ThreadWeaver
#include <WeaverImpl.h>
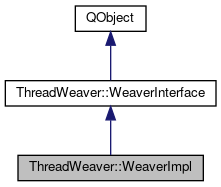
Signals | |
void | asyncThreadSuspended (ThreadWeaver::Thread *) |
void | threadBusy (ThreadWeaver::Thread *, ThreadWeaver::Job *j) |
void | threadExited (ThreadWeaver::Thread *) |
void | threadStarted (ThreadWeaver::Thread *) |
void | threadSuspended (ThreadWeaver::Thread *) |
![]() | |
void | finished () |
void | jobDone (ThreadWeaver::Job *) |
void | stateChanged (ThreadWeaver::State *) |
void | suspended () |
Public Member Functions | |
WeaverImpl (QObject *parent=0) | |
virtual | ~WeaverImpl () |
int | activeThreadCount () |
virtual Job * | applyForWork (Thread *thread, Job *previous) |
void | assignJobs () |
void | blockThreadUntilJobsAreBeingAssigned (Thread *th) |
int | currentNumberOfThreads () const |
void | decActiveThreadCount () |
virtual bool | dequeue (Job *) |
virtual void | dequeue () |
void | dumpJobs () |
virtual void | enqueue (Job *) |
virtual void | finish () |
void | incActiveThreadCount () |
bool | isEmpty () const |
bool | isIdle () const |
int | maximumNumberOfThreads () const |
int | queueLength () const |
void | registerObserver (WeaverObserver *) |
void | requestAbort () |
virtual void | resume () |
void | setMaximumNumberOfThreads (int cap) |
void | setState (StateId) |
const State & | state () const |
virtual void | suspend () |
Job * | takeFirstAvailableJob (Job *previous) |
void | waitForAvailableJob (Thread *th) |
![]() | |
WeaverInterface (QObject *parent=0) | |
virtual | ~WeaverInterface () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
void | adjustActiveThreadCount (int diff) |
void | adjustInventory (int noOfNewJobs) |
virtual Thread * | createThread () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
int | m_active |
QList< Job * > | m_assignments |
QList< Thread * > | m_inventory |
int | m_inventoryMax |
QWaitCondition | m_jobAvailable |
QWaitCondition | m_jobFinished |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
A WeaverImpl is the manager of worker threads (Thread objects) to which it assigns jobs from its queue.
It extends the API of WeaverInterface to provide additional methods needed by the Thread objects.
Definition at line 51 of file WeaverImpl.h.
Constructor & Destructor Documentation
|
explicit |
Construct a WeaverImpl object.
Definition at line 52 of file WeaverImpl.cpp.
|
virtual |
Destruct a WeaverImpl object.
Definition at line 78 of file WeaverImpl.cpp.
Member Function Documentation
int WeaverImpl::activeThreadCount | ( | ) |
Returns the number of active threads.
Threads are active if they process a job.
Definition at line 327 of file WeaverImpl.cpp.
|
protected |
Adjust active thread count.
This is a helper function for incActiveThreadCount and decActiveThreadCount.
Definition at line 313 of file WeaverImpl.cpp.
|
protected |
Adjust the inventory size.
This method creates threads on demand. Threads in the inventory are not created upon construction of the WeaverImpl object, but when jobs are queued. This avoids costly delays on the application startup time. Threads are created when the inventory size is under inventoryMin and new jobs are queued.
Definition at line 205 of file WeaverImpl.cpp.
Assign a job to the calling thread.
This is supposed to be called from the Thread objects in the inventory. Do not call this method from your code.
Returns 0 if the weaver is shutting down, telling the calling thread to finish and exit. If no jobs are available and shut down is not in progress, the calling thread is suspended until either condition is met. In previous, threads give the job they have completed. If this is the first job, previous is zero.
Definition at line 354 of file WeaverImpl.cpp.
void WeaverImpl::assignJobs | ( | ) |
Schedule enqueued jobs to be executed by idle threads.
This will try to distribute as many jobs as possible to all idle threads.
Definition at line 288 of file WeaverImpl.cpp.
|
signal |
void WeaverImpl::blockThreadUntilJobsAreBeingAssigned | ( | Thread * | th | ) |
Blocks the calling thread until some actor calls assignJobs.
Definition at line 364 of file WeaverImpl.cpp.
|
protectedvirtual |
Factory method to create the threads.
Overload in adapted Weaver implementations.
Definition at line 233 of file WeaverImpl.cpp.
|
virtual |
Returns the current number of threads in the inventory.
Implements ThreadWeaver::WeaverInterface.
Definition at line 162 of file WeaverImpl.cpp.
void WeaverImpl::decActiveThreadCount | ( | ) |
Decrement the count of active threads.
Definition at line 305 of file WeaverImpl.cpp.
|
virtual |
Remove a job from the queue.
If the job was queued but not started so far, it is simply removed from the queue. For now, it is unsupported to dequeue a job once its execution has started.
For that case, you will have to provide a method to interrupt your job's execution (and receive the done signal). Returns true if the job has been dequeued, false if the job has already been started or is not found in the queue.
Implements ThreadWeaver::WeaverInterface.
Definition at line 238 of file WeaverImpl.cpp.
|
virtual |
Remove all queued jobs.
Please note that this will not kill the threads, therefore all jobs that are being processed will be continued.
Implements ThreadWeaver::WeaverInterface.
Definition at line 265 of file WeaverImpl.cpp.
void WeaverImpl::dumpJobs | ( | ) |
Dump the current jobs to the console.
Not part of the API.
Definition at line 414 of file WeaverImpl.cpp.
|
virtual |
Add a job to be executed.
It depends on the state if execution of the job will be attempted immediately. In suspended state, jobs can be added to the queue, but the threads remain suspended. In WorkongHard state, an idle thread may immediately execute the job, or it might be queued if all threads are busy.
Implements ThreadWeaver::WeaverInterface.
Definition at line 182 of file WeaverImpl.cpp.
|
virtual |
Finish all queued operations, then return.
This method is used in imperative (not event driven) programs that cannot react on events to have the controlling (main) thread wait wait for the jobs to finish. The call will block the calling thread and return when all queued jobs have been processed.
Warning: This will suspend your thread! Warning: If one of your jobs enters an infinite loop, this will never return!
Implements ThreadWeaver::WeaverInterface.
Definition at line 386 of file WeaverImpl.cpp.
void WeaverImpl::incActiveThreadCount | ( | ) |
Increment the count of active threads.
Definition at line 300 of file WeaverImpl.cpp.
|
virtual |
Is the queue empty? The queue is empty if no more jobs are queued.
Implements ThreadWeaver::WeaverInterface.
Definition at line 293 of file WeaverImpl.cpp.
|
virtual |
Is the weaver idle? The weaver is idle if no jobs are queued and no jobs are processed by the threads.
Implements ThreadWeaver::WeaverInterface.
Definition at line 380 of file WeaverImpl.cpp.
|
virtual |
Get the maximum number of threads this Weaver may start.
Implements ThreadWeaver::WeaverInterface.
Definition at line 156 of file WeaverImpl.cpp.
|
virtual |
Returns the number of pending jobs.
This will return the number of queued jobs. Jobs that are currently being executed are not part of the queue. All jobs in the queue are waiting to be executed.
Implements ThreadWeaver::WeaverInterface.
Definition at line 374 of file WeaverImpl.cpp.
|
virtual |
Register an observer.
Observers provides signals on different weaver events that are otherwise only available through objects of different classes (threads, jobs). Usually, access to the signals of those objects is not provided through the weaver API. Use an observer to reveice notice, for example, on thread activity.
To unregister, simply delete the observer.
Implements ThreadWeaver::WeaverInterface.
Definition at line 168 of file WeaverImpl.cpp.
|
virtual |
Request aborts of the currently executed jobs.
It is important to understand that aborts are requested, but cannot be guaranteed, as not all Job classes support it. It is up to the application to decide if and how job aborts are necessary.
Implements ThreadWeaver::WeaverInterface.
Definition at line 406 of file WeaverImpl.cpp.
|
virtual |
Resume job queueing.
- See also
- suspend
Implements ThreadWeaver::WeaverInterface.
Definition at line 283 of file WeaverImpl.cpp.
|
virtual |
Set the maximum number of threads this Weaver object may start.
Implements ThreadWeaver::WeaverInterface.
Definition at line 149 of file WeaverImpl.cpp.
void WeaverImpl::setState | ( | StateId | id | ) |
Set the object state.
Definition at line 124 of file WeaverImpl.cpp.
|
virtual |
Return the state of the weaver object.
Implements ThreadWeaver::WeaverInterface.
Definition at line 143 of file WeaverImpl.cpp.
|
virtual |
Suspend job execution.
When suspending, all threads are allowed to finish the currently assigned job but will not receive a new assignment. When all threads are done processing the assigned job, the signal suspended will() be emitted. If you call suspend() and there are no jobs left to be done, you will immediately receive the suspended() signal.
Implements ThreadWeaver::WeaverInterface.
Definition at line 278 of file WeaverImpl.cpp.
Take the first available job out of the queue and return it.
The job will be removed from the queue (therefore, take). Only jobs that have no unresolved dependencies are considered available. If only jobs that depened on other, unfinished jobs are in the queue, this method returns a nil pointer.
Definition at line 333 of file WeaverImpl.cpp.
|
signal |
The thread is busy executing job j.
|
signal |
A thread has exited.
|
signal |
A Thread has been created.
|
signal |
A thread has been suspended.
void WeaverImpl::waitForAvailableJob | ( | Thread * | th | ) |
Wait for a job to become available.
Definition at line 359 of file WeaverImpl.cpp.
Member Data Documentation
|
protected |
The number of jobs that are assigned to the worker threads, but not finished.
Definition at line 165 of file WeaverImpl.h.
The job queue.
Definition at line 162 of file WeaverImpl.h.
Lock the mutex for this weaver.
The threads in the inventory need to lock the weaver's mutex to synchronize the job management. Unlock. See lock(). The thread inventory.
Definition at line 160 of file WeaverImpl.h.
|
protected |
Stored setting .
Definition at line 167 of file WeaverImpl.h.
|
protected |
Wait condition all idle or done threads wait for.
Definition at line 169 of file WeaverImpl.h.
|
protected |
Wait for a job to finish.
Definition at line 171 of file WeaverImpl.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:23:33 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.