lokalize
#include <catalog.h>
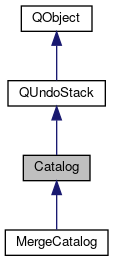
Signals | |
void | activePhaseChanged () |
void | signalEntryModified (const DocPosition &) |
void | signalFileAutoSaveFailed (const QString &) |
Q_SCRIPTABLE void | signalFileLoaded () |
void | signalFileLoaded (const KUrl &) |
Q_SCRIPTABLE void | signalFileSaved () |
void | signalFileSaved (const KUrl &) |
void | signalNumberOfEmptyChanged () |
void | signalNumberOfFuzziesChanged () |
Public Member Functions | |
Catalog (QObject *parent) | |
virtual | ~Catalog () |
void | attachAltTrans (int entry, const AltTrans &trans) |
void | attachAltTransCatalog (Catalog *) |
bool | autoSaveRecovered () |
int | capabilities () const |
void | clear () |
int | firstBookmarkIndex () const |
int | firstFuzzyIndex () const |
int | firstUntranslatedIndex () const |
bool | isEmpty () |
bool | isReadOnly () |
int | lastBookmarkIndex () const |
int | lastFuzzyIndex () const |
int | lastUntranslatedIndex () const |
QString | msgid (const DocPosition &) const |
virtual QString | msgstr (const DocPosition &) const |
int | nextBookmarkIndex (uint index) const |
int | nextFuzzyIndex (uint index) const |
int | nextUntranslatedIndex (uint index) const |
int | prevBookmarkIndex (uint index) const |
int | prevFuzzyIndex (uint index) const |
int | prevUntranslatedIndex (uint index) const |
void | push (QUndoCommand *cmd) |
virtual const DocPosition & | redo () |
void | setTarget (DocPosition pos, const CatalogString &s) |
void | setUrl (const KUrl &u) |
virtual const DocPosition & | undo () |
![]() | |
QUndoStack (QObject *parent) | |
~QUndoStack () | |
void | beginMacro (const QString &text) |
bool | canRedo () const |
void | canRedoChanged (bool canRedo) |
bool | canUndo () const |
void | canUndoChanged (bool canUndo) |
void | cleanChanged (bool clean) |
int | cleanIndex () const |
void | clear () |
const QUndoCommand * | command (int index) const |
int | count () const |
QAction * | createRedoAction (QObject *parent, const QString &prefix) const |
QAction * | createUndoAction (QObject *parent, const QString &prefix) const |
void | endMacro () |
int | index () const |
void | indexChanged (int idx) |
bool | isActive () const |
bool | isClean () const |
void | push (QUndoCommand *cmd) |
void | redo () |
QString | redoText () const |
void | redoTextChanged (const QString &redoText) |
void | setActive (bool active) |
void | setClean () |
void | setIndex (int idx) |
void | setUndoLimit (int limit) |
QString | text (int idx) const |
void | undo () |
int | undoLimit () const |
QString | undoText () const |
void | undoTextChanged (const QString &undoText) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static bool | extIsSupported (const QString &path) |
static const char *const * | states () |
static QStringList | supportedExtensions () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Static Public Attributes | |
static QString | supportedMimeFilters |
Protected Slots | |
void | doAutoSave () |
void | flushUpdateDBBuffer () |
void | projectConfigChanged () |
void | setAutoSaveDirty () |
Protected Member Functions | |
virtual KAutoSaveFile * | checkAutoSave (const KUrl &url) |
void | setApproved (const DocPosition &pos, bool approved) |
void | setEquivTrans (const DocPosition &, bool equivTrans) |
void | setLastModifiedPos (const DocPosition &) |
bool | setModified (DocPos entry, bool modif) |
TargetState | setState (const DocPosition &pos, TargetState state) |
void | targetDelete (const DocPosition &pos, int count) |
InlineTag | targetDeleteTag (const DocPosition &pos) |
void | targetInsert (const DocPosition &pos, const QString &arg) |
void | targetInsertTag (const DocPosition &pos, const InlineTag &tag) |
void | updateApprovedEmptyIndexCache () |
Phase | updatePhase (const Phase &phase) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
CatalogPrivate * | d |
CatalogStorage * | m_storage |
Additional Inherited Members | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
active | |
undoLimit | |
![]() | |
objectName | |
Detailed Description
This class represents a catalog It uses CatalogStorage interface to work with catalogs in different formats Also it defines all necessary functions to set and get the entries.
Wrapper class that represents a translation catalog
Constructor & Destructor Documentation
Catalog::Catalog | ( | QObject * | parent | ) |
Definition at line 102 of file catalog.cpp.
|
virtual |
Definition at line 122 of file catalog.cpp.
Member Function Documentation
|
signal |
|
inlineslot |
Definition at line 385 of file catalog.cpp.
Definition at line 400 of file catalog.cpp.
|
slot |
Definition at line 272 of file catalog.cpp.
void Catalog::attachAltTrans | ( | int | entry, |
const AltTrans & | trans | ||
) |
Definition at line 267 of file catalog.cpp.
void Catalog::attachAltTransCatalog | ( | Catalog * | altCat | ) |
Definition at line 260 of file catalog.cpp.
|
slot |
so DocPosition::entry may actually be < size()+binUnitsCount()
Definition at line 448 of file catalog.cpp.
int Catalog::capabilities | ( | ) | const |
Definition at line 164 of file catalog.cpp.
|
slot |
Definition at line 219 of file catalog.cpp.
|
protectedvirtual |
Definition at line 489 of file catalog.cpp.
void Catalog::clear | ( | ) |
Definition at line 130 of file catalog.cpp.
|
slot |
Definition at line 703 of file catalog.cpp.
|
slot |
Definition at line 322 of file catalog.cpp.
|
slot |
Definition at line 236 of file catalog.cpp.
|
protectedslot |
Definition at line 681 of file catalog.cpp.
|
static |
Definition at line 93 of file catalog.cpp.
|
protectedslot |
updates DB for _posBuffer and accompanying _originalForLastModified
Definition at line 752 of file catalog.cpp.
|
slot |
Definition at line 314 of file catalog.cpp.
|
slot |
Definition at line 410 of file catalog.cpp.
|
inlineslot |
|
slot |
- Returns
- true if at least one form is untranslated
Definition at line 432 of file catalog.cpp.
|
slot |
Definition at line 437 of file catalog.cpp.
|
slot |
Definition at line 443 of file catalog.cpp.
|
slot |
Definition at line 928 of file catalog.cpp.
|
slot |
Definition at line 933 of file catalog.cpp.
|
slot |
Definition at line 1015 of file catalog.cpp.
|
slot |
Definition at line 405 of file catalog.cpp.
|
inlineslot |
|
slot |
- Returns
- 0 if success, >0 erroneous line (parsing error)
Definition at line 508 of file catalog.cpp.
|
slot |
Definition at line 458 of file catalog.cpp.
QString Catalog::msgid | ( | const DocPosition & | pos | ) | const |
Definition at line 187 of file catalog.cpp.
|
virtual |
Reimplemented in MergeCatalog.
Definition at line 195 of file catalog.cpp.
|
inline |
|
slot |
Definition at line 252 of file catalog.cpp.
|
slot |
Definition at line 228 of file catalog.cpp.
|
slot |
Definition at line 171 of file catalog.cpp.
|
slot |
Definition at line 372 of file catalog.cpp.
Definition at line 380 of file catalog.cpp.
Definition at line 390 of file catalog.cpp.
|
inline |
|
protectedslot |
Definition at line 698 of file catalog.cpp.
void Catalog::push | ( | QUndoCommand * | cmd | ) |
Definition at line 155 of file catalog.cpp.
|
virtual |
Definition at line 746 of file catalog.cpp.
|
slot |
Definition at line 609 of file catalog.cpp.
|
slot |
Definition at line 615 of file catalog.cpp.
|
slot |
Definition at line 339 of file catalog.cpp.
|
protected |
(EDITING) accessed from undo/redo code accessed from mergeCatalog) it does check if action should be taken
|
slot |
Definition at line 984 of file catalog.cpp.
|
protected |
Definition at line 910 of file catalog.cpp.
|
protected |
(EDITING) accessed from undo/redo code called BEFORE modification
Definition at line 783 of file catalog.cpp.
|
protected |
- Returns
- true if entry wasn't modified before
Definition at line 915 of file catalog.cpp.
|
slot |
pos.form is note number
- Returns
- previous note contents, if any
Definition at line 244 of file catalog.cpp.
|
slot |
- Returns
- previous phase-name
Definition at line 330 of file catalog.cpp.
- pos.entry - number of phase,
- pos.form - number of note
Definition at line 395 of file catalog.cpp.
|
protected |
Definition at line 872 of file catalog.cpp.
void Catalog::setTarget | ( | DocPosition | pos, |
const CatalogString & | s | ||
) |
Definition at line 866 of file catalog.cpp.
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
inlineslot |
|
slot |
Definition at line 306 of file catalog.cpp.
|
slot |
Definition at line 466 of file catalog.cpp.
|
slot |
Definition at line 203 of file catalog.cpp.
|
slot |
Definition at line 421 of file catalog.cpp.
|
static |
Definition at line 79 of file catalog.cpp.
|
static |
Definition at line 84 of file catalog.cpp.
|
inlineslot |
|
protected |
Definition at line 805 of file catalog.cpp.
|
protected |
Definition at line 852 of file catalog.cpp.
|
protected |
Definition at line 828 of file catalog.cpp.
|
protected |
Definition at line 840 of file catalog.cpp.
|
slot |
Definition at line 474 of file catalog.cpp.
|
slot |
Definition at line 211 of file catalog.cpp.
|
virtual |
Definition at line 740 of file catalog.cpp.
|
slot |
Definition at line 453 of file catalog.cpp.
|
protected |
Definition at line 347 of file catalog.cpp.
Definition at line 905 of file catalog.cpp.
Member Data Documentation
|
protected |
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:40:07 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.