kmail
#include <kmmainwidget.h>
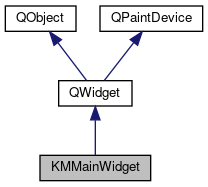
Public Types | |
typedef QList< KMMainWidget * > | PtrList |
Signals | |
void | captionChangeRequest (const QString &caption) |
void | messagesTransfered (bool) |
void | recreateGui () |
Public Member Functions | |
KMMainWidget (QWidget *parent, KXMLGUIClient *aGUIClient, KActionCollection *actionCollection, KSharedConfig::Ptr config=KMKernel::self() ->config()) | |
virtual | ~KMMainWidget () |
QAction * | action (const QString &name) |
void | addRecentFile (const KUrl &mUrl) |
void | clearViewer () |
QSharedPointer < MailCommon::FolderCollection > | currentFolder () const |
void | destruct () |
KAction * | editAction () const |
KActionMenu * | filterMenu () const |
KMail::FolderShortcutActionManager * | folderShortcutActionManager () const |
MailCommon::FolderTreeView * | folderTreeView () const |
KXMLGUIClient * | guiClient () const |
KActionMenu * | mailingListActionMenu () const |
KMail::MessageActions * | messageActions () const |
CollectionPane * | messageListPane () const |
KMReaderWin * | messageView () const |
void | populateMessageListStatusFilterCombo () |
void | readConfig () |
void | readFolderConfig () |
void | readPreConfig () |
void | savePaneSelection () |
KAction * | sendAgainAction () const |
KAction * | sendQueuedAction () const |
KActionMenuTransport * | sendQueueViaMenu () const |
KMail::TagActionManager * | tagActionManager () const |
void | updatePaneTagComboBox () |
void | updateQuickSearchLineText () |
void | updateVacationScriptStatus () |
QWidget * | vacationScriptIndicator () const |
void | writeConfig (bool force=true) |
void | writeFolderConfig () |
void | writeReaderConfig () |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
virtual QSize | minimumSizeHint () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
virtual QSize | sizeHint () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Static Public Member Functions | |
static void | cleanup () |
static const PtrList * | mainWidgetList () |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
Protected Member Functions | |
KActionCollection * | actionCollection () const |
KSharedConfig::Ptr | config () |
void | copySelectedMessagesToFolder (const Akonadi::Collection &dest) |
void | createWidgets () |
void | deleteWidgets () |
void | layoutSplitters () |
void | moveSelectedMessagesToFolder (const Akonadi::Collection &dest) |
void | newFromTemplate (const Akonadi::Item &) |
void | setupActions () |
virtual void | showEvent (QShowEvent *event) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEvent *event) |
virtual bool | event (QEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual void | paintEvent (QPaintEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual void | wheelEvent (QWheelEvent *event) |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
Additional Inherited Members | |
![]() | |
typedef | RenderFlags |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
acceptDrops | |
accessibleDescription | |
accessibleName | |
autoFillBackground | |
baseSize | |
childrenRect | |
childrenRegion | |
contextMenuPolicy | |
cursor | |
enabled | |
focus | |
focusPolicy | |
font | |
frameGeometry | |
frameSize | |
fullScreen | |
geometry | |
height | |
inputMethodHints | |
isActiveWindow | |
layoutDirection | |
locale | |
maximized | |
maximumHeight | |
maximumSize | |
maximumWidth | |
minimized | |
minimumHeight | |
minimumSize | |
minimumSizeHint | |
minimumWidth | |
modal | |
mouseTracking | |
normalGeometry | |
palette | |
pos | |
rect | |
size | |
sizeHint | |
sizeIncrement | |
sizePolicy | |
statusTip | |
styleSheet | |
toolTip | |
updatesEnabled | |
visible | |
whatsThis | |
width | |
windowFilePath | |
windowFlags | |
windowIcon | |
windowIconText | |
windowModality | |
windowModified | |
windowOpacity | |
windowTitle | |
x | |
y | |
![]() | |
objectName | |
Detailed Description
Definition at line 91 of file kmmainwidget.h.
Member Typedef Documentation
Definition at line 96 of file kmmainwidget.h.
Constructor & Destructor Documentation
KMMainWidget::KMMainWidget | ( | QWidget * | parent, |
KXMLGUIClient * | aGUIClient, | ||
KActionCollection * | actionCollection, | ||
KSharedConfig::Ptr | config = KMKernel::self()->config() |
||
) |
Definition at line 208 of file kmmainwidget.cpp.
|
virtual |
Definition at line 373 of file kmmainwidget.cpp.
Member Function Documentation
Definition at line 129 of file kmmainwidget.h.
|
inlineprotected |
Definition at line 283 of file kmmainwidget.h.
|
slot |
Convenience function to get the action collection in a list.
- Returns
- a list of action collections. The list only has one item, and that is the action collection of this main widget as returned by actionCollection().
Definition at line 4045 of file kmmainwidget.cpp.
void KMMainWidget::addRecentFile | ( | const KUrl & | mUrl | ) |
Definition at line 4401 of file kmmainwidget.cpp.
|
slot |
Definition at line 4349 of file kmmainwidget.cpp.
|
slot |
Definition at line 4354 of file kmmainwidget.cpp.
|
signal |
|
static |
|
slot |
Clear and create actions for marked filters.
Definition at line 4065 of file kmmainwidget.cpp.
void KMMainWidget::clearViewer | ( | ) |
Definition at line 533 of file kmmainwidget.cpp.
|
protected |
- Returns
- the correct config dialog depending on whether the parent of the mainWidget is a KPart or a KMMainWindow. When dealing with geometries, use this pointer
|
protected |
Definition at line 1832 of file kmmainwidget.cpp.
|
protected |
Definition at line 958 of file kmmainwidget.cpp.
QSharedPointer< FolderCollection > KMMainWidget::currentFolder | ( | ) | const |
Definition at line 4161 of file kmmainwidget.cpp.
|
protected |
Definition at line 939 of file kmmainwidget.cpp.
void KMMainWidget::destruct | ( | ) |
Definition at line 383 of file kmmainwidget.cpp.
|
inline |
Definition at line 132 of file kmmainwidget.h.
|
inline |
Definition at line 130 of file kmmainwidget.h.
|
slot |
Select the given folder If the folder is 0 the intro is shown.
Definition at line 457 of file kmmainwidget.cpp.
KMail::FolderShortcutActionManager * KMMainWidget::folderShortcutActionManager | ( | ) | const |
Definition at line 4250 of file kmmainwidget.cpp.
|
inline |
Definition at line 148 of file kmmainwidget.h.
|
inline |
Returns the XML GUI client.
Definition at line 153 of file kmmainwidget.h.
|
slot |
Definition at line 4087 of file kmmainwidget.cpp.
|
protected |
Definition at line 597 of file kmmainwidget.cpp.
|
inline |
Definition at line 131 of file kmmainwidget.h.
|
static |
Returns a list of all KMMainWidgets.
Warning, the list itself can be 0.
- Returns
- the list of all main widgets, or 0 if it is not yet initialized
Definition at line 4151 of file kmmainwidget.cpp.
|
inline |
Definition at line 137 of file kmmainwidget.h.
|
inline |
Access to the header list pane.
Definition at line 124 of file kmmainwidget.h.
|
signal |
|
inline |
Easy access to main components of the window.
Definition at line 122 of file kmmainwidget.h.
|
protected |
Definition at line 1779 of file kmmainwidget.cpp.
|
protected |
Definition at line 1416 of file kmmainwidget.cpp.
void KMMainWidget::populateMessageListStatusFilterCombo | ( | ) |
Definition at line 4458 of file kmmainwidget.cpp.
void KMMainWidget::readConfig | ( | ) |
Read configuration options after widgets are created.
Definition at line 802 of file kmmainwidget.cpp.
void KMMainWidget::readFolderConfig | ( | ) |
Read configuration for current folder.
Definition at line 563 of file kmmainwidget.cpp.
void KMMainWidget::readPreConfig | ( | ) |
Read configuration options before widgets are created.
Definition at line 542 of file kmmainwidget.cpp.
|
signal |
|
slot |
Definition at line 1936 of file kmmainwidget.cpp.
|
slot |
Definition at line 344 of file kmmainwidget.cpp.
void KMMainWidget::savePaneSelection | ( | ) |
Definition at line 4375 of file kmmainwidget.cpp.
|
inline |
Definition at line 133 of file kmmainwidget.h.
|
inline |
Definition at line 134 of file kmmainwidget.h.
|
inline |
Definition at line 135 of file kmmainwidget.h.
|
protected |
Definition at line 2779 of file kmmainwidget.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 4175 of file kmmainwidget.cpp.
|
protectedslot |
Show a message screen explaining that we are currently offline, when an online folder is selected.
Definition at line 2312 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2319 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 3510 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 3802 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2141 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2149 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1518 of file kmmainwidget.cpp.
|
slot |
Definition at line 1302 of file kmmainwidget.cpp.
|
slot |
Definition at line 1308 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2175 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1542 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 3580 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 3566 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1203 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1185 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1314 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2209 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2605 of file kmmainwidget.cpp.
|
slot |
This will ask for a destination folder and copy the currently selected messages (in MessageListView) into it.
Definition at line 1819 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 4389 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1198 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2053 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2030 of file kmmainwidget.cpp.
|
protectedslot |
Slot to reply to a message.
Definition at line 2010 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2196 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1374 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1729 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1737 of file kmmainwidget.cpp.
|
protectedslot |
etc.
Definition at line 2508 of file kmmainwidget.cpp.
|
protectedslot |
Trigger the dialog for editing out-of-office scripts.
Definition at line 2186 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1477 of file kmmainwidget.cpp.
|
slot |
Definition at line 412 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 3571 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 3561 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1548 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1437 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2427 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2476 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1284 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2395 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2400 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1250 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1618 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1600 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2089 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1277 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 3990 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1224 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1241 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1234 of file kmmainwidget.cpp.
|
slot |
Definition at line 2539 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2121 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1289 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2580 of file kmmainwidget.cpp.
|
slot |
Open a separate viewer window containing the specified message.
Definition at line 2515 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2586 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1590 of file kmmainwidget.cpp.
|
slot |
Opens mail in the internal viewer.
Definition at line 4255 of file kmmainwidget.cpp.
|
slot |
Definition at line 2561 of file kmmainwidget.cpp.
|
slot |
This will ask for a destination folder and move the currently selected messages (in MessageListView) into it.
Definition at line 1767 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1405 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 3543 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2257 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2227 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 4395 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1564 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1426 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 3552 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 3532 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1998 of file kmmainwidget.cpp.
|
slot |
Definition at line 4359 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1528 of file kmmainwidget.cpp.
|
slot |
Definition at line 2328 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1643 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2235 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2217 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1257 of file kmmainwidget.cpp.
|
slot |
Definition at line 2133 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2405 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2415 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2410 of file kmmainwidget.cpp.
|
slot |
Definition at line 1911 of file kmmainwidget.cpp.
|
protectedslot |
Message navigation.
Definition at line 2420 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2437 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2469 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2486 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2287 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2295 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1990 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1968 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1973 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1978 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1963 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1983 of file kmmainwidget.cpp.
|
protectedslot |
Show a splash screen for the longer-lasting operation.
Definition at line 2305 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1352 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 526 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 4005 of file kmmainwidget.cpp.
|
slot |
Definition at line 406 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2079 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2103 of file kmmainwidget.cpp.
|
slot |
Implements the "move to trash" action.
Definition at line 1882 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1890 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2112 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 418 of file kmmainwidget.cpp.
|
slot |
Adds if not existing/removes if existing the tag identified by aLabel
in all selected messages.
Definition at line 1927 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 2270 of file kmmainwidget.cpp.
|
protectedslot |
Update the undo action.
Definition at line 4050 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 1636 of file kmmainwidget.cpp.
|
inlineslot |
Definition at line 254 of file kmmainwidget.h.
|
slot |
Start a timer to update message actions.
Definition at line 3595 of file kmmainwidget.cpp.
KMail::TagActionManager * KMMainWidget::tagActionManager | ( | ) | const |
Definition at line 4245 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 4143 of file kmmainwidget.cpp.
|
protectedslot |
Update html and threaded messages preferences in Folder menu.
Definition at line 3918 of file kmmainwidget.cpp.
|
slot |
Update message actions.
Definition at line 3605 of file kmmainwidget.cpp.
|
slot |
Definition at line 3625 of file kmmainwidget.cpp.
|
slot |
Update message menu.
Definition at line 3590 of file kmmainwidget.cpp.
void KMMainWidget::updatePaneTagComboBox | ( | ) |
Definition at line 4382 of file kmmainwidget.cpp.
void KMMainWidget::updateQuickSearchLineText | ( | ) |
Definition at line 4423 of file kmmainwidget.cpp.
void KMMainWidget::updateVacationScriptStatus | ( | ) |
Definition at line 4240 of file kmmainwidget.cpp.
|
protectedslot |
Definition at line 4229 of file kmmainwidget.cpp.
QWidget * KMMainWidget::vacationScriptIndicator | ( | ) | const |
Definition at line 4235 of file kmmainwidget.cpp.
void KMMainWidget::writeConfig | ( | bool | force = true | ) |
Write configuration options.
Definition at line 872 of file kmmainwidget.cpp.
void KMMainWidget::writeFolderConfig | ( | ) |
Write configuration for current folder.
Definition at line 583 of file kmmainwidget.cpp.
void KMMainWidget::writeReaderConfig | ( | ) |
Definition at line 929 of file kmmainwidget.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:34:34 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.