messageviewer
#include <viewer.h>
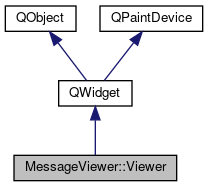
Public Types | |
enum | AttachmentAction { Open = 1, OpenWith = 2, View = 3, Save = 4, Properties = 5, ChiasmusEncrypt = 6, Delete = 7, Edit = 8, Copy = 9, ScrollTo = 10 } |
enum | DisplayFormatMessage { UseGlobalSetting = 0, Text = 1, Html = 2 } |
enum | ResourceOnlineMode { AllResources = 0, SelectedResource = 1 } |
enum | UpdateMode { Force = 0, Delayed } |
Public Slots | |
void | slotAttachmentSaveAll () |
void | slotAttachmentSaveAs () |
void | slotChangeDisplayMail (Viewer::DisplayFormatMessage, bool) |
void | slotFind () |
void | slotJumpDown () |
void | slotSaveMessage () |
void | slotScrollDown (int pixels=10) |
void | slotScrollNext () |
void | slotScrollPrior () |
void | slotScrollUp (int pixels=10) |
void | slotShowMessageSource () |
void | slotTranslate () |
void | slotZoomIn () |
void | slotZoomOut () |
void | slotZoomReset () |
Signals | |
void | deleteMessage (const Akonadi::Item &) |
void | itemRemoved () |
void | makeResourceOnline (MessageViewer::Viewer::ResourceOnlineMode mode) |
void | moveMessageToTrash () |
void | popupMenu (const Akonadi::Item &msg, const KUrl &url, const KUrl &imageUrl, const QPoint &mousePos) |
void | replaceMsgByUnencryptedVersion () |
void | requestConfigSync () |
void | showMessage (KMime::Message::Ptr message, const QString &encoding) |
void | showReader (KMime::Content *aMsgPart, bool aHTML, const QString &encoding) |
void | showStatusBarMessage (const QString &message) |
void | urlClicked (const Akonadi::Item &, const KUrl &) |
Public Member Functions | |
Viewer (QWidget *parent, QWidget *mainWindow=0, KActionCollection *actionCollection=0, Qt::WindowFlags f=0) | |
virtual | ~Viewer () |
bool | adblockEnabled () const |
void | addMessageLoadedHandler (AbstractMessageLoadedHandler *handler) |
bool | atBottom () const |
const AttachmentStrategy * | attachmentStrategy () const |
KAction * | blockImage () |
void | clear (UpdateMode updateMode=Delayed) |
void | clearSelection () |
QWidget * | configWidget () |
KAction * | copyAction () |
KAction * | copyImageLocation () |
void | copySelectionToClipboard () |
KAction * | copyURLAction () |
KAction * | createEventAction () |
KAction * | createTodoAction () |
CSSHelper * | cssHelper () const |
void | deleteMessage () |
Viewer::DisplayFormatMessage | displayFormatMessageOverwrite () const |
void | displaySplashPage (const QString &info) |
void | enableMessageDisplay () |
KAction * | expandShortUrlAction () |
KAction * | findInMessageAction () |
HeaderStrategy * | headerStrategy () const |
HeaderStyle * | headerStyle () const |
bool | htmlLoadExternal () const |
bool | htmlLoadExtOverride () const |
bool | htmlMail () const |
KUrl | imageUrlClicked () const |
bool | isAShortUrl (const KUrl &url) const |
bool | isFixedFont () const |
QWidget * | mainWindow () |
KMime::Message::Ptr | message () const |
Akonadi::Item | messageItem () const |
QString | messagePath () const |
QAbstractItemModel * | messageTreeModel () const |
bool | mimePartTreeIsEmpty () const |
KAction * | openBlockableItems () |
QString | overrideEncoding () const |
void | print () |
void | printMessage (const Akonadi::Item &msg) |
void | printPreview () |
void | printPreviewMessage (const Akonadi::Item &message) |
void | readConfig () |
void | removeMessageLoadedHandler (AbstractMessageLoadedHandler *handler) |
KAction * | resetMessageDisplayFormatAction () |
KAction * | saveAsAction () |
void | saveMainFrameScreenshotInFile (const QString &filename) |
KAction * | saveMessageDisplayFormatAction () |
Qt::ScrollBarPolicy | scrollBarPolicy (Qt::Orientation orientation) const |
void | selectAll () |
KAction * | selectAllAction () |
QString | selectedText () const |
void | setAppName (const QString &appName) |
void | setAttachmentStrategy (const AttachmentStrategy *strategy) |
void | setDecryptMessageOverwrite (bool overwrite=true) |
void | setDisplayFormatMessageOverwrite (Viewer::DisplayFormatMessage format) |
void | setExternalWindow (bool b) |
void | setHeaderStyleAndStrategy (HeaderStyle *style, HeaderStrategy *strategy) |
void | setHtmlLoadExtOverride (bool override) |
void | setMessage (KMime::Message::Ptr message, UpdateMode updateMode=Delayed) |
void | setMessageItem (const Akonadi::Item &item, UpdateMode updateMode=Delayed) |
void | setMessagePart (KMime::Content *aMsgPart) |
void | setMessagePath (const QString &path) |
void | setOverrideEncoding (const QString &encoding) |
void | setPrinting (bool enable) |
void | setScrollBarPolicy (Qt::Orientation orientation, Qt::ScrollBarPolicy policy) |
void | setUseFixedFont (bool useFixedFont) |
void | setZoomFactor (qreal zoomFactor) |
void | setZoomTextOnly (bool textOnly) |
void | showOpenAttachmentFolderWidget (const KUrl &url) |
KAction * | speakTextAction () |
KToggleAction * | toggleFixFontAction () |
KToggleAction * | toggleMimePartTreeAction () |
KAction * | translateAction () |
void | update (UpdateMode updateMode=Delayed) |
KUrl | urlClicked () const |
KAction * | urlOpenAction () |
KAction * | viewSourceAction () |
void | writeConfig (bool withSync=true) |
bool | zoomTextOnly () const |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
virtual QSize | minimumSizeHint () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
virtual QSize | sizeHint () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Static Public Member Functions | |
static Akonadi::ItemFetchJob * | createFetchJob (const Akonadi::Item &item) |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
Protected Member Functions | |
virtual void | closeEvent (QCloseEvent *) |
virtual bool | event (QEvent *e) |
virtual void | resizeEvent (QResizeEvent *) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual void | paintEvent (QPaintEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual void | wheelEvent (QWheelEvent *event) |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
Protected Attributes | |
ViewerPrivate *const | d_ptr |
Additional Inherited Members | |
![]() | |
typedef | RenderFlags |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
acceptDrops | |
accessibleDescription | |
accessibleName | |
autoFillBackground | |
baseSize | |
childrenRect | |
childrenRegion | |
contextMenuPolicy | |
cursor | |
enabled | |
focus | |
focusPolicy | |
font | |
frameGeometry | |
frameSize | |
fullScreen | |
geometry | |
height | |
inputMethodHints | |
isActiveWindow | |
layoutDirection | |
locale | |
maximized | |
maximumHeight | |
maximumSize | |
maximumWidth | |
minimized | |
minimumHeight | |
minimumSize | |
minimumSizeHint | |
minimumWidth | |
modal | |
mouseTracking | |
normalGeometry | |
palette | |
pos | |
rect | |
size | |
sizeHint | |
sizeIncrement | |
sizePolicy | |
statusTip | |
styleSheet | |
toolTip | |
updatesEnabled | |
visible | |
whatsThis | |
width | |
windowFilePath | |
windowFlags | |
windowIcon | |
windowIconText | |
windowModality | |
windowModified | |
windowOpacity | |
windowTitle | |
x | |
y | |
![]() | |
objectName | |
Detailed Description
This is the main widget for the viewer.
See the documentation of ViewerPrivate for implementation details. See Mainpage.dox for an overview of the classes in the messageviewer library.
Member Enumeration Documentation
Constructor & Destructor Documentation
|
explicit |
Create a mail viewer widget.
- Parameters
-
parent parent widget mainWindow the application's main window actionCollection the action collection where the widget's actions will belong to f window flags
Definition at line 52 of file viewer.cpp.
|
virtual |
Definition at line 60 of file viewer.cpp.
Member Function Documentation
bool MessageViewer::Viewer::adblockEnabled | ( | ) | const |
Definition at line 670 of file viewer.cpp.
void MessageViewer::Viewer::addMessageLoadedHandler | ( | AbstractMessageLoadedHandler * | handler | ) |
Adds a handler
for actions that will be executed when the message has been loaded into the view.
Definition at line 555 of file viewer.cpp.
bool MessageViewer::Viewer::atBottom | ( | ) | const |
Returns true if the message view is scrolled to the bottom.
Definition at line 211 of file viewer.cpp.
const AttachmentStrategy * MessageViewer::Viewer::attachmentStrategy | ( | ) | const |
Definition at line 353 of file viewer.cpp.
KAction * MessageViewer::Viewer::blockImage | ( | ) |
Definition at line 664 of file viewer.cpp.
|
inline |
void MessageViewer::Viewer::clearSelection | ( | ) |
Definition at line 584 of file viewer.cpp.
|
protectedvirtual |
Some necessary event handling.
Reimplemented from QWidget.
Definition at line 174 of file viewer.cpp.
QWidget * MessageViewer::Viewer::configWidget | ( | ) |
Get an instance for the configuration widget.
The caller has the ownership and must delete the widget. See also configObject(); The caller should also call the widget's slotSettingsChanged() if the configuration has changed.
Definition at line 308 of file viewer.cpp.
KAction * MessageViewer::Viewer::copyAction | ( | ) |
Definition at line 446 of file viewer.cpp.
KAction * MessageViewer::Viewer::copyImageLocation | ( | ) |
Definition at line 458 of file viewer.cpp.
void MessageViewer::Viewer::copySelectionToClipboard | ( | ) |
Definition at line 591 of file viewer.cpp.
KAction * MessageViewer::Viewer::copyURLAction | ( | ) |
Definition at line 440 of file viewer.cpp.
KAction * MessageViewer::Viewer::createEventAction | ( | ) |
Definition at line 703 of file viewer.cpp.
|
static |
Create an item fetch job that is suitable for using to fetch the message item that will be displayed on this viewer.
It will set the correct fetch scope. You still need to connect to the job's result signal.
Definition at line 539 of file viewer.cpp.
KAction * MessageViewer::Viewer::createTodoAction | ( | ) |
Definition at line 697 of file viewer.cpp.
CSSHelper * MessageViewer::Viewer::cssHelper | ( | ) | const |
Definition at line 378 of file viewer.cpp.
void MessageViewer::Viewer::deleteMessage | ( | ) |
Initiates a delete, by sending a signal to delete the message item.
Definition at line 572 of file viewer.cpp.
|
signal |
Viewer::DisplayFormatMessage MessageViewer::Viewer::displayFormatMessageOverwrite | ( | ) | const |
Get the html override setting.
Definition at line 242 of file viewer.cpp.
void MessageViewer::Viewer::displaySplashPage | ( | const QString & | info | ) |
Display a generic HTML splash page instead of a message.
- Parameters
-
info - the text to be displayed in HTML format
Definition at line 125 of file viewer.cpp.
void MessageViewer::Viewer::enableMessageDisplay | ( | ) |
Enable the displaying of messages again after an splash (or other) page was displayed.
Definition at line 131 of file viewer.cpp.
|
protectedvirtual |
KAction * MessageViewer::Viewer::expandShortUrlAction | ( | ) |
Definition at line 691 of file viewer.cpp.
KAction * MessageViewer::Viewer::findInMessageAction | ( | ) |
Definition at line 633 of file viewer.cpp.
HeaderStrategy * MessageViewer::Viewer::headerStrategy | ( | ) | const |
Definition at line 409 of file viewer.cpp.
HeaderStyle * MessageViewer::Viewer::headerStyle | ( | ) | const |
Definition at line 415 of file viewer.cpp.
bool MessageViewer::Viewer::htmlLoadExternal | ( | ) | const |
Is loading ext.
references to be supported? Takes into account override
Definition at line 278 of file viewer.cpp.
bool MessageViewer::Viewer::htmlLoadExtOverride | ( | ) | const |
Get the load external references override setting.
Definition at line 266 of file viewer.cpp.
bool MessageViewer::Viewer::htmlMail | ( | ) | const |
Is html mail to be supported? Takes into account override.
Definition at line 272 of file viewer.cpp.
KUrl MessageViewer::Viewer::imageUrlClicked | ( | ) | const |
Definition at line 500 of file viewer.cpp.
bool MessageViewer::Viewer::isAShortUrl | ( | const KUrl & | url | ) | const |
Definition at line 685 of file viewer.cpp.
bool MessageViewer::Viewer::isFixedFont | ( | ) | const |
Definition at line 284 of file viewer.cpp.
|
signal |
Emitted when the item, previously set with setMessageItem, has been removed.
QWidget * MessageViewer::Viewer::mainWindow | ( | ) |
Definition at line 296 of file viewer.cpp.
|
signal |
KMime::Message::Ptr MessageViewer::Viewer::message | ( | ) | const |
Returns the current message displayed in the viewer.
Definition at line 316 of file viewer.cpp.
Akonadi::Item MessageViewer::Viewer::messageItem | ( | ) | const |
Returns the current message item displayed in the viewer.
Definition at line 322 of file viewer.cpp.
QString MessageViewer::Viewer::messagePath | ( | ) | const |
The path to the message in terms of Akonadi collection hierarchy.
Definition at line 113 of file viewer.cpp.
QAbstractItemModel * MessageViewer::Viewer::messageTreeModel | ( | ) | const |
A QAIM tree model of the message structure.
Definition at line 530 of file viewer.cpp.
bool MessageViewer::Viewer::mimePartTreeIsEmpty | ( | ) | const |
Definition at line 391 of file viewer.cpp.
|
signal |
KAction * MessageViewer::Viewer::openBlockableItems | ( | ) |
Definition at line 679 of file viewer.cpp.
QString MessageViewer::Viewer::overrideEncoding | ( | ) | const |
Definition at line 365 of file viewer.cpp.
|
signal |
The user presses the right mouse button.
'url' may be 0.
void MessageViewer::Viewer::print | ( | ) |
Print the currently displayed message.
Definition at line 155 of file viewer.cpp.
void MessageViewer::Viewer::printMessage | ( | const Akonadi::Item & | msg | ) |
Sets a message as the current one and print it immediately.
- Parameters
-
message the message to display and print
Definition at line 137 of file viewer.cpp.
void MessageViewer::Viewer::printPreview | ( | ) |
Definition at line 149 of file viewer.cpp.
void MessageViewer::Viewer::printPreviewMessage | ( | const Akonadi::Item & | message | ) |
Definition at line 143 of file viewer.cpp.
void MessageViewer::Viewer::readConfig | ( | ) |
Definition at line 524 of file viewer.cpp.
void MessageViewer::Viewer::removeMessageLoadedHandler | ( | AbstractMessageLoadedHandler * | handler | ) |
Removes the handler
for actions that will be executed when the message has been loaded into the view.
Definition at line 565 of file viewer.cpp.
|
signal |
Emitted after parsing of a message to have it stored in unencrypted state in it's folder.
|
signal |
KAction * MessageViewer::Viewer::resetMessageDisplayFormatAction | ( | ) |
Definition at line 652 of file viewer.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 161 of file viewer.cpp.
KAction * MessageViewer::Viewer::saveAsAction | ( | ) |
Definition at line 470 of file viewer.cpp.
void MessageViewer::Viewer::saveMainFrameScreenshotInFile | ( | const QString & | filename | ) |
Definition at line 658 of file viewer.cpp.
KAction * MessageViewer::Viewer::saveMessageDisplayFormatAction | ( | ) |
Definition at line 646 of file viewer.cpp.
Qt::ScrollBarPolicy MessageViewer::Viewer::scrollBarPolicy | ( | Qt::Orientation | orientation | ) | const |
Returns the scrollbar policy for the scrollbar defined by orientation.
- See also
- setScrollBarPolicy()
void MessageViewer::Viewer::selectAll | ( | ) |
Definition at line 578 of file viewer.cpp.
KAction * MessageViewer::Viewer::selectAllAction | ( | ) |
Definition at line 403 of file viewer.cpp.
QString MessageViewer::Viewer::selectedText | ( | ) | const |
Definition at line 235 of file viewer.cpp.
void MessageViewer::Viewer::setAppName | ( | const QString & | appName | ) |
Set the application name that is shown when the splash screen is active.
- Parameters
-
appName - A QString that is set to the calling application name.
Definition at line 260 of file viewer.cpp.
void MessageViewer::Viewer::setAttachmentStrategy | ( | const AttachmentStrategy * | strategy | ) |
Definition at line 359 of file viewer.cpp.
void MessageViewer::Viewer::setDecryptMessageOverwrite | ( | bool | overwrite = true | ) |
Enforce message decryption.
Definition at line 302 of file viewer.cpp.
void MessageViewer::Viewer::setDisplayFormatMessageOverwrite | ( | Viewer::DisplayFormatMessage | format | ) |
Override default html mail setting.
Definition at line 248 of file viewer.cpp.
void MessageViewer::Viewer::setExternalWindow | ( | bool | b | ) |
Definition at line 428 of file viewer.cpp.
void MessageViewer::Viewer::setHeaderStyleAndStrategy | ( | HeaderStyle * | style, |
HeaderStrategy * | strategy | ||
) |
Definition at line 421 of file viewer.cpp.
void MessageViewer::Viewer::setHtmlLoadExtOverride | ( | bool | override | ) |
Override default load external references setting.
Definition at line 254 of file viewer.cpp.
void MessageViewer::Viewer::setMessage | ( | KMime::Message::Ptr | message, |
UpdateMode | updateMode = Delayed |
||
) |
Set the message that shall be shown.
- Parameters
-
msg - the message to be shown. If 0, an empty page is displayed. updateMode - update the display immediately or not. See UpdateMode.
Definition at line 90 of file viewer.cpp.
void MessageViewer::Viewer::setMessageItem | ( | const Akonadi::Item & | item, |
UpdateMode | updateMode = Delayed |
||
) |
Set the Akonadi item that will be displayed.
- Parameters
-
item - the Akonadi item to be displayed. If it doesn't hold a mail (KMime::Message::Ptr as payload data), an empty page is shown. updateMode - update the display immediately or not. See UpdateMode.
Definition at line 99 of file viewer.cpp.
void MessageViewer::Viewer::setMessagePart | ( | KMime::Content * | aMsgPart | ) |
Instead of settings a message to be shown sets a message part to be shown.
Definition at line 512 of file viewer.cpp.
void MessageViewer::Viewer::setMessagePath | ( | const QString & | path | ) |
Set the path to the message in terms of Akonadi collection hierarchy.
Definition at line 119 of file viewer.cpp.
void MessageViewer::Viewer::setOverrideEncoding | ( | const QString & | encoding | ) |
Definition at line 371 of file viewer.cpp.
void MessageViewer::Viewer::setPrinting | ( | bool | enable | ) |
Definition at line 482 of file viewer.cpp.
void MessageViewer::Viewer::setScrollBarPolicy | ( | Qt::Orientation | orientation, |
Qt::ScrollBarPolicy | policy | ||
) |
Sets the scrollbar policy for the scrollbar defined by orientation to policy.
- See also
- scrollBarPolicy()
Definition at line 549 of file viewer.cpp.
void MessageViewer::Viewer::setUseFixedFont | ( | bool | useFixedFont | ) |
Definition at line 290 of file viewer.cpp.
void MessageViewer::Viewer::setZoomFactor | ( | qreal | zoomFactor | ) |
Definition at line 597 of file viewer.cpp.
void MessageViewer::Viewer::setZoomTextOnly | ( | bool | textOnly | ) |
Definition at line 621 of file viewer.cpp.
|
signal |
Emitted when the message should be shown in a separate window.
void MessageViewer::Viewer::showOpenAttachmentFolderWidget | ( | const KUrl & | url | ) |
Definition at line 709 of file viewer.cpp.
|
signal |
Emitted when the content should be shown in a separate window.
|
signal |
Emitted when a status bar message is shown.
Note that the status bar message is also set to KPIM::BroadcastStatus in addition.
|
slot |
Definition at line 187 of file viewer.cpp.
|
slot |
Definition at line 181 of file viewer.cpp.
|
slot |
Definition at line 639 of file viewer.cpp.
|
slot |
Definition at line 340 of file viewer.cpp.
|
slot |
Definition at line 217 of file viewer.cpp.
|
slot |
Definition at line 193 of file viewer.cpp.
|
slot |
Definition at line 205 of file viewer.cpp.
|
slot |
Definition at line 229 of file viewer.cpp.
|
slot |
Definition at line 223 of file viewer.cpp.
|
slot |
HTML Widget scrollbar and layout handling.
Scrolling always happens in the direction of the slot that is called. I.e. the methods take the absolute value of
Definition at line 199 of file viewer.cpp.
|
slot |
Definition at line 518 of file viewer.cpp.
|
slot |
Definition at line 346 of file viewer.cpp.
|
slot |
Definition at line 609 of file viewer.cpp.
|
slot |
Definition at line 615 of file viewer.cpp.
|
slot |
Definition at line 603 of file viewer.cpp.
KAction * MessageViewer::Viewer::speakTextAction | ( | ) |
Definition at line 452 of file viewer.cpp.
KToggleAction * MessageViewer::Viewer::toggleFixFontAction | ( | ) |
Definition at line 385 of file viewer.cpp.
KToggleAction * MessageViewer::Viewer::toggleMimePartTreeAction | ( | ) |
Definition at line 397 of file viewer.cpp.
KAction * MessageViewer::Viewer::translateAction | ( | ) |
Definition at line 464 of file viewer.cpp.
void MessageViewer::Viewer::update | ( | UpdateMode | updateMode = Delayed | ) |
Definition at line 506 of file viewer.cpp.
KUrl MessageViewer::Viewer::urlClicked | ( | ) | const |
Definition at line 494 of file viewer.cpp.
|
signal |
The message viewer handles some types of urls itself, most notably http(s) and ftp(s).
When it can't handle the url it will emit this signal.
KAction * MessageViewer::Viewer::urlOpenAction | ( | ) |
Definition at line 476 of file viewer.cpp.
KAction * MessageViewer::Viewer::viewSourceAction | ( | ) |
Definition at line 434 of file viewer.cpp.
void MessageViewer::Viewer::writeConfig | ( | bool | withSync = true | ) |
Definition at line 488 of file viewer.cpp.
bool MessageViewer::Viewer::zoomTextOnly | ( | ) | const |
Definition at line 627 of file viewer.cpp.
Member Data Documentation
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:32:46 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.