kabc
#include <addressbook.h>
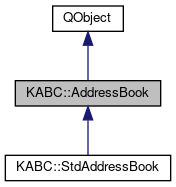
Classes | |
class | ConstIterator |
class | Iterator |
Signals | |
void | addressBookChanged (AddressBook *addressBook) |
void | addressBookLocked (AddressBook *addressBook) |
void | addressBookUnlocked (AddressBook *addressBook) |
void | loadingFinished (Resource *resource) |
void | savingFinished (Resource *resource) |
Public Member Functions | |
AddressBook () | |
AddressBook (const QString &config) | |
virtual | ~AddressBook () |
bool | addCustomField (const QString &label, int category=Field::All, const QString &key=QString(), const QString &app=QString()) const |
bool | addResource (Resource *resource) |
Addressee::List | allAddressees () const |
QStringList | allDistributionListNames () const |
QList< DistributionList * > | allDistributionLists () |
bool | asyncLoad () |
bool | asyncSave (Ticket *ticket) |
ConstIterator | begin () const |
Iterator | begin () |
void | clear () |
ConstIterator | constBegin () const |
ConstIterator | constEnd () const |
DistributionList * | createDistributionList (const QString &name, Resource *resource=0) |
void | dump () const |
void | emitAddressBookChanged () |
void | emitAddressBookLocked () |
void | emitAddressBookUnlocked () |
ConstIterator | end () const |
Iterator | end () |
void | error (const QString &msg) |
Field::List | fields (int category=Field::All) const |
Iterator | find (const Addressee &addr) |
ConstIterator | find (const Addressee &addr) const |
Addressee::List | findByCategory (const QString &category) const |
Addressee::List | findByEmail (const QString &email) const |
Addressee::List | findByName (const QString &name) const |
Addressee | findByUid (const QString &uid) const |
DistributionList * | findDistributionListByIdentifier (const QString &identifier) |
DistributionList * | findDistributionListByName (const QString &name, Qt::CaseSensitivity caseSensitivity=Qt::CaseSensitive) |
virtual QString | identifier () const |
void | insertAddressee (const Addressee &addr) |
bool | load () |
bool | loadingHasFinished () const |
void | releaseSaveTicket (Ticket *ticket) |
void | removeAddressee (const Addressee &addr) |
void | removeAddressee (const Iterator &it) |
void | removeDistributionList (DistributionList *list) |
bool | removeResource (Resource *resource) |
Ticket * | requestSaveTicket (Resource *resource=0) |
QList< Resource * > | resources () const |
bool | save (Ticket *ticket) |
void | setErrorHandler (ErrorHandler *errorHandler) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Slots | |
void | resourceLoadingError (Resource *resource, const QString &errMsg) |
void | resourceLoadingFinished (Resource *resource) |
void | resourceSavingError (Resource *resource, const QString &errMsg) |
void | resourceSavingFinished (Resource *resource) |
Protected Member Functions | |
KRES::Manager< Resource > * | resourceManager () |
void | setStandardResource (Resource *resource) |
Resource * | standardResource () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Friends | |
QDataStream & | operator<< (QDataStream &lhs, const AddressBook &rhs) |
QDataStream & | operator>> (QDataStream &lhs, AddressBook &rhs) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Address Book.
This class provides access to a collection of address book entries.
Definition at line 46 of file addressbook.h.
Constructor & Destructor Documentation
AddressBook::AddressBook | ( | ) |
Constructs an address book object.
You have to add the resources manually before calling load().
Definition at line 307 of file addressbook.cpp.
AddressBook::AddressBook | ( | const QString & | config | ) |
Constructs an address book object.
The resources are loaded automatically.
- Parameters
-
config The config file which contains the resource settings.
Definition at line 319 of file addressbook.cpp.
|
virtual |
Destructor.
Definition at line 336 of file addressbook.cpp.
Member Function Documentation
bool AddressBook::addCustomField | ( | const QString & | label, |
int | category = Field::All , |
||
const QString & | key = QString() , |
||
const QString & | app = QString() |
||
) | const |
Add custom field to address book.
- Parameters
-
label User visible label of the field. category Ored list of field categories. key Identifier used as key for reading and writing the field. app String used as application key for reading and writing the field.
Definition at line 790 of file addressbook.cpp.
bool AddressBook::addResource | ( | Resource * | resource | ) |
Adds a resource to the address book.
- Parameters
-
resource The resource you want to add.
- Returns
- Whether opening the resource was successfully.
Definition at line 831 of file addressbook.cpp.
|
signal |
Emitted when one of the resources discovered a change in its backend or the asynchronous loading of all resources has finished.
You should connect to this signal to update the presentation of the contact data in your application.
- Parameters
-
addressBook The address book which emitted this signal.
|
signal |
Emitted when one of the resources has been locked for writing.
- Parameters
-
addressBook The address book which emitted this signal.
|
signal |
Emitted when one of the resources has been unlocked.
You should connect to this signal if you want to save your changes to a resource which is currently locked, and want to get notified when saving is possible again.
- Parameters
-
addressBook The address book which emitted this signal.
Addressee::List AddressBook::allAddressees | ( | ) | const |
Returns a list of all addressees in the address book.
Definition at line 626 of file addressbook.cpp.
QStringList AddressBook::allDistributionListNames | ( | ) | const |
Returns a list of names of all distribution lists of all resources of this address book.
Convenience function, equal to iterate over the list returned by allDistributionLists()
Definition at line 731 of file addressbook.cpp.
QList< DistributionList * > AddressBook::allDistributionLists | ( | ) |
Returns a list of all distribution lists of all resources of this address book.
Definition at line 719 of file addressbook.cpp.
bool AddressBook::asyncLoad | ( | ) |
Loads all addressees asynchronously.
This function returns immediately and emits the addressBookChanged() signal as soon as the loading has finished.
- Returns
- Whether the synchronous part of loading was successfully.
Definition at line 365 of file addressbook.cpp.
bool AddressBook::asyncSave | ( | Ticket * | ticket | ) |
Saves all addressees of one resource asynchronously.
If the save is successful the ticket is deleted.
- Parameters
-
ticket The ticket returned by requestSaveTicket().
- Returns
- Whether the synchronous part of saving was successfully.
Definition at line 399 of file addressbook.cpp.
AddressBook::ConstIterator AddressBook::begin | ( | ) | const |
Returns an iterator pointing to the first addressee of address book.
This iterator equals end() if the address book is empty.
Definition at line 446 of file addressbook.cpp.
AddressBook::Iterator AddressBook::begin | ( | ) |
This is an overloaded member function, provided for convenience.
It behaves essentially like the above function.
Definition at line 415 of file addressbook.cpp.
void AddressBook::clear | ( | ) |
Removes all addressees from the address book.
Definition at line 505 of file addressbook.cpp.
DistributionList * AddressBook::createDistributionList | ( | const QString & | name, |
Resource * | resource = 0 |
||
) |
Creates a distribution list with a given name
storing it in a given resource
.
- Note
- The newly created list will be added to the addressbook automatically on creation.
- Parameters
-
name The localized name of the new distribution list. resource The resource which should save the list. If 0
(default) the addressbook's standard resource will be used.
- See also
- standardResource()
Definition at line 674 of file addressbook.cpp.
void AddressBook::dump | ( | ) | const |
Used for debug output.
This function prints out the list of all addressees to kDebug(5700).
Definition at line 743 of file addressbook.cpp.
|
inline |
Emits the signal addressBookChanged() using this
as the parameter.
Definition at line 597 of file addressbook.h.
|
inline |
Emits the signal addressBookLocked() using this
as the parameter.
Definition at line 589 of file addressbook.h.
|
inline |
Emits the signal addressBookUnlocked() using this
as the parameter.
Definition at line 593 of file addressbook.h.
AddressBook::ConstIterator AddressBook::end | ( | ) | const |
Returns an iterator pointing to the last addressee of address book.
This iterator equals begin() if the address book is empty.
Definition at line 491 of file addressbook.cpp.
AddressBook::Iterator AddressBook::end | ( | ) |
This is an overloaded member function, provided for convenience.
It behaves essentially like the above function.
Definition at line 477 of file addressbook.cpp.
void AddressBook::error | ( | const QString & | msg | ) |
Shows GUI independent error messages.
- Parameters
-
msg The error message that shall be displayed.
Definition at line 901 of file addressbook.cpp.
Field::List AddressBook::fields | ( | int | category = Field::All | ) | const |
Returns a list of all Fields known to the address book which are associated with the given field category.
Definition at line 769 of file addressbook.cpp.
AddressBook::Iterator AddressBook::find | ( | const Addressee & | addr | ) |
Returns an iterator pointing to the specified addressee.
It will return end() if no addressee matched.
- Parameters
-
addr The addressee you are looking for.
Definition at line 589 of file addressbook.cpp.
AddressBook::ConstIterator AddressBook::find | ( | const Addressee & | addr | ) | const |
Returns an iterator pointing to the specified addressee.
It will return end() if no addressee matched.
- Parameters
-
addr The addressee you are looking for.
Definition at line 601 of file addressbook.cpp.
Addressee::List AddressBook::findByCategory | ( | const QString & | category | ) | const |
Searches all addressees which belongs to the specified category.
- Parameters
-
category The category you are looking for.
- Returns
- A list of all matching addressees.
Definition at line 662 of file addressbook.cpp.
Addressee::List AddressBook::findByEmail | ( | const QString & | ) | const |
Searches all addressees which match the specified email address.
- Parameters
-
email The email address you are looking for.
- Returns
- A list of all matching addressees.
Definition at line 650 of file addressbook.cpp.
Addressee::List AddressBook::findByName | ( | const QString & | name | ) | const |
Searches all addressees which match the specified name.
- Parameters
-
name The name you are looking for.
- Returns
- A list of all matching addressees.
Definition at line 638 of file addressbook.cpp.
Searches an addressee with the specified unique identifier.
- Parameters
-
uid The unique identifier you are looking for.
- Returns
- The addressee with the specified unique identifier or an empty addressee.
Definition at line 613 of file addressbook.cpp.
DistributionList * AddressBook::findDistributionListByIdentifier | ( | const QString & | identifier | ) |
Returns a distribution list for the given identifier
or 0
.
- Parameters
-
identifier The ID of the list for look for.
Definition at line 692 of file addressbook.cpp.
DistributionList * AddressBook::findDistributionListByName | ( | const QString & | name, |
Qt::CaseSensitivity | caseSensitivity = Qt::CaseSensitive |
||
) |
Returns a distribution list with the given name
or 0
.
- Parameters
-
name The localized name of the list for look for. caseSensitivity Whether to do string matching case sensitive or case insensitive. Default is Qt::CaseSensitive
Definition at line 705 of file addressbook.cpp.
|
virtual |
Returns a string identifying this addressbook.
The identifier is created by concatenation of the resource identifiers.
Definition at line 755 of file addressbook.cpp.
void AddressBook::insertAddressee | ( | const Addressee & | addr | ) |
Insert an addressee into the address book.
If an addressee with the same unique id already exists, it is replaced by the new one, otherwise it is appended.
- Parameters
-
addr The addressee which shall be insert.
Definition at line 546 of file addressbook.cpp.
bool AddressBook::load | ( | ) |
Loads all addressees synchronously.
- Returns
- Whether the loading was successfully.
Definition at line 347 of file addressbook.cpp.
|
signal |
Emitted when the asynchronous loading of one resource has finished after calling asyncLoad().
- Parameters
-
resource The resource which emitted this signal.
bool AddressBook::loadingHasFinished | ( | ) | const |
Returns true when the loading of the addressbook has finished, otherwise false.
Definition at line 930 of file addressbook.cpp.
void AddressBook::releaseSaveTicket | ( | Ticket * | ticket | ) |
Releases the ticket requested previously with requestSaveTicket().
Call this function, if you want to release a ticket without saving.
- Parameters
-
ticket the save ticket acquired with requestSaveTicket()
Definition at line 535 of file addressbook.cpp.
void AddressBook::removeAddressee | ( | const Addressee & | addr | ) |
Removes an addressee from the address book.
- Parameters
-
addr The addressee which shall be removed.
Definition at line 575 of file addressbook.cpp.
void AddressBook::removeAddressee | ( | const Iterator & | it | ) |
This is an overloaded member function, provided for convenience.
It behaves essentially like the above function.
- Parameters
-
it An iterator pointing to the addressee which shall be removed.
Definition at line 582 of file addressbook.cpp.
void AddressBook::removeDistributionList | ( | DistributionList * | list | ) |
Removes a distribution list
from its associated resource.
- Parameters
-
list The list to remove.
Definition at line 683 of file addressbook.cpp.
bool AddressBook::removeResource | ( | Resource * | resource | ) |
Removes a resource from the address book.
- Parameters
-
resource The resource you want to remove.
- Returns
- Whether closing the resource was successfully.
Definition at line 854 of file addressbook.cpp.
Requests a ticket for saving the addressbook.
Calling this function locks the addressbook for all other processes. You need the returned ticket object for calling the save() function.
- Parameters
-
resource A pointer to the resource which shall be locked. If 0, the default resource is locked.
- Returns
- 0 if the resource is already locked or a valid save ticket otherwise.
- See also
- save()
Definition at line 513 of file addressbook.cpp.
|
protectedslot |
Handles loading errors.
Resource will be removed from the list of those pending for loading. If this has been the last one in this list, signal addressBookChanged() is emitted.
- Parameters
-
resource The resource which could not be loaded. errMsg The message describing the error. See error()
Definition at line 952 of file addressbook.cpp.
|
protectedslot |
Handles loading success.
Resource will be removed from the list of those pending for loading and signal loadingFinished() will be emitted. It this has been the last one in this list, signal addressBookChanged() is emitted as well.
- Parameters
-
resource The resource which has been saved successfully.
Definition at line 935 of file addressbook.cpp.
|
protected |
Returns the addressbook's resource manager.
Definition at line 925 of file addressbook.cpp.
Returns a list of all resources.
Definition at line 879 of file addressbook.cpp.
Handles saving errors.
Resource will be removed from the list of those pending for saving.
- Parameters
-
resource The resource which could not be saved. errMsg The message describing the error. See error()
Definition at line 963 of file addressbook.cpp.
|
protectedslot |
Handles saving success.
Resource will be removed from the list of those pending for saving.
- Parameters
-
resource The resource which has been saved successfully.
Definition at line 945 of file addressbook.cpp.
bool AddressBook::save | ( | Ticket * | ticket | ) |
Saves all addressees of one resource synchronously.
If the save is successful the ticket is deleted.
- Parameters
-
ticket The ticket returned by requestSaveTicket().
- Returns
- Whether the saving was successfully.
Definition at line 384 of file addressbook.cpp.
|
signal |
Emitted when the asynchronous saving of one resource has finished after calling asyncSave().
- Parameters
-
resource The resource which emitted this signal.
void AddressBook::setErrorHandler | ( | ErrorHandler * | errorHandler | ) |
Sets the ErrorHandler
, that is used by error() to provide GUI independent error messages.
- Parameters
-
errorHandler The error handler you want to use.
Definition at line 895 of file addressbook.cpp.
|
protected |
Sets the resource manager's standard resource.
Convenience method for resourceManager()->setStandardResource()
- Parameters
-
resource The resource to use as the standard
- See also
- standardResource()
Definition at line 915 of file addressbook.cpp.
|
protected |
Returns the addressbook resource manager's standard resource.
Convenience method for resourceManager()->standardResource()
- See also
- setStandardResource()
Definition at line 920 of file addressbook.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:39 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.