kgpg
#include <kgpgimport.h>
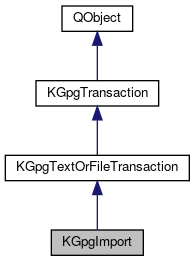
Public Member Functions | |
KGpgImport (QObject *parent, const QString &text=QString()) | |
KGpgImport (QObject *parent, const KUrl::List &files) | |
virtual | ~KGpgImport () |
QStringList | getImportedIds (const int reason=-1) const |
QStringList | getImportedKeys () const |
QString | getImportMessage () const |
![]() | |
virtual | ~KGpgTextOrFileTransaction () |
const QStringList & | getMessages () const |
void | setText (const QString &text) |
void | setUrls (const KUrl::List &files) |
![]() | |
KGpgTransaction (QObject *parent=0, const bool allowChaining=false) | |
virtual | ~KGpgTransaction () |
void | clearInputTransaction () |
const QString & | getDescription () const |
bool | hasInputTransaction () const |
void | kill () |
void | setGnuPGHome (const QString &home) |
void | setInputTransaction (KGpgTransaction *ta) |
void | start () |
int | waitForFinished (const int msecs=-1) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static QString | getDetailedImportMessage (const QStringList &log, const KGpgItemModel *model=NULL) |
static QStringList | getImportedIds (const QStringList &log, const int reason=-1) |
static QString | getImportMessage (const QStringList &log) |
static int | isKey (const QString &text, const bool incomplete=false) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
virtual QStringList | command () const |
![]() | |
KGpgTextOrFileTransaction (QObject *parent=0, const QString &text=QString(), const bool allowChaining=false) | |
KGpgTextOrFileTransaction (QObject *parent, const KUrl::List &files, const bool allowChaining=false) | |
virtual void | finish () |
const KUrl::List & | getInputFiles () const |
virtual bool | nextLine (const QString &line) |
virtual bool | preStart () |
![]() | |
int | addArgument (const QString &arg) |
void | addArgumentRef (int *ref) |
int | addArguments (const QStringList &args) |
void | addIdHint (QString txt) |
void | askNewPassphrase (const QString &text) |
bool | askPassphrase (const QString &message=QString()) |
virtual ts_boolanswer | boolQuestion (const QString &line) |
virtual ts_boolanswer | confirmOverwrite (KUrl ¤tFile) |
QString | getIdHints () const |
GPGProc * | getProcess () |
int | getSuccess () const |
virtual bool | hintLine (const ts_hintType hint, const QString &args) |
void | insertArgument (const int pos, const QString &arg) |
void | insertArguments (const int pos, const QStringList &args) |
virtual void | newPassphraseEntered () |
virtual bool | passphraseReceived () |
virtual bool | passphraseRequested () |
virtual void | postStart () |
void | replaceArgument (const int pos, const QString &arg) |
void | setDescription (const QString &description) |
void | setSuccess (const int v) |
void | unexpectedLine (const QString &line) |
void | waitForInputTransaction () |
void | write (const QByteArray &a, const bool lf=true) |
void | write (const int i) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | ts_import { TS_KIO_FAILED = TS_COMMON_END + 1 } |
![]() | |
enum | ts_boolanswer { BA_UNKNOWN = 0, BA_YES = 1, BA_NO = 2 } |
enum | ts_hintType { HT_KEYEXPIRED = 0, HT_SIGEXPIRED = 1, HT_NOSECKEY = 2, HT_ENCTO = 3 } |
enum | ts_transaction { TS_OK = 0, TS_BAD_PASSPHRASE = 1, TS_MSG_SEQUENCE = 2, TS_USER_ABORTED = 3, TS_INVALID_EMAIL = 4, TS_INPUT_PROCESS_ERROR = 5, TS_COMMON_END = 100 } |
![]() | |
void | done (int result) |
void | infoProgress (qulonglong processedAmount, qulonglong totalAmount) |
void | statusMessage (const QString &msg) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
import one or more keys into the keyring
Definition at line 31 of file kgpgimport.h.
Constructor & Destructor Documentation
import given text
- Parameters
-
parent parent object text key text to import
Definition at line 22 of file kgpgimport.cpp.
KGpgImport::KGpgImport | ( | QObject * | parent, |
const KUrl::List & | files | ||
) |
import key(s) from file(s)
- Parameters
-
parent parent object files list of file locations to import from
Definition at line 27 of file kgpgimport.cpp.
|
virtual |
destructor
Definition at line 32 of file kgpgimport.cpp.
Member Function Documentation
|
protectedvirtual |
Implements KGpgTextOrFileTransaction.
Definition at line 37 of file kgpgimport.cpp.
|
static |
get detailed summary of import
- Parameters
-
log import log
- Returns
- message describing which keys changed and how
The log must contain a "IMPORT_RES" line. If this is not present the result string will contain an error message.
Definition at line 206 of file kgpgimport.cpp.
|
static |
get the full fingerprints of the imported keys
- Parameters
-
log transaction log to scan reason key import reason
- Returns
- list of ids that were imported
You can filter the list of keys returned by the status of that key as reported by GnuPG. See doc/DETAILS of GnuPG for the meaning of the different flags.
If reason is -1 (the default) all processed key ids are returned. If reason is 0 only keys of status 0 (unchanged) are returned. For any other value a key is returned if one of his status bits matched one of the bits in reason (i.e. (reason & status) != 0).
Definition at line 59 of file kgpgimport.cpp.
QStringList KGpgImport::getImportedIds | ( | const int | reason = -1 | ) | const |
get the full fingerprints of the imported keys
This is an overloaded member. It calls the static function with the result log from this transaction object.
Definition at line 90 of file kgpgimport.cpp.
QStringList KGpgImport::getImportedKeys | ( | ) | const |
get the names and short fingerprints of the imported keys
- Returns
- list of keys that were imported
Definition at line 47 of file kgpgimport.cpp.
QString KGpgImport::getImportMessage | ( | ) | const |
get textual summary of the import events
- Returns
- messages describing what was imported
This is an overloaded member. It calls the static function with the result log from this transaction object.
Definition at line 96 of file kgpgimport.cpp.
|
static |
get textual summary of the import events
- Parameters
-
log import log
- Returns
- messages describing what was imported
The log must contain a "IMPORT_RES" line. If this is not present the result string will contain an error message.
Definition at line 102 of file kgpgimport.cpp.
|
static |
check if the given text contains a private or public key
- Parameters
-
text text to check incomplete assume text is only the beginning of the data
- Returns
- if text contains a key or not
- Return values
-
0 no key found 1 public key found 2 private key found
Definition at line 276 of file kgpgimport.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:42:08 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.