KMIME Library
#include <kmime_codecs.h>
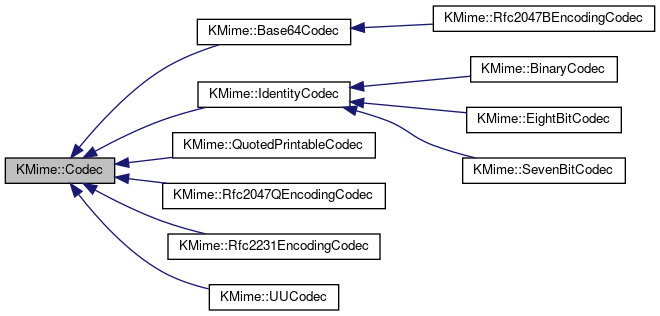
Public Member Functions | |
virtual | ~Codec () |
virtual bool | decode (const char *&scursor, const char *const send, char *&dcursor, const char *const dend, bool withCRLF=false) const |
virtual QByteArray | decode (const QByteArray &src, bool withCRLF=false) const |
virtual bool | encode (const char *&scursor, const char *const send, char *&dcursor, const char *const dend, bool withCRLF=false) const |
virtual QByteArray | encode (const QByteArray &src, bool withCRLF=false) const |
virtual Decoder * | makeDecoder (bool withCRLF=false) const =0 |
virtual Encoder * | makeEncoder (bool withCRLF=false) const =0 |
virtual int | maxDecodedSizeFor (int insize, bool withCRLF=false) const =0 |
virtual int | maxEncodedSizeFor (int insize, bool withCRLF=false) const =0 |
virtual const char * | name () const =0 |
Static Public Member Functions | |
static Codec * | codecForName (const char *name) |
static Codec * | codecForName (const QByteArray &name) |
Protected Member Functions | |
Codec () | |
Detailed Description
An abstract base class of codecs for common mail transfer encodings.
Provides an abstract base class of codecs like base64 and quoted-printable. Implemented as a singleton.
Definition at line 83 of file kmime_codecs.h.
Constructor & Destructor Documentation
|
inlineprotected |
Contructs the codec.
Definition at line 93 of file kmime_codecs.h.
|
inlinevirtual |
Destroys the codec.
Definition at line 256 of file kmime_codecs.h.
Member Function Documentation
|
static |
Returns a codec associated with the specified name
.
- Parameters
-
name points to a character string containing a valid codec name.
Definition at line 82 of file kmime_codecs.cpp.
|
static |
Returns a codec associated with the specified name
.
- Parameters
-
name is a QByteArray containing a valid codec name.
Definition at line 88 of file kmime_codecs.cpp.
|
virtual |
Convenience wrapper that can be used for small chunks of data when you can provide a large enough buffer.
The default implementation creates a Decoder and uses it.
Decodes a chunk of bytes starting at scursor
and extending to send
into the buffer described by dcursor
and dend
.
This function doesn't support chaining of blocks. The returned block cannot be added to, but you don't need to finalize it, too.
Example usage (in
contains the input data):
KMime::Codec *codec = KMime::Codec::codecForName( "base64" ); kFatal( !codec ) << "no base64 codec found!?"; QByteArray out( in.size() ); // good guess for any encoding... QByteArray::Iterator iit = in.begin(); QByteArray::Iterator oit = out.begin(); if ( !codec->decode( iit, in.end(), oit, out.end() ) ) { kDebug() << "output buffer too small"; return; } kDebug() << "Size of decoded data:" << oit - out.begin();
- Parameters
-
scursor is a pointer to the start of the input buffer. send is a pointer to the end of the input buffer. dcursor is a pointer to the start of the output buffer. dend is a pointer to the end of the output buffer. withCRLF if true, make the newlines CRLF; else use LF.
- Returns
- false if the decoded data didn't fit into the output buffer; true otherwise.
Definition at line 183 of file kmime_codecs.cpp.
|
virtual |
Even more convenient, but also a bit slower and more memory intensive, since it allocates storage for the worst case and then shrinks the result QByteArray to the actual size again.
For use with small src
.
- Parameters
-
src is a QByteArray containing the data to decode. withCRLF if true, make the newlines CRLF; else use LF.
Reimplemented in KMime::IdentityCodec.
Definition at line 160 of file kmime_codecs.cpp.
|
virtual |
Convenience wrapper that can be used for small chunks of data when you can provide a large enough buffer.
The default implementation creates an Encoder and uses it.
Encodes a chunk of bytes starting at scursor
and extending to send
into the buffer described by dcursor
and dend
.
This function doesn't support chaining of blocks. The returned block cannot be added to, but you don't need to finalize it, too.
Example usage (in
contains the input data):
KMime::Codec *codec = KMime::Codec::codecForName( "base64" ); kFatal( !codec ) << "no base64 codec found!?"; QByteArray out( in.size()*1.4 ); // crude maximal size of b64 encoding QByteArray::Iterator iit = in.begin(); QByteArray::Iterator oit = out.begin(); if ( !codec->encode( iit, in.end(), oit, out.end() ) ) { kDebug() << "output buffer too small"; return; } kDebug() << "Size of encoded data:" << oit - out.begin();
- Parameters
-
scursor is a pointer to the start of the input buffer. send is a pointer to the end of the input buffer. dcursor is a pointer to the start of the output buffer. dend is a pointer to the end of the output buffer. withCRLF if true, make the newlines CRLF; else use LF.
- Returns
- false if the encoded data didn't fit into the output buffer; true otherwise.
Definition at line 108 of file kmime_codecs.cpp.
|
virtual |
Even more convenient, but also a bit slower and more memory intensive, since it allocates storage for the worst case and then shrinks the result QByteArray to the actual size again.
For use with small src
.
- Parameters
-
src is a QByteArray containing the data to encode. withCRLF if true, make the newlines CRLF; else use LF.
Reimplemented in KMime::IdentityCodec.
Definition at line 137 of file kmime_codecs.cpp.
|
pure virtual |
Creates the decoder for the codec.
- Returns
- a pointer to an instance of the codec's decoder.
Implemented in KMime::Rfc2231EncodingCodec, KMime::Rfc2047QEncodingCodec, KMime::Base64Codec, KMime::IdentityCodec, KMime::QuotedPrintableCodec, and KMime::UUCodec.
|
pure virtual |
Creates the encoder for the codec.
- Returns
- a pointer to an instance of the codec's encoder.
Implemented in KMime::Rfc2231EncodingCodec, KMime::Rfc2047BEncodingCodec, KMime::Rfc2047QEncodingCodec, KMime::Base64Codec, KMime::IdentityCodec, KMime::QuotedPrintableCodec, and KMime::UUCodec.
|
pure virtual |
Computes the maximum size, in characters, needed for the deccoding.
- Parameters
-
insize is the number of input characters to be decoded. withCRLF if true, make the newlines CRLF; else use LF.
- Returns
- the maximum number of characters in the decoding.
Implemented in KMime::Rfc2231EncodingCodec, KMime::BinaryCodec, KMime::Rfc2047BEncodingCodec, KMime::Rfc2047QEncodingCodec, KMime::Base64Codec, KMime::QuotedPrintableCodec, KMime::IdentityCodec, and KMime::UUCodec.
|
pure virtual |
Computes the maximum size, in characters, needed for the encoding.
- Parameters
-
insize is the number of input characters to be encoded. withCRLF if true, make the newlines CRLF; else use LF.
- Returns
- the maximum number of characters in the encoding.
Implemented in KMime::BinaryCodec, KMime::Rfc2231EncodingCodec, KMime::Rfc2047BEncodingCodec, KMime::Rfc2047QEncodingCodec, KMime::Base64Codec, KMime::QuotedPrintableCodec, KMime::IdentityCodec, and KMime::UUCodec.
|
pure virtual |
Returns the name of the encoding.
Guaranteed to be lowercase.
Implemented in KMime::BinaryCodec, KMime::Rfc2231EncodingCodec, KMime::EightBitCodec, KMime::Rfc2047BEncodingCodec, KMime::SevenBitCodec, KMime::Rfc2047QEncodingCodec, KMime::Base64Codec, KMime::QuotedPrintableCodec, and KMime::UUCodec.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:37:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.