kalarm
#include <alarmcalendar.h>
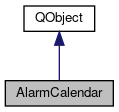
Signals | |
void | calendarSaved (AlarmCalendar *) |
void | earliestAlarmChanged () |
void | haveDisabledAlarmsChanged (bool haveDisabled) |
Public Member Functions | |
virtual | ~AlarmCalendar () |
bool | addEvent (KAEvent *, QWidget *promptParent=0, bool useEventID=false, AlarmResource *=0, bool noPrompt=false, bool *cancelled=0) |
void | adjustStartOfDay () |
KAEvent::List | atLoginAlarms () const |
void | close () |
KCal::Event * | createKCalEvent (const KAEvent *e) const |
bool | deleteEvent (const QString &eventID, bool save=false) |
void | disabledChanged (const KAEvent *) |
KAEvent * | earliestAlarm () const |
bool | endUpdate () |
KAEvent * | event (const QString &uniqueId) |
bool | eventReadOnly (const QString &uniqueID) const |
KAEvent::List | events (CalEvent::Types s=CalEvent::EMPTY) const |
KAEvent::List | events (AlarmResource *, CalEvent::Types=CalEvent::EMPTY) const |
KAEvent::List | events (const KDateTime &from, const KDateTime &to, CalEvent::Types) |
bool | haveDisabledAlarms () const |
bool | isOpen () |
KCal::Event * | kcalEvent (const QString &uniqueId) |
KCal::Event::List | kcalEvents (CalEvent::Type s=CalEvent::EMPTY) |
KCal::Event::List | kcalEvents (AlarmResource *, CalEvent::Type=CalEvent::EMPTY) |
int | load () |
void | loadResource (AlarmResource *, QWidget *parent) |
bool | modifyEvent (const QString &oldEventId, KAEvent *newEvent) |
bool | open () |
QString | path () const |
void | purgeEvents (const KAEvent::List &) |
bool | reload () |
void | reloadFromCache (const QString &resourceID) |
AlarmResource * | resourceForEvent (const QString &eventID) const |
bool | save () |
void | setAlarmPending (KAEvent *, bool pending=true) |
void | startUpdate () |
KAEvent * | templateEvent (const QString &templateName) |
CalEvent::Type | type () const |
KAEvent * | updateEvent (const KAEvent &) |
KAEvent * | updateEvent (const KAEvent *) |
QString | urlString () const |
bool | valid () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static AlarmCalendar * | displayCalendar () |
static AlarmCalendar * | displayCalendarOpen () |
static bool | exportAlarms (const KAEvent::List &, QWidget *parent) |
static KAEvent * | getEvent (const QString &uniqueId) |
static bool | importAlarms (QWidget *, AlarmResource *=0) |
static bool | initialiseCalendars () |
static AlarmCalendar * | resources () |
static void | terminateCalendars () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Provides read and write access to calendar files and resources.
Either vCalendar or iCalendar files may be read, but the calendar is saved only in iCalendar format to avoid information loss.
Definition at line 58 of file alarmcalendar.h.
Constructor & Destructor Documentation
|
virtual |
Definition at line 227 of file alarmcalendar.cpp.
Member Function Documentation
bool AlarmCalendar::addEvent | ( | KAEvent * | event, |
QWidget * | promptParent = 0 , |
||
bool | useEventID = false , |
||
AlarmResource * | resource = 0 , |
||
bool | noPrompt = false , |
||
bool * | cancelled = 0 |
||
) |
Definition at line 1258 of file alarmcalendar.cpp.
void AlarmCalendar::adjustStartOfDay | ( | ) |
Definition at line 2328 of file alarmcalendar.cpp.
KAEvent::List AlarmCalendar::atLoginAlarms | ( | ) | const |
Definition at line 2182 of file alarmcalendar.cpp.
|
signal |
void AlarmCalendar::close | ( | ) |
Definition at line 495 of file alarmcalendar.cpp.
|
inline |
Definition at line 85 of file alarmcalendar.h.
bool AlarmCalendar::deleteEvent | ( | const QString & | eventID, |
bool | save = false |
||
) |
Definition at line 1653 of file alarmcalendar.cpp.
void AlarmCalendar::disabledChanged | ( | const KAEvent * | event | ) |
Definition at line 2128 of file alarmcalendar.cpp.
|
inlinestatic |
Definition at line 131 of file alarmcalendar.h.
|
static |
Definition at line 130 of file alarmcalendar.cpp.
KAEvent * AlarmCalendar::earliestAlarm | ( | ) | const |
Definition at line 2276 of file alarmcalendar.cpp.
|
signal |
bool AlarmCalendar::endUpdate | ( | ) |
Definition at line 1193 of file alarmcalendar.cpp.
KAEvent * AlarmCalendar::event | ( | const QString & | uniqueId | ) |
Definition at line 1817 of file alarmcalendar.cpp.
bool AlarmCalendar::eventReadOnly | ( | const QString & | uniqueID | ) | const |
Definition at line 2090 of file alarmcalendar.cpp.
|
inline |
Definition at line 96 of file alarmcalendar.h.
KAEvent::List AlarmCalendar::events | ( | AlarmResource * | resource, |
CalEvent::Types | type = CalEvent::EMPTY |
||
) | const |
Definition at line 1909 of file alarmcalendar.cpp.
KAEvent::List AlarmCalendar::events | ( | const KDateTime & | from, |
const KDateTime & | to, | ||
CalEvent::Types | type | ||
) |
Definition at line 2004 of file alarmcalendar.cpp.
|
static |
Definition at line 1074 of file alarmcalendar.cpp.
|
static |
Definition at line 150 of file alarmcalendar.cpp.
|
inline |
Definition at line 78 of file alarmcalendar.h.
|
signal |
|
static |
Definition at line 875 of file alarmcalendar.cpp.
|
static |
Definition at line 88 of file alarmcalendar.cpp.
bool AlarmCalendar::isOpen | ( | ) |
Definition at line 235 of file alarmcalendar.cpp.
Event * AlarmCalendar::kcalEvent | ( | const QString & | uniqueId | ) |
Definition at line 1858 of file alarmcalendar.cpp.
|
inline |
Definition at line 107 of file alarmcalendar.h.
Event::List AlarmCalendar::kcalEvents | ( | AlarmResource * | resource, |
CalEvent::Type | type = CalEvent::EMPTY |
||
) |
Definition at line 1964 of file alarmcalendar.cpp.
int AlarmCalendar::load | ( | ) |
Definition at line 321 of file alarmcalendar.cpp.
void AlarmCalendar::loadResource | ( | AlarmResource * | resource, |
QWidget * | parent | ||
) |
Definition at line 536 of file alarmcalendar.cpp.
bool AlarmCalendar::modifyEvent | ( | const QString & | oldEventId, |
KAEvent * | newEvent | ||
) |
Definition at line 1504 of file alarmcalendar.cpp.
bool AlarmCalendar::open | ( | ) |
Definition at line 250 of file alarmcalendar.cpp.
|
inline |
Definition at line 124 of file alarmcalendar.h.
void AlarmCalendar::purgeEvents | ( | const KAEvent::List & | events | ) |
Definition at line 1226 of file alarmcalendar.cpp.
bool AlarmCalendar::reload | ( | ) |
Definition at line 401 of file alarmcalendar.cpp.
void AlarmCalendar::reloadFromCache | ( | const QString & | resourceID | ) |
Definition at line 853 of file alarmcalendar.cpp.
AlarmResource * AlarmCalendar::resourceForEvent | ( | const QString & | eventID | ) | const |
Definition at line 2117 of file alarmcalendar.cpp.
|
inlinestatic |
Definition at line 130 of file alarmcalendar.h.
bool AlarmCalendar::save | ( | ) |
Definition at line 1209 of file alarmcalendar.cpp.
void AlarmCalendar::setAlarmPending | ( | KAEvent * | event, |
bool | pending = true |
||
) |
Definition at line 2299 of file alarmcalendar.cpp.
void AlarmCalendar::startUpdate | ( | ) |
Definition at line 1184 of file alarmcalendar.cpp.
KAEvent * AlarmCalendar::templateEvent | ( | const QString & | templateName | ) |
Definition at line 1868 of file alarmcalendar.cpp.
|
static |
Definition at line 119 of file alarmcalendar.cpp.
|
inline |
Definition at line 64 of file alarmcalendar.h.
KAEvent * AlarmCalendar::updateEvent | ( | const KAEvent & | evnt | ) |
Definition at line 1582 of file alarmcalendar.cpp.
KAEvent * AlarmCalendar::updateEvent | ( | const KAEvent * | evnt | ) |
Definition at line 1586 of file alarmcalendar.cpp.
|
inline |
Definition at line 125 of file alarmcalendar.h.
|
inline |
Definition at line 63 of file alarmcalendar.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:34:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.