, int height, int depth, int numColors, Endian bitOrder) | |
QImage (const QSize &size, int depth, int numColors, Endian bitOrder) | |
QImage (uchar *data, int width, int height, int depth, const QRgb *colortable, int numColors, Endian bitOrder) | |
QImage (uchar *data, int width, int height, int depth, int bytesPerLine, const QRgb *colortable, int numColors, Endian bitOrder) | |
QImage (const uchar *data, int width, int height, int bytesPerLine, Format format) | |
QImage (const QByteArray &data) | |
~QImage () | |
bool | allGray () const |
QImage | alphaChannel () const |
Endian | bitOrder () const |
int | bitPlaneCount () const |
uchar * | bits () |
const uchar * | bits () const |
int | byteCount () const |
int | bytesPerLine () const |
qint64 | cacheKey () const |
QRgb | color (int i) const |
int | colorCount () const |
QVector< QRgb > | colorTable () const |
const uchar * | constBits () const |
const uchar * | constScanLine (int i) const |
QImage | convertBitOrder (Endian bitOrder) const |
QImage | convertDepth (int depth, QFlags< Qt::ImageConversionFlag > flags) const |
QImage | convertDepthWithPalette (int depth, QRgb *palette, int palette_count, QFlags< Qt::ImageConversionFlag > flags) const |
QImage | convertToFormat (Format format, QFlags< Qt::ImageConversionFlag > flags) const |
QImage | convertToFormat (Format format, const QVector< QRgb > &colorTable, QFlags< Qt::ImageConversionFlag > flags) const |
QImage | copy (const QRect &rectangle) const |
QImage | copy (int x, int y, int width, int height) const |
QImage | copy (const QRect &rect, QFlags< Qt::ImageConversionFlag > flags) const |
QImage | copy (int x, int y, int w, int h, QFlags< Qt::ImageConversionFlag > flags) const |
bool | create (const QSize &size, int depth, int numColors, Endian bitOrder) |
bool | create (int width, int height, int depth, int numColors, Endian bitOrder) |
QImage | createAlphaMask (QFlags< Qt::ImageConversionFlag > flags) const |
QImage | createHeuristicMask (bool clipTight) const |
QImage | createMaskFromColor (QRgb color, Qt::MaskMode mode) const |
int | depth () const |
int | dotsPerMeterX () const |
int | dotsPerMeterY () const |
void | fill (uint pixelValue) |
void | fill (const QColor &color) |
void | fill (Qt::GlobalColor color) |
Format | format () const |
bool | hasAlphaBuffer () const |
bool | hasAlphaChannel () const |
int | height () const |
void | invertPixels (InvertMode mode) |
void | invertPixels (bool invertAlpha) |
bool | isGrayscale () const |
bool | isNull () const |
uchar ** | jumpTable () |
const uchar *const * | jumpTable () const |
bool | load (QIODevice *device, const char *format) |
bool | load (const QString &fileName, const char *format) |
bool | loadFromData (const QByteArray &data, const char *format) |
bool | loadFromData (const uchar *data, int len, const char *format) |
QImage | mirror (bool horizontal, bool vertical) const |
QImage | mirrored (bool horizontal, bool vertical) const |
int | numBytes () const |
int | numColors () const |
QPoint | offset () const |
operator QVariant () const | |
bool | operator!= (const QImage &image) const |
QImage & | operator= (const QImage &image) |
bool | operator== (const QImage &image) const |
QRgb | pixel (int x, int y) const |
QRgb | pixel (const QPoint &position) const |
int | pixelIndex (const QPoint &position) const |
int | pixelIndex (int x, int y) const |
QRect | rect () const |
void | reset () |
QImage | rgbSwapped () const |
bool | save (const QString &fileName, const char *format, int quality) const |
bool | save (QIODevice *device, const char *format, int quality) const |
QImage | scaled (int width, int height, Qt::AspectRatioMode aspectRatioMode, Qt::TransformationMode transformMode) const |
QImage | scaled (const QSize &size, Qt::AspectRatioMode aspectRatioMode, Qt::TransformationMode transformMode) const |
QImage | scaledToHeight (int height, Qt::TransformationMode mode) const |
QImage | scaledToWidth (int width, Qt::TransformationMode mode) const |
QImage | scaleHeight (int h) const |
QImage | scaleWidth (int w) const |
const uchar * | scanLine (int i) const |
uchar * | scanLine (int i) |
int | serialNumber () const |
void | setAlphaBuffer (bool enable) |
void | setAlphaChannel (const QImage &alphaChannel) |
void | setColor (int index, QRgb colorValue) |
void | setColorCount (int colorCount) |
void | setColorTable (const QVector< QRgb > colors) |
void | setDotsPerMeterX (int x) |
void | setDotsPerMeterY (int y) |
void | setNumColors (int numColors) |
void | setOffset (const QPoint &offset) |
void | setPixel (int x, int y, uint index_or_rgb) |
void | setPixel (const QPoint &position, uint index_or_rgb) |
void | setText (const char *key, const char *language, const QString &text) |
void | setText (const QString &key, const QString &text) |
QSize | size () const |
QImage | smoothScale (const QSize &size, Qt::AspectRatioMode mode) const |
QImage | smoothScale (int width, int height, Qt::AspectRatioMode mode) const |
void | swap (QImage &other) |
QImage | swapRGB () const |
QString | text (const QImageTextKeyLang &keywordAndLanguage) const |
QString | text (const char *key, const char *language) const |
QString | text (const QString &key) const |
QStringList | textKeys () const |
QStringList | textLanguages () const |
QList< QImageTextKeyLang > | textList () const |
QImage | transformed (const QTransform &matrix, Qt::TransformationMode mode) const |
QImage | transformed (const QMatrix &matrix, Qt::TransformationMode mode) const |
bool | valid (int x, int y) const |
bool | valid (const QPoint &pos) const |
int | width () const |
QImage | xForm (const QMatrix &matrix) const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QImage | fromData (const uchar *data, int size, const char *format) |
QImage | fromData (const QByteArray &data, const char *format) |
Endian | systemBitOrder () |
Endian | systemByteOrder () |
QMatrix | trueMatrix (const QMatrix &matrix, int width, int height) |
QTransform | trueMatrix (const QTransform &matrix, int width, int height) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
virtual int | metric (PaintDeviceMetric metric) const |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Convenience class for images with remote sources.
This class represents a fire-and-forget approach of loading images in applications. The image will load itself. Using this class also requires using QAsyncFrame or similar UI elements which allow for asynchronous image loading.
This class is used internally by the DownloadDialog class.
Definition at line 44 of file qasyncimage_p.h.
Constructor & Destructor Documentation
Definition at line 30 of file qasyncimage.cpp.
Member Function Documentation
The documentation for this class was generated from the following files:
This file is part of the KDE documentation.
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:25:43 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:25:43 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.