Plasma
#include <Plasma/Scripting/AppletScript>
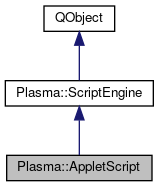
Public Slots | |
virtual void | configChanged () |
virtual void | showConfigurationInterface () |
Signals | |
void | popupEvent (bool popped) const |
void | saveState (KConfigGroup &group) const |
Public Member Functions | |
AppletScript (QObject *parent=0) | |
~AppletScript () | |
Plasma::Applet * | applet () const |
void | configNeedsSaving () const |
virtual void | constraintsEvent (Plasma::Constraints constraints) |
Containment::Type | containmentType () const |
virtual QList< QAction * > | contextualActions () |
bool | drawWallpaper () const |
Extender * | extender () const |
virtual void | paintInterface (QPainter *painter, const QStyleOptionGraphicsItem *option, const QRect &contentsRect) |
void | setApplet (Plasma::Applet *applet) |
void | setConfigurationRequired (bool req, const QString &reason=QString()) |
void | setContainmentType (Containment::Type type) |
void | setDrawWallpaper (bool drawWallpaper) |
void | setFailedToLaunch (bool failed, const QString &reason=QString()) |
void | setHasConfigurationInterface (bool hasInterface) |
virtual QPainterPath | shape () const |
Q_INVOKABLE QSizeF | size () const |
![]() | |
~ScriptEngine () | |
virtual bool | init () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
void | addStandardConfigurationPages (KConfigDialog *dialog) |
Q_INVOKABLE DataEngine * | dataEngine (const QString &engine) const |
KPluginInfo | description () const |
bool | isRegisteredAsDragHandle (QGraphicsItem *item) |
Animation * | loadAnimationFromPackage (const QString &name, QObject *parent) |
QString | mainScript () const |
const Package * | package () const |
void | registerAsDragHandle (QGraphicsItem *item) |
void | showMessage (const QIcon &icon, const QString &message, const MessageButtons buttons) |
KConfigDialog * | standardConfigurationDialog () |
void | unregisterAsDragHandle (QGraphicsItem *item) |
![]() | |
ScriptEngine (QObject *parent=0) | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Provides a restricted interface for scripted applets.
Definition at line 51 of file appletscript.h.
Constructor & Destructor Documentation
|
explicit |
Default constructor for an AppletScript.
Subclasses should not attempt to access the Plasma::Applet associated with this AppletScript in the constructor. All such set up that requires the Applet itself should be done in the init() method.
Definition at line 40 of file appletscript.cpp.
Plasma::AppletScript::~AppletScript | ( | ) |
Definition at line 47 of file appletscript.cpp.
Member Function Documentation
|
protected |
This method should be called after a scripting applet has added its own pages to a configuration dialog.
- Since
- 4.3.1
Definition at line 148 of file appletscript.cpp.
Applet * Plasma::AppletScript::applet | ( | ) | const |
Returns the Plasma::Applet associated with this script component.
Definition at line 57 of file appletscript.cpp.
|
virtualslot |
Configure was changed.
Definition at line 224 of file appletscript.cpp.
void Plasma::AppletScript::configNeedsSaving | ( | ) | const |
- See also
- Applet
Definition at line 123 of file appletscript.cpp.
|
virtual |
Called when any of the geometry constraints have been updated.
This is always called prior to painting and should be used as an opportunity to layout the widget, calculate sizings, etc.
Do not call update() from this method; an update() will be triggered at the appropriate time for the applet.
- Parameters
-
constraints the type of constraints that were updated
Definition at line 81 of file appletscript.cpp.
Containment::Type Plasma::AppletScript::containmentType | ( | ) | const |
Returns a list of context-related QAction instances.
- Returns
- A list of actions. The default implementation returns an empty list.
Definition at line 86 of file appletscript.cpp.
|
protected |
- Parameters
-
engine name of the engine
- Returns
- a data engine associated with this plasmoid
Definition at line 228 of file appletscript.cpp.
|
protected |
- Returns
- the KPluginInfo associated with this plasmoid
Definition at line 246 of file appletscript.cpp.
bool Plasma::AppletScript::drawWallpaper | ( | ) | const |
- Returns
- true if the applet is a containment AND if the wallpaper is enabled
- See also
- Containment
- Since
- 4.7
Definition at line 258 of file appletscript.cpp.
Extender * Plasma::AppletScript::extender | ( | ) | const |
- See also
- Applet
Definition at line 252 of file appletscript.cpp.
|
protected |
- See also
- Applet
Definition at line 176 of file appletscript.cpp.
|
protected |
Loads an animation from the applet package.
- Parameters
-
animation the animation to load
- Returns
- an Animation object on success, a NULL pointer on failure
- Since
- 4.5
Definition at line 184 of file appletscript.cpp.
|
protectedvirtual |
- Returns
- absolute path to the main script file for this plasmoid
Reimplemented from Plasma::ScriptEngine.
Definition at line 234 of file appletscript.cpp.
|
protectedvirtual |
- Returns
- the Package associated with this plasmoid which can be used to request resources, such as images and interface files.
Reimplemented from Plasma::ScriptEngine.
Definition at line 240 of file appletscript.cpp.
|
virtual |
Called when the script should paint the applet.
- Parameters
-
painter the QPainter to use option the style option containing such flags as selection, level of detail, etc contentsRect the rect to paint within; automatically adjusted for the background, if any
Definition at line 63 of file appletscript.cpp.
|
signal |
- See also
- PopupApplet
|
protected |
- See also
- Applet
Definition at line 162 of file appletscript.cpp.
|
signal |
- See also
- Applet
void Plasma::AppletScript::setApplet | ( | Plasma::Applet * | applet | ) |
Sets the applet associated with this AppletScript.
Definition at line 52 of file appletscript.cpp.
void Plasma::AppletScript::setConfigurationRequired | ( | bool | req, |
const QString & | reason = QString() |
||
) |
- See also
- Applet
Definition at line 109 of file appletscript.cpp.
void Plasma::AppletScript::setContainmentType | ( | Containment::Type | type | ) |
void Plasma::AppletScript::setDrawWallpaper | ( | bool | drawWallpaper | ) |
set if the containment draws its own wallpaper: it has no effect if the applet is not a containment
- See also
- Containment
- Since
- 4.7
Definition at line 269 of file appletscript.cpp.
- See also
- Applet
Definition at line 116 of file appletscript.cpp.
void Plasma::AppletScript::setHasConfigurationInterface | ( | bool | hasInterface | ) |
Sets whether or not this script has a configuration interface or not.
- Parameters
-
hasInterface true if the applet is user configurable
Definition at line 102 of file appletscript.cpp.
|
virtual |
Returns the shape of the widget, defaults to the bounding rect.
Definition at line 91 of file appletscript.cpp.
|
virtualslot |
Show a configuration dialog.
Definition at line 130 of file appletscript.cpp.
|
protected |
- See also
- Applet
Definition at line 155 of file appletscript.cpp.
QSizeF Plasma::AppletScript::size | ( | ) | const |
Returns the area within which contents can be painted.
Definition at line 72 of file appletscript.cpp.
|
protected |
- Returns
- a standard Plasma applet configuration dialog, ready to have pages added to it.
Note that the dialog returned is set to delete on close.
Definition at line 139 of file appletscript.cpp.
|
protected |
- See also
- Applet
Definition at line 169 of file appletscript.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:23:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.