akonadi
#include <standardactionmanager.h>
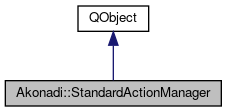
Signals | |
void | actionStateUpdated () |
Public Member Functions | |
StandardActionManager (KActionCollection *actionCollection, QWidget *parent=0) | |
~StandardActionManager () | |
KAction * | action (Type type) const |
KAction * | createAction (Type type) |
void | createActionFolderMenu (QMenu *menu, Type type) |
void | createAllActions () |
void | interceptAction (Type type, bool intercept=true) |
Akonadi::Collection::List | selectedCollections () const |
Akonadi::Item::List | selectedItems () const |
void | setActionText (Type type, const KLocalizedString &text) |
void | setCapabilityFilter (const QStringList &capabilities) |
void | setCollectionPropertiesPageNames (const QStringList &names) |
void | setCollectionSelectionModel (QItemSelectionModel *selectionModel) |
void | setContextText (Type type, TextContext context, const QString &text) |
void | setContextText (Type type, TextContext context, const KLocalizedString &text) |
void | setFavoriteCollectionsModel (FavoriteCollectionsModel *favoritesModel) |
void | setFavoriteSelectionModel (QItemSelectionModel *selectionModel) |
void | setItemSelectionModel (QItemSelectionModel *selectionModel) |
void | setMimeTypeFilter (const QStringList &mimeTypes) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Manages generic actions for collection and item views.
Manages generic Akonadi actions common for all types. This covers creating of the actions with appropriate labels, icons, shortcuts etc., updating the action state depending on the current selection as well as default implementations for the actual operations.
If the default implementation is not appropriate for your application you can still use the state tracking by disconnecting the triggered() signal and re-connecting it to your implementation. The actual KAction objects can be retrieved by calling createAction() or action() for that.
If the default look and feel (labels, icons, shortcuts) of the actions is not appropriate for your application, you can access them as noted above and customize them to your needs. Additionally, you can set a KLocalizedString which should be used as a action label with correct plural handling for actions operating on multiple objects with setActionText().
Finally, if you have special needs for the action states, connect to the actionStateUpdated() signal and adjust the state accordingly.
The following actions are provided (KAction name in parenthesis):
- Creation of a new collection (
akonadi_collection_create
) - Copying of selected collections (
akonadi_collection_copy
) - Deletion of selected collections (
akonadi_collection_delete
) - Synchronization of selected collections (
akonadi_collection_sync
) - Showing the collection properties dialog for the current collection (
akonadi_collection_properties
) - Copying of selected items (
akonadi_itemcopy
) - Pasting collections, items or raw data (
akonadi_paste
) - Deleting of selected items (
akonadi_item_delete
) - Managing local subscriptions (
akonadi_manage_local_subscriptions
)
The following example shows how to use standard actions in your application:
Additionally you have to add the actions to the KXMLGUI file of your application, using the names listed above.
If you only need a subset of the actions provided, you can call createAction() instead of createAllActions() for the action types you want.
If you want to use your own implementation of the actual action operation and not the default implementation, you can call interceptAction() on the action type you want to handle yourself and connect the slot with your own implementation to the triggered() signal of the action:
- Todo:
- collection deleting and sync do not support multi-selection yet
Definition at line 126 of file standardactionmanager.h.
Member Enumeration Documentation
Describes the text context that can be customized.
Definition at line 177 of file standardactionmanager.h.
Describes the supported actions.
Enumerator | |
---|---|
CreateCollection |
Creates an collection. |
CopyCollections |
Copies the selected collections. |
DeleteCollections |
Deletes the selected collections. |
SynchronizeCollections |
Synchronizes collections. |
CollectionProperties |
Provides collection properties. |
CopyItems |
Copies the selected items. |
Paste |
Paste collections or items. |
DeleteItems |
Deletes the selected items. |
ManageLocalSubscriptions |
Manages local subscriptions. |
AddToFavoriteCollections |
Add the collection to the favorite collections model.
|
RemoveFromFavoriteCollections |
Remove the collection from the favorite collections model.
|
RenameFavoriteCollection |
Rename the collection of the favorite collections model.
|
CopyCollectionToMenu |
Menu allowing to quickly copy a collection into another collection.
|
CopyItemToMenu |
Menu allowing to quickly copy an item into a collection.
|
MoveItemToMenu |
Menu allowing to move item into a collection.
|
MoveCollectionToMenu |
Menu allowing to move a collection into another collection.
|
CutItems |
Cuts the selected items.
|
CutCollections |
Cuts the selected collections.
|
CreateResource |
Creates a new resource.
|
DeleteResources |
Deletes the selected resources.
|
ResourceProperties |
Provides the resource properties.
|
SynchronizeResources |
Synchronizes the selected resources.
|
ToggleWorkOffline |
Toggles the work offline state of all resources.
|
CopyCollectionToDialog |
Copy a collection into another collection, select the target in a dialog.
|
MoveCollectionToDialog |
Move a collection into another collection, select the target in a dialog.
|
CopyItemToDialog |
Copy an item into a collection, select the target in a dialog.
|
MoveItemToDialog |
Move an item into a collection, select the target in a dialog.
|
SynchronizeCollectionsRecursive |
Synchronizes collections in a recursive way.
|
MoveCollectionsToTrash |
Moves the selected collection to trash and marks it as deleted, needs EntityDeletedAttribute.
|
MoveItemsToTrash |
Moves the selected items to trash and marks them as deleted, needs EntityDeletedAttribute.
|
RestoreCollectionsFromTrash |
Restores the selected collection from trash, needs EntityDeletedAttribute.
|
RestoreItemsFromTrash |
Restores the selected items from trash, needs EntityDeletedAttribute.
|
MoveToTrashRestoreCollection |
Move Collection to Trash or Restore it from Trash, needs EntityDeletedAttribute.
|
MoveToTrashRestoreCollectionAlternative |
Helper type for MoveToTrashRestoreCollection, do not create directly. Use this to override texts of the restore action.
|
MoveToTrashRestoreItem |
Move Item to Trash or Restore it from Trash, needs EntityDeletedAttribute.
|
MoveToTrashRestoreItemAlternative |
Helper type for MoveToTrashRestoreItem, do not create directly. Use this to override texts of the restore action.
|
SynchronizeFavoriteCollections |
Synchronize favorite collections.
|
LastType |
Marks last action. |
Definition at line 133 of file standardactionmanager.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new standard action manager.
- Parameters
-
actionCollection The action collection to operate on. parent The parent widget.
Akonadi::StandardActionManager::~StandardActionManager | ( | ) |
Destroys the standard action manager.
Member Function Documentation
KAction* Akonadi::StandardActionManager::action | ( | Type | type | ) | const |
Returns the action of the given type, 0 if it has not been created (yet).
- Parameters
-
type action type
|
signal |
This signal is emitted whenever the action state has been updated.
In case you have special needs for changing the state of some actions, connect to this signal and adjust the action state.
KAction* Akonadi::StandardActionManager::createAction | ( | Type | type | ) |
Creates the action of the given type and adds it to the action collection specified in the constructor if it does not exist yet.
The action is connected to its default implementation provided by this class.
- Parameters
-
type action to be created
Create a popup menu.
- Parameters
-
menu parent menu for a popup type action type
- Since
- 4.8
void Akonadi::StandardActionManager::createAllActions | ( | ) |
Convenience method to create all standard actions.
- See also
- createAction()
void Akonadi::StandardActionManager::interceptAction | ( | Type | type, |
bool | intercept = true |
||
) |
Sets whether the default implementation for the given action type
shall be executed when the action is triggered.
- Parameters
-
type action type intercept If false
, the default implementation will be executed, iftrue
no action is taken.
- Since
- 4.6
Akonadi::Collection::List Akonadi::StandardActionManager::selectedCollections | ( | ) | const |
Returns the list of collections that are currently selected.
The list is empty if no collection is currently selected.
- Since
- 4.6
Akonadi::Item::List Akonadi::StandardActionManager::selectedItems | ( | ) | const |
Returns the list of items that are currently selected.
The list is empty if no item is currently selected.
- Since
- 4.6
void Akonadi::StandardActionManager::setActionText | ( | Type | type, |
const KLocalizedString & | text | ||
) |
Sets the label of the action type
to text
, which is used during updating the action state and substituted according to the number of selected objects.
This is mainly useful to customize the label of actions that can operate on multiple objects.
- Parameters
-
type the action to set a text for text the text to display for the given action Example: acctMgr->setActionText( Akonadi::StandardActionManager::CopyItems,ki18np( "Copy Mail", "Copy %1 Mails" ) );
void Akonadi::StandardActionManager::setCapabilityFilter | ( | const QStringList & | capabilities | ) |
Sets the capability filter that will be used when creating new resources.
- Parameters
-
capabilities filter for creating new resources
- Since
- 4.6
void Akonadi::StandardActionManager::setCollectionPropertiesPageNames | ( | const QStringList & | names | ) |
Sets the page names
of the config pages that will be used by the built-in collection properties dialog.
- Parameters
-
names list of names which will be used
- Since
- 4.6
void Akonadi::StandardActionManager::setCollectionSelectionModel | ( | QItemSelectionModel * | selectionModel | ) |
Sets the collection selection model based on which the collection related actions should operate.
If none is set, all collection actions will be disabled.
- Parameters
-
selectionModel model to be set for collection
void Akonadi::StandardActionManager::setContextText | ( | Type | type, |
TextContext | context, | ||
const QString & | text | ||
) |
Sets the text
of the action type
for the given context
.
- Parameters
-
type action type context context for action text content to set for the action
- Since
- 4.6
void Akonadi::StandardActionManager::setContextText | ( | Type | type, |
TextContext | context, | ||
const KLocalizedString & | text | ||
) |
Sets the text
of the action type
for the given context
.
- Parameters
-
type action type context context for action text content to set for the action
- Since
- 4.6
void Akonadi::StandardActionManager::setFavoriteCollectionsModel | ( | FavoriteCollectionsModel * | favoritesModel | ) |
Sets the favorite collections model based on which the collection relatedactions should operate.
If none is set, the "Add to Favorite Folders" action will be disabled.
- Parameters
-
favoritesModel model for the user's favorite collections
- Since
- 4.4
void Akonadi::StandardActionManager::setFavoriteSelectionModel | ( | QItemSelectionModel * | selectionModel | ) |
Sets the favorite collection selection model based on which the favorite collection related actions should operate.
If none is set, all favorite modifications actions will be disabled.
- Parameters
-
selectionModel selection model for favorite collections
- Since
- 4.4
void Akonadi::StandardActionManager::setItemSelectionModel | ( | QItemSelectionModel * | selectionModel | ) |
Sets the item selection model based on which the item related actions should operate.
If none is set, all item actions will be disabled.
- Parameters
-
selectionModel selection model for items
void Akonadi::StandardActionManager::setMimeTypeFilter | ( | const QStringList & | mimeTypes | ) |
Sets the mime type filter that will be used when creating new resources.
- Parameters
-
mimeTypes filter for creating new resources
- Since
- 4.6
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:05 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.