kpimidentities
#include <identitymanager.h>
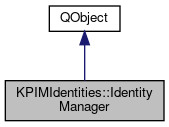
Public Types | |
typedef QList< Identity > ::ConstIterator | ConstIterator |
typedef QList< Identity >::Iterator | Iterator |
Signals | |
void | added (const KPIMIdentities::Identity &ident) |
void | changed () |
void | changed (uint uoid) |
void | changed (const KPIMIdentities::Identity &ident) |
void | deleted (uint uoid) |
void | identitiesChanged (const QString &id) |
Public Member Functions | |
IdentityManager (bool readonly=false, QObject *parent=0, const char *name=0) | |
QStringList | allEmails () const |
ConstIterator | begin () const |
void | commit () |
const Identity & | defaultIdentity () const |
ConstIterator | end () const |
bool | hasPendingChanges () const |
QStringList | identities () const |
const Identity & | identityForAddress (const QString &addresses) const |
const Identity & | identityForUoid (uint uoid) const |
const Identity & | identityForUoidOrDefault (uint uoid) const |
bool | isUnique (const QString &name) const |
QString | makeUnique (const QString &name) const |
Iterator | modifyBegin () |
Iterator | modifyEnd () |
Identity & | modifyIdentityForName (const QString &identityName) |
Identity & | modifyIdentityForUoid (uint uoid) |
Identity & | newFromControlCenter (const QString &name) |
Identity & | newFromExisting (const Identity &other, const QString &name=QString()) |
Identity & | newFromScratch (const QString &name) |
bool | removeIdentity (const QString &identityName) |
bool | removeIdentityForced (const QString &identityName) |
void | rollback () |
bool | setAsDefault (uint uoid) |
QStringList | shadowIdentities () const |
void | sort () |
bool | thatIsMe (const QString &addressList) const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Slots | |
void | slotRollback () |
Protected Member Functions | |
virtual void | createDefaultIdentity (QString &, QString &) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
QList< Identity > | mIdentities |
QList< Identity > | mShadowIdentities |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Manages the list of identities.
Definition at line 39 of file identitymanager.h.
Constructor & Destructor Documentation
|
explicit |
Create an identity manager, which loads the emailidentities file to create identities.
- Parameters
-
readonly if true, no changes can be made to the identity manager This means in particular that if there is no identity configured, the default identity created here will not be saved. It is assumed that a minimum of one identity is always present.
Definition at line 61 of file identitymanager.cpp.
Member Function Documentation
|
signal |
Emitted on commit() for each new identity.
QStringList KPIMIdentities::IdentityManager::allEmails | ( | ) | const |
Returns the list of all email addresses (only name) from all identities.
Definition at line 638 of file identitymanager.cpp.
|
signal |
Emitted whenever a commit changes any configure option.
|
signal |
Emitted whenever the identity with Unique Object Identifier (UOID) uoid
changed.
Useful for more fine-grained change notifications than what is possible with the standard changed() signal.
|
signal |
Emitted whenever the identity ident
changed.
Useful for more fine-grained change notifications than what is possible with the standard changed() signal.
void IdentityManager::commit | ( | ) |
Commit changes to disk and emit changed() if necessary.
Definition at line 161 of file identitymanager.cpp.
|
inlineprotectedvirtual |
This is called when no identity has been defined, so we need to create a default one.
The parameters are filled with some default values from KUser, but reimplementations of this method can give them another value.
Definition at line 216 of file identitymanager.h.
const Identity & IdentityManager::defaultIdentity | ( | ) | const |
- Returns
- the default identity
Definition at line 428 of file identitymanager.cpp.
|
signal |
Emitted on commit() for each deleted identity.
At the time this signal is emitted, the identity does still exist and can be retrieved by identityForUoid() if needed
bool IdentityManager::hasPendingChanges | ( | ) | const |
Check whether there are any unsaved changes.
Definition at line 230 of file identitymanager.cpp.
QStringList IdentityManager::identities | ( | ) | const |
- Returns
- the list of identities
Definition at line 235 of file identitymanager.cpp.
- Returns
- an identity whose address matches any in
addresses
or Identity::null if no such identity exists.
- Parameters
-
addresses the string of addresses to scan for matches
Definition at line 379 of file identitymanager.cpp.
const Identity & IdentityManager::identityForUoid | ( | uint | uoid | ) | const |
- Returns
- the identity with Unique Object Identifier (UOID)
uoid
or Identity::null if not found.
- Parameters
-
uoid the Unique Object Identifier to find identity with
Definition at line 359 of file identitymanager.cpp.
const Identity & IdentityManager::identityForUoidOrDefault | ( | uint | uoid | ) | const |
Convenience menthod.
- Returns
- the identity with Unique Object Identifier (UOID)
uoid
or the default identity if not found.
- Parameters
-
uoid the Unique Object Identifier to find identity with
Definition at line 369 of file identitymanager.cpp.
bool IdentityManager::isUnique | ( | const QString & | name | ) | const |
- Returns
- whether the
name
is unique
- Parameters
-
name the name to be examined
Definition at line 156 of file identitymanager.cpp.
- Returns
- a unique name for a new identity based on
name
- Parameters
-
name the name of the base identity
Definition at line 143 of file identitymanager.cpp.
IdentityManager::Iterator IdentityManager::modifyBegin | ( | ) |
Iterator used by the configuration dialog, which works on a separate list of identities, for modification.
Changes are made effective by commit().
Definition at line 349 of file identitymanager.cpp.
- Returns
- the identity named
identityName
. This method returns a reference to the identity that can be modified. To let others see this change, use commit.
- Parameters
-
identityName the identity name to return modifiable reference
Definition at line 400 of file identitymanager.cpp.
Identity & IdentityManager::modifyIdentityForUoid | ( | uint | uoid | ) |
- Returns
- the identity with Unique Object Identifier (UOID)
uoid
. This method returns a reference to the identity that can be modified. To let others see this change, use commit.
Definition at line 414 of file identitymanager.cpp.
bool IdentityManager::removeIdentity | ( | const QString & | identityName | ) |
Removes the identity with name identityName
Will return false if the identity is not found, or when one tries to remove the last identity.
- Parameters
-
identityName the identity to remove
Definition at line 470 of file identitymanager.cpp.
bool IdentityManager::removeIdentityForced | ( | const QString & | identityName | ) |
Removes the identity with name identityName
Will return false
if the identity is not found, true
otherwise.
- Note
- In opposite to removeIdentity, this method allows to remove the last remaining identity.
- Since
- 4.6
Definition at line 489 of file identitymanager.cpp.
void IdentityManager::rollback | ( | ) |
Re-read the config from disk and forget changes.
Definition at line 225 of file identitymanager.cpp.
bool IdentityManager::setAsDefault | ( | uint | uoid | ) |
Sets the identity with Unique Object Identifier (UOID) uoid
to be new the default identity.
As usual, use commit to make this permanent.
- Parameters
-
uoid the default identity to set
- Returns
- false if an identity with UOID
uoid
was not found
Definition at line 444 of file identitymanager.cpp.
QStringList IdentityManager::shadowIdentities | ( | ) | const |
Convenience method.
- Returns
- the list of (shadow) identities, ie. the ones currently under configuration.
Definition at line 246 of file identitymanager.cpp.
void IdentityManager::sort | ( | ) |
Sort the identities by name (the default is always first).
This operates on the shadow list, so you need to commit for the changes to take effect.
Definition at line 257 of file identitymanager.cpp.
bool IdentityManager::thatIsMe | ( | const QString & | addressList | ) | const |
- Returns
- true if
addressList
contains any of our addresses, false otherwise.
- Parameters
-
addressList the addressList to examine
- See also
- identityForAddress
Definition at line 395 of file identitymanager.cpp.
Member Data Documentation
The list that will be seen by everyone.
Definition at line 224 of file identitymanager.h.
The list that will be seen by the config dialog.
Definition at line 226 of file identitymanager.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:46 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.