KCal Library
#include <sortablelist.h>
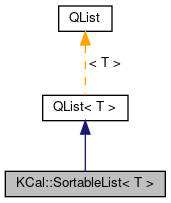
Public Member Functions | |
SortableList () | |
SortableList (const QList< T > &list) | |
bool | containsSorted (const T &value) const |
int | findGE (const T &value, int start=0) const |
int | findGT (const T &value, int start=0) const |
int | findLE (const T &value, int start=0) const |
int | findLT (const T &value, int start=0) const |
int | findSorted (const T &value, int start=0) const |
int | insertSorted (const T &value) |
int | removeSorted (const T &value, int start=0) |
void | sortUnique () |
![]() | |
QList (const QList< T > &other) | |
QList (std::initializer_list< T > args) | |
void | append (const T &value) |
void | append (const QList< T > &value) |
const T & | at (int i) const |
T & | back () |
const T & | back () const |
iterator | begin () |
const_iterator | begin () const |
void | clear () |
const_iterator | constBegin () const |
const_iterator | constEnd () const |
bool | contains (const T &value) const |
int | count (const T &value) const |
int | count () const |
bool | empty () const |
iterator | end () |
const_iterator | end () const |
bool | endsWith (const T &value) const |
iterator | erase (iterator pos) |
iterator | erase (iterator begin, iterator end) |
iterator | find (iterator from, const T &t) |
iterator | find (const T &t) |
const_iterator | find (const T &t) const |
const_iterator | find (const_iterator from, const T &t) const |
int | findIndex (const T &t) const |
T & | first () |
const T & | first () const |
T & | front () |
const T & | front () const |
int | indexOf (const T &value, int from) const |
void | insert (int i, const T &value) |
iterator | insert (iterator before, const T &value) |
bool | isEmpty () const |
T & | last () |
const T & | last () const |
int | lastIndexOf (const T &value, int from) const |
int | length () const |
QList< T > | mid (int pos, int length) const |
void | move (int from, int to) |
bool | operator!= (const QList< T > &other) const |
QList< T > | operator+ (const QList< T > &other) const |
QList< T > & | operator+= (const QList< T > &other) |
QList< T > & | operator+= (const T &value) |
QList< T > & | operator<< (const T &value) |
QList< T > & | operator<< (const QList< T > &other) |
QList< T > & | operator= (const QList< T > &other) |
bool | operator== (const QList< T > &other) const |
T & | operator[] (int i) |
const T & | operator[] (int i) const |
void | pop_back () |
void | pop_front () |
void | prepend (const T &value) |
void | push_back (const T &value) |
void | push_front (const T &value) |
iterator | remove (iterator pos) |
int | remove (const T &t) |
int | removeAll (const T &value) |
void | removeAt (int i) |
void | removeFirst () |
void | removeLast () |
bool | removeOne (const T &value) |
void | replace (int i, const T &value) |
void | reserve (int alloc) |
int | size () const |
bool | startsWith (const T &value) const |
void | swap (int i, int j) |
void | swap (QList< T > &other) |
T | takeAt (int i) |
T | takeFirst () |
T | takeLast () |
QSet< T > | toSet () const |
std::list< T > | toStdList () const |
QVector< T > | toVector () const |
T | value (int i, const T &defaultValue) const |
T | value (int i) const |
Additional Inherited Members | |
![]() | |
QList< T > | fromSet (const QSet< T > &set) |
QList< T > | fromStdList (const std::list< T > &list) |
QList< T > | fromVector (const QVector< T > &vector) |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | pointer |
typedef | reference |
typedef | size_type |
typedef | value_type |
Detailed Description
template<class T>
class KCal::SortableList< T >
A QList which can be sorted.
For a QList is capable of being sorted, SortedList provides additional optimized methods which can be used when the list is sorted and has no duplicate entries.
Because SortableList has no data members, an object may be referred to interchangeably as either a QList or SortableList. Just bear in mind that the results of the SortableList methods are undefined when the list is unsorted or contains duplicate entries.
To sort the list and remove duplicate entries, thereby allowing use of other SortableList methods, use sortUnique(). Once sortUnique() has been called, use findSorted(), containsSorted() and removeSorted() in preference to QList::indexOf(), QList::contains() and QList::removeAll(). Use findLE(), findLT(), findGE(), findGT() to find the index to the nearest value in the list which is <=, <, >= or > a given value. To add a value to the list, use insertSorted() in preference to insert(), append(), prepend(), operator<<() or operator+=().
Definition at line 86 of file sortablelist.h.
Constructor & Destructor Documentation
|
inline |
Constructs an empty sortable list.
Definition at line 92 of file sortablelist.h.
|
inline |
Constructs a sortable list by copying another one.
- Parameters
-
list is the list to copy.
Definition at line 99 of file sortablelist.h.
Member Function Documentation
|
inline |
Return whether the list contains value value
.
The list must be sorted; if not, the result is undefined. When the list is sorted, use this optimised method in preference to QList<T>::contains().
- Parameters
-
value is the value to find.
- Returns
- true if list contains
value
; false otherwise.
Definition at line 110 of file sortablelist.h.
int KCal::SortableList< T >::findGE | ( | const T & | value, |
int | start = 0 |
||
) | const |
Search the list for the first item >= value
.
The list must be sorted; if not, the result is undefined.
- Parameters
-
value is the value to find. start is the start index for search (default is from beginning).
- Returns
- index to item in list, or -1 if
value
> last value in the list.
Definition at line 246 of file sortablelist.h.
int KCal::SortableList< T >::findGT | ( | const T & | value, |
int | start = 0 |
||
) | const |
Search the list for the first item > value
.
The list must be sorted; if not, the result is undefined.
- Parameters
-
value is the value to find. start is the start index for search (default is from beginning).
- Returns
- index to item in list, or -1 if
value
>= last value in the list.
Definition at line 264 of file sortablelist.h.
int KCal::SortableList< T >::findLE | ( | const T & | value, |
int | start = 0 |
||
) | const |
Search the list for the last item <= value
.
The list must be sorted; if not, the result is undefined.
- Parameters
-
value is the value to find. start is the start index for search (default is from beginning).
- Returns
- index to item in list, or -1 if
value
< first value in the list.
Definition at line 212 of file sortablelist.h.
int KCal::SortableList< T >::findLT | ( | const T & | value, |
int | start = 0 |
||
) | const |
Search the list for the last item < value
.
The list must be sorted; if not, the result is undefined.
- Parameters
-
value is the value to find. start is the start index for search (default is from beginning).
- Returns
- index to item in list, or -1 if
value
<= first value in the list.
Definition at line 229 of file sortablelist.h.
int KCal::SortableList< T >::findSorted | ( | const T & | value, |
int | start = 0 |
||
) | const |
Search the list for the item equal to value
.
The list must be sorted; if not, the result is undefined. When the list is sorted, use this optimised method in preference to QList<T>::indexOf().
- Parameters
-
value is the value to find. start is the start index for search (default is from beginning).
- Returns
- index to item in list, or -1 if
value
not found in the list.
Definition at line 195 of file sortablelist.h.
int KCal::SortableList< T >::insertSorted | ( | const T & | value | ) |
Insert a value in the list, in correct sorted order.
If the same value is already in the list, no change is made.
The list must already be sorted before calling this method; otherwise the result is undefined.
- Parameters
-
value is the value to insert.
- Returns
- index to inserted item in list, or to the pre-existing entry equal to
value
.
Definition at line 282 of file sortablelist.h.
int KCal::SortableList< T >::removeSorted | ( | const T & | value, |
int | start = 0 |
||
) |
Remove value value
from the list.
The list must be sorted. When the list is sorted, use this optimised method in preference to QList<T>::removeAll().
- Parameters
-
value is the value to remove. start is the start index for search (default is from beginning).
- Returns
- index to removed value, or -1 if not found.
Definition at line 292 of file sortablelist.h.
|
inline |
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:30 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.