KParts
#include <partmanager.h>
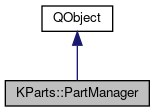
Public Types | |
enum | Reason { ReasonLeftClick = 100, ReasonMidClick, ReasonRightClick, NoReason } |
enum | SelectionPolicy { Direct, TriState } |
Signals | |
void | activePartChanged (KParts::Part *newPart) |
void | partAdded (KParts::Part *part) |
void | partRemoved (KParts::Part *part) |
Public Member Functions | |
PartManager (QWidget *parent) | |
PartManager (QWidget *topLevel, QObject *parent) | |
virtual | ~PartManager () |
short int | activationButtonMask () const |
virtual Part * | activePart () const |
virtual QWidget * | activeWidget () const |
void | addManagedTopLevelWidget (const QWidget *topLevel) |
virtual void | addPart (Part *part, bool setActive=true) |
bool | allowNestedParts () const |
virtual bool | eventFilter (QObject *obj, QEvent *ev) |
bool | ignoreScrollBars () const |
const QList< Part * > | parts () const |
int | reason () const |
void | removeManagedTopLevelWidget (const QWidget *topLevel) |
virtual void | removePart (Part *part) |
virtual void | replacePart (Part *oldPart, Part *newPart, bool setActive=true) |
virtual Part * | selectedPart () const |
virtual QWidget * | selectedWidget () const |
SelectionPolicy | selectionPolicy () const |
void | setActivationButtonMask (short int buttonMask) |
virtual void | setActivePart (Part *part, QWidget *widget=0) |
void | setAllowNestedParts (bool allow) |
void | setIgnoreScrollBars (bool ignore) |
virtual void | setSelectedPart (Part *part, QWidget *widget=0) |
void | setSelectionPolicy (SelectionPolicy policy) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Slots | |
void | slotManagedTopLevelWidgetDestroyed () |
void | slotObjectDestroyed () |
void | slotWidgetDestroyed () |
Protected Member Functions | |
virtual void | setActiveComponent (const KComponentData &instance) |
void | setIgnoreExplictFocusRequests (bool) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Properties | |
bool | allowNestedParts |
bool | ignoreScrollBars |
SelectionPolicy | selectionPolicy |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
The part manager is an object which knows about a collection of parts (even nested ones) and handles activation/deactivation.
Applications that want to embed parts without merging GUIs only use a KParts::PartManager. Those who want to merge GUIs use a KParts::MainWindow for example, in addition to a part manager.
Parts know about the part manager to add nested parts to it. See also KParts::Part::manager() and KParts::Part::setManager().
Definition at line 47 of file partmanager.h.
Member Enumeration Documentation
This extends QFocusEvent::Reason with the non-focus-event reasons for partmanager to activate a part.
To test for "any focusin reason", use < ReasonLeftClick NoReason usually means: explicit activation with setActivePart.
Enumerator | |
---|---|
ReasonLeftClick | |
ReasonMidClick | |
ReasonRightClick | |
NoReason |
Definition at line 63 of file partmanager.h.
Selection policy. The default policy of a PartManager is Direct.
Enumerator | |
---|---|
Direct | |
TriState |
Definition at line 56 of file partmanager.h.
Constructor & Destructor Documentation
PartManager::PartManager | ( | QWidget * | parent | ) |
Constructs a part manager.
- Parameters
-
parent The toplevel widget (window / dialog) the partmanager should monitor for activation/selection events
Definition at line 105 of file partmanager.cpp.
Constructs a part manager.
- Parameters
-
topLevel The toplevel widget (window / dialog ) the partmanager should monitor for activation/selection events parent The parent QObject.
Definition at line 116 of file partmanager.cpp.
|
virtual |
Definition at line 127 of file partmanager.cpp.
Member Function Documentation
short int PartManager::activationButtonMask | ( | ) | const |
- See also
- setActivationButtonMask
Definition at line 180 of file partmanager.cpp.
|
virtual |
Returns the active part.
Definition at line 501 of file partmanager.cpp.
|
signal |
Emitted when the active part has changed.
- See also
- setActivePart()
|
virtual |
Returns the active widget of the current active part (see activePart ).
Definition at line 506 of file partmanager.cpp.
void PartManager::addManagedTopLevelWidget | ( | const QWidget * | topLevel | ) |
Adds the topLevel
widget to the list of managed toplevel widgets.
Usually a PartManager only listens for events (for activation/selection) for one toplevel widget (and its children) , the one specified in the constructor. Sometimes however (like for example when using the KDE dockwidget library) , it is necessary to extend this.
Definition at line 569 of file partmanager.cpp.
Adds a part to the manager.
Sets it to the active part automatically if setActive
is true (default ). Behavior fix in KDE3.4: the part's widget is shown only if setActive is true, it used to be shown in all cases before.
Definition at line 340 of file partmanager.cpp.
bool KParts::PartManager::allowNestedParts | ( | ) | const |
- See also
- setAllowNestedParts
Reimplemented from QObject.
Definition at line 185 of file partmanager.cpp.
bool KParts::PartManager::ignoreScrollBars | ( | ) | const |
- See also
- setIgnoreScrollBars
|
signal |
Emitted when a new part has been added.
- See also
- addPart()
|
signal |
Emitted when a part has been removed.
- See also
- removePart()
Returns the list of parts being managed by the partmanager.
Definition at line 564 of file partmanager.cpp.
int PartManager::reason | ( | ) | const |
- Returns
- the reason for the last activePartChanged signal emitted.
- See also
- Reason
Definition at line 596 of file partmanager.cpp.
void PartManager::removeManagedTopLevelWidget | ( | const QWidget * | topLevel | ) |
Removes the topLevel
widget from the list of managed toplevel widgets.
- See also
- addManagedTopLevelWidget
Definition at line 582 of file partmanager.cpp.
|
virtual |
Removes a part from the manager (this does not delete the object) .
Sets the active part to 0 if part
is the activePart() .
Definition at line 378 of file partmanager.cpp.
Replaces oldPart
with newPart
, and sets newPart
as active if setActive
is true.
This is an optimised version of removePart + addPart
Definition at line 397 of file partmanager.cpp.
|
virtual |
Returns the current selected part.
Definition at line 540 of file partmanager.cpp.
|
virtual |
Returns the selected widget of the current selected part (see selectedPart ).
Definition at line 545 of file partmanager.cpp.
SelectionPolicy KParts::PartManager::selectionPolicy | ( | ) | const |
Returns the current selection policy.
void PartManager::setActivationButtonMask | ( | short int | buttonMask | ) |
Specifies which mouse buttons the partmanager should react upon.
By default it reacts on all mouse buttons (LMB/MMB/RMB).
- Parameters
-
buttonMask a combination of Qt::ButtonState values e.g. Qt::LeftButton | Qt::MidButton
Definition at line 175 of file partmanager.cpp.
|
protectedvirtual |
Changes the active instance when the active part changes.
The active instance is used by KBugReport and KAboutDialog. Override if you really need to - usually you don't need to.
Definition at line 495 of file partmanager.cpp.
Sets the active part.
The active part receives activation events.
widget
can be used to specify which widget was responsible for the activation. This is important if you have multiple views for a document/part , like in KOffice .
Definition at line 415 of file partmanager.cpp.
void PartManager::setAllowNestedParts | ( | bool | allow | ) |
Specifies whether the partmanager should handle/allow nested parts or not.
This is a property the shell has to set/specify. Per default we assume that the shell cannot handle nested parts. However in case of a KOffice shell for example we allow nested parts. A Part is nested (a child part) if its parent object inherits KParts::Part. If a child part is activated and nested parts are not allowed/handled, then the top parent part in the tree is activated.
Definition at line 155 of file partmanager.cpp.
|
protected |
Sets whether the PartManager ignores explict set focus requests from the part.
By default this option is set to false. Set it to true to prevent the part from sending explicit set focus requests to the client application.
- Since
- 4.10
Definition at line 601 of file partmanager.cpp.
void PartManager::setIgnoreScrollBars | ( | bool | ignore | ) |
Specifies whether the partmanager should ignore mouse click events for scrollbars or not.
If the partmanager ignores them, then clicking on the scrollbars of a non-active/non-selected part will not change the selection or activation state.
The default value is false (read: scrollbars are NOT ignored).
Definition at line 165 of file partmanager.cpp.
Sets the selected part.
The selected part receives selection events.
widget
can be used to specify which widget was responsible for the selection. This is important if you have multiple views for a document/part , like in KOffice .
Definition at line 511 of file partmanager.cpp.
void PartManager::setSelectionPolicy | ( | SelectionPolicy | policy | ) |
Sets the selection policy of the partmanager.
Definition at line 145 of file partmanager.cpp.
|
protectedslot |
Definition at line 590 of file partmanager.cpp.
|
protectedslot |
Removes a part when it is destroyed.
Definition at line 550 of file partmanager.cpp.
|
protectedslot |
Definition at line 556 of file partmanager.cpp.
Property Documentation
|
readwrite |
Definition at line 52 of file partmanager.h.
|
readwrite |
Definition at line 53 of file partmanager.h.
|
readwrite |
Definition at line 51 of file partmanager.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:25:36 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.