ktimetracker
#include <taskview.h>
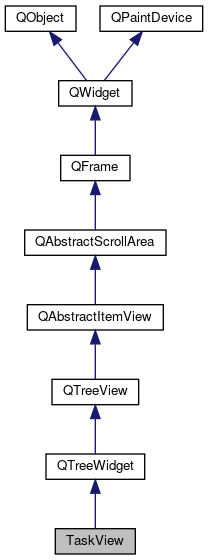
Signals | |
void | contextMenuRequested (const QPoint &) |
void | reSetTimes () |
void | setStatusBarText (QString) |
void | tasksChanged (QList< Task * > activeTasks) |
void | timersActive () |
void | timersInactive () |
void | totalTimesChanged (long session, long total) |
void | updateButtons () |
Public Member Functions | |
TaskView (QWidget *parent=0) | |
virtual | ~TaskView () |
QList< Task * > | activeTasks () const |
QString | addTask (const QString &taskame, const QString &taskdescription=QString(), long total=0, long session=0, const DesktopList &desktops=QVector< int >(0, 0), Task *parent=0) |
bool | allEventsHaveEndTiMe () |
void | closeStorage () |
long | count () |
Task * | currentItem () const |
QList< HistoryEvent > | getHistory (const QDate &from, const QDate &to) const |
bool | isFocusTrackingActive () const |
Task * | itemAt (int i) |
void | load (const QString &filename) |
void | resetDisplayTimeForAllTasks () |
void | resetTimeForAllTasks () |
void | scheduleSave () |
void | startNewSession () |
Task * | task (const QString &uid) |
QStringList | tasks () |
![]() | |
QTreeWidget (QWidget *parent) | |
~QTreeWidget () | |
void | addTopLevelItem (QTreeWidgetItem *item) |
void | addTopLevelItems (const QList< QTreeWidgetItem * > &items) |
void | clear () |
void | closePersistentEditor (QTreeWidgetItem *item, int column) |
void | collapseItem (const QTreeWidgetItem *item) |
int | columnCount () const |
int | currentColumn () const |
QTreeWidgetItem * | currentItem () const |
void | currentItemChanged (QTreeWidgetItem *current, QTreeWidgetItem *previous) |
void | editItem (QTreeWidgetItem *item, int column) |
void | expandItem (const QTreeWidgetItem *item) |
QList< QTreeWidgetItem * > | findItems (const QString &text, QFlags< Qt::MatchFlag > flags, int column) const |
QTreeWidgetItem * | headerItem () const |
int | indexOfTopLevelItem (QTreeWidgetItem *item) const |
void | insertTopLevelItem (int index, QTreeWidgetItem *item) |
void | insertTopLevelItems (int index, const QList< QTreeWidgetItem * > &items) |
QTreeWidgetItem * | invisibleRootItem () const |
bool | isFirstItemColumnSpanned (const QTreeWidgetItem *item) const |
bool | isItemExpanded (const QTreeWidgetItem *item) const |
bool | isItemHidden (const QTreeWidgetItem *item) const |
bool | isItemSelected (const QTreeWidgetItem *item) const |
QTreeWidgetItem * | itemAbove (const QTreeWidgetItem *item) const |
void | itemActivated (QTreeWidgetItem *item, int column) |
QTreeWidgetItem * | itemAt (const QPoint &p) const |
QTreeWidgetItem * | itemAt (int x, int y) const |
QTreeWidgetItem * | itemBelow (const QTreeWidgetItem *item) const |
void | itemChanged (QTreeWidgetItem *item, int column) |
void | itemClicked (QTreeWidgetItem *item, int column) |
void | itemCollapsed (QTreeWidgetItem *item) |
void | itemDoubleClicked (QTreeWidgetItem *item, int column) |
void | itemEntered (QTreeWidgetItem *item, int column) |
void | itemExpanded (QTreeWidgetItem *item) |
void | itemPressed (QTreeWidgetItem *item, int column) |
void | itemSelectionChanged () |
QWidget * | itemWidget (QTreeWidgetItem *item, int column) const |
void | openPersistentEditor (QTreeWidgetItem *item, int column) |
void | removeItemWidget (QTreeWidgetItem *item, int column) |
void | scrollToItem (const QTreeWidgetItem *item, QAbstractItemView::ScrollHint hint) |
QList< QTreeWidgetItem * > | selectedItems () const |
void | setColumnCount (int columns) |
void | setCurrentItem (QTreeWidgetItem *item) |
void | setCurrentItem (QTreeWidgetItem *item, int column) |
void | setCurrentItem (QTreeWidgetItem *item, int column, QFlags< QItemSelectionModel::SelectionFlag > command) |
void | setFirstItemColumnSpanned (const QTreeWidgetItem *item, bool span) |
void | setHeaderItem (QTreeWidgetItem *item) |
void | setHeaderLabel (const QString &label) |
void | setHeaderLabels (const QStringList &labels) |
void | setItemExpanded (const QTreeWidgetItem *item, bool expand) |
void | setItemHidden (const QTreeWidgetItem *item, bool hide) |
void | setItemSelected (const QTreeWidgetItem *item, bool select) |
void | setItemWidget (QTreeWidgetItem *item, int column, QWidget *widget) |
virtual void | setSelectionModel (QItemSelectionModel *selectionModel) |
int | sortColumn () const |
void | sortItems (int column, Qt::SortOrder order) |
QTreeWidgetItem * | takeTopLevelItem (int index) |
QTreeWidgetItem * | topLevelItem (int index) const |
int | topLevelItemCount () const |
QRect | visualItemRect (const QTreeWidgetItem *item) const |
![]() | |
QTreeView (QWidget *parent) | |
~QTreeView () | |
bool | allColumnsShowFocus () const |
int | autoExpandDelay () const |
void | collapse (const QModelIndex &index) |
void | collapseAll () |
void | collapsed (const QModelIndex &index) |
int | columnAt (int x) const |
int | columnViewportPosition (int column) const |
int | columnWidth (int column) const |
virtual void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight) |
void | expand (const QModelIndex &index) |
void | expandAll () |
void | expanded (const QModelIndex &index) |
bool | expandsOnDoubleClick () const |
void | expandToDepth (int depth) |
QHeaderView * | header () const |
void | hideColumn (int column) |
int | indentation () const |
QModelIndex | indexAbove (const QModelIndex &index) const |
virtual QModelIndex | indexAt (const QPoint &point) const |
QModelIndex | indexBelow (const QModelIndex &index) const |
bool | isAnimated () const |
bool | isColumnHidden (int column) const |
bool | isExpanded (const QModelIndex &index) const |
bool | isFirstColumnSpanned (int row, const QModelIndex &parent) const |
bool | isHeaderHidden () const |
bool | isRowHidden (int row, const QModelIndex &parent) const |
bool | isSortingEnabled () const |
bool | itemsExpandable () const |
virtual void | keyboardSearch (const QString &search) |
virtual void | reset () |
void | resizeColumnToContents (int column) |
bool | rootIsDecorated () const |
virtual void | scrollTo (const QModelIndex &index, ScrollHint hint) |
virtual void | selectAll () |
void | setAllColumnsShowFocus (bool enable) |
void | setAnimated (bool enable) |
void | setAutoExpandDelay (int delay) |
void | setColumnHidden (int column, bool hide) |
void | setColumnWidth (int column, int width) |
void | setExpanded (const QModelIndex &index, bool expanded) |
void | setExpandsOnDoubleClick (bool enable) |
void | setFirstColumnSpanned (int row, const QModelIndex &parent, bool span) |
void | setHeader (QHeaderView *header) |
void | setHeaderHidden (bool hide) |
void | setIndentation (int i) |
void | setItemsExpandable (bool enable) |
virtual void | setModel (QAbstractItemModel *model) |
virtual void | setRootIndex (const QModelIndex &index) |
void | setRootIsDecorated (bool show) |
void | setRowHidden (int row, const QModelIndex &parent, bool hide) |
void | setSortingEnabled (bool enable) |
void | setUniformRowHeights (bool uniform) |
void | setWordWrap (bool on) |
void | showColumn (int column) |
void | sortByColumn (int column) |
void | sortByColumn (int column, Qt::SortOrder order) |
bool | uniformRowHeights () const |
virtual QRect | visualRect (const QModelIndex &index) const |
bool | wordWrap () const |
![]() | |
QAbstractItemView (QWidget *parent) | |
~QAbstractItemView () | |
void | activated (const QModelIndex &index) |
bool | alternatingRowColors () const |
int | autoScrollMargin () const |
void | clearSelection () |
void | clicked (const QModelIndex &index) |
void | closePersistentEditor (const QModelIndex &index) |
QModelIndex | currentIndex () const |
Qt::DropAction | defaultDropAction () const |
void | doubleClicked (const QModelIndex &index) |
DragDropMode | dragDropMode () const |
bool | dragDropOverwriteMode () const |
bool | dragEnabled () const |
void | edit (const QModelIndex &index) |
EditTriggers | editTriggers () const |
void | entered (const QModelIndex &index) |
bool | hasAutoScroll () const |
ScrollMode | horizontalScrollMode () const |
QSize | iconSize () const |
virtual QModelIndex | indexAt (const QPoint &point) const =0 |
QWidget * | indexWidget (const QModelIndex &index) const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
QAbstractItemDelegate * | itemDelegate () const |
QAbstractItemDelegate * | itemDelegate (const QModelIndex &index) const |
QAbstractItemDelegate * | itemDelegateForColumn (int column) const |
QAbstractItemDelegate * | itemDelegateForRow (int row) const |
QAbstractItemModel * | model () const |
void | openPersistentEditor (const QModelIndex &index) |
void | pressed (const QModelIndex &index) |
QModelIndex | rootIndex () const |
virtual void | scrollTo (const QModelIndex &index, ScrollHint hint)=0 |
void | scrollToBottom () |
void | scrollToTop () |
QAbstractItemView::SelectionBehavior | selectionBehavior () const |
QAbstractItemView::SelectionMode | selectionMode () const |
QItemSelectionModel * | selectionModel () const |
void | setAlternatingRowColors (bool enable) |
void | setAutoScroll (bool enable) |
void | setAutoScrollMargin (int margin) |
void | setCurrentIndex (const QModelIndex &index) |
void | setDefaultDropAction (Qt::DropAction dropAction) |
void | setDragDropMode (DragDropMode behavior) |
void | setDragDropOverwriteMode (bool overwrite) |
void | setDragEnabled (bool enable) |
void | setDropIndicatorShown (bool enable) |
void | setEditTriggers (QFlags< QAbstractItemView::EditTrigger > triggers) |
void | setHorizontalScrollMode (ScrollMode mode) |
void | setIconSize (const QSize &size) |
void | setIndexWidget (const QModelIndex &index, QWidget *widget) |
void | setItemDelegate (QAbstractItemDelegate *delegate) |
void | setItemDelegateForColumn (int column, QAbstractItemDelegate *delegate) |
void | setItemDelegateForRow (int row, QAbstractItemDelegate *delegate) |
void | setSelectionBehavior (QAbstractItemView::SelectionBehavior behavior) |
void | setSelectionMode (QAbstractItemView::SelectionMode mode) |
void | setTabKeyNavigation (bool enable) |
void | setTextElideMode (Qt::TextElideMode mode) |
void | setVerticalScrollMode (ScrollMode mode) |
bool | showDropIndicator () const |
QSize | sizeHintForIndex (const QModelIndex &index) const |
virtual int | sizeHintForRow (int row) const |
bool | tabKeyNavigation () const |
Qt::TextElideMode | textElideMode () const |
void | update (const QModelIndex &index) |
ScrollMode | verticalScrollMode () const |
void | viewportEntered () |
virtual QRect | visualRect (const QModelIndex &index) const =0 |
![]() | |
QAbstractScrollArea (QWidget *parent) | |
~QAbstractScrollArea () | |
void | addScrollBarWidget (QWidget *widget, QFlags< Qt::AlignmentFlag > alignment) |
QWidget * | cornerWidget () const |
QScrollBar * | horizontalScrollBar () const |
Qt::ScrollBarPolicy | horizontalScrollBarPolicy () const |
QSize | maximumViewportSize () const |
virtual QSize | minimumSizeHint () const |
QWidgetList | scrollBarWidgets (QFlags< Qt::AlignmentFlag > alignment) |
void | setCornerWidget (QWidget *widget) |
void | setHorizontalScrollBar (QScrollBar *scrollBar) |
void | setHorizontalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setVerticalScrollBar (QScrollBar *scrollBar) |
void | setVerticalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setViewport (QWidget *widget) |
virtual QSize | sizeHint () const |
QScrollBar * | verticalScrollBar () const |
Qt::ScrollBarPolicy | verticalScrollBarPolicy () const |
QWidget * | viewport () const |
![]() | |
QFrame (QWidget *parent, QFlags< Qt::WindowType > f) | |
QFrame (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QFrame () | |
QRect | frameRect () const |
Shadow | frameShadow () const |
Shape | frameShape () const |
int | frameStyle () const |
int | frameWidth () const |
int | lineWidth () const |
int | midLineWidth () const |
void | setFrameRect (const QRect &) |
void | setFrameShadow (Shadow) |
void | setFrameShape (Shape) |
void | setFrameStyle (int style) |
void | setLineWidth (int) |
void | setMidLineWidth (int) |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Protected Slots | |
void | dropEvent (QDropEvent *event) |
void | iCalFileModified () |
void | itemStateChanged (QTreeWidgetItem *item) |
void | minuteUpdate () |
void | newFocusWindowDetected (const QString &) |
void | slotColumnToggled (int) |
void | slotCustomContextMenuRequested (const QPoint &) |
void | slotItemDoubleClicked (QTreeWidgetItem *item, int) |
void | slotSetPercentage (QAction *) |
void | slotSetPriority (QAction *) |
Protected Member Functions | |
void | mouseMoveEvent (QMouseEvent *) |
void | mousePressEvent (QMouseEvent *) |
![]() | |
virtual void | dropEvent (QDropEvent *event) |
virtual bool | dropMimeData (QTreeWidgetItem *parent, int index, const QMimeData *data, Qt::DropAction action) |
virtual bool | event (QEvent *e) |
QModelIndex | indexFromItem (QTreeWidgetItem *item, int column) const |
QTreeWidgetItem * | itemFromIndex (const QModelIndex &index) const |
QList< QTreeWidgetItem * > | items (const QMimeData *data) const |
virtual QMimeData * | mimeData (const QList< QTreeWidgetItem * > items) const |
virtual QStringList | mimeTypes () const |
virtual Qt::DropActions | supportedDropActions () const |
![]() | |
void | columnCountChanged (int oldCount, int newCount) |
void | columnMoved () |
void | columnResized (int column, int oldSize, int newSize) |
virtual void | currentChanged (const QModelIndex ¤t, const QModelIndex &previous) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | drawBranches (QPainter *painter, const QRect &rect, const QModelIndex &index) const |
virtual void | drawRow (QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const |
void | drawTree (QPainter *painter, const QRegion ®ion) const |
virtual int | horizontalOffset () const |
int | indexRowSizeHint (const QModelIndex &index) const |
virtual bool | isIndexHidden (const QModelIndex &index) const |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual QModelIndex | moveCursor (CursorAction cursorAction, QFlags< Qt::KeyboardModifier > modifiers) |
virtual void | paintEvent (QPaintEvent *event) |
int | rowHeight (const QModelIndex &index) const |
virtual void | rowsAboutToBeRemoved (const QModelIndex &parent, int start, int end) |
virtual void | rowsInserted (const QModelIndex &parent, int start, int end) |
void | rowsRemoved (const QModelIndex &parent, int start, int end) |
virtual void | scrollContentsBy (int dx, int dy) |
virtual QModelIndexList | selectedIndexes () const |
virtual void | selectionChanged (const QItemSelection &selected, const QItemSelection &deselected) |
virtual void | setSelection (const QRect &rect, QFlags< QItemSelectionModel::SelectionFlag > command) |
virtual int | sizeHintForColumn (int column) const |
virtual void | timerEvent (QTimerEvent *event) |
virtual void | updateGeometries () |
virtual int | verticalOffset () const |
virtual bool | viewportEvent (QEvent *event) |
virtual QRegion | visualRegionForSelection (const QItemSelection &selection) const |
![]() | |
virtual void | closeEditor (QWidget *editor, QAbstractItemDelegate::EndEditHint hint) |
virtual void | commitData (QWidget *editor) |
QPoint | dirtyRegionOffset () const |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
DropIndicatorPosition | dropIndicatorPosition () const |
virtual bool | edit (const QModelIndex &index, EditTrigger trigger, QEvent *event) |
virtual void | editorDestroyed (QObject *editor) |
void | executeDelayedItemsLayout () |
virtual void | focusInEvent (QFocusEvent *event) |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
virtual int | horizontalOffset () const =0 |
int | horizontalStepsPerItem () const |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual bool | isIndexHidden (const QModelIndex &index) const =0 |
virtual QModelIndex | moveCursor (CursorAction cursorAction, QFlags< Qt::KeyboardModifier > modifiers)=0 |
virtual void | resizeEvent (QResizeEvent *event) |
void | scheduleDelayedItemsLayout () |
void | scrollDirtyRegion (int dx, int dy) |
virtual QItemSelectionModel::SelectionFlags | selectionCommand (const QModelIndex &index, const QEvent *event) const |
void | setDirtyRegion (const QRegion ®ion) |
void | setHorizontalStepsPerItem (int steps) |
virtual void | setSelection (const QRect &rect, QFlags< QItemSelectionModel::SelectionFlag > flags)=0 |
void | setState (State state) |
void | setVerticalStepsPerItem (int steps) |
virtual void | startDrag (QFlags< Qt::DropAction > supportedActions) |
State | state () const |
virtual int | verticalOffset () const =0 |
int | verticalStepsPerItem () const |
virtual QStyleOptionViewItem | viewOptions () const |
virtual QRegion | visualRegionForSelection (const QItemSelection &selection) const =0 |
![]() | |
virtual void | contextMenuEvent (QContextMenuEvent *e) |
void | setupViewport (QWidget *viewport) |
void | setViewportMargins (const QMargins &margins) |
void | setViewportMargins (int left, int top, int right, int bottom) |
virtual void | wheelEvent (QWheelEvent *e) |
![]() | |
virtual void | changeEvent (QEvent *ev) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | enterEvent (QEvent *event) |
bool | focusNextChild () |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
![]() | |
QPaintDevice () | |
Detailed Description
Container and interface for the tasks.
Definition at line 50 of file taskview.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 163 of file taskview.cpp.
|
virtual |
Definition at line 392 of file taskview.cpp.
Member Function Documentation
Returns a list of the current active tasks.
Definition at line 1186 of file taskview.cpp.
QString TaskView::addTask | ( | const QString & | taskame, |
const QString & | taskdescription = QString() , |
||
long | total = 0 , |
||
long | session = 0 , |
||
const DesktopList & | desktops = QVector<int>(0,0) , |
||
Task * | parent = 0 |
||
) |
Add a task to view and storage.
Definition at line 902 of file taskview.cpp.
bool TaskView::allEventsHaveEndTiMe | ( | ) |
Deliver if all events from the storage have and end time.
Definition at line 508 of file taskview.cpp.
|
slot |
clears all active tasks.
Needed e.g. if iCal file was modified by another program and taskview is cleared without stopping tasks IF YOU DO NOT KNOW WHAT YOU ARE DOING, CALL stopAllTimers INSTEAD
Definition at line 740 of file taskview.cpp.
|
slot |
Copy totals for current and all sub tasks to clipboard.
Definition at line 1079 of file taskview.cpp.
void TaskView::closeStorage | ( | ) |
Close the storage and release lock.
Definition at line 503 of file taskview.cpp.
|
signal |
long TaskView::count | ( | ) |
Return the total number of items in the view.
Definition at line 653 of file taskview.cpp.
Task * TaskView::currentItem | ( | ) | const |
Return the current item in the view, cast to a Task pointer.
Definition at line 399 of file taskview.cpp.
|
slot |
Deletes the given or the current task (and children) from the view.
Depending on configuration, there may be a user dialog.
- Parameters
-
task Task to be deleted. If empty, the current task is deleted. if non-existent, an error message is displayed.
Definition at line 1018 of file taskview.cpp.
|
slot |
Deletes the given or the current task (and children) from the view.
It does this in batch mode, no user dialog.
- Parameters
-
task Task to be deleted. If empty, the current task is deleted. if non-existent, an error message is displayed.
Definition at line 1000 of file taskview.cpp.
|
slot |
receiving signal that a task is being deleted
Definition at line 1063 of file taskview.cpp.
|
protectedslot |
Definition at line 687 of file taskview.cpp.
|
slot |
Calls editTask dialog for the current task.
Definition at line 939 of file taskview.cpp.
|
slot |
Export comma separated values format for task time totals.
Definition at line 622 of file taskview.cpp.
|
slot |
Export comma-separated values format for task history.
Definition at line 637 of file taskview.cpp.
QList<HistoryEvent> TaskView::getHistory | ( | const QDate & | from, |
const QDate & | to | ||
) | const |
Return list of start/stop events for given date range.
|
protectedslot |
React on another process having modified the iCal file we rely on.
This is not iCalFileChanged.
Definition at line 513 of file taskview.cpp.
|
slot |
used to import tasks from imendio planner
Definition at line 602 of file taskview.cpp.
bool TaskView::isFocusTrackingActive | ( | ) | const |
Returns whether the focus tracking is currently active.
Definition at line 1181 of file taskview.cpp.
Task * TaskView::itemAt | ( | int | i | ) |
Return the i'th item (zero-based), cast to a Task pointer.
Definition at line 405 of file taskview.cpp.
|
protectedslot |
item state stores if a task is expanded so you can see the subtasks
Definition at line 494 of file taskview.cpp.
void TaskView::load | ( | const QString & | filename | ) |
Load the view from storage.
Definition at line 422 of file taskview.cpp.
|
slot |
Definition at line 1045 of file taskview.cpp.
|
slot |
Definition at line 1074 of file taskview.cpp.
|
protectedslot |
Definition at line 854 of file taskview.cpp.
|
protectedvirtual |
Reimplemented from QTreeView.
Definition at line 316 of file taskview.cpp.
|
protectedvirtual |
Reimplemented from QTreeView.
Definition at line 351 of file taskview.cpp.
|
protectedslot |
React on the focus having changed to Window QString.
Definition at line 275 of file taskview.cpp.
|
slot |
Calls newTask dialog with caption "New Sub Task".
Definition at line 929 of file taskview.cpp.
|
slot |
Calls newTask dialog with caption "New Task".
Definition at line 865 of file taskview.cpp.
Display edit task dialog and create a new task with results.
- Parameters
-
caption Window title of the edit task dialog
Definition at line 870 of file taskview.cpp.
|
slot |
Reconfigures taskView depending on current configuration.
Definition at line 1191 of file taskview.cpp.
|
slot |
|
slot |
Refresh the times of the tasks, e.g.
when the history has been changed by the user
Definition at line 548 of file taskview.cpp.
|
slot |
call export function for csv totals or history
Definition at line 617 of file taskview.cpp.
void TaskView::resetDisplayTimeForAllTasks | ( | ) |
Reset session and total time for all tasks - do not touch the storage.
Definition at line 814 of file taskview.cpp.
void TaskView::resetTimeForAllTasks | ( | ) |
Reset session and total time to zero for all tasks.
Definition at line 799 of file taskview.cpp.
|
signal |
|
slot |
Save to persistent storage.
Definition at line 698 of file taskview.cpp.
void TaskView::scheduleSave | ( | ) |
Schedule that we should save very soon.
Definition at line 693 of file taskview.cpp.
Set the text of the application's clipboard.
Definition at line 1090 of file taskview.cpp.
|
slot |
Sets % completed for the current task.
- Parameters
-
completion The percentage complete to mark the task as.
Definition at line 981 of file taskview.cpp.
|
signal |
|
protectedslot |
Definition at line 1117 of file taskview.cpp.
|
protectedslot |
Definition at line 1143 of file taskview.cpp.
|
protectedslot |
Definition at line 1097 of file taskview.cpp.
|
protectedslot |
Definition at line 1164 of file taskview.cpp.
|
protectedslot |
Definition at line 1173 of file taskview.cpp.
|
slot |
Start the timer on the current item (task) in view.
Definition at line 716 of file taskview.cpp.
void TaskView::startNewSession | ( | ) |
Reset session time to zero for all tasks.
Definition at line 783 of file taskview.cpp.
|
slot |
starts timer for task.
- Parameters
-
task task to start timer of startTime if taskview has been modified by another program, we have to set the starting time to not-now.
Definition at line 721 of file taskview.cpp.
|
slot |
Stop all running timers.
- Parameters
-
when When the timer stopped - this makes sense if the idletime- detector detects the user stopped working 5 minutes ago.
Definition at line 745 of file taskview.cpp.
|
slot |
Stop the timer for the current item in the view.
Definition at line 845 of file taskview.cpp.
|
slot |
Definition at line 828 of file taskview.cpp.
|
slot |
Returns a pointer to storage object.
This is poor object oriented design–the task view should expose wrappers around the storage methods we want to access instead of giving clients full access to objects that we own.
Hopefully, this will be redesigned as part of the Qt4 migration.
Definition at line 387 of file taskview.cpp.
|
slot |
Subtracts time from all active tasks, and does not log event.
Definition at line 1058 of file taskview.cpp.
return the task with the given UID
Definition at line 677 of file taskview.cpp.
QStringList TaskView::tasks | ( | ) |
|
inlineslot |
Definition at line 197 of file taskview.h.
|
signal |
|
signal |
|
slot |
Toggles the automatic tracking of focused windows.
Definition at line 766 of file taskview.cpp.
|
signal |
|
signal |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:31:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.