Plasma
#include <extenderitem.h>
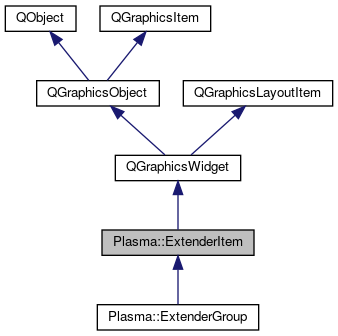
Public Slots | |
void | destroy () |
void | hideCloseButton () |
void | returnToSource () |
void | setCollapsed (bool collapsed) |
void | showCloseButton () |
Signals | |
void | destroyed (Plasma::ExtenderItem *item) |
Public Member Functions | |
ExtenderItem (Extender *hostExtender, uint extenderItemId=0) | |
~ExtenderItem () | |
QAction * | action (const QString &name) const |
void | addAction (const QString &name, QAction *action) |
uint | autoExpireDelay () const |
KConfigGroup | config () const |
Extender * | extender () const |
ExtenderGroup * | group () const |
QIcon | icon () const |
bool | isCollapsed () const |
bool | isDetached () const |
bool | isGroup () const |
bool | isTransient () const |
QString | name () const |
void | setAutoExpireDelay (uint time) |
void | setExtender (Extender *extender, const QPointF &pos=QPointF(-1,-1)) |
void | setGroup (ExtenderGroup *group) |
void | setGroup (ExtenderGroup *group, const QPointF &pos) |
void | setIcon (const QString &icon) |
void | setIcon (const QIcon &icon) |
void | setName (const QString &name) |
void | setTitle (const QString &title) |
void | setTransient (const bool transient) |
void | setWidget (QGraphicsItem *widget) |
QString | title () const |
QGraphicsItem * | widget () const |
![]() | |
QGraphicsWidget (QGraphicsItem *parent, QFlags< Qt::WindowType > wFlags) | |
~QGraphicsWidget () | |
QList< QAction * > | actions () const |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
virtual QRectF | boundingRect () const |
bool | close () |
Qt::FocusPolicy | focusPolicy () const |
QGraphicsWidget * | focusWidget () const |
QFont | font () const |
void | geometryChanged () |
virtual void | getContentsMargins (qreal *left, qreal *top, qreal *right, qreal *bottom) const |
void | getWindowFrameMargins (qreal *left, qreal *top, qreal *right, qreal *bottom) const |
int | grabShortcut (const QKeySequence &sequence, Qt::ShortcutContext context) |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
QGraphicsLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
virtual void | paintWindowFrame (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) |
QPalette | palette () const |
QRectF | rect () const |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | resize (const QSizeF &size) |
void | resize (qreal w, qreal h) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setContentsMargins (qreal left, qreal top, qreal right, qreal bottom) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFont (const QFont &font) |
virtual void | setGeometry (const QRectF &rect) |
void | setGeometry (qreal x, qreal y, qreal w, qreal h) |
void | setLayout (QGraphicsLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setPalette (const QPalette &palette) |
void | setShortcutAutoRepeat (int id, bool enabled) |
void | setShortcutEnabled (int id, bool enabled) |
void | setStyle (QStyle *style) |
void | setWindowFlags (QFlags< Qt::WindowType > wFlags) |
void | setWindowFrameMargins (qreal left, qreal top, qreal right, qreal bottom) |
void | setWindowTitle (const QString &title) |
virtual QPainterPath | shape () const |
QSizeF | size () const |
QStyle * | style () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
virtual int | type () const |
void | unsetLayoutDirection () |
void | unsetWindowFrameMargins () |
Qt::WindowFlags | windowFlags () const |
QRectF | windowFrameGeometry () const |
QRectF | windowFrameRect () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
![]() | |
QGraphicsObject (QGraphicsItem *parent) | |
void | enabledChanged () |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | opacityChanged () |
void | parentChanged () |
void | rotationChanged () |
void | scaleChanged () |
void | ungrabGesture (Qt::GestureType gesture) |
void | visibleChanged () |
void | xChanged () |
void | yChanged () |
void | zChanged () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
QGraphicsItem (QGraphicsItem *parent) | |
virtual | ~QGraphicsItem () |
bool | acceptDrops () const |
Qt::MouseButtons | acceptedMouseButtons () const |
bool | acceptHoverEvents () const |
bool | acceptsHoverEvents () const |
bool | acceptTouchEvents () const |
virtual void | advance (int phase) |
virtual QRectF | boundingRect () const =0 |
QRegion | boundingRegion (const QTransform &itemToDeviceTransform) const |
qreal | boundingRegionGranularity () const |
CacheMode | cacheMode () const |
QList< QGraphicsItem * > | childItems () const |
QList< QGraphicsItem * > | children () const |
QRectF | childrenBoundingRect () const |
void | clearFocus () |
QPainterPath | clipPath () const |
virtual bool | collidesWithItem (const QGraphicsItem *other, Qt::ItemSelectionMode mode) const |
virtual bool | collidesWithPath (const QPainterPath &path, Qt::ItemSelectionMode mode) const |
QList< QGraphicsItem * > | collidingItems (Qt::ItemSelectionMode mode) const |
QGraphicsItem * | commonAncestorItem (const QGraphicsItem *other) const |
virtual bool | contains (const QPointF &point) const |
QCursor | cursor () const |
QVariant | data (int key) const |
QTransform | deviceTransform (const QTransform &viewportTransform) const |
qreal | effectiveOpacity () const |
void | ensureVisible (const QRectF &rect, int xmargin, int ymargin) |
void | ensureVisible (qreal x, qreal y, qreal w, qreal h, int xmargin, int ymargin) |
bool | filtersChildEvents () const |
GraphicsItemFlags | flags () const |
QGraphicsItem * | focusItem () const |
QGraphicsItem * | focusProxy () const |
void | grabKeyboard () |
void | grabMouse () |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsItemGroup * | group () const |
bool | handlesChildEvents () const |
bool | hasCursor () const |
bool | hasFocus () const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const |
void | installSceneEventFilter (QGraphicsItem *filterItem) |
bool | isActive () const |
bool | isAncestorOf (const QGraphicsItem *child) const |
bool | isBlockedByModalPanel (QGraphicsItem **blockingPanel) const |
bool | isClipped () const |
bool | isEnabled () const |
bool | isObscured () const |
bool | isObscured (const QRectF &rect) const |
bool | isObscured (qreal x, qreal y, qreal w, qreal h) const |
virtual bool | isObscuredBy (const QGraphicsItem *item) const |
bool | isPanel () const |
bool | isSelected () const |
bool | isUnderMouse () const |
bool | isVisible () const |
bool | isVisibleTo (const QGraphicsItem *parent) const |
bool | isWidget () const |
bool | isWindow () const |
QTransform | itemTransform (const QGraphicsItem *other, bool *ok) const |
QPointF | mapFromItem (const QGraphicsItem *item, const QPointF &point) const |
QPolygonF | mapFromItem (const QGraphicsItem *item, const QRectF &rect) const |
QPolygonF | mapFromItem (const QGraphicsItem *item, const QPolygonF &polygon) const |
QPainterPath | mapFromItem (const QGraphicsItem *item, const QPainterPath &path) const |
QPointF | mapFromItem (const QGraphicsItem *item, qreal x, qreal y) const |
QPolygonF | mapFromItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const |
QPointF | mapFromParent (const QPointF &point) const |
QPolygonF | mapFromParent (const QRectF &rect) const |
QPolygonF | mapFromParent (const QPolygonF &polygon) const |
QPainterPath | mapFromParent (const QPainterPath &path) const |
QPointF | mapFromParent (qreal x, qreal y) const |
QPolygonF | mapFromParent (qreal x, qreal y, qreal w, qreal h) const |
QPolygonF | mapFromScene (const QPolygonF &polygon) const |
QPointF | mapFromScene (const QPointF &point) const |
QPolygonF | mapFromScene (const QRectF &rect) const |
QPainterPath | mapFromScene (const QPainterPath &path) const |
QPointF | mapFromScene (qreal x, qreal y) const |
QPolygonF | mapFromScene (qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectFromItem (const QGraphicsItem *item, const QRectF &rect) const |
QRectF | mapRectFromItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectFromParent (const QRectF &rect) const |
QRectF | mapRectFromParent (qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectFromScene (qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectFromScene (const QRectF &rect) const |
QRectF | mapRectToItem (const QGraphicsItem *item, const QRectF &rect) const |
QRectF | mapRectToItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectToParent (const QRectF &rect) const |
QRectF | mapRectToParent (qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectToScene (const QRectF &rect) const |
QRectF | mapRectToScene (qreal x, qreal y, qreal w, qreal h) const |
QPointF | mapToItem (const QGraphicsItem *item, const QPointF &point) const |
QPolygonF | mapToItem (const QGraphicsItem *item, const QRectF &rect) const |
QPolygonF | mapToItem (const QGraphicsItem *item, const QPolygonF &polygon) const |
QPainterPath | mapToItem (const QGraphicsItem *item, const QPainterPath &path) const |
QPointF | mapToItem (const QGraphicsItem *item, qreal x, qreal y) const |
QPolygonF | mapToItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const |
QPointF | mapToParent (const QPointF &point) const |
QPolygonF | mapToParent (const QRectF &rect) const |
QPainterPath | mapToParent (const QPainterPath &path) const |
QPointF | mapToParent (qreal x, qreal y) const |
QPolygonF | mapToParent (qreal x, qreal y, qreal w, qreal h) const |
QPolygonF | mapToParent (const QPolygonF &polygon) const |
QPolygonF | mapToScene (const QRectF &rect) const |
QPainterPath | mapToScene (const QPainterPath &path) const |
QPointF | mapToScene (qreal x, qreal y) const |
QPolygonF | mapToScene (qreal x, qreal y, qreal w, qreal h) const |
QPolygonF | mapToScene (const QPolygonF &polygon) const |
QPointF | mapToScene (const QPointF &point) const |
QMatrix | matrix () const |
void | moveBy (qreal dx, qreal dy) |
qreal | opacity () const |
virtual QPainterPath | opaqueArea () const |
virtual void | paint (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget)=0 |
QGraphicsItem * | panel () const |
PanelModality | panelModality () const |
QGraphicsItem * | parentItem () const |
QGraphicsObject * | parentObject () const |
QGraphicsWidget * | parentWidget () const |
QPointF | pos () const |
void | removeSceneEventFilter (QGraphicsItem *filterItem) |
void | resetMatrix () |
void | resetTransform () |
void | rotate (qreal angle) |
qreal | rotation () const |
void | scale (qreal sx, qreal sy) |
qreal | scale () const |
QGraphicsScene * | scene () const |
QRectF | sceneBoundingRect () const |
QMatrix | sceneMatrix () const |
QPointF | scenePos () const |
QTransform | sceneTransform () const |
void | scroll (qreal dx, qreal dy, const QRectF &rect) |
void | setAcceptDrops (bool on) |
void | setAcceptedMouseButtons (QFlags< Qt::MouseButton > buttons) |
void | setAcceptHoverEvents (bool enabled) |
void | setAcceptsHoverEvents (bool enabled) |
void | setAcceptTouchEvents (bool enabled) |
void | setActive (bool active) |
void | setBoundingRegionGranularity (qreal granularity) |
void | setCacheMode (CacheMode mode, const QSize &logicalCacheSize) |
void | setCursor (const QCursor &cursor) |
void | setData (int key, const QVariant &value) |
void | setEnabled (bool enabled) |
void | setFiltersChildEvents (bool enabled) |
void | setFlag (GraphicsItemFlag flag, bool enabled) |
void | setFlags (QFlags< QGraphicsItem::GraphicsItemFlag > flags) |
void | setFocus (Qt::FocusReason focusReason) |
void | setFocusProxy (QGraphicsItem *item) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setGroup (QGraphicsItemGroup *group) |
void | setHandlesChildEvents (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setMatrix (const QMatrix &matrix, bool combine) |
void | setOpacity (qreal opacity) |
void | setPanelModality (PanelModality panelModality) |
void | setParentItem (QGraphicsItem *newParent) |
void | setPos (const QPointF &pos) |
void | setPos (qreal x, qreal y) |
void | setRotation (qreal angle) |
void | setScale (qreal factor) |
void | setSelected (bool selected) |
void | setToolTip (const QString &toolTip) |
void | setTransform (const QTransform &matrix, bool combine) |
void | setTransformations (const QList< QGraphicsTransform * > &transformations) |
void | setTransformOriginPoint (qreal x, qreal y) |
void | setTransformOriginPoint (const QPointF &origin) |
void | setVisible (bool visible) |
void | setX (qreal x) |
void | setY (qreal y) |
void | setZValue (qreal z) |
void | shear (qreal sh, qreal sv) |
void | show () |
void | stackBefore (const QGraphicsItem *sibling) |
QGraphicsObject * | toGraphicsObject () |
const QGraphicsObject * | toGraphicsObject () const |
QString | toolTip () const |
QGraphicsItem * | topLevelItem () const |
QGraphicsWidget * | topLevelWidget () const |
QTransform | transform () const |
QList< QGraphicsTransform * > | transformations () const |
QPointF | transformOriginPoint () const |
void | translate (qreal dx, qreal dy) |
void | ungrabKeyboard () |
void | ungrabMouse () |
void | unsetCursor () |
void | update (qreal x, qreal y, qreal width, qreal height) |
void | update (const QRectF &rect) |
QGraphicsWidget * | window () const |
qreal | x () const |
qreal | y () const |
qreal | zValue () const |
![]() | |
QGraphicsLayoutItem (QGraphicsLayoutItem *parent, bool isLayout) | |
virtual | ~QGraphicsLayoutItem () |
QRectF | contentsRect () const |
QSizeF | effectiveSizeHint (Qt::SizeHint which, const QSizeF &constraint) const |
QRectF | geometry () const |
QGraphicsItem * | graphicsItem () const |
bool | isLayout () const |
qreal | maximumHeight () const |
QSizeF | maximumSize () const |
qreal | maximumWidth () const |
qreal | minimumHeight () const |
QSizeF | minimumSize () const |
qreal | minimumWidth () const |
bool | ownedByLayout () const |
QGraphicsLayoutItem * | parentLayoutItem () const |
qreal | preferredHeight () const |
QSizeF | preferredSize () const |
qreal | preferredWidth () const |
void | setMaximumHeight (qreal height) |
void | setMaximumSize (const QSizeF &size) |
void | setMaximumSize (qreal w, qreal h) |
void | setMaximumWidth (qreal width) |
void | setMinimumHeight (qreal height) |
void | setMinimumSize (const QSizeF &size) |
void | setMinimumSize (qreal w, qreal h) |
void | setMinimumWidth (qreal width) |
void | setParentLayoutItem (QGraphicsLayoutItem *parent) |
void | setPreferredHeight (qreal height) |
void | setPreferredSize (const QSizeF &size) |
void | setPreferredSize (qreal w, qreal h) |
void | setPreferredWidth (qreal width) |
void | setSizePolicy (const QSizePolicy &policy) |
void | setSizePolicy (QSizePolicy::Policy hPolicy, QSizePolicy::Policy vPolicy, QSizePolicy::ControlType controlType) |
QSizePolicy | sizePolicy () const |
Properties | |
uint | autoExpireDelay |
bool | collapsed |
bool | detached |
Extender | extender |
QIcon | icon |
QString | name |
QString | title |
QGraphicsItem | widget |
![]() | |
autoFillBackground | |
focusPolicy | |
font | |
geometry | |
layout | |
layoutDirection | |
maximumSize | |
minimumSize | |
palette | |
preferredSize | |
size | |
sizePolicy | |
windowFlags | |
windowTitle | |
![]() | |
effect | |
enabled | |
opacity | |
parent | |
pos | |
rotation | |
scale | |
transformOriginPoint | |
visible | |
x | |
y | |
z | |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
void | setTabOrder (QGraphicsWidget *first, QGraphicsWidget *second) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | GraphicsItemFlags |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
Provides detachable items for an Extender.
This class wraps around a QGraphicsWidget and provides drag&drop handling, a draghandle, title and ability to display qactions as a row of icon, ability to expand, collapse, return to source and tracks configuration associated with this item for you.
Typical usage of ExtenderItems in your applet could look like this:
Note that we first check if the item already exists: ExtenderItems are persistent between sessions so we can't blindly add items since they might already exist.
You'll then need to implement the initExtenderItem function. Having this function in your applet makes sure that detached extender items get restored after plasma is restarted, just like applets are. That is also the reason that we write an entry in item->config(). In this function you should instantiate a QGraphicsWidget or QGraphicsItem and call the setWidget function on the ExtenderItem. This is the only correct way of adding actual content to a extenderItem. An example:
Definition at line 80 of file extenderitem.h.
Constructor & Destructor Documentation
|
explicit |
The constructor takes care of adding this item to an extender.
- Parameters
-
hostExtender The extender the extender item belongs to. extenderItemId the id of the extender item. Use the default 0 to assign a new, unique id to this extender item.
Definition at line 128 of file extenderitem.cpp.
Plasma::ExtenderItem::~ExtenderItem | ( | ) |
Definition at line 234 of file extenderitem.cpp.
Member Function Documentation
- Returns
- the QAction with the given name from our collection. By default the action collection contains a "movebacktosource" action which will be only shown when the item is detached.
Definition at line 554 of file extenderitem.cpp.
- Parameters
-
name the name to store the action under in our collection. action the action to add. Actions will be displayed as an icon in the drag handle.
Definition at line 540 of file extenderitem.cpp.
uint Plasma::ExtenderItem::autoExpireDelay | ( | ) | const |
- Returns
- whether or not this extender item has an auto expire delay.
KConfigGroup Plasma::ExtenderItem::config | ( | ) | const |
fetch the configuration of this widget.
- Returns
- the configuration of this widget.
Definition at line 240 of file extenderitem.cpp.
|
slot |
Destroys the extender item.
As opposed to calling delete on this class, destroy also removes the config group associated with this item.
Definition at line 579 of file extenderitem.cpp.
|
signal |
Emitted when the extender item is destroyed.
- Since
- 4.4.1
Extender* Plasma::ExtenderItem::extender | ( | ) | const |
- Returns
- the extender this items belongs to.
ExtenderGroup * Plasma::ExtenderItem::group | ( | ) | const |
|
slot |
Hides the close button in this item's drag handle.
Definition at line 569 of file extenderitem.cpp.
|
protectedvirtual |
Reimplemented from QGraphicsWidget.
Definition at line 797 of file extenderitem.cpp.
|
protectedvirtual |
Reimplemented from QGraphicsWidget.
Definition at line 793 of file extenderitem.cpp.
QIcon Plasma::ExtenderItem::icon | ( | ) | const |
- Returns
- the icon being displayed in the extender item's drag handle.
bool Plasma::ExtenderItem::isCollapsed | ( | ) | const |
- Returns
- whether or not the extender item is collapsed.
Definition at line 493 of file extenderitem.cpp.
bool Plasma::ExtenderItem::isDetached | ( | ) | const |
- Returns
- whether or not this item is detached from its original source.
Definition at line 531 of file extenderitem.cpp.
bool Plasma::ExtenderItem::isGroup | ( | ) | const |
- Returns
- whether or not this is an ExtenderGroup.
- Since
- 4.3
Definition at line 488 of file extenderitem.cpp.
bool Plasma::ExtenderItem::isTransient | ( | ) | const |
- Returns
- true if the ExtenderItem is transient.
- Since
- 4.6
Definition at line 811 of file extenderitem.cpp.
|
protectedvirtual |
Reimplemented from QGraphicsItem.
Definition at line 775 of file extenderitem.cpp.
|
protectedvirtual |
Reimplemented from QGraphicsItem.
Definition at line 677 of file extenderitem.cpp.
|
protectedvirtual |
Reimplemented from QGraphicsItem.
Definition at line 668 of file extenderitem.cpp.
|
protectedvirtual |
Reimplemented from QGraphicsItem.
Definition at line 787 of file extenderitem.cpp.
|
protectedvirtual |
Reimplemented from QGraphicsWidget.
Definition at line 654 of file extenderitem.cpp.
QString Plasma::ExtenderItem::name | ( | ) | const |
- Returns
- the name of the item.
|
protectedvirtual |
Reimplemented from QGraphicsWidget.
Definition at line 644 of file extenderitem.cpp.
|
protectedvirtual |
Reimplemented from QGraphicsWidget.
Definition at line 660 of file extenderitem.cpp.
|
slot |
Returns the extender item to its source applet.
Definition at line 633 of file extenderitem.cpp.
|
protectedvirtual |
Reimplemented from QGraphicsItem.
Definition at line 782 of file extenderitem.cpp.
void Plasma::ExtenderItem::setAutoExpireDelay | ( | uint | time | ) |
- Parameters
-
time (in ms) before this extender item destroys itself unless it is detached, in which case this extender stays around. 0 means forever and is the default.
Definition at line 498 of file extenderitem.cpp.
|
slot |
Collapse or expand the extender item.
Defaults to false.
Definition at line 602 of file extenderitem.cpp.
void Plasma::ExtenderItem::setExtender | ( | Extender * | extender, |
const QPointF & | pos = QPointF(-1, -1) |
||
) |
- Parameters
-
extender the extender this item belongs to. pos the position in the extender this item should be added. Defaults to 'just append'.
Definition at line 348 of file extenderitem.cpp.
void Plasma::ExtenderItem::setGroup | ( | ExtenderGroup * | group | ) |
- Parameters
-
group the group you want this item to belong to. Note that you can't nest ExtenderGroups.
- Since
- 4.3
Definition at line 442 of file extenderitem.cpp.
void Plasma::ExtenderItem::setGroup | ( | ExtenderGroup * | group, |
const QPointF & | pos | ||
) |
- Parameters
-
group the group you want this item to belong to. Note that you can't nest ExtenderGroups. group the new group pos position inside the extender group
- Since
- 4.5
Definition at line 447 of file extenderitem.cpp.
void Plasma::ExtenderItem::setIcon | ( | const QString & | icon | ) |
- Parameters
-
icon the icon name to display in the extender item's drag handle. Defaults to the source applet's icon. This icon name will also be stored in the item's configuration, so you don't have to manually store/restore this information.
Definition at line 334 of file extenderitem.cpp.
void Plasma::ExtenderItem::setIcon | ( | const QIcon & | icon | ) |
- Parameters
-
icon the icon to display in the extender item's drag handle. Defaults to the source applet's icon.
Definition at line 325 of file extenderitem.cpp.
void Plasma::ExtenderItem::setName | ( | const QString & | name | ) |
You can assign names to extender items to look them up through the item() function.
Make sure you only use unique names. This name will be stored in the item's configuration.
- Parameters
-
name the name of the item. Defaults to an empty string.
Definition at line 291 of file extenderitem.cpp.
void Plasma::ExtenderItem::setTitle | ( | const QString & | title | ) |
- Parameters
-
title the title that will be shown in the extender item's dragger. Default is no title. This title will also be stored in the item's configuration, so you don't have to manually store/restore this information for your extender items.
Definition at line 277 of file extenderitem.cpp.
void Plasma::ExtenderItem::setTransient | ( | const bool | transient | ) |
Set the ExtenderItem as transient: won't be saved in the Plasma config and won't be restored.
This is intended for items that have contents valid only for this session.
- Parameters
-
transient true if the ExtenderItem will be transient
- Since
- 4.6
Definition at line 806 of file extenderitem.cpp.
void Plasma::ExtenderItem::setWidget | ( | QGraphicsItem * | widget | ) |
- Parameters
-
widget The widget that should be wrapped into the extender item. It has to be a QGraphicsWidget.
Definition at line 302 of file extenderitem.cpp.
|
slot |
Shows a close button in this item's drag handle.
By default a close button will not be shown.
Definition at line 559 of file extenderitem.cpp.
|
protectedvirtual |
Reimplemented from QGraphicsWidget.
Definition at line 801 of file extenderitem.cpp.
QString Plasma::ExtenderItem::title | ( | ) | const |
- Returns
- the title shown in the extender item's dragger.
QGraphicsItem* Plasma::ExtenderItem::widget | ( | ) | const |
- Returns
- The widget that is wrapped into the extender item.
Property Documentation
|
readwrite |
Definition at line 90 of file extenderitem.h.
|
readwrite |
Definition at line 88 of file extenderitem.h.
|
read |
Definition at line 89 of file extenderitem.h.
|
readwrite |
Definition at line 87 of file extenderitem.h.
|
readwrite |
Definition at line 86 of file extenderitem.h.
|
readwrite |
Definition at line 85 of file extenderitem.h.
|
readwrite |
Definition at line 84 of file extenderitem.h.
|
readwrite |
Definition at line 83 of file extenderitem.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:23:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.