KXMLRPC Client Library
#include <client.h>
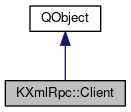
Public Slots | |
void | call (const QString &method, const QList< QVariant > &args, QObject *msgObj, const char *messageSlot, QObject *faultObj, const char *faultSlot, const QVariant &id=QVariant()) |
void | call (const QString &method, const QVariant &arg, QObject *msgObj, const char *messageSlot, QObject *faultObj, const char *faultSlot, const QVariant &id=QVariant()) |
void | call (const QString &method, int arg, QObject *msgObj, const char *messageSlot, QObject *faultObj, const char *faultSlot, const QVariant &id=QVariant()) |
void | call (const QString &method, bool arg, QObject *msgObj, const char *messageSlot, QObject *faultObj, const char *faultSlot, const QVariant &id=QVariant()) |
void | call (const QString &method, double arg, QObject *msgObj, const char *messageSlot, QObject *faultObj, const char *faultSlot, const QVariant &id=QVariant()) |
void | call (const QString &method, const QString &arg, QObject *msgObj, const char *messageSlot, QObject *faultObj, const char *faultSlot, const QVariant &id=QVariant()) |
void | call (const QString &method, const QByteArray &arg, QObject *msgObj, const char *messageSlot, QObject *faultObj, const char *faultSlot, const QVariant &id=QVariant()) |
void | call (const QString &method, const QDateTime &arg, QObject *msgObj, const char *messageSlot, QObject *faultObj, const char *faultSlot, const QVariant &id=QVariant()) |
void | call (const QString &method, const QStringList &arg, QObject *msgObj, const char *messageSlot, QObject *faultObj, const char *faultSlot, const QVariant &id=QVariant()) |
Public Member Functions | |
Client (QObject *parent=0) | |
Client (const KUrl &url, QObject *parent=0) | |
~Client () | |
bool | isDigestAuthEnabled () const |
void | setDigestAuthEnabled (bool enabled) |
void | setUrl (const KUrl &url) |
void | setUserAgent (const QString &userAgent) |
KUrl | url () const |
QString | userAgent () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
A class that represents a connection to a XML-RPC server.
This is the main interface to the XML-RPC client library.
Constructor & Destructor Documentation
|
explicit |
Constructs a XML-RPC Client.
- Parameters
-
parent the parent of this object, defaults to NULL.
Definition at line 53 of file client.cpp.
|
explicit |
Constructs a XML-RPC Client, which will connect to url
.
- Parameters
-
url the url of the xml-rpc server. parent the parent of this object, defaults to NULL.
Definition at line 58 of file client.cpp.
Client::~Client | ( | ) |
Destroys the XML-RPC Client.
Definition at line 64 of file client.cpp.
Member Function Documentation
|
slot |
Calls the given method on a XML-RPC server, with the given argument list.
- Parameters
-
method the method on the server we are going to be calling args the argument list to pass to the server msgObj the object containing the data slot messageSlot the data slot itself faultObj the object containing the error slot faultSlot the error slot itself id the id for our Client object, defaults to empty
Definition at line 106 of file client.cpp.
|
slot |
Calls the given method on a XML-RPC server, with the given argument.
- Parameters
-
method the method on the server we are going to be calling arg the argument to pass to the server msgObj the object containing the data slot messageSlot the data slot itself faultObj the object containing the error slot faultSlot the error slot itself id the id for our Client object, defaults to empty
Definition at line 137 of file client.cpp.
|
slot |
Calls the given method on a XML-RPC server, with the given int as the argument.
- Parameters
-
method the method on the server we are going to be calling arg the int to pass to the server msgObj the object containing the data slot messageSlot the data slot itself faultObj the object containing the error slot faultSlot the error slot itself id the id for our Client object, defaults to empty
Definition at line 147 of file client.cpp.
|
slot |
Calls the given method on a XML-RPC server, with the given bool as the argument.
- Parameters
-
method the method on the server we are going to be calling arg the bool to pass to the server msgObj the object containing the data slot messageSlot the data slot itself faultObj the object containing the error slot faultSlot the error slot itself id the id for our Client object, defaults to empty
Definition at line 157 of file client.cpp.
|
slot |
Calls the given method on a XML-RPC server, with the given double as the argument.
- Parameters
-
method the method on the server we are going to be calling arg the double to pass to the server msgObj the object containing the data slot messageSlot the data slot itself faultObj the object containing the error slot faultSlot the error slot itself id the id for our Client object, defaults to empty
Definition at line 167 of file client.cpp.
|
slot |
Calls the given method on a XML-RPC server, with the given string as the argument.
- Parameters
-
method the method on the server we are going to be calling arg the string to pass to the server msgObj the object containing the data slot messageSlot the data slot itself faultObj the object containing the error slot faultSlot the error slot itself id the id for our Client object, defaults to empty
Definition at line 177 of file client.cpp.
|
slot |
Calls the given method on a XML-RPC server, with the given byte array as the argument.
- Parameters
-
method the method on the server we are going to be calling arg the array to pass to the server msgObj the object containing the data slot messageSlot the data slot itself faultObj the object containing the error slot faultSlot the error slot itself id the id for our Client object, defaults to empty
Definition at line 187 of file client.cpp.
|
slot |
Calls the given method on a XML-RPC server, with the given date as the argument.
- Parameters
-
method the method on the server we are going to be calling arg the date and/or time to pass to the server msgObj the object containing the data slot messageSlot the data slot itself faultObj the object containing the error slot faultSlot the error slot itself id the id for our Client object, defaults to empty
Definition at line 197 of file client.cpp.
|
slot |
Calls the given method on a XML-RPC server, with the given string list as the argument.
- Parameters
-
method the method on the server we are going to be calling arg the list of strings to pass to the server msgObj the object containing the data slot messageSlot the data slot itself faultObj the object containing the error slot faultSlot the error slot itself id the id for our Client object, defaults to empty
Definition at line 207 of file client.cpp.
bool Client::isDigestAuthEnabled | ( | ) | const |
Returns true if HTTP-Digest authentication is enabled, false if not.
- See also
- setDigestAuthEnabled()
Definition at line 96 of file client.cpp.
void Client::setDigestAuthEnabled | ( | bool | enabled | ) |
Enables/disables HTTP-Digest authentication.
- See also
- isDigestAuthEnabled()
Definition at line 101 of file client.cpp.
void Client::setUrl | ( | const KUrl & | url | ) |
Sets the url the Client will connect to.
- Parameters
-
url the url for the xml-rpc server we will be connecting to.
- See also
- url()
Definition at line 76 of file client.cpp.
void Client::setUserAgent | ( | const QString & | userAgent | ) |
Sets the userAgent string the Client will use to identify itself.
- Parameters
-
userAgent the user agent string to use.
- See also
- userAgent()
Definition at line 91 of file client.cpp.
KUrl Client::url | ( | ) | const |
Returns the current url the XML-RPC Client will connect to.
- See also
- setUrl()
Definition at line 81 of file client.cpp.
QString Client::userAgent | ( | ) | const |
Returns the user agent string currently used by the Client.
- See also
- setUserAgent()
Definition at line 86 of file client.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:37:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.