KLDAP Library
#include <ldapmodel.h>
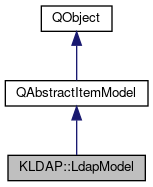
Classes | |
class | LdapModelPrivate |
Public Types | |
enum | LdapDataType { DistinguishedName = 0, Attribute } |
enum | Roles { NodeTypeRole = Qt::UserRole + 1 } |
Public Slots | |
virtual void | revert () |
virtual bool | submit () |
Signals | |
void | ready () |
Public Member Functions | |
LdapModel (QObject *parent=0) | |
LdapModel (LdapConnection &connection, QObject *parent=0) | |
virtual bool | canFetchMore (const QModelIndex &parent) const |
virtual int | columnCount (const QModelIndex &parent) const |
virtual QVariant | data (const QModelIndex &index, int role) const |
virtual bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) |
virtual void | fetchMore (const QModelIndex &parent) |
virtual Qt::ItemFlags | flags (const QModelIndex &index) const |
virtual bool | hasChildren (const QModelIndex &parent) const |
bool | hasChildrenOfType (const QModelIndex &parent, LdapDataType type) const |
virtual QVariant | headerData (int section, Qt::Orientation orientation, int role) const |
virtual QModelIndex | index (int row, int col, const QModelIndex &parent) const |
virtual bool | insertRows (int row, int count, const QModelIndex &parent=QModelIndex()) |
virtual QMimeData * | mimeData (const QModelIndexList &indexes) const |
virtual QModelIndex | parent (const QModelIndex &child) const |
virtual bool | removeRows (int row, int count, const QModelIndex &parent=QModelIndex()) |
virtual int | rowCount (const QModelIndex &parent) const |
void | setConnection (LdapConnection &connection) |
virtual bool | setData (const QModelIndex &index, const QVariant &value, int role=Qt::EditRole) |
virtual void | sort (int column, Qt::SortOrder order=Qt::AscendingOrder) |
virtual Qt::DropActions | supportedDropActions () const |
![]() | |
QAbstractItemModel (QObject *parent) | |
virtual QModelIndex | buddy (const QModelIndex &index) const |
virtual int | columnCount (const QModelIndex &parent) const =0 |
void | columnsAboutToBeInserted (const QModelIndex &parent, int start, int end) |
void | columnsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsAboutToBeRemoved (const QModelIndex &parent, int start, int end) |
void | columnsInserted (const QModelIndex &parent, int start, int end) |
void | columnsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsRemoved (const QModelIndex &parent, int start, int end) |
virtual QVariant | data (const QModelIndex &index, int role) const =0 |
void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight) |
bool | hasIndex (int row, int column, const QModelIndex &parent) const |
void | headerDataChanged (Qt::Orientation orientation, int first, int last) |
virtual QModelIndex | index (int row, int column, const QModelIndex &parent) const =0 |
bool | insertColumn (int column, const QModelIndex &parent) |
virtual bool | insertColumns (int column, int count, const QModelIndex &parent) |
bool | insertRow (int row, const QModelIndex &parent) |
virtual QMap< int, QVariant > | itemData (const QModelIndex &index) const |
void | layoutAboutToBeChanged () |
void | layoutChanged () |
virtual QModelIndexList | match (const QModelIndex &start, int role, const QVariant &value, int hits, QFlags< Qt::MatchFlag > flags) const |
virtual QStringList | mimeTypes () const |
void | modelAboutToBeReset () |
void | modelReset () |
virtual QModelIndex | parent (const QModelIndex &index) const =0 |
bool | removeColumn (int column, const QModelIndex &parent) |
virtual bool | removeColumns (int column, int count, const QModelIndex &parent) |
bool | removeRow (int row, const QModelIndex &parent) |
virtual void | revert () |
const QHash< int, QByteArray > & | roleNames () const |
virtual int | rowCount (const QModelIndex &parent) const =0 |
void | rowsAboutToBeInserted (const QModelIndex &parent, int start, int end) |
void | rowsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsAboutToBeRemoved (const QModelIndex &parent, int start, int end) |
void | rowsInserted (const QModelIndex &parent, int start, int end) |
void | rowsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsRemoved (const QModelIndex &parent, int start, int end) |
virtual bool | setHeaderData (int section, Qt::Orientation orientation, const QVariant &value, int role) |
virtual bool | setItemData (const QModelIndex &index, const QMap< int, QVariant > &roles) |
void | setSupportedDragActions (QFlags< Qt::DropAction > actions) |
QModelIndex | sibling (int row, int column, const QModelIndex &index) const |
virtual QSize | span (const QModelIndex &index) const |
virtual bool | submit () |
Qt::DropActions | supportedDragActions () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
void | beginInsertColumns (const QModelIndex &parent, int first, int last) |
void | beginInsertRows (const QModelIndex &parent, int first, int last) |
bool | beginMoveColumns (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
bool | beginMoveRows (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
void | beginRemoveColumns (const QModelIndex &parent, int first, int last) |
void | beginRemoveRows (const QModelIndex &parent, int first, int last) |
void | beginResetModel () |
void | changePersistentIndex (const QModelIndex &from, const QModelIndex &to) |
void | changePersistentIndexList (const QModelIndexList &from, const QModelIndexList &to) |
QModelIndex | createIndex (int row, int column, void *ptr) const |
QModelIndex | createIndex (int row, int column, int id) const |
QModelIndex | createIndex (int row, int column, quint32 id) const |
void | endInsertColumns () |
void | endInsertRows () |
void | endMoveColumns () |
void | endMoveRows () |
void | endRemoveColumns () |
void | endRemoveRows () |
void | endResetModel () |
QModelIndexList | persistentIndexList () const |
void | reset () |
void | resetInternalData () |
void | setRoleNames (const QHash< int, QByteArray > &roleNames) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
A ModelView interface to an LDAP tree.
At present the model is read only. Editing is planned for a future release.
This class is best used in conjunction with an LdapStructureProxyModel object for displaying the structure of an LDAP tree, and with LdapAttributeProxyModel for displaying the attributes of particular objects within the tree.
Definition at line 42 of file ldapmodel.h.
Constructor & Destructor Documentation
|
explicit |
Constructs an LdapModel.
You should set a connection for the model to use with setConnection(). Clients of this class should connect a slot to the ready() signal before setting this model onto a view.
- Parameters
-
parent the parent QObject
- See also
- setConnection()
- ready()
Definition at line 34 of file ldapmodel.cpp.
|
explicit |
Constructs an LdapModel.
Clients of this class should connect a slot to the ready() signal before setting this model onto a view.
- Parameters
-
connection the Ldap connection to use in model construction parent the parent QObject
- See also
- setConnection()
- ready()
Definition at line 41 of file ldapmodel.cpp.
Member Function Documentation
|
virtual |
Reimplemented from QAbstractItemModel::canFetchMore().
Reimplemented from QAbstractItemModel.
Definition at line 206 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::columnCount().
Definition at line 170 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::data().
- Todo:
- Include support for nice decorative icons dependent upon the objectClass + other role data.
- Todo:
- Include support for other roles as needed
Definition at line 101 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::dropMimedata().
This is a placeholder for when LdapModel beomes writeable and always returns false.
Reimplemented from QAbstractItemModel.
Definition at line 262 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::fetchMore().
Reimplemented from QAbstractItemModel.
Definition at line 213 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::flags().
Read-only for now, make read-write upon request
Reimplemented from QAbstractItemModel.
Definition at line 160 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::hasChildren().
Reimplemented from QAbstractItemModel.
Definition at line 188 of file ldapmodel.cpp.
bool LdapModel::hasChildrenOfType | ( | const QModelIndex & | parent, |
LdapDataType | type | ||
) | const |
Checks to see if the item referenced by parent
has any children of the type type
.
If the item has not been populated by fetchMore() yet, then this function returns true.
- See also
- fetchMore()
- Parameters
-
parent Index to the item to query. type The type of child item to search for.
Definition at line 274 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::headerData().
Reimplemented from QAbstractItemModel.
Definition at line 147 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::index().
Definition at line 83 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::insertRows().
This is a placeholder for when LdapModel beomes writeable and always returns false.
Reimplemented from QAbstractItemModel.
Definition at line 227 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::mimedata().
This is a placeholder for when LdapModel beomes writeable and always returns 0.
Reimplemented from QAbstractItemModel.
Definition at line 256 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::parent().
Definition at line 67 of file ldapmodel.cpp.
|
signal |
The ready() signal is emitted when the model is ready for use by other components.
When the model is first created and a connection is set, the model queries the LDAP server for its base DN and automatically creates items down to that level. This requires the event loop to be running. This signal indicates that this process has completed and the model can now be set onto views or queried directly from code.
|
virtual |
Reimplemented from QAbstractItemModel::removeRows().
This is a placeholder for when LdapModel beomes writeable and always returns false.
Reimplemented from QAbstractItemModel.
Definition at line 236 of file ldapmodel.cpp.
|
virtualslot |
Reimplemented from QAbstractItemModel::revert().
This is a placeholder for when LdapModel beomes writeable. This implementation does nothing.
Definition at line 312 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::rowCount().
Definition at line 177 of file ldapmodel.cpp.
void LdapModel::setConnection | ( | LdapConnection & | connection | ) |
Set the connection that the model should use.
- Parameters
-
connection the model connection to set
- See also
- LdapConnection
- LdapUrl
Definition at line 56 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::setData().
This is a placeholder for when LdapModel beomes writeable and always returns false.
Reimplemented from QAbstractItemModel.
Definition at line 137 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::removeRows().
The default implementation does nothing.
Reimplemented from QAbstractItemModel.
Definition at line 245 of file ldapmodel.cpp.
|
virtualslot |
Reimplemented from QAbstractItemModel::revert().
This is a placeholder for when LdapModel beomes writeable. This implementation does nothing and returns false.
Definition at line 317 of file ldapmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::supportedDropActions().
The default implementation returns Qt::MoveAction.
Reimplemented from QAbstractItemModel.
Definition at line 251 of file ldapmodel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:37:58 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.