akonadi
#include <itemsearchjob.h>
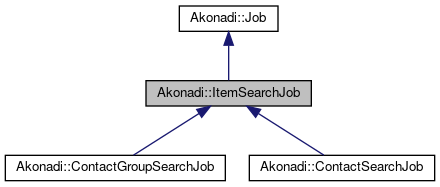
Signals | |
void | itemsReceived (const Akonadi::Item::List &items) |
![]() | |
void | aboutToStart (Akonadi::Job *job) |
void | writeFinished (Akonadi::Job *job) |
Public Member Functions | |
AKONADI_DEPRECATED | ItemSearchJob (const QString &query, QObject *parent=0) |
ItemSearchJob (const SearchQuery &query, QObject *parent=0) | |
~ItemSearchJob () | |
ItemFetchScope & | fetchScope () |
bool | isRecursive () const |
bool | isRemoteSearchEnabled () const |
Item::List | items () const |
QStringList | mimeTypes () const |
Collection::List | searchCollections () const |
void | setFetchScope (const ItemFetchScope &fetchScope) |
void | setMimeTypes (const QStringList &mimeTypes) |
void AKONADI_DEPRECATED | setQuery (const QString &query) |
void | setQuery (const SearchQuery &query) |
void | setRecursive (bool recursive) |
void | setRemoteSearchEnabled (bool enabled) |
void | setSearchCollections (const Collection::List &collections) |
![]() | |
Job (QObject *parent=0) | |
virtual | ~Job () |
virtual QString | errorString () const |
void | start () |
Static Public Member Functions | |
static AKONADI_DEPRECATED QUrl | akonadiItemIdUri () |
Protected Member Functions | |
virtual void | doHandleResponse (const QByteArray &tag, const QByteArray &data) |
void | doStart () |
![]() | |
virtual bool | addSubjob (KJob *job) |
virtual bool | doKill () |
void | emitWriteFinished () |
virtual bool | removeSubjob (KJob *job) |
Additional Inherited Members | |
![]() | |
enum | Error { ConnectionFailed = UserDefinedError, ProtocolVersionMismatch, UserCanceled, Unknown, UserError = UserDefinedError + 42 } |
typedef QList< Job * > | List |
![]() | |
virtual void | slotResult (KJob *job) |
Detailed Description
Job that searches for items in the Akonadi storage.
This job searches for items that match a given search query and returns the list of matching item.
- Since
- 4.4
Definition at line 67 of file itemsearchjob.h.
Constructor & Destructor Documentation
Creates an item search job.
- Parameters
-
query The search query in raw Akonadi search metalanguage format (JSON) parent The parent object.
- Deprecated:
- Deprecated as of 4.13. Use SearchQuery instead.
Definition at line 126 of file itemsearchjob.cpp.
|
explicit |
Creates an item search job.
- Parameters
-
query The search query. parent The parent object.
- Since
- 4.13
Definition at line 118 of file itemsearchjob.cpp.
ItemSearchJob::~ItemSearchJob | ( | ) |
Destroys the item search job.
Definition at line 134 of file itemsearchjob.cpp.
Member Function Documentation
|
static |
Returns an URI that represents a predicate that is always added to the Nepomuk resource by the Akonadi Nepomuk feeders.
The statement containing this predicate has the Akonadi Item ID of the resource as string as the object, and the Nepomuk resource, e.g. a PersonContact, as the subject.
Always limit your searches to statements that contain this URI as predicate.
- Since
- 4.4.3
- Deprecated:
- Deprecated as of 4.13, where SPARQL queries were replaced by Baloo
Definition at line 278 of file itemsearchjob.cpp.
|
protectedvirtual |
This method should be reimplemented in the concrete jobs in case you want to handle incoming data.
It will be called on received data from the backend. The default implementation does nothing.
- Parameters
-
tag The tag of the corresponding command, empty if this is an untagged response. data The received data.
Reimplemented from Akonadi::Job.
Definition at line 242 of file itemsearchjob.cpp.
|
protectedvirtual |
This method must be reimplemented in the concrete jobs.
It will be called after the job has been started and a connection to the Akonadi backend has been established.
Implements Akonadi::Job.
Definition at line 214 of file itemsearchjob.cpp.
ItemFetchScope & ItemSearchJob::fetchScope | ( | ) |
Returns the item fetch scope.
Since this returns a reference it can be used to conveniently modify the current scope in-place, i.e. by calling a method on the returned reference without storing it in a local variable. See the ItemFetchScope documentation for an example.
- Returns
- a reference to the current item fetch scope
- See also
- setFetchScope() for replacing the current item fetch scope
Definition at line 159 of file itemsearchjob.cpp.
bool ItemSearchJob::isRecursive | ( | ) | const |
Returns whether the search is recursive.
- Since
- 4.13
Definition at line 197 of file itemsearchjob.cpp.
bool ItemSearchJob::isRemoteSearchEnabled | ( | ) | const |
Returns whether remote search is enabled.
- Since
- 4.13
Definition at line 209 of file itemsearchjob.cpp.
Item::List ItemSearchJob::items | ( | ) | const |
Returns the items that matched the search query.
Definition at line 271 of file itemsearchjob.cpp.
|
signal |
This signal is emitted whenever new matching items have been fetched completely.
- Note
- This is an optimization, instead of waiting for the end of the job and calling items(), you can connect to this signal and get the items incrementally.
- Parameters
-
items The matching items.
QStringList ItemSearchJob::mimeTypes | ( | ) | const |
Returns list of mime types to search in.
- Since
- 4.13
Definition at line 185 of file itemsearchjob.cpp.
Collection::List ItemSearchJob::searchCollections | ( | ) | const |
Returns list of collections to search.
This list does not include child collections that will be searched when recursive search is enabled
- Since
- 4.13
Definition at line 173 of file itemsearchjob.cpp.
void ItemSearchJob::setFetchScope | ( | const ItemFetchScope & | fetchScope | ) |
Sets the item fetch scope.
The ItemFetchScope controls how much of an matching item's data is fetched from the server, e.g. whether to fetch the full item payload or only meta data.
- Parameters
-
fetchScope The new scope for item fetch operations.
- See also
- fetchScope()
Definition at line 152 of file itemsearchjob.cpp.
void ItemSearchJob::setMimeTypes | ( | const QStringList & | mimeTypes | ) |
Search only for items of given mime types.
- Since
- 4.13
Definition at line 178 of file itemsearchjob.cpp.
void ItemSearchJob::setQuery | ( | const QString & | query | ) |
Sets the search query
in Akonadi search metalanguage format (JSON)
- Deprecated:
- Deprecated as of 4.13. Use SearchQuery instead.
Definition at line 138 of file itemsearchjob.cpp.
void ItemSearchJob::setQuery | ( | const SearchQuery & | query | ) |
void ItemSearchJob::setRecursive | ( | bool | recursive | ) |
Sets whether the search should recurse into collections.
When set to true, all child collections of the specific collections will be search recursively.
- Parameters
-
recursive Whether to search recursively
- Since
- 4.13
Definition at line 190 of file itemsearchjob.cpp.
void ItemSearchJob::setRemoteSearchEnabled | ( | bool | enabled | ) |
Sets whether resources should be queried too.
When set to true, Akonadi will search local indexed items and will also query resources that support server-side search, to forward the query to remote storage (for example using SEARCH feature on IMAP servers) and merge their results with results from local index.
This is useful especially when searching resources, that don't fetch full payload by default, for example the IMAP resource, which only fetches headers by default and the body is fetched on demand, which means that emails that were not yet fully fetched cannot be indexed in local index, and thus cannot be searched. With remote search, even those emails can be included in search results.
This feature is disabled by default.
Results are streamed back to client as they are received from queried sources, so this job can take some time to finish, but will deliver initial results from local index fairly quickly.
- Parameters
-
enabled Whether remote search is enabled
- Since
- 4.13
Definition at line 202 of file itemsearchjob.cpp.
void ItemSearchJob::setSearchCollections | ( | const Collection::List & | collections | ) |
Search only in given collections.
When recursive search is enabled, all child collections of each specified collection will be searched too
By default all collections are be searched.
- Parameters
-
collections Collections to search
- Since
- 4.13
Definition at line 166 of file itemsearchjob.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:05 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.