liblancelot
#include <ActionListModel.h>
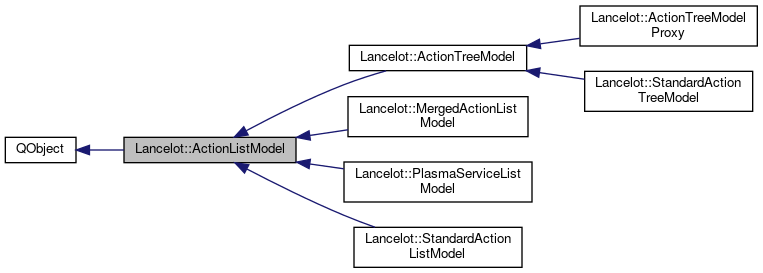
Public Slots | |
void | activated (int index) |
Signals | |
void | itemActivated (int index) |
void | itemAltered (int index) |
void | itemDeleted (int index) |
void | itemInserted (int index) |
void | updated () |
Public Member Functions | |
ActionListModel () | |
virtual | ~ActionListModel () |
virtual void | contextActivate (int index, QAction *context) |
virtual void | dataDragFinished (int index, Qt::DropAction action) |
virtual bool | dataDropAvailable (int where, const QMimeData *mimeData) |
virtual void | dataDropped (int where, const QMimeData *mimeData) |
virtual QString | description (int index) const |
virtual bool | hasContextActions (int index) const |
virtual QIcon | icon (int index) const |
virtual bool | isCategory (int index) const |
virtual QMimeData * | mimeData (int index) const |
virtual QIcon | selfIcon () const |
virtual QMimeData * | selfMimeData () const |
virtual QString | selfShortTitle () const |
virtual QString | selfTitle () const |
virtual void | setContextActions (int index, Lancelot::PopupMenu *menu) |
virtual void | setDropActions (int index, Qt::DropActions &actions, Qt::DropAction &defaultAction) |
virtual int | size () const =0 |
virtual QString | title (int index) const =0 |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
virtual void | activate (int index) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This class represents a list data model.
Definition at line 37 of file ActionListModel.h.
Constructor & Destructor Documentation
Lancelot::ActionListModel::ActionListModel | ( | ) |
Creates a new instance of ActionListModel.
Definition at line 26 of file ActionListModel.cpp.
|
virtual |
Destroys this ActionListModel.
Definition at line 30 of file ActionListModel.cpp.
Member Function Documentation
|
protectedvirtual |
Models should reimplement this function.
It is invoked when an item is activated, before the itemActivated signal is emitted
- Parameters
-
index of the item that is activated
Reimplemented in Lancelot::MergedActionListModel, Lancelot::ActionTreeModelProxy, and Lancelot::PlasmaServiceListModel.
Definition at line 108 of file ActionListModel.cpp.
|
slot |
Activates the specified element.
- Parameters
-
index of the element that should be activated
Definition at line 84 of file ActionListModel.cpp.
|
virtual |
Method for handling context menu actions.
- Parameters
-
index of the activated item context index of the context action
Reimplemented in Lancelot::MergedActionListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 102 of file ActionListModel.cpp.
|
virtual |
This function is invoked when a data is dropped.
- Parameters
-
index index of the dragged item action invoked drop action
Reimplemented in Lancelot::MergedActionListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 65 of file ActionListModel.cpp.
|
virtual |
- Returns
- whether the data can be dropped at the specified position
- Parameters
-
where position mimeData dragged data
Reimplemented in Lancelot::MergedActionListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 52 of file ActionListModel.cpp.
|
virtual |
Invoked when the data is dropped into the model.
- Parameters
-
where position mimeData dropped data
Reimplemented in Lancelot::MergedActionListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 59 of file ActionListModel.cpp.
|
virtual |
- Parameters
-
index index of the item
- Returns
- description of the specified item
Reimplemented in Lancelot::MergedActionListModel, Lancelot::StandardActionTreeModel, Lancelot::StandardActionListModel, Lancelot::PlasmaServiceListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 34 of file ActionListModel.cpp.
|
virtual |
- Returns
- whether the specified item has context actions
- Parameters
-
index index of the item
Reimplemented in Lancelot::MergedActionListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 90 of file ActionListModel.cpp.
|
virtual |
- Parameters
-
index index of the item
- Returns
- icon for the specified item
Reimplemented in Lancelot::MergedActionListModel, Lancelot::StandardActionTreeModel, Lancelot::StandardActionListModel, Lancelot::PlasmaServiceListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 40 of file ActionListModel.cpp.
|
virtual |
- Parameters
-
index index of the item
- Returns
- whether the item represents a category
Reimplemented in Lancelot::MergedActionListModel, Lancelot::StandardActionTreeModel, Lancelot::StandardActionListModel, Lancelot::PlasmaServiceListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 78 of file ActionListModel.cpp.
|
signal |
This signal is emitted when an item is activated.
- Parameters
-
index of the activated element
|
signal |
This signal is emitted when an item is altered.
- Parameters
-
index index of the altered item
|
signal |
This signal is emitted when an item is deleted from the model.
- Parameters
-
index index of the deleted item
|
signal |
This signal is emitted when an item is inserted into the model.
- Parameters
-
index place where the new item is inserted
|
virtual |
- Parameters
-
index index of the item
- Returns
- mime data for the specified item
Reimplemented in Lancelot::MergedActionListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 46 of file ActionListModel.cpp.
|
virtual |
Reimplemented in Lancelot::StandardActionTreeModel, Lancelot::PlasmaServiceListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 123 of file ActionListModel.cpp.
|
virtual |
Definition at line 128 of file ActionListModel.cpp.
|
virtual |
Definition at line 118 of file ActionListModel.cpp.
|
virtual |
Reimplemented in Lancelot::StandardActionTreeModel, Lancelot::PlasmaServiceListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 113 of file ActionListModel.cpp.
|
virtual |
Adds actions ofr the specifies item to menu.
- Parameters
-
index index of the item menu menu to add the actions to
Reimplemented in Lancelot::MergedActionListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 96 of file ActionListModel.cpp.
|
virtual |
- Parameters
-
index index of the item to be dragged actions supported drop actions defaultAction default drop action
Reimplemented in Lancelot::MergedActionListModel, and Lancelot::ActionTreeModelProxy.
Definition at line 71 of file ActionListModel.cpp.
|
pure virtual |
- Returns
- the number of items in model
Implemented in Lancelot::MergedActionListModel, Lancelot::StandardActionTreeModel, Lancelot::StandardActionListModel, Lancelot::ActionTreeModelProxy, and Lancelot::PlasmaServiceListModel.
|
pure virtual |
- Parameters
-
index index of the item
- Returns
- title for the specified item
Implemented in Lancelot::MergedActionListModel, Lancelot::StandardActionTreeModel, Lancelot::StandardActionListModel, Lancelot::PlasmaServiceListModel, and Lancelot::ActionTreeModelProxy.
|
signal |
This signal is emitted when the model is updated and the update is too complex to explain using itemInserted, itemDeleted and itemAltered methods.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:43:01 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.