akonadi
#include <entity.h>
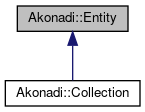
Public Types | |
enum | CreateOption { AddIfMissing } |
typedef qint64 | Id |
Public Member Functions | |
void | addAttribute (Attribute *attribute) |
Attribute * | attribute (const QByteArray &name) const |
template<typename T > | |
T * | attribute (CreateOption option) |
template<typename T > | |
T * | attribute () const |
Attribute::List | attributes () const |
void | clearAttributes () |
bool | hasAttribute (const QByteArray &name) const |
template<typename T > | |
bool | hasAttribute () const |
Id | id () const |
bool | isValid () const |
bool | operator!= (const Entity &other) const |
bool | operator< (const Entity &other) const |
Entity & | operator= (const Entity &other) |
bool | operator== (const Entity &other) const |
Collection | parentCollection () const |
Collection & | parentCollection () |
QString | remoteId () const |
QString | remoteRevision () const |
void | removeAttribute (const QByteArray &name) |
template<typename T > | |
void | removeAttribute () |
void | setId (Id identifier) |
void | setParentCollection (const Collection &parent) |
void | setRemoteId (const QString &id) |
void | setRemoteRevision (const QString &revision) |
Protected Member Functions | |
Entity (const Entity &other) | |
~Entity () | |
Detailed Description
The base class for Item and Collection.
Entity is the common base class for Item and Collection that provides unique IDs and attributes handling.
This class is not meant to be used directly, use Item or Collection instead.
Member Typedef Documentation
typedef qint64 Akonadi::Entity::Id |
Member Enumeration Documentation
Constructor & Destructor Documentation
|
protected |
Creates an entity from an other
entity.
Definition at line 53 of file entity.cpp.
|
protected |
Destroys the entity.
Definition at line 63 of file entity.cpp.
Member Function Documentation
void Entity::addAttribute | ( | Attribute * | attribute | ) |
Adds an attribute to the entity.
If an attribute of the same type name already exists, it is deleted and replaced with the new one.
- Parameters
-
attribute The new attribute.
- Note
- The entity takes the ownership of the attribute.
Definition at line 127 of file entity.cpp.
Attribute * Entity::attribute | ( | const QByteArray & | name | ) | const |
Returns the attribute of the given type name
if available, 0 otherwise.
Definition at line 167 of file entity.cpp.
|
inline |
|
inline |
Attribute::List Entity::attributes | ( | ) | const |
Returns a list of all attributes of the entity.
Definition at line 153 of file entity.cpp.
void Akonadi::Entity::clearAttributes | ( | ) |
Removes and deletes all attributes of the entity.
Definition at line 158 of file entity.cpp.
bool Entity::hasAttribute | ( | const QByteArray & | name | ) | const |
Returns true
if the entity has an attribute of the given type name
, false otherwise.
Definition at line 148 of file entity.cpp.
|
inline |
Entity::Id Entity::id | ( | ) | const |
Returns the unique identifier of the entity.
Definition at line 72 of file entity.cpp.
bool Entity::isValid | ( | ) | const |
Returns whether the entity is valid.
Definition at line 97 of file entity.cpp.
bool Akonadi::Entity::operator!= | ( | const Entity & | other | ) | const |
Returns whether the entity's id does not equal the id of the other
entity.
Definition at line 108 of file entity.cpp.
bool Akonadi::Entity::operator< | ( | const Entity & | other | ) | const |
Assigns the other
to this entity and returns a reference to this entity.
- Parameters
-
other the entity to assign
Definition at line 113 of file entity.cpp.
bool Entity::operator== | ( | const Entity & | other | ) | const |
Returns whether the entity's id equals the id of the other
entity.
Definition at line 102 of file entity.cpp.
Collection Entity::parentCollection | ( | ) | const |
Returns the parent collection of this object.
- Note
- This will of course only return a useful value if it was explictly retrieved from the Akonadi server.
- Since
- 4.4
Definition at line 185 of file entity.cpp.
Collection & Entity::parentCollection | ( | ) |
Returns a reference to the parent collection of this object.
- Note
- This will of course only return a useful value if it was explictly retrieved from the Akonadi server.
- Since
- 4.4
Definition at line 177 of file entity.cpp.
QString Entity::remoteId | ( | ) | const |
Returns the remote id of the entity.
Definition at line 82 of file entity.cpp.
QString Entity::remoteRevision | ( | ) | const |
Returns the remote revision of the entity.
- Note
- This method is supposed to be used by resources only.
- Since
- 4.5
Definition at line 92 of file entity.cpp.
void Entity::removeAttribute | ( | const QByteArray & | name | ) |
Removes and deletes the attribute of the given type name
.
Definition at line 142 of file entity.cpp.
|
inline |
void Entity::setId | ( | Id | identifier | ) |
Sets the unique identifier
of the entity.
Definition at line 67 of file entity.cpp.
void Entity::setParentCollection | ( | const Collection & | parent | ) |
Set the parent collection of this object.
- Note
- Calling this method has no immediate effect for the object itself, such as being moved to another collection. It is mainly relevant to provide a context for RID-based operations inside resources.
- Parameters
-
parent The parent collection.
- Since
- 4.4
Definition at line 194 of file entity.cpp.
void Entity::setRemoteId | ( | const QString & | id | ) |
Sets the remote id
of the entity.
Definition at line 77 of file entity.cpp.
void Entity::setRemoteRevision | ( | const QString & | revision | ) |
Sets the remote revision
of the entity.
- Parameters
-
revision the entity's remote revision The remote revision can be used by resources to store some revision information of the backend to detect changes there.
- Note
- This method is supposed to be used by resources only.
- Since
- 4.5
Definition at line 87 of file entity.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.