Kontact Plugin Interface Library
#include <plugin.h>
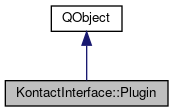
Public Slots | |
void | slotConfigUpdated () |
Public Member Functions | |
Plugin (Core *core, QObject *parent, const char *appName, const char *pluginName=0) | |
virtual | ~Plugin () |
virtual const KAboutData * | aboutData () const |
void | aboutToSelect () |
virtual void | bringToForeground () |
virtual bool | canDecodeMimeData (const QMimeData *data) const |
virtual void | configUpdated () |
Core * | core () const |
virtual bool | createDBUSInterface (const QString &serviceType) |
virtual Summary * | createSummaryWidget (QWidget *parent) |
bool | disabled () const |
QString | executableName () const |
QString | icon () const |
QString | identifier () const |
void | insertNewAction (KAction *action) |
void | insertSyncAction (KAction *action) |
virtual QStringList | invisibleToolbarActions () const |
virtual bool | isRunningStandalone () const |
QList< KAction * > | newActions () const |
KParts::ReadOnlyPart * | part () |
virtual void | processDropEvent (QDropEvent *) |
virtual bool | queryClose () const |
virtual void | readProperties (const KConfigGroup &) |
QString | registerClient () |
virtual void | saveProperties (KConfigGroup &) |
virtual void | select () |
void | setDisabled (bool value) |
void | setExecutableName (const QString &name) |
void | setIcon (const QString &icon) |
void | setIdentifier (const QString &identifier) |
void | setPartLibraryName (const QByteArray &name) |
void | setShowInSideBar (bool hasPart) |
void | setTitle (const QString &title) |
virtual void | shortcutChanged () |
virtual bool | showInSideBar () const |
QList< KAction * > | syncActions () const |
virtual QString | tipFile () const |
QString | title () const |
virtual int | weight () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
virtual KParts::ReadOnlyPart * | createPart ()=0 |
KParts::ReadOnlyPart * | loadPart () |
virtual void | virtual_hook (int id, void *data) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Base class for all Plugins in Kontact.
Inherit from it to get a plugin. It can insert an icon into the sidepane, add widgets to the widgetstack and add menu items via XMLGUI.
Constructor & Destructor Documentation
Creates a new plugin.
Private class that helps to provide binary compatibility between releases.
- Parameters
-
core The core object that manages the plugin. parent The parent object. appName The name of the application that provides the part. This is the name used for DBus registration. It's ok to have several plugins using the same application name. pluginName The unique name of the plugin. Defaults to appName if not set.
Definition at line 76 of file plugin.cpp.
|
virtual |
Destroys the plugin.
Definition at line 90 of file plugin.cpp.
Member Function Documentation
|
virtual |
Reimplement this method if you want to add your credits to the Kontact about dialog.
Definition at line 157 of file plugin.cpp.
void Plugin::aboutToSelect | ( | ) |
Called by kontact when the plugin is selected by the user.
Calls the virtual method select(), but also handles some standard behavior like "invisible toolbar actions".
Definition at line 274 of file plugin.cpp.
|
virtual |
Reimplement this method if your application needs a different approach to be brought in the foreground.
The default behaviour is calling the binary. This is only required if your part is also available as standalone application.
Definition at line 367 of file plugin.cpp.
|
virtual |
Returns whether the plugin can handle the drag object of the given mime type.
Definition at line 251 of file plugin.cpp.
|
virtual |
This function is called whenever the config dialog has been closed successfully.
Definition at line 287 of file plugin.cpp.
Core * Plugin::core | ( | ) | const |
Returns a pointer to the kontact core object.
Definition at line 269 of file plugin.cpp.
|
virtual |
Create the D-Bus interface for the given serviceType
, if this plugin provides it.
Returns true
on success, false
otherwise.
- Parameters
-
serviceType the D-Bus service type to create an interface for
Definition at line 141 of file plugin.cpp.
|
protectedpure virtual |
Reimplement and return the part here.
Reimplementing createPart() is mandatory!
Reimplement this method if you want to add a widget for your application to Kontact's summary page.
- Parameters
-
parent The parent widget of the summary widget.
Definition at line 379 of file plugin.cpp.
bool Plugin::disabled | ( | ) | const |
Returns whether the plugin is disabled.
Definition at line 405 of file plugin.cpp.
QString Plugin::executableName | ( | ) | const |
Returns the name of the executable (if existent).
Definition at line 131 of file plugin.cpp.
QString Plugin::icon | ( | ) | const |
Returns the icon name that is used for the plugin.
Definition at line 121 of file plugin.cpp.
QString Plugin::identifier | ( | ) | const |
Returns the identifier of the plugin.
Definition at line 101 of file plugin.cpp.
void Plugin::insertNewAction | ( | KAction * | action | ) |
Inserts a custom "New" action
.
- Parameters
-
action the new action to insert
Definition at line 226 of file plugin.cpp.
void Plugin::insertSyncAction | ( | KAction * | action | ) |
Inserts a custom "Sync" action
.
- Parameters
-
action the custom Sync action to insert
Definition at line 231 of file plugin.cpp.
|
virtual |
Returns a list of action names that shall be hidden in the main toolbar.
Definition at line 246 of file plugin.cpp.
|
virtual |
Reimplement this method and return whether a standalone application is still running.
This is only required if your part is also available as standalone application.
Definition at line 147 of file plugin.cpp.
|
protected |
Returns the loaded part.
Definition at line 152 of file plugin.cpp.
QList< KAction * > Plugin::newActions | ( | ) | const |
Returns the list of custom "New" actions.
Definition at line 236 of file plugin.cpp.
KParts::ReadOnlyPart * Plugin::part | ( | ) |
You can use this method if you need to access the current part.
You can be sure that you always get the same pointer as long as the part has not been deleted.
Definition at line 190 of file plugin.cpp.
|
virtual |
Process drop event.
Definition at line 257 of file plugin.cpp.
|
virtual |
Reimplement this method if you want to add checks before closing the main kontact window.
Return true if it's OK to close the window. If any loaded plugin returns false from this method, then the main kontact window will not close.
Definition at line 395 of file plugin.cpp.
|
virtual |
Session management: read properties.
Definition at line 261 of file plugin.cpp.
QString Plugin::registerClient | ( | ) |
Registers the client at DBus and returns the dbus identifier.
Definition at line 208 of file plugin.cpp.
|
virtual |
Session management: save properties.
Definition at line 265 of file plugin.cpp.
|
virtual |
This function is called when the plugin is selected by the user before the widget of the KPart belonging to the plugin is raised.
Definition at line 283 of file plugin.cpp.
void Plugin::setDisabled | ( | bool | value | ) |
Sets whether the plugin shall be disabled.
Definition at line 400 of file plugin.cpp.
void Plugin::setExecutableName | ( | const QString & | name | ) |
Sets the name
of executable (if existent).
Definition at line 126 of file plugin.cpp.
void Plugin::setIcon | ( | const QString & | icon | ) |
Sets the icon
name that is used for the plugin.
Definition at line 116 of file plugin.cpp.
void Plugin::setIdentifier | ( | const QString & | identifier | ) |
Sets the identifier
of the plugin.
Definition at line 96 of file plugin.cpp.
void Plugin::setPartLibraryName | ( | const QByteArray & | name | ) |
Set name
of library which contains the KPart used by this plugin.
Definition at line 136 of file plugin.cpp.
void Plugin::setShowInSideBar | ( | bool | hasPart | ) |
Set if the plugin provides a part that should be shown in the sidebar.
- Parameters
-
hasPart shows part in sidebar if set as true
Definition at line 390 of file plugin.cpp.
void Plugin::setTitle | ( | const QString & | title | ) |
Sets the localized title
of the plugin.
Definition at line 106 of file plugin.cpp.
|
virtual |
- Since
- 4.13
Definition at line 410 of file plugin.cpp.
|
virtual |
Returns whether the plugin provides a part that should be shown in the sidebar.
Definition at line 385 of file plugin.cpp.
|
slot |
usage
This slot is called whenever the configuration has been changed.
Definition at line 362 of file plugin.cpp.
QList< KAction * > Plugin::syncActions | ( | ) | const |
Returns the list of custom "Sync" actions.
Definition at line 241 of file plugin.cpp.
|
virtual |
Reimplement this method and return the a path relative to "data" to the tips file.
The tips file contains hints/tips that are displayed at the beginning of the program as "tip of the day". It has nothing to do with tooltips.
Definition at line 203 of file plugin.cpp.
QString Plugin::title | ( | ) | const |
Returns the localized title of the plugin.
Definition at line 111 of file plugin.cpp.
|
protectedvirtual |
Virtual hook for BC extension.
Definition at line 414 of file plugin.cpp.
|
virtual |
Return the weight of the plugin.
The higher the weight the lower it will be displayed in the sidebar. The default implementation returns 0.
Definition at line 221 of file plugin.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:37:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.