mailtransport
#include <transportmanager.h>
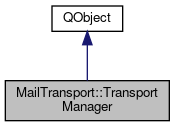
Public Types | |
enum | ShowCondition { Always, IfNoTransportExists } |
Public Slots | |
Q_SCRIPTABLE int | defaultTransportId () const |
Q_SCRIPTABLE QString | defaultTransportName () const |
Q_SCRIPTABLE bool | isEmpty () const |
Q_SCRIPTABLE void | removeTransport (int id) |
Q_SCRIPTABLE void | setDefaultTransport (int id) |
Q_SCRIPTABLE QList< int > | transportIds () const |
Q_SCRIPTABLE QStringList | transportNames () const |
Signals | |
Q_SCRIPTABLE void | changesCommitted () |
void | passwordsChanged () |
void | transportRemoved (int id, const QString &name) |
void | transportRenamed (int id, const QString &oldName, const QString &newName) |
Q_SCRIPTABLE void | transportsChanged () |
Public Member Functions | |
virtual | ~TransportManager () |
void | addTransport (Transport *transport) |
bool | configureTransport (Transport *transport, QWidget *parent) |
void | createDefaultTransport () |
Transport * | createTransport () const |
MAILTRANSPORT_DEPRECATED TransportJob * | createTransportJob (int transportId) |
MAILTRANSPORT_DEPRECATED TransportJob * | createTransportJob (const QString &transport) |
void | loadPasswordsAsync () |
MAILTRANSPORT_DEPRECATED void | schedule (TransportJob *job) |
bool | showTransportCreationDialog (QWidget *parent, ShowCondition showCondition=Always) |
Transport * | transportById (int id, bool def=true) const |
Transport * | transportByName (const QString &name, bool def=true) const |
QList< Transport * > | transports () const |
TransportType::List | types () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static TransportManager * | self () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
TransportManager () | |
void | loadPasswords () |
KWallet::Wallet * | wallet () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Central transport management interface.
This class manages the creation, configuration, and removal of mail transports, as well as the loading and storing of mail transport settings.
It also handles the creation of transport jobs, although that behaviour is deprecated and you are encouraged to use MessageQueueJob.
- See also
- MessageQueueJob.
Definition at line 54 of file transportmanager.h.
Member Enumeration Documentation
Describes when to show the transport creation dialog.
Definition at line 167 of file transportmanager.h.
Constructor & Destructor Documentation
|
virtual |
Destructor.
Definition at line 156 of file transportmanager.cpp.
|
protected |
Singleton class, the only instance resides in the static object sSelf.
Definition at line 123 of file transportmanager.cpp.
Member Function Documentation
void TransportManager::addTransport | ( | Transport * | transport | ) |
Adds the given transport.
The object ownership is transferred to TransportMananger, ie. you must not delete transport
.
- Parameters
-
transport The Transport object to add.
Definition at line 216 of file transportmanager.cpp.
|
signal |
Internal signal to synchronize all TransportManager instances.
This signal is emitted by the instance writing the changes. You probably want to use transportsChanged() instead.
Open a configuration dialog for an existing transport.
- Parameters
-
transport The transport to configure. It can be a new transport, or one already managed by TransportManager. parent The parent widget for the dialog.
- Returns
- True if the user clicked Ok, false if the user cancelled.
- Since
- 4.4
Definition at line 282 of file transportmanager.cpp.
void TransportManager::createDefaultTransport | ( | ) |
Tries to create a transport based on KEMailSettings.
If the data in KEMailSettings is incomplete, no transport is created.
Definition at line 244 of file transportmanager.cpp.
Transport * TransportManager::createTransport | ( | ) | const |
Creates a new, empty Transport object.
The object is owned by the caller. If you want to add the Transport permanently (eg. after configuring it) call addTransport().
Definition at line 208 of file transportmanager.cpp.
TransportJob * TransportManager::createTransportJob | ( | int | transportId | ) |
Creates a mail transport job for the given transport identifier.
Returns 0 if the specified transport is invalid.
- Parameters
-
transportId The transport identifier.
- Deprecated:
- use MessageQueueJob to queue messages and rely on the Dispatcher Agent to send them.
Definition at line 303 of file transportmanager.cpp.
TransportJob * TransportManager::createTransportJob | ( | const QString & | transport | ) |
Creates a mail transport job for the given transport identifer, or transport name.
Returns 0 if the specified transport is invalid.
- Parameters
-
transport A string defining a mail transport.
- Deprecated:
- use MessageQueueJob to queue messages and rely on the Dispatcher Agent to send them.
Definition at line 323 of file transportmanager.cpp.
|
slot |
Returns the default transport identifier.
Invalid if there are no transports at all.
Definition at line 376 of file transportmanager.cpp.
|
slot |
Returns the default transport name.
Definition at line 367 of file transportmanager.cpp.
|
slot |
Returns true if there are no mail transports at all.
Definition at line 344 of file transportmanager.cpp.
|
protected |
Loads all passwords synchronously.
Definition at line 614 of file transportmanager.cpp.
void TransportManager::loadPasswordsAsync | ( | ) |
Tries to load passwords asynchronously from KWallet if needed.
The passwordsChanged() signal is emitted once the passwords have been loaded. Nothing happens if the passwords were already available.
Definition at line 630 of file transportmanager.cpp.
|
signal |
Emitted when passwords have been loaded from the wallet.
If you made a deep copy of a transport, you should call updatePasswordState() for the cloned transport to ensure its password is updated as well.
|
slot |
Deletes the specified transport.
- Parameters
-
id The identifier of the mail transport to remove.
Definition at line 390 of file transportmanager.cpp.
void TransportManager::schedule | ( | TransportJob * | job | ) |
Executes the given transport job.
This is the preferred way to start transport jobs. It takes care of asynchronously loading passwords from KWallet if necessary.
- Parameters
-
job The completely configured transport job to execute.
- Deprecated:
- use MessageQueueJob to queue messages and rely on the Dispatcher Agent to send them.
Definition at line 229 of file transportmanager.cpp.
|
static |
Returns the TransportManager instance.
Definition at line 162 of file transportmanager.cpp.
|
slot |
Sets the default transport.
The change will be in effect immediately.
- Parameters
-
id The identifier of the new default transport.
Definition at line 381 of file transportmanager.cpp.
bool TransportManager::showTransportCreationDialog | ( | QWidget * | parent, |
ShowCondition | showCondition = Always |
||
) |
Shows a dialog for creating and configuring a new transport.
- Parameters
-
parent Parent widget of the dialog. showCondition the condition under which the dialog is shown at all
- Returns
- True if a new transport has been created and configured.
- Since
- 4.4
Definition at line 258 of file transportmanager.cpp.
Transport * TransportManager::transportById | ( | int | id, |
bool | def = true |
||
) | const |
Returns the Transport object with the given id.
- Parameters
-
id The identifier of the Transport. def if set to true, the default transport will be returned if the specified Transport object could not be found, 0 otherwise.
- Returns
- A Transport object for immediate use. It might become invalid as soon as the event loop is entered again due to remote changes. If you need to store a Transport object, store the transport identifier instead.
Definition at line 171 of file transportmanager.cpp.
Returns the transport object with the given name.
- Parameters
-
name The transport name. def if set to true, the default transport will be returned if the specified Transport object could not be found, 0 otherwise.
- Returns
- A Transport object for immediate use, see transportById() for limitations.
Definition at line 185 of file transportmanager.cpp.
|
slot |
Returns a list of transport identifiers.
Definition at line 349 of file transportmanager.cpp.
|
slot |
Returns a list of transport names.
Definition at line 358 of file transportmanager.cpp.
|
signal |
Emitted when a transport is deleted.
- Parameters
-
id The identifier of the deleted transport. name The name of the deleted transport.
|
signal |
Emitted when a transport has been renamed.
- Parameters
-
id The identifier of the renamed transport. oldName The old name. newName The new name.
Returns a list of all available transports.
Note: The Transport objects become invalid as soon as a change occur, so they are only suitable for immediate use.
Definition at line 198 of file transportmanager.cpp.
|
signal |
Emitted when transport settings have changed (by this or any other TransportManager instance).
TransportType::List TransportManager::types | ( | ) | const |
Returns a list of all available transport types.
Definition at line 203 of file transportmanager.cpp.
|
protected |
Returns a pointer to an open wallet if available, 0 otherwise.
The wallet is opened synchronously if necessary.
Definition at line 574 of file transportmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:37:49 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.