KIMAP Library
#include <getmetadatajob.h>
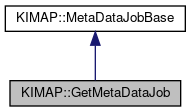
Public Types | |
enum | Depth { NoDepth = 0, OneLevel, AllLevels } |
![]() | |
enum | ServerCapability { Metadata = 0, Annotatemore } |
Public Member Functions | |
GetMetaDataJob (Session *session) | |
KIMAP_DEPRECATED void | addEntry (const QByteArray &entry, const QByteArray &attribute=QByteArray()) |
void | addRequestedEntry (const QByteArray &entry) |
QMap< QByteArray, QMap < QByteArray, QByteArray > > | allMetaData (const QString &mailBox) const |
QMap< QByteArray, QByteArray > | allMetaData () const |
KIMAP_DEPRECATED QByteArray | metaData (const QString &mailBox, const QByteArray &entry, const QByteArray &attribute=QByteArray()) const |
QByteArray | metaData (const QByteArray &entry) const |
void | setDepth (Depth depth) |
void | setMaximumSize (qint64 size) |
![]() | |
MetaDataJobBase (Session *session) | |
QString | mailBox () const |
ServerCapability | serverCapability () const |
void | setMailBox (const QString &mailBox) |
void | setServerCapability (const ServerCapability &capability) |
Protected Member Functions | |
virtual void | doStart () |
virtual void | handleResponse (const Message &response) |
![]() | |
MetaDataJobBase (JobPrivate &dd) | |
Detailed Description
Fetches mailbox metadata.
Provides support for the IMAP METADATA extension; both the final RFC version (RFC 5464) and the older, incompatible draft version (known as ANNOTATEMORE) (draft-daboo-imap-annotatemore-07). See setServerCompatibility().
This job can only be run when the session is in the authenticated (or selected) state.
If the server supports ACLs, the user will need the Acl::Lookup right on the mailbox, as well as one of
- Acl::Read
- Acl::KeepSeen
- Acl::Write
- Acl::Insert
- Acl::Post Otherwise, the user must be able to list the mailbox and either read or write the message content.
Note also that on servers that implement the Annotatemore version of the extension, only Acl::Lookup rights are required (ie: the user must be able to list the mailbox).
Definition at line 61 of file getmetadatajob.h.
Member Enumeration Documentation
Used to specify the depth of the metadata heirachy to walk.
Enumerator | |
---|---|
NoDepth |
Only the requested entries. |
OneLevel |
The requested entries and all their direct children. |
AllLevels |
The requested entries and all their descendants. |
Definition at line 75 of file getmetadatajob.h.
Member Function Documentation
void GetMetaDataJob::addEntry | ( | const QByteArray & | entry, |
const QByteArray & | attribute = QByteArray() |
||
) |
Add an entry to the query list.
See SetMetaDataJob for a description of metadata entry names.
When operating in Annotatemore mode, you should provide an attribute name. Typically this will be "value", "value.priv" or "value.shared", although you might want to fetch the "content-type" or "content-language" attributes as well.
- Parameters
-
entry the metadata entry name attribute the attribute name, in Annotatemore mode
- Deprecated:
- use addRequestedEntry(QByteArray) instead
Definition at line 164 of file getmetadatajob.cpp.
void GetMetaDataJob::addRequestedEntry | ( | const QByteArray & | entry | ) |
Add an entry to the query list.
See SetMetaDataJob for a description of metadata entry names.
Note that this expects METADATA style entries (with a /shared or /private prefix typically). In ANNOTATEMORE mode, this prefix is automatically replaced with an appropriate attribute.
- Parameters
-
entry the metadata entry name
Definition at line 174 of file getmetadatajob.cpp.
QMap< QByteArray, QMap< QByteArray, QByteArray > > GetMetaDataJob::allMetaData | ( | const QString & | mailBox | ) | const |
Get all the metadata for a given mailbox.
The returned map is from metadata entry names to attributes or values.
If operating in Metadata mode, the metadata value is stored against the empty QByteArray:
The equivalent in Annotatemore mode would be:
- Parameters
-
mailBox a mailbox name or an empty string for server metadata
- Returns
- a map from metadata entry names to attributes or values
Definition at line 228 of file getmetadatajob.cpp.
QMap< QByteArray, QByteArray > GetMetaDataJob::allMetaData | ( | ) | const |
Get all the metadata.
Note that the returned map uses METADATA style entries (with a /shared or /private prefix typically), also in ANNOTATEMORE mode.
- Returns
- a map from metadata entry names to values
Definition at line 234 of file getmetadatajob.cpp.
QByteArray GetMetaDataJob::metaData | ( | const QString & | mailBox, |
const QByteArray & | entry, | ||
const QByteArray & | attribute = QByteArray() |
||
) | const |
Get a single metadata entry.
The metadata must have been requested using addEntry(), and the job must have completed successfully, or this method will not return anything.
Note that if setMaximumSize() was used to limit the size of returned metadata, this method may return an empty QByteArray even if the metadata entry was requested and exists on the server. This will happen when the metadata entry is larger than the size limit given to setMaximumSize().
- Parameters
-
mailBox the mailbox the metadata is attached to, or an empty string for server metadata entry the entry to get attribute (only in Annotatemore mode) the attribute to get
- Returns
- the metadata entry value
- Deprecated:
- use metaData(QByteArray entry) instead
Definition at line 203 of file getmetadatajob.cpp.
QByteArray GetMetaDataJob::metaData | ( | const QByteArray & | entry | ) | const |
Get a single metadata entry.
The metadata must have been requested using addEntry(), and the job must have completed successfully, or this method will not return anything.
Note that if setMaximumSize() was used to limit the size of returned metadata, this method may return an empty QByteArray even if the metadata entry was requested and exists on the server. This will happen when the metadata entry is larger than the size limit given to setMaximumSize().
Note that this expects METADATA style entries (with a /shared or /private prefix typically). In ANNOTATEMORE mode, this prefix is automatically replaced with an appropriate attribute.
- Parameters
-
entry the entry to get
- Returns
- the metadata entry value
Definition at line 221 of file getmetadatajob.cpp.
void GetMetaDataJob::setDepth | ( | Depth | depth | ) |
Sets whether to retrieve children or descendants of the requested entries.
Metadata entry names are heirachical, much like UNIX path names. It therefore makes sense to ask for an entry and all its children (OneLevel) or an entry and all its descendants (AllLevels).
For example, /shared/foo/bar/baz is a child of /shared/foo/bar and a descendent of /shared/foo. So if you request the entry "/shared/foo" with depth NoDepth, you will only get the "/shared/foo" entry. If you set the depth to OneLevel, you will also get "/shared/foo/bar". If you set the depth to AllLevels, you will also get "/shared/foo/bar/baz", and every other metadata entry that starts with "/shared/foo/".
Note that this is only used when the server capability mode is Metadata.
- Parameters
-
depth the depth of the metadata tree to return
Definition at line 187 of file getmetadatajob.cpp.
void GetMetaDataJob::setMaximumSize | ( | qint64 | size | ) |
Limits the size of returned metadata entries.
In order to save time or bandwidth, it is possible to prevent the server from returning metadata entries that are larger than a certain size. These entries will simply not appear in the list returned by allMetaData(), and will not be accessible using metaData().
Note that this is only used when the server capability mode is Metadata.
The default is no limit (-1). A value of less than -1 will cause the job to fail.
- Parameters
-
size the entry size limit, in octets, or -1 for no limit
Definition at line 181 of file getmetadatajob.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:37:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.