blogilo
#include <backend.h>
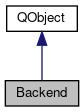
Signals | |
void | sigCategoryListFetched (int blog_id) |
void | sigEntriesListFetched (int blog_id) |
void | sigError (const QString &errorMessage) |
void | sigMediaError (const QString &errorMessage, BilboMedia *media) |
void | sigMediaUploaded (BilboMedia *media) |
void | sigPostFetched (BilboPost *post) |
void | sigPostPublished (int blog_id, BilboPost *post) |
void | sigPostRemoved (int blog_id, const BilboPost &post) |
Public Member Functions | |
Backend (int blog_id, QObject *parent=0) | |
~Backend () | |
void | fetchPost (BilboPost *post) |
void | getCategoryListFromServer () |
void | getEntriesListFromServer (int count) |
void | modifyPost (BilboPost *post) |
void | publishPost (BilboPost *post) |
void | removePost (BilboPost *post) |
void | uploadMedia (BilboMedia *media) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Slots | |
void | bloggerAuthenticated (const QMap< QString, QString > &authData) |
void | categoriesListed (const QList< QMap< QString, QString > > &categories) |
void | entriesListed (const QList< KBlog::BlogPost > &posts) |
void | error (KBlog::Blog::ErrorType type, const QString &errorMessage) |
void | mediaUploaded (KBlog::BlogMedia *media) |
void | postPublished (KBlog::BlogPost *post) |
void | savePostInDbAndEmitResult (KBlog::BlogPost *post) |
void | slotMediaError (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogMedia *media) |
void | slotPostFetched (KBlog::BlogPost *post) |
void | slotPostRemoved (KBlog::BlogPost *post) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Constructor & Destructor Documentation
|
explicit |
Definition at line 62 of file backend.cpp.
Backend::~Backend | ( | ) |
Definition at line 86 of file backend.cpp.
Member Function Documentation
Definition at line 486 of file backend.cpp.
|
protectedslot |
Definition at line 107 of file backend.cpp.
|
protectedslot |
Definition at line 141 of file backend.cpp.
|
protectedslot |
Definition at line 347 of file backend.cpp.
void Backend::fetchPost | ( | BilboPost * | post | ) |
Fetch a blog post from the server with a specific ID.
The ID of the existing post must be retrieved using getRecentPosts and then be modified and provided to this method or a new BlogPost created with the existing ID.
- Parameters
-
post a blog post with the ID identifying the blog post to fetch.
- See also
- sigPostFetched()
Definition at line 333 of file backend.cpp.
void Backend::getCategoryListFromServer | ( | ) |
Request to Fetch categories list from server.
and after it's fetched, categoriesListed() SLOT will insert them to database, and update list in database and emit categoriesFetched() SIGNAL.
- Parameters
-
blog_id Id of blog in DB!
Definition at line 92 of file backend.cpp.
void Backend::getEntriesListFromServer | ( | int | count | ) |
retrieve latest posts from server
- Parameters
-
count number of post to retrieve.
Definition at line 133 of file backend.cpp.
|
protectedslot |
Definition at line 264 of file backend.cpp.
void Backend::modifyPost | ( | BilboPost * | post | ) |
Modify an existing post.
Note: posiId must sets correctly.
- Parameters
-
post post to modify.
Definition at line 301 of file backend.cpp.
|
protectedslot |
Definition at line 174 of file backend.cpp.
void Backend::publishPost | ( | BilboPost * | post | ) |
Use this to publish a post to server.
- Parameters
-
post Post to publish.
Definition at line 159 of file backend.cpp.
void Backend::removePost | ( | BilboPost * | post | ) |
Remove an existing post from server.
- Parameters
-
post post to remove.
Definition at line 311 of file backend.cpp.
|
protectedslot |
This function is called after a post published fine, to insert it to DB and emit sigPostPublished.
Definition at line 394 of file backend.cpp.
|
signal |
emit when a categoriesListed() Done and Categories added to DB
- Parameters
-
blog_id id of Blog owner of categories.
|
signal |
emit when a entriesListed() Done and Entries added to DB
- Parameters
-
blog_id id of Blog owner of Entries.
|
signal |
this signal emitted when an error occurred on current transaction.
- Parameters
-
type type of error errorMessage error message.
|
signal |
|
signal |
This signal is emitted when a media has been uploaded to the server.
- Parameters
-
media Uploaded media Object.
|
signal |
|
signal |
This signal is emitted when a post published/modified and added/edited to Database.
- Parameters
-
blog_id blog id. post The post has been saved.
|
signal |
this signal is emitted when a post removed successfully.
|
protectedslot |
Definition at line 357 of file backend.cpp.
|
protectedslot |
Definition at line 341 of file backend.cpp.
|
protectedslot |
Definition at line 321 of file backend.cpp.
void Backend::uploadMedia | ( | BilboMedia * | media | ) |
Upload a new Media object e.g.
image to server.
- Parameters
-
media Media Object to upload.
Definition at line 191 of file backend.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:32:16 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.