akonadi
#include <itiphandlerhelper_p.h>
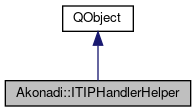
Public Types | |
enum | Action { ActionAsk, ActionSendMessage, ActionDontSendMessage } |
enum | SendResult { ResultCanceled, ResultFailKeepUpdate, ResultFailAbortUpdate, ResultNoSendingNeeded, ResultError, ResultSuccess } |
Signals | |
void | finished (Akonadi::ITIPHandlerHelper::SendResult result, const QString &errorMessage) |
Public Member Functions | |
ITIPHandlerHelper (QWidget *parent=0) | |
void | calendarJobFinished (bool success, const QString &errorString) |
bool | handleIncidenceAboutToBeModified (const KCalCore::Incidence::Ptr &incidence) |
ITIPHandlerHelper::SendResult | sendCounterProposal (const KCalCore::Incidence::Ptr &oldIncidence, const KCalCore::Incidence::Ptr &newIncidence) |
ITIPHandlerHelper::SendResult | sendIncidenceCreatedMessage (KCalCore::iTIPMethod method, const KCalCore::Incidence::Ptr &incidence) |
ITIPHandlerHelper::SendResult | sendIncidenceDeletedMessage (KCalCore::iTIPMethod method, const KCalCore::Incidence::Ptr &incidence) |
ITIPHandlerHelper::SendResult | sendIncidenceModifiedMessage (KCalCore::iTIPMethod method, const KCalCore::Incidence::Ptr &incidence, bool attendeeStatusChanged) |
void | setDefaultAction (Action action) |
void | setDialogParent (QWidget *parent) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This class handles sending of invitations to attendees when Incidences (e.g.
events or todos) are created/modified/deleted.
There are two scenarios: o "we" are the organizer, where "we" means any of the identities or mail addresses known to Kontact/PIM. If there are attendees, we need to mail them all, even if one or more of them are also "us". Otherwise there would be no way to invite a resource or our boss, other identities we also manage. o "we: are not the organizer, which means we changed the completion status of a todo, or we changed our attendee status from, say, tentative to accepted. In both cases we only mail the organizer. All other changes bring us out of sync with the organizer, so we won't mail, if the user insists on applying them.
NOTE: Currently only events and todos are support, meaning Incidence::type() should either return "Event" or "Todo"
Definition at line 66 of file itiphandlerhelper_p.h.
Member Enumeration Documentation
Definition at line 73 of file itiphandlerhelper_p.h.
Member Function Documentation
bool Akonadi::ITIPHandlerHelper::handleIncidenceAboutToBeModified | ( | const KCalCore::Incidence::Ptr & | incidence | ) |
Checks if the incidence should really be modified.
If the user is not the organizer of this incidence, he will be asked if he really wants to proceed.
Only create the ItemModifyJob if this method returns true.
- Parameters
-
incidence The modified incidence. It may not be null.
ITIPHandlerHelper::SendResult Akonadi::ITIPHandlerHelper::sendCounterProposal | ( | const KCalCore::Incidence::Ptr & | oldIncidence, |
const KCalCore::Incidence::Ptr & | newIncidence | ||
) |
Send counter proposal message.
- Parameters
-
oldIncidence The original event provided in the invitations. newIncidence The new event as edited by the user.
ITIPHandlerHelper::SendResult Akonadi::ITIPHandlerHelper::sendIncidenceCreatedMessage | ( | KCalCore::iTIPMethod | method, |
const KCalCore::Incidence::Ptr & | incidence | ||
) |
Handles sending of invitations for newly created incidences.
This method asserts that we (as in any of the identities or mail addresses known to Kontact/PIM) are the organizer.
- Parameters
-
incidence The new incidence.
ITIPHandlerHelper::SendResult Akonadi::ITIPHandlerHelper::sendIncidenceDeletedMessage | ( | KCalCore::iTIPMethod | method, |
const KCalCore::Incidence::Ptr & | incidence | ||
) |
Handles sending of ivitations for deleted incidences.
- Parameters
-
incidence The deleted incidence.
ITIPHandlerHelper::SendResult Akonadi::ITIPHandlerHelper::sendIncidenceModifiedMessage | ( | KCalCore::iTIPMethod | method, |
const KCalCore::Incidence::Ptr & | incidence, | ||
bool | attendeeStatusChanged | ||
) |
Handles sending of invitations for modified incidences.
- Parameters
-
incidence The modified incidence. attendeeStatusChanged if true
andmethod
is #iTIPRequest ask the user whether to send a status update as well
void Akonadi::ITIPHandlerHelper::setDefaultAction | ( | Action | action | ) |
When an Incidence is created/modified/deleted the user can choose to send an ICal message to the other participants.
By default the user will be asked if he wants to send a message to other participants. In some cases it is preferably though to not bother the user with this question. This method allows to change the default behavior. This method applies to the sendIncidence*Message() methods.
- Parameters
-
action the action to set as default
void Akonadi::ITIPHandlerHelper::setDialogParent | ( | QWidget * | parent | ) |
Before an invitation is sent the user is asked for confirmation by means of an dialog.
- Parameters
-
parent The parent widget used for the dialogs.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:05 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.