PIMPrint Library
#include <calprintbase.h>
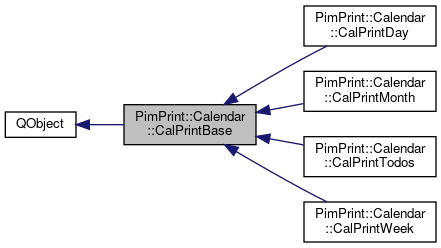
Public Types | |
enum | ExtraOption { ExtraSingleLine = 0x00000001, ExtraNoteLines = 0x00000002, ExtraWeekNumbers = 0x00000004, ExtraStrikeDoneTodos = 0x00000008, ExtraConnectSubTodos = 0x00000010, ExtraFooter = 0x00000020 } |
enum | InfoOption { InfoAll = 0x00000001, InfoDescription = 0x00000002, InfoLocation = 0x00000004, InfoAttendees = 0x00000008, InfoCategories = 0x00000010, InfoPriority = 0x00000020, InfoDueDate = 0x00000040, InfoPercentDone = 0x00000080, InfoTimeRange = 0x00000100 } |
enum | RangeOption { RangeTimeExpand = 0x00000001, RangeRecurAny = 0x00000002, RangeRecurDaily = 0x00000004, RangeRecurWeekly = 0x00000008 } |
enum | Style { None, Incidence, DayFiloFax, DayTimeTable, DaySingleTimeTable, WeekFiloFax, WeekTimeTable, WeekSplitWeek, MonthClassic, TodoList, Journal, Year } |
enum | TypeOption { TypeAll = 0x00000001, TypeEvent = 0x00000002, TypeTodo = 0x00000004, TypeJournal = 0x00000008, TypeConfidential = 0x00000010, TypePrivate = 0x00000020 } |
Public Member Functions | |
CalPrintBase (QPrinter *printer) | |
~CalPrintBase () | |
int | boxBorderWidth () const |
KCalendarSystem * | calendarSystem () const |
ExtraOptions | extraOptions () const |
int | footerHeight () const |
int | headerHeight () const |
InfoOptions | infoOptions () const |
int | itemBoxBorderWidth () const |
int | margins () const |
int | padding () const |
int | pageHeight () const |
int | pageWidth () const |
virtual void | print (QPainter &p)=0 |
KCalCore::Calendar::Ptr | printCalendar () const |
Style | printStyle () const |
RangeOptions | rangeOptions () const |
void | setCalendarSystem (KCalendarSystem *calSystem) |
void | setExtraOptions (ExtraOptions flags) |
void | setFooterHeight (const int height) |
void | setHeaderHeight (const int height) |
void | setInfoOptions (InfoOptions flags) |
void | setPadding (const int padding) |
void | setPageHeight (const int height) const |
void | setPageWidth (const int width) const |
void | setPrintCalendar (const KCalCore::Calendar::Ptr &calendar) |
void | setPrintStyle (const Style style) |
void | setRangeOptions (RangeOptions flags) |
void | setSubHeaderHeight (const int height) |
void | setThePrinter (QPrinter *printer) |
void | setTypeOptions (TypeOptions flags) |
void | setUseColor (const bool useColor) |
void | setUseLandscape (const bool landscape) |
int | subHeaderHeight () const |
QPrinter * | thePrinter () const |
int | timeLineWidth () const |
TypeOptions | typeOptions () const |
bool | useColor () const |
bool | useLandscape () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
QString | cleanString (const QString &str) const |
void | drawAgendaDayBox (QPainter &p, const QRect &box, const QDate &date, const KCalCore::Event::List &eventList, const QTime &startTime, const QTime &endTime, const QList< QDate > &workDays) const |
void | drawAgendaItem (PrintCellItem *item, QPainter &p, const KDateTime &startPrintDate, const KDateTime &endPrintDate, float minlen, const QRect &box) const |
void | drawBox (QPainter &p, const int linewidth, const QRect &box) const |
void | drawDayBox (QPainter &p, const QRect &box, const QDate &date, const QTime &startTime, const QTime &endTime, bool fullDate=false) const |
void | drawDaysOfWeek (QPainter &p, const QRect &box, const QDate &fromDate, const QDate &toDate) const |
int | drawFooter (QPainter &p, const QRect &box) const |
int | drawHeader (QPainter &p, const QRect &box, const QString &title, const QDate &leftMonth=QDate(), const QDate &rightMonth=QDate(), const bool expand=false, const QColor &backColor=QColor()) const |
void | drawItemBox (QPainter &p, int linewidth, const QRect &box, const KCalCore::Incidence::Ptr &incidence, const QString &str, int flags=-1) const |
void | drawItemString (QPainter &p, const QRect &box, const QString &str, int flags=-1) const |
void | drawShadedBox (QPainter &p, const int linewidth, const QBrush &brush, const QRect &box) const |
void | drawSubHeader (QPainter &p, const QRect &box, const QString &str) const |
void | drawTimeLine (QPainter &p, const QTime &startTime, const QTime &endTime, const QRect &box) const |
void | drawTimeTable (QPainter &p, const QRect &box, const QDate &startDate, const QDate &endDate, const QTime &startTime, const QTime &endTime, bool expandAll=false) const |
void | drawVerticalBox (QPainter &p, const int linewidth, const QRect &box, const QString &str, int flags=-1) const |
QString | toPlainText (const QString &htmlText) const |
int | weekdayColumn (int weekday) const |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Properties | |
int | boxBorderWidth |
int | footerHeight |
int | headerHeight |
int | itemBoxBorderWidth |
int | margins |
int | padding |
int | pageHeight |
int | pageWidth |
int | subheaderHeight |
int | timeLineWidth |
bool | useColor |
bool | useLandscape |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
Definition at line 67 of file calprintbase.h.
Member Enumeration Documentation
Enumerator | |
---|---|
ExtraSingleLine | |
ExtraNoteLines | |
ExtraWeekNumbers | |
ExtraStrikeDoneTodos | |
ExtraConnectSubTodos | |
ExtraFooter |
Definition at line 303 of file calprintbase.h.
Enumerator | |
---|---|
InfoAll | |
InfoDescription | |
InfoLocation | |
InfoAttendees | |
InfoCategories | |
InfoPriority | |
InfoDueDate | |
InfoPercentDone | |
InfoTimeRange |
Definition at line 272 of file calprintbase.h.
Enumerator | |
---|---|
RangeTimeExpand | |
RangeRecurAny | |
RangeRecurDaily | |
RangeRecurWeekly |
Definition at line 295 of file calprintbase.h.
Enumerator | |
---|---|
None | |
Incidence | |
DayFiloFax | |
DayTimeTable | |
DaySingleTimeTable | |
WeekFiloFax | |
WeekTimeTable | |
WeekSplitWeek | |
MonthClassic | |
TodoList | |
Journal | |
Year |
Definition at line 121 of file calprintbase.h.
Enumerator | |
---|---|
TypeAll | |
TypeEvent | |
TypeTodo | |
TypeJournal | |
TypeConfidential | |
TypePrivate |
Definition at line 285 of file calprintbase.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
Private class that helps to provide binary compatibility between releases.
Definition at line 119 of file calprintbase.cpp.
CalPrintBase::~CalPrintBase | ( | ) |
Destructor.
Definition at line 125 of file calprintbase.cpp.
Member Function Documentation
int PimPrint::Calendar::CalPrintBase::boxBorderWidth | ( | ) | const |
Returns the width of box borders.
Not settable. A nice default value is used.
KCalendarSystem * CalPrintBase::calendarSystem | ( | ) | const |
Returns a pointer to the currently set calendar system.
- See also
- setCalendarSystem()
Definition at line 188 of file calprintbase.cpp.
Cleans a string of newlines and other characters that shouldn't be printed.
The unwanted chars are either replaces with white-space are simply removed.
- Parameters
-
str is the QString to clean.
- Returns
- a QString with the unwanted characters replaced or removed.
Definition at line 1336 of file calprintbase.cpp.
|
protected |
Draws the agenda box.
Also draws a grid with half-hour spacing of the grid lines. Does NOT draw allday events. Use drawAllDayBox for allday events.
- Parameters
-
p QPainter of the printout date The date of the currently printed day eventList The list of the events that are supposed to be printed inside this box The height of the box will not be affected by this (but the height of one hour will be scaled down so that the whole range fits into by this function). startTime Start of the time range to be printed. Might be adjusted to include all events if expandable
is set to true.endTime End of the time range to be printed. Might be adjusted to include all events if expandable
is set to true.workDays List of workDays
Definition at line 724 of file calprintbase.cpp.
|
protected |
Definition at line 840 of file calprintbase.cpp.
Draws a box with given width at the given coordinates.
- Parameters
-
p The printer to be used. linewidth The border width of the box. box Coordinates of the box.
Definition at line 371 of file calprintbase.cpp.
|
protected |
Draws the box containing a list of all events and to-dos of the given day.
(with their timesof course). Used in the Filofax and the month print style.
- Parameters
-
p QPainter of the printout. box coordinates of the day box. date The date of the currently printed day. All events and to-dos occurring on this day will be printed. startTime Start time of the time range to display. endTime End time of the time range to display. fullDate Whether the title bar of the box should contain the full date string or just a short.
Definition at line 1141 of file calprintbase.cpp.
|
protected |
Draws a horizontal bar with the weekday names of the given date range in the given area of the painter.
Used for the weekday-bar on top of the timetable view and the month view.
- Parameters
-
p QPainter of the printout box coordinates of the box for the days of the week fromDate First date of the printed dates toDate Last date of the printed dates
Definition at line 926 of file calprintbase.cpp.
Draws a page footer containing the printing date and possibly other things, like a page number.
- Parameters
-
p QPainter of the printout box coordinates of the footer box.
- Returns
- The bottom of the printed box.
Definition at line 568 of file calprintbase.cpp.
|
protected |
Draws the gray header bar of the printout to the QPainter.
It prints the given text and optionally one or two small month views, as specified by the two QDate. The printed text can also contain a line feed. If month2 is invalid, only the month that contains month1 is printed. E.g. the filofax week view draws just the current month, while the month view draws the previous and the next month.
- Parameters
-
p QPainter of the printout. box coordinates of the header box. title The string printed as the title of the page. leftMonth The month-year to draw for the left one of the small month views in the title bar. If an invalid QDate, the left small month view will not be printed. rightMonth The month-year to draw for the right one of the small month views in the title bar. If an invalid QDate, the right small month view will not be printed. expand Whether to expand the box vertically to fit the whole title in it. backColor background color for the header box.
- Returns
- The bottom of the printed box. If expand==false, this is box.bottom, otherwise it is larger than box.bottom and matches the y-coordinate of the surrounding rectangle.
Definition at line 497 of file calprintbase.cpp.
|
protected |
Draws the box for the specified item with the given string.
- Parameters
-
p QPainter of the printout. linewidth is the width of the line used to draw the box. box Coordinates of the incidence's box. incidence The incidence (if available), from which the category color will be deduced, if applicable. str The string to print inside the box. flags is a bitwise OR of Qt::AlignmentFlags and Qt::TextFlags values.
Definition at line 404 of file calprintbase.cpp.
|
protected |
Draws the given string (incidence summary) in the given rectangle.
Margins and justification (centered or not) are automatically adjusted.
- Parameters
-
p QPainter of the printout. box Coordinates of the surrounding item box. str The text to be printed in the box.
Definition at line 395 of file calprintbase.cpp.
|
protected |
Draws a shaded box with given width at the given coordinates.
- Parameters
-
p The printer to be used. linewidth The border width of the box. brush The brush to fill the box. box Coordinates of the box.
Definition at line 386 of file calprintbase.cpp.
|
protected |
Draws a subheader with a shaded background and the specified string.
- Parameters
-
p QPainter of the printout. box Coordinates of the box. str Text to be printed inside the box.
Definition at line 558 of file calprintbase.cpp.
|
protected |
Draws a (vertical) time scale from time startTime to endTime inside the given area of the painter.
Every hour will have a one-pixel line over the whole width, every half-hour the line will only span the left half of the width. This is used in the day and timetable print styles
- Parameters
-
p QPainter of the printout. startTime Start time of the time range to display. endTime End time of the time range to display. box coordinates of the timeline.
Definition at line 583 of file calprintbase.cpp.
|
protected |
Draws the timetable view of the given time range from startDate to endDate.
On the left side the time scale is printed (using drawTimeLine), then each day gets one column (printed using drawAgendaDayBox), and the events are displayed as boxes (like in korganizer's day/week view).
The first cell of each column contains the all-day events (using drawAllDayBox with expandable=false).
- Parameters
-
p QPainter of the printout. box coordinates of the time table. startDate First day to be included in the page. endDate Last day to be included in the page. startTime Start time of the displayed time range. endTime End time of the displayed time range. expandAll If true, the start and end times are adjusted to include the whole range of all events of that day, not just of the given time range.
Definition at line 655 of file calprintbase.cpp.
|
protected |
Draws an event box with vertical text.
- Parameters
-
p QPainter of the printout linewidth is the width of the line used to draw the box, ignored if less than 1. box Coordinates of the box str ext to be printed inside the box flags is a bitwise OR of Qt::AlignmentFlags and Qt::TextFlags values.
Definition at line 425 of file calprintbase.cpp.
CalPrintBase::ExtraOptions CalPrintBase::extraOptions | ( | ) | const |
Returns the current extra option flags.
- See also
- setExtraOptions()
Definition at line 366 of file calprintbase.cpp.
int PimPrint::Calendar::CalPrintBase::footerHeight | ( | ) | const |
Returns the current height of the page footer.
If a height has not been set explicitly, then a default value based on the printer orientation is returned.
- Returns
- height of the page footer of the printout.
- See also
- setFooterHeight()
int PimPrint::Calendar::CalPrintBase::headerHeight | ( | ) | const |
Returns the current height of the page header.
If a height has not been set explicitly, then a default value based on the printer orientation is returned.
- Returns
- height of the page header of the printout.
- See also
- setHeaderHeight()
CalPrintBase::InfoOptions CalPrintBase::infoOptions | ( | ) | const |
Returns the current information option flags.
- See also
- setInfoOptions()
Definition at line 336 of file calprintbase.cpp.
int PimPrint::Calendar::CalPrintBase::itemBoxBorderWidth | ( | ) | const |
Returns the width of item box borders.
Not settable. A nice default value is used.
int PimPrint::Calendar::CalPrintBase::margins | ( | ) | const |
Returns the size of the page margins.
Not settable. The same margin is used on all 4 sides of the page. A nice default value is used.
int PimPrint::Calendar::CalPrintBase::padding | ( | ) | const |
Returns the current padding margin.
If this value has not been set explicitly, a nice default value is returned.
- Returns
- padding margin of of the printout.
- See also
- setPadding()
int PimPrint::Calendar::CalPrintBase::pageHeight | ( | ) | const |
Returns the current printed page height.
- See also
- setPageHeight().
int PimPrint::Calendar::CalPrintBase::pageWidth | ( | ) | const |
Returns the current printed page width.
- See also
- setPageWidth().
|
pure virtual |
KCalCore::Calendar::Ptr CalPrintBase::printCalendar | ( | ) | const |
Returns a pointer to the currently set print calendar.
- See also
- setPrintCalendar()
Definition at line 178 of file calprintbase.cpp.
CalPrintBase::Style CalPrintBase::printStyle | ( | ) | const |
Returns the current printing Style.
- See also
- setStyle()
Definition at line 198 of file calprintbase.cpp.
CalPrintBase::RangeOptions CalPrintBase::rangeOptions | ( | ) | const |
Returns the current time-range option flags.
- See also
- setRangeOptions()
Definition at line 356 of file calprintbase.cpp.
void CalPrintBase::setCalendarSystem | ( | KCalendarSystem * | calSystem | ) |
Sets the calendar system to use when printing.
- Parameters
-
calSystem is a pointer to a valid KCalendarSystem object.
- See also
- calendarSystem()
Definition at line 183 of file calprintbase.cpp.
void CalPrintBase::setExtraOptions | ( | ExtraOptions | flags | ) |
Sets extra options for printing.
- Parameters
-
flags bitwise ORd ExtraOption flags.
- See also
- extraFlags()
Definition at line 361 of file calprintbase.cpp.
void CalPrintBase::setFooterHeight | ( | const int | height | ) |
Sets the height of the page footer.
If the height is not explicitly set, a default value based on the printer orientation is used.
- See also
- footerHeight()
Definition at line 281 of file calprintbase.cpp.
void CalPrintBase::setHeaderHeight | ( | const int | height | ) |
Sets the height of the page header.
If the height is not explicitly set, a default value based on the printer orientation is used.
- See also
- headerHeight()
Definition at line 255 of file calprintbase.cpp.
void CalPrintBase::setInfoOptions | ( | InfoOptions | flags | ) |
Sets information options for printing.
- Parameters
-
flags bitwise ORd InfoOption flags.
- See also
- infoFlags()
Definition at line 331 of file calprintbase.cpp.
void CalPrintBase::setPadding | ( | const int | padding | ) |
Sets the padding margin.
If not explicitly set, a nice default value is used.
- See also
- padding()
Definition at line 301 of file calprintbase.cpp.
void CalPrintBase::setPageHeight | ( | const int | height | ) | const |
Sets the printed page height.
- Parameters
-
height is the height of the desired printed page.
- See also
- pageHeight()
Definition at line 203 of file calprintbase.cpp.
void CalPrintBase::setPageWidth | ( | const int | width | ) | const |
Sets the printed page width.
- Parameters
-
width is the width of the desired printed page.
- See also
- pageWidth()
Definition at line 219 of file calprintbase.cpp.
void CalPrintBase::setPrintCalendar | ( | const KCalCore::Calendar::Ptr & | calendar | ) |
Sets the calendar containing the data to print.
- Parameters
-
calendar is a pointer to a Calendar object containing the data to print.
- See also
- printCalendar()
Definition at line 173 of file calprintbase.cpp.
void CalPrintBase::setPrintStyle | ( | const Style | style | ) |
Sets the printing Style.
- Parameters
-
style is the Style of print desired.
- See also
- style()
Definition at line 193 of file calprintbase.cpp.
void CalPrintBase::setRangeOptions | ( | RangeOptions | flags | ) |
Sets time-range options for printing.
- Parameters
-
flags bitwise ORd RangeOption flags.
- See also
- rangeFlags()
Definition at line 351 of file calprintbase.cpp.
void CalPrintBase::setSubHeaderHeight | ( | const int | height | ) |
Sets the height of the page sub-header.
If the height is not explicitly set, a default value based on the printer orientation is used.
- See also
- subHeaderHeight()
Definition at line 271 of file calprintbase.cpp.
void CalPrintBase::setThePrinter | ( | QPrinter * | printer | ) |
Sets the QPrinter.
- Parameters
-
printer is a pointer to a valid QPrinter where the printout will be made.
- See also
- thePrinter()
Definition at line 163 of file calprintbase.cpp.
void CalPrintBase::setTypeOptions | ( | TypeOptions | flags | ) |
Sets type options for printing.
- Parameters
-
flags bitwise ORd TypeOption flags.
- See also
- typeFlags()
Definition at line 341 of file calprintbase.cpp.
void CalPrintBase::setUseColor | ( | const bool | useColor | ) |
Sets if a color print is desired.
- Parameters
-
useColor if true, then colors are used in the print; greyscale otherwise.
- See also
- useColor()
Definition at line 245 of file calprintbase.cpp.
void CalPrintBase::setUseLandscape | ( | const bool | landscape | ) |
Sets the printed page orientation to landscape.
- Parameters
-
landscape if true use landscape when printing; portrait otherwise.
- See also
- useLandscape()
Definition at line 235 of file calprintbase.cpp.
int CalPrintBase::subHeaderHeight | ( | ) | const |
Returns the current height of the page sub-header.
If a height has not been set explicitly, then a default value based on the printer orientation is returned.
- Returns
- height of the page sub-header of the printout.
- See also
- setSubHeaderHeight()
Definition at line 276 of file calprintbase.cpp.
QPrinter * CalPrintBase::thePrinter | ( | ) | const |
Returns a pointer to the currently set QPrinter.
- See also
- setThePrinter()
Definition at line 168 of file calprintbase.cpp.
int PimPrint::Calendar::CalPrintBase::timeLineWidth | ( | ) | const |
Returns the width of timelines.
Not settable. A nice default value is used.
Converts possible rich text to plain text.
- Parameters
-
htmlText is a QString containing possible RichText to convert.
- Returns
- a plain text string representation of the input string.
- See also
- cleanStr()
Definition at line 1342 of file calprintbase.cpp.
CalPrintBase::TypeOptions CalPrintBase::typeOptions | ( | ) | const |
Returns the current type option flags.
- See also
- setTypeOptions()
Definition at line 346 of file calprintbase.cpp.
bool PimPrint::Calendar::CalPrintBase::useColor | ( | ) | const |
Returns if a color printing is currently set.
- See also
- setUseColor()
bool PimPrint::Calendar::CalPrintBase::useLandscape | ( | ) | const |
Returns if the current printed page orientation is landscape.
- See also
- setUseLandscape()
|
protected |
Determines the column of the given weekday (1=Monday, 7=Sunday), taking the start of the week setting into account as given in the user's locale.
- Parameters
-
weekday Index of the weekday
Definition at line 1330 of file calprintbase.cpp.
Property Documentation
|
read |
Definition at line 102 of file calprintbase.h.
|
readwrite |
Definition at line 95 of file calprintbase.h.
|
readwrite |
Definition at line 89 of file calprintbase.h.
|
read |
Definition at line 104 of file calprintbase.h.
|
read |
Definition at line 100 of file calprintbase.h.
|
readwrite |
Definition at line 98 of file calprintbase.h.
|
readwrite |
Definition at line 86 of file calprintbase.h.
|
readwrite |
Definition at line 83 of file calprintbase.h.
|
readwrite |
Definition at line 92 of file calprintbase.h.
|
read |
Definition at line 106 of file calprintbase.h.
|
readwrite |
Definition at line 77 of file calprintbase.h.
|
readwrite |
Definition at line 80 of file calprintbase.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:32:09 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.