kabc
#include <addresseelist.h>
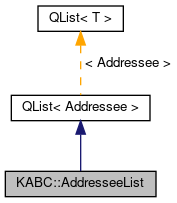
Public Member Functions | |
AddresseeList () | |
AddresseeList (const AddresseeList &) | |
AddresseeList (const QList< Addressee > &) | |
~AddresseeList () | |
AddresseeList & | operator= (const AddresseeList &other) |
bool | reverseSorting () const |
void | setReverseSorting (bool reverseSorting=true) |
void | sort () |
void | sortBy (SortingCriterion c) |
void | sortByField (Field *field=0) |
void | sortByMode (SortMode *mode=0) |
template<class Trait > | |
void | sortByTrait () |
SortingCriterion | sortingCriterion () const |
Field * | sortingField () const |
QString | toString () const |
![]() | |
QList (const QList< Addressee > &other) | |
QList (std::initializer_list< Addressee > args) | |
void | append (const Addressee &value) |
void | append (const QList< Addressee > &value) |
const Addressee & | at (int i) const |
Addressee & | back () |
const Addressee & | back () const |
iterator | begin () |
const_iterator | begin () const |
void | clear () |
const_iterator | constBegin () const |
const_iterator | constEnd () const |
bool | contains (const Addressee &value) const |
int | count (const Addressee &value) const |
int | count () const |
bool | empty () const |
iterator | end () |
const_iterator | end () const |
bool | endsWith (const Addressee &value) const |
iterator | erase (iterator pos) |
iterator | erase (iterator begin, iterator end) |
iterator | find (iterator from, const Addressee &t) |
iterator | find (const Addressee &t) |
const_iterator | find (const Addressee &t) const |
const_iterator | find (const_iterator from, const Addressee &t) const |
int | findIndex (const Addressee &t) const |
Addressee & | first () |
const Addressee & | first () const |
Addressee & | front () |
const Addressee & | front () const |
int | indexOf (const Addressee &value, int from) const |
void | insert (int i, const Addressee &value) |
iterator | insert (iterator before, const Addressee &value) |
bool | isEmpty () const |
Addressee & | last () |
const Addressee & | last () const |
int | lastIndexOf (const Addressee &value, int from) const |
int | length () const |
QList< Addressee > | mid (int pos, int length) const |
void | move (int from, int to) |
bool | operator!= (const QList< Addressee > &other) const |
QList< Addressee > | operator+ (const QList< Addressee > &other) const |
QList< Addressee > & | operator+= (const QList< Addressee > &other) |
QList< Addressee > & | operator+= (const Addressee &value) |
QList< Addressee > & | operator<< (const Addressee &value) |
QList< Addressee > & | operator<< (const QList< Addressee > &other) |
QList< Addressee > & | operator= (const QList< Addressee > &other) |
bool | operator== (const QList< Addressee > &other) const |
Addressee & | operator[] (int i) |
const Addressee & | operator[] (int i) const |
void | pop_back () |
void | pop_front () |
void | prepend (const Addressee &value) |
void | push_back (const Addressee &value) |
void | push_front (const Addressee &value) |
iterator | remove (iterator pos) |
int | remove (const Addressee &t) |
int | removeAll (const Addressee &value) |
void | removeAt (int i) |
void | removeFirst () |
void | removeLast () |
bool | removeOne (const Addressee &value) |
void | replace (int i, const Addressee &value) |
void | reserve (int alloc) |
int | size () const |
bool | startsWith (const Addressee &value) const |
void | swap (int i, int j) |
void | swap (QList< Addressee > &other) |
Addressee | takeAt (int i) |
Addressee | takeFirst () |
Addressee | takeLast () |
QSet< Addressee > | toSet () const |
std::list< Addressee > | toStdList () const |
QVector< Addressee > | toVector () const |
Addressee | value (int i, const Addressee &defaultValue) const |
Addressee | value (int i) const |
Additional Inherited Members | |
![]() | |
QList< Addressee > | fromSet (const QSet< Addressee > &set) |
QList< Addressee > | fromStdList (const std::list< Addressee > &list) |
QList< Addressee > | fromVector (const QVector< Addressee > &vector) |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | pointer |
typedef | reference |
typedef | size_type |
typedef | value_type |
Detailed Description
a QValueList of Addressee, with sorting functionality
This class extends the functionality of QValueList with sorting methods specific to the Addressee class. It can be used just like any other QValueList but is no template class.
An AddresseeList does not automatically keep sorted when addressees are added or removed or the sorting order is changed, as this would slow down larger operations by sorting after every step. So after such operations you have to call sort or sortBy to create a defined order again.
Iterator usage is inherited from QList and extensively documented there. Please remember that the state of an iterator is undefined after any sorting operation.
For the enumeration Type SortingCriterion, which specifies the field by the collection will be sorted, the following values exist: Uid, Name, FormattedName, FamilyName, GivenName.
Definition at line 288 of file addresseelist.h.
Constructor & Destructor Documentation
AddresseeList::AddresseeList | ( | ) |
Creates a new addressee list.
Definition at line 176 of file addresseelist.cpp.
AddresseeList::AddresseeList | ( | const AddresseeList & | other | ) |
Creates a new addressee list.
Definition at line 185 of file addresseelist.cpp.
Creates a new addressee list.
Definition at line 190 of file addresseelist.cpp.
AddresseeList::~AddresseeList | ( | ) |
Destroys the addressee list.
Definition at line 181 of file addresseelist.cpp.
Member Function Documentation
AddresseeList & AddresseeList::operator= | ( | const AddresseeList & | other | ) |
Assignment operator.
- Parameters
-
other the list to assign from
- Returns
- a reference to
this
Definition at line 195 of file addresseelist.cpp.
bool AddresseeList::reverseSorting | ( | ) | const |
Returns the direction of sorting.
- Returns
true
if sorting is done reverse,false
otherwise
Definition at line 246 of file addresseelist.cpp.
void AddresseeList::setReverseSorting | ( | bool | reverseSorting = true | ) |
Determines the direction of sorting.
On change, the list will not automatically be resorted.
- Parameters
-
reverseSorting true
if sorting should be done reverse,false
otherwise
Definition at line 241 of file addresseelist.cpp.
void AddresseeList::sort | ( | ) |
Sorts this list by its active sorting criterion.
This normally is the criterion of the last sortBy operation or FormattedName
if up to now there has been no sortBy operation.
Please note that the sorting trait of the last sortByTrait method call is not remembered and thus the action can not be repeated by this method.
Definition at line 270 of file addresseelist.cpp.
void AddresseeList::sortBy | ( | SortingCriterion | c | ) |
Sorts this list by a specific criterion.
- Parameters
-
c the criterion by which should be sorted
Definition at line 251 of file addresseelist.cpp.
void AddresseeList::sortByField | ( | Field * | field = 0 | ) |
Sorts this list by a specific field.
If no parameter is given, the last used Field object will be used.
- Parameters
-
field pointer to the Field object to be sorted by
Definition at line 312 of file addresseelist.cpp.
void AddresseeList::sortByMode | ( | SortMode * | mode = 0 | ) |
Sorts this list by a specific sorting mode.
- Parameters
-
mode pointer to the sorting mode object to be sorted by
Definition at line 334 of file addresseelist.cpp.
void AddresseeList::sortByTrait | ( | ) |
Templated sort function.
You normally will not want to use this but sortBy and sort instead as the existing sorting criteria completely suffice for most cases.
However, if you do want to use some special sorting criterion, you can write a trait class that will be provided to this templated method. This trait class has to have a class declaration like the following:
You can then pass this class to the sortByTrait method like this:
Please note that the sort method can not be used to repeat the sorting of the last sortByTrait
action.
Right now this method uses the bubble sort algorithm. This should be replaced for a better one when I have time.
Definition at line 276 of file addresseelist.cpp.
SortingCriterion AddresseeList::sortingCriterion | ( | ) | const |
Returns the active sorting criterion, ie the sorting criterion that will be used by a sort call.
Definition at line 345 of file addresseelist.cpp.
Field * AddresseeList::sortingField | ( | ) | const |
Returns the active sorting field, ie a pointer to the Field object which was used for the last sortByField operation.
This function returns the last GLOBAL sorting field, not the class specific one. You're a lot better off by keeping track of this locally.
Definition at line 350 of file addresseelist.cpp.
QString AddresseeList::toString | ( | ) | const |
Returns a string representation of the addressee list.
Definition at line 205 of file addresseelist.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:39 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.