umbrello/umbrello
#include <layoutgenerator.h>
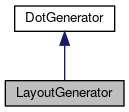
Public Types | |
typedef QList< QPointF > | EdgePoints |
typedef QHash< QString, EdgePoints > | EdgeType |
typedef QHash< QString, QRectF > | NodeType |
typedef QMap< QString, QStringList > | ParameterList |
Public Member Functions | |
LayoutGenerator () | |
bool | apply (UMLScene *scene) |
bool | generate (UMLScene *scene, const QString &variant=QString()) |
bool | isEnabled () |
QPointF | mapToScene (const QPointF &p) |
QPoint | origin (const QString &id) |
bool | parseLine (const QString &line) |
bool | readGeneratedDotFile (const QString &fileName) |
bool | splitParameters (QMap< QString, QStringList > &map, const QString &s) |
![]() | |
DotGenerator () | |
bool | createDotFile (UMLScene *scene, const QString &fileName, const QString &variant=QLatin1String("default")) |
bool | readConfigFile (QString diagramType, const QString &variant=QLatin1String("default")) |
void | setUseFullNodeLabels (bool state) |
void | setUsePosition (bool state) |
bool | useFullNodeLabels () |
bool | usePosition () |
Static Public Member Functions | |
static bool | availableConfigFiles (UMLScene *scene, QHash< QString, QString > &configFiles) |
static QString | currentDotPath () |
![]() | |
static bool | availableConfigFiles (UMLScene *scene, QHash< QString, QString > &configFiles) |
Protected Attributes | |
QRectF | m_boundingRect |
QString | m_dotPath |
QHash< QString, QPointF > | m_edgeLabelPosition |
EdgeType | m_edges |
NodeType | m_nodes |
![]() | |
QString | m_configFileName |
QHash< QString, QString > | m_dotParameters |
QHash< QString, QString > | m_edgeParameters |
QString | m_generator |
QHash< QString, QString > | m_nodeParameters |
QPointF | m_origin |
double | m_scale |
bool | m_useFullNodeLabels |
bool | m_usePosition |
Friends | |
QDebug | operator<< (QDebug out, LayoutGenerator &c) |
Additional Inherited Members | |
![]() | |
bool | findItem (QStringList ¶ms, const QString &search) |
QString | fixID (const QString &_id) |
Detailed Description
The class LayoutGenerator provides calculated layouts of diagrams.
It uses the dot executable from the graphviz package for calculation of widget positions.
The implementation calls dot with information from the displayed widgets and associations by creating a temporary dot file based on a layout configure file, which is located in the umbrello/layouts subdir of the "data" resource type. The config file is determined from the type of the currently displayed diagram and the layout chosen by the user.
Dot creates a file containing the calculated widget positions. The widget positions are retrieved from this file and used to move widgets on the provided diagram. Additional points in association lines are removed.
Definition at line 38 of file layoutgenerator.h.
Member Typedef Documentation
Definition at line 42 of file layoutgenerator.h.
Definition at line 43 of file layoutgenerator.h.
Definition at line 41 of file layoutgenerator.h.
Definition at line 44 of file layoutgenerator.h.
Constructor & Destructor Documentation
LayoutGenerator::LayoutGenerator | ( | ) |
constructor
Definition at line 72 of file layoutgenerator.cpp.
Member Function Documentation
bool LayoutGenerator::apply | ( | UMLScene * | scene | ) |
apply auto layout to the given scene
- Parameters
-
scene
- Returns
- true if autolayout has been applied
Definition at line 202 of file layoutgenerator.cpp.
|
static |
Return a list of available templates for a given scene type.
- Parameters
-
scene The diagram configFiles will contain the collected list of config files
- Returns
- true if collecting succeeds
Definition at line 284 of file layoutgenerator.cpp.
|
static |
Return the path where dot is installed.
- Returns
- string with dot path
Definition at line 100 of file layoutgenerator.cpp.
generate layout and apply it to the given diagram.
- Returns
- true if generating succeeded
Definition at line 129 of file layoutgenerator.cpp.
bool LayoutGenerator::isEnabled | ( | ) |
Return state if layout generator is enabled.
It is enabled when the dot application has been found.
- Returns
- true if enabled
Definition at line 83 of file layoutgenerator.cpp.
map dot coordinate to scene coordinate
- Parameters
-
p dot point to map
- Returns
- uml scene coordinate
Definition at line 567 of file layoutgenerator.cpp.
Return the origin of node based on the bottom/left corner.
- Parameters
-
id The widget id to fetch the origin from
- Returns
- QPoint instance with the coordinates
Definition at line 311 of file layoutgenerator.cpp.
bool LayoutGenerator::parseLine | ( | const QString & | line | ) |
Parse line from dot generated plain-ext output format.
The format is documented at http://graphviz.org/content/output-formats#dplain-ext and looks like:
graph 1 28.083 10.222 node ITfDmJvJE00m 8.0833 8.7361 0.86111 0.45833 QObject solid box black lightgrey edge sL4cKPpHnJkU sL4cKPpHnJkU 7 8.1253 7.2568 8.2695 7.2687 8.375 7.3127 8.375 7.3889 8.375 7.4377 8.3317 7.4733 8.2627 7.4957 Aggregation 8.8472 7.3889 solid black
- Parameters
-
line line in dot plain-ext output format
- Returns
- true if line could be parsed successfully
Definition at line 363 of file layoutgenerator.cpp.
bool LayoutGenerator::readGeneratedDotFile | ( | const QString & | fileName | ) |
Read generated dot file and extract positions of the contained widgets.
- Returns
- true if extracting succeeded
Definition at line 334 of file layoutgenerator.cpp.
bool LayoutGenerator::splitParameters | ( | QMap< QString, QStringList > & | map, |
const QString & | s | ||
) |
Friends And Related Function Documentation
|
friend |
Member Data Documentation
|
protected |
Definition at line 60 of file layoutgenerator.h.
|
protected |
contains path to dot executable
Definition at line 64 of file layoutgenerator.h.
contains global node parameters
Definition at line 63 of file layoutgenerator.h.
|
protected |
list of edges found in parsed dot file
Definition at line 62 of file layoutgenerator.h.
|
protected |
list of nodes found in parsed dot file
Definition at line 61 of file layoutgenerator.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:40:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.