akregator
#include <feed.h>
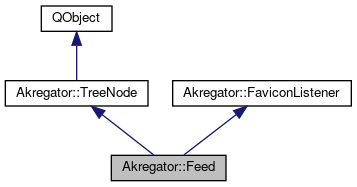
Public Types | |
enum | ArchiveMode { globalDefault, keepAllArticles, disableArchiving, limitArticleNumber, limitArticleAge } |
Public Slots | |
void | fetch (bool followDiscovery=false) |
void | slotAbortFetch () |
void | slotAddFeedIconListener () |
void | slotAddToFetchQueue (Akregator::FetchQueue *queue, bool intervalFetchOnly=false) |
![]() | |
virtual void | slotAddToFetchQueue (Akregator::FetchQueue *queue, bool intervalFetchesOnly=false)=0 |
Signals | |
void | fetchAborted (Akregator::Feed *) |
void | fetchDiscovery (Akregator::Feed *) |
void | fetched (Akregator::Feed *) |
void | fetchError (Akregator::Feed *) |
void | fetchStarted (Akregator::Feed *) |
![]() | |
void | signalArticlesAdded (Akregator::TreeNode *, const QList< Akregator::Article > &guids) |
void | signalArticlesRemoved (Akregator::TreeNode *, const QList< Akregator::Article > &guids) |
void | signalArticlesUpdated (Akregator::TreeNode *, const QList< Akregator::Article > &guids) |
void | signalChanged (Akregator::TreeNode *) |
void | signalDestroyed (Akregator::TreeNode *) |
Public Member Functions | |
Feed (Akregator::Backend::Storage *storage) | |
~Feed () | |
bool | accept (TreeNodeVisitor *visitor) |
ArchiveMode | archiveMode () const |
KJob * | createMarkAsReadJob () |
void | deleteExpiredArticles (Akregator::ArticleDeleteJob *job) |
QString | description () const |
QVector< const Feed * > | feeds () const |
QVector< Feed * > | feeds () |
Syndication::ErrorCode | fetchErrorCode () const |
bool | fetchErrorOccurred () const |
int | fetchInterval () const |
Article | findArticle (const QString &guid) const |
QVector< const Folder * > | folders () const |
QVector< Folder * > | folders () |
QString | htmlUrl () const |
QIcon | icon () const |
QPixmap | image () const |
bool | isAggregation () const |
bool | isArticlesLoaded () const |
bool | isFetching () const |
bool | isGroup () const |
bool | loadLinkedWebsite () const |
bool | markImmediatelyAsRead () const |
int | maxArticleAge () const |
int | maxArticleNumber () const |
const TreeNode * | next () const |
TreeNode * | next () |
void | setArchiveMode (ArchiveMode archiveMode) |
void | setCustomFetchIntervalEnabled (bool enabled) |
void | setDescription (const QString &s) |
void | setFavicon (const QIcon &icon) |
void | setFetchInterval (int interval) |
void | setHtmlUrl (const QString &s) |
void | setImage (const QPixmap &p) |
void | setLoadLinkedWebsite (bool enabled) |
void | setMarkImmediatelyAsRead (bool enabled) |
void | setMaxArticleAge (int maxArticleAge) |
void | setMaxArticleNumber (int maxArticleNumber) |
void | setUseNotification (bool enabled) |
void | setXmlUrl (const QString &s) |
QDomElement | toOPML (QDomElement parent, QDomDocument document) const |
int | totalCount () const |
int | unread () const |
bool | useCustomFetchInterval () const |
bool | useNotification () const |
QString | xmlUrl () const |
![]() | |
TreeNode () | |
virtual | ~TreeNode () |
virtual TreeNode * | childAt (int pos) |
virtual const TreeNode * | childAt (int pos) const |
virtual QList< const TreeNode * > | children () const |
virtual QList< TreeNode * > | children () |
ArticleListJob * | createListJob () |
virtual uint | id () const |
QPoint | listViewScrollBarPositions () const |
virtual const TreeNode * | nextSibling () const |
virtual TreeNode * | nextSibling () |
virtual const Folder * | parent () const |
virtual Folder * | parent () |
virtual const TreeNode * | prevSibling () const |
virtual TreeNode * | prevSibling () |
virtual void | setId (uint id) |
void | setListViewScrollBarPositions (const QPoint &pos) |
virtual void | setNotificationMode (bool doNotify) |
virtual void | setParent (Folder *parent) |
void | setTitle (const QString &title) |
QString | title () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~FaviconListener () |
Static Public Member Functions | |
static QString | archiveModeToString (ArchiveMode mode) |
static Feed * | fromOPML (QDomElement e, Akregator::Backend::Storage *storage) |
static ArchiveMode | stringToArchiveMode (const QString &str) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
virtual void | articlesModified () |
void | emitSignalDestroyed () |
virtual void | nodeModified () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Member Enumeration Documentation
the archiving modes
Enumerator | |
---|---|
globalDefault |
use default from Settings (default) |
keepAllArticles |
Don't delete any articles. |
disableArchiving |
Don't save any articles except articles with keep flag set (equal to maxArticleNumber() == 0) |
limitArticleNumber |
Save maxArticleNumber() articles, plus the ones with keep flag set. |
limitArticleAge |
Save articles not older than maxArticleAge() (or keep flag set) |
Constructor & Destructor Documentation
|
explicit |
Member Function Documentation
|
virtual |
Implements Akregator::TreeNode.
Akregator::Feed::ArchiveMode Akregator::Feed::archiveMode | ( | ) | const |
|
static |
|
virtual |
Implements Akregator::TreeNode.
void Akregator::Feed::deleteExpiredArticles | ( | Akregator::ArticleDeleteJob * | job | ) |
QString Akregator::Feed::description | ( | ) | const |
|
virtual |
Implements Akregator::TreeNode.
|
virtual |
Implements Akregator::TreeNode.
|
slot |
|
signal |
emitted when a fetch is aborted
|
signal |
emitted when a feed URL was found by auto discovery
|
signal |
emitted when feed finished fetching
|
signal |
emitted when a fetch error occurred
Syndication::ErrorCode Akregator::Feed::fetchErrorCode | ( | ) | const |
bool Akregator::Feed::fetchErrorOccurred | ( | ) | const |
int Akregator::Feed::fetchInterval | ( | ) | const |
|
signal |
emitted when fetching started
Implements Akregator::TreeNode.
Implements Akregator::TreeNode.
|
static |
QString Akregator::Feed::htmlUrl | ( | ) | const |
|
virtual |
Implements Akregator::TreeNode.
QPixmap Akregator::Feed::image | ( | ) | const |
|
inlinevirtual |
returns if the node represents an aggregation, i.e.
containing items from more than once source feed. Folders and virtual folders are aggregations, feeds are not.
Implements Akregator::TreeNode.
bool Akregator::Feed::isArticlesLoaded | ( | ) | const |
|
inlinevirtual |
returns if this node is a feed group (false
here)
Implements Akregator::TreeNode.
bool Akregator::Feed::markImmediatelyAsRead | ( | ) | const |
int Akregator::Feed::maxArticleAge | ( | ) | const |
int Akregator::Feed::maxArticleNumber | ( | ) | const |
|
virtual |
returns the next node in the tree.
Calling next() unless it returns 0 iterates through the tree in pre-order
Implements Akregator::TreeNode.
|
virtual |
Implements Akregator::TreeNode.
void Akregator::Feed::setArchiveMode | ( | ArchiveMode | archiveMode | ) |
void Akregator::Feed::setCustomFetchIntervalEnabled | ( | bool | enabled | ) |
void Akregator::Feed::setDescription | ( | const QString & | s | ) |
|
virtual |
sets the favicon (used in the tree view)
Implements Akregator::FaviconListener.
void Akregator::Feed::setFetchInterval | ( | int | interval | ) |
void Akregator::Feed::setHtmlUrl | ( | const QString & | s | ) |
void Akregator::Feed::setImage | ( | const QPixmap & | p | ) |
void Akregator::Feed::setLoadLinkedWebsite | ( | bool | enabled | ) |
void Akregator::Feed::setMarkImmediatelyAsRead | ( | bool | enabled | ) |
void Akregator::Feed::setMaxArticleAge | ( | int | maxArticleAge | ) |
void Akregator::Feed::setMaxArticleNumber | ( | int | maxArticleNumber | ) |
void Akregator::Feed::setXmlUrl | ( | const QString & | s | ) |
|
slot |
|
static |
|
virtual |
exports the feed settings to OPML
Implements Akregator::TreeNode.
|
virtual |
returns the number of total articles in this feed
- Returns
- number of articles
Implements Akregator::TreeNode.
|
virtual |
returns the unread count for this feed
Implements Akregator::TreeNode.
bool Akregator::Feed::useCustomFetchInterval | ( | ) | const |
QString Akregator::Feed::xmlUrl | ( | ) | const |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:34:01 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.