akonadi
#include <scheduler_p.h>
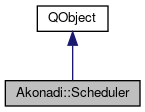
Signals | |
void | transactionFinished (Akonadi::Scheduler::Result, const QString &errorMessage) |
Public Member Functions | |
Scheduler (QObject *parent=0) | |
void | acceptTransaction (const KCalCore::IncidenceBase::Ptr &incidence, const Akonadi::CalendarBase::Ptr &calendar, KCalCore::iTIPMethod method, KCalCore::ScheduleMessage::Status status, const QString &email=QString()) |
KCalCore::FreeBusyCache * | freeBusyCache () const |
virtual QString | freeBusyDir () const =0 |
virtual void | performTransaction (const KCalCore::IncidenceBase::Ptr &incidence, KCalCore::iTIPMethod method)=0 |
virtual void | performTransaction (const KCalCore::IncidenceBase::Ptr &incidence, KCalCore::iTIPMethod method, const QString &recipients)=0 |
virtual void | publish (const KCalCore::IncidenceBase::Ptr &incidence, const QString &recipients)=0 |
void | setFreeBusyCache (KCalCore::FreeBusyCache *) |
void | setShowDialogs (bool enable) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
void | acceptAdd (const KCalCore::IncidenceBase::Ptr &, KCalCore::ScheduleMessage::Status status) |
void | acceptCancel (const KCalCore::IncidenceBase::Ptr &, const Akonadi::CalendarBase::Ptr &calendar, KCalCore::ScheduleMessage::Status status, const QString &attendee) |
void | acceptCounter (const KCalCore::IncidenceBase::Ptr &, KCalCore::ScheduleMessage::Status status) |
void | acceptDeclineCounter (const KCalCore::IncidenceBase::Ptr &, KCalCore::ScheduleMessage::Status status) |
void | acceptFreeBusy (const KCalCore::IncidenceBase::Ptr &, KCalCore::iTIPMethod method) |
void | acceptPublish (const KCalCore::IncidenceBase::Ptr &, const Akonadi::CalendarBase::Ptr &calendar, KCalCore::ScheduleMessage::Status status, KCalCore::iTIPMethod method) |
void | acceptRefresh (const KCalCore::IncidenceBase::Ptr &, KCalCore::ScheduleMessage::Status status) |
void | acceptReply (const KCalCore::IncidenceBase::Ptr &, const Akonadi::CalendarBase::Ptr &calendar, KCalCore::ScheduleMessage::Status status, KCalCore::iTIPMethod method) |
void | acceptRequest (const KCalCore::IncidenceBase::Ptr &, const Akonadi::CalendarBase::Ptr &calendar, KCalCore::ScheduleMessage::Status status, const QString &email) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
KCalCore::ICalFormat * | mFormat |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This class provides an encapsulation of iTIP transactions (RFC 2446).
It is an abstract base class for inheritance by implementations of the iTIP scheme like iMIP or iRIP.
Definition at line 43 of file scheduler_p.h.
Constructor & Destructor Documentation
|
explicit |
Creates a scheduler for calendar specified as argument.
Member Function Documentation
void Akonadi::Scheduler::acceptTransaction | ( | const KCalCore::IncidenceBase::Ptr & | incidence, |
const Akonadi::CalendarBase::Ptr & | calendar, | ||
KCalCore::iTIPMethod | method, | ||
KCalCore::ScheduleMessage::Status | status, | ||
const QString & | email = QString() |
||
) |
Accepts the transaction.
The incidence argument specifies the iCal component on which the transaction acts. The status is the result of processing a iTIP message with the current calendar and specifies the action to be taken for this incidence.
- Parameters
-
incidence the incidence for the transaction. Must be valid. calendar a loaded calendar. method iTIP transaction method to check. status scheduling status. email the email address of the person for whom this transaction is to be performed.
Listen to the acceptTransactionFinished() signal to know the success.
KCalCore::FreeBusyCache* Akonadi::Scheduler::freeBusyCache | ( | ) | const |
Returns the free/busy cache.
|
pure virtual |
Returns the directory where the free-busy information is stored.
|
pure virtual |
Performs iTIP transaction on incidence.
The method is specified as the method argument and can be any valid iTIP method.
- Parameters
-
incidence the incidence for the transaction. Must be valid. method the iTIP transaction method to use.
|
pure virtual |
Performs iTIP transaction on incidence to specified recipient(s).
The method is specified as the method argumanet and can be any valid iTIP method.
- Parameters
-
incidence the incidence for the transaction. Must be valid. method the iTIP transaction method to use. recipients the receipients of the transaction.
|
pure virtual |
Notify recipients
about incidence
.
- Parameters
-
incidence the incidence to send recipients the people to send it to
void Akonadi::Scheduler::setFreeBusyCache | ( | KCalCore::FreeBusyCache * | ) |
Sets the free/busy cache used to store free/busy information.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:05 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.