Kross
Kross::FormModule Class Reference
#include <form.h>
Inheritance diagram for Kross::FormModule:
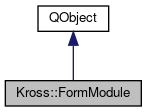
Public Member Functions | |
FormModule () | |
virtual | ~FormModule () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
The FormModule provides access to UI functionality like dialogs or widgets.
Example (in Python) :
import Kross
forms = Kross.module("forms")
dialog = forms.createDialog("My Dialog")
dialog.setButtons("Ok|Cancel")
page = dialog.addPage("Welcome","Welcome Page","document-open")
label = forms.createWidget(page,"QLabel")
label.text = "Hello World Label"
if dialog.exec_loop():
forms.showMessageBox("Information", "Okay...", "The Ok-button was pressed")
Constructor & Destructor Documentation
Member Function Documentation
|
slot |
|
slot |
Create and return a new FormAssistant instance.
- Parameters
-
caption The displayed caption of the dialog.
Create and return a new FormDialog instance.
- Parameters
-
caption The displayed caption of the dialog.
|
slot |
Create and return a new FormFileWidget instance.
- Parameters
-
parent the parent QWidget the new FormFileWidget instance is a child of. startDirOrVariable the start-directory or -variable.
- Returns
- the new FormFileWidget instance or NULL.
Create and return a new FormListView instance.
- Parameters
-
parent the parent QWidget the new FormListView instance is a child of.
- Returns
- the new FormFileWidget instance or NULL.
|
slot |
|
slot |
Show a messagebox.
- Parameters
-
dialogtype The type of the dialog which could be one of the following; - Sorry
- Error
- Information
- QuestionYesNo
- WarningYesNo
- WarningContinueCancel
- WarningYesNoCancel
- QuestionYesNoCancel
caption The caption the messagedialog displays. message The message that is displayed in the messagedialog. details The optional details
- Returns
- The buttoncode which chould be one of the following;
- Ok
- Cancel
- Yes
- No
- Continue
|
slot |
Show a progressdialog to provide visible feedback on the progress of a slow operation.
- Parameters
-
caption The caption the progressdialog displays. labelText The displayed label.
- Returns
- The QProgressDialog widget instance.
The documentation for this class was generated from the following files:
This file is part of the KDE documentation.
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:44 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:44 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.