liblancelot
#include <FullBorderLayout.h>
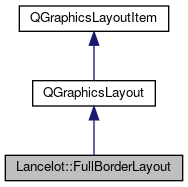
Public Types | |
enum | Border { TopBorder = 1, BottomBorder = 2, LeftBorder = 4, RightBorder = 8 } |
enum | Place { Center = 0, Top = TopBorder, Bottom = BottomBorder, Left = LeftBorder, Right = RightBorder, TopLeft = TopBorder | LeftBorder, TopRight = TopBorder | RightBorder, BottomLeft = BottomBorder | LeftBorder, BottomRight = BottomBorder | RightBorder } |
Public Member Functions | |
FullBorderLayout (QGraphicsLayoutItem *parent=0) | |
virtual | ~FullBorderLayout () |
void | addItem (QGraphicsLayoutItem *item) |
void | addItem (QGraphicsLayoutItem *item, Place position) |
L_Override int | count () const |
L_Override QGraphicsLayoutItem * | itemAt (int i) const |
L_Override void | removeAt (int index) |
void | setAutoSize (Border border) |
L_Override void | setGeometry (const QRectF &rect) |
void | setSize (qreal size, Border border) |
qreal | size (Border border) const |
L_Override QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint=QSizeF()) const |
![]() | |
QGraphicsLayout (QGraphicsLayoutItem *parent) | |
~QGraphicsLayout () | |
void | activate () |
virtual int | count () const =0 |
virtual void | getContentsMargins (qreal *left, qreal *top, qreal *right, qreal *bottom) const |
virtual void | invalidate () |
bool | isActivated () const |
virtual QGraphicsLayoutItem * | itemAt (int i) const =0 |
virtual void | removeAt (int index)=0 |
void | setContentsMargins (qreal left, qreal top, qreal right, qreal bottom) |
virtual void | updateGeometry () |
virtual void | widgetEvent (QEvent *e) |
![]() | |
QGraphicsLayoutItem (QGraphicsLayoutItem *parent, bool isLayout) | |
virtual | ~QGraphicsLayoutItem () |
QRectF | contentsRect () const |
QSizeF | effectiveSizeHint (Qt::SizeHint which, const QSizeF &constraint) const |
QRectF | geometry () const |
QGraphicsItem * | graphicsItem () const |
bool | isLayout () const |
qreal | maximumHeight () const |
QSizeF | maximumSize () const |
qreal | maximumWidth () const |
qreal | minimumHeight () const |
QSizeF | minimumSize () const |
qreal | minimumWidth () const |
bool | ownedByLayout () const |
QGraphicsLayoutItem * | parentLayoutItem () const |
qreal | preferredHeight () const |
QSizeF | preferredSize () const |
qreal | preferredWidth () const |
void | setMaximumHeight (qreal height) |
void | setMaximumSize (const QSizeF &size) |
void | setMaximumSize (qreal w, qreal h) |
void | setMaximumWidth (qreal width) |
void | setMinimumHeight (qreal height) |
void | setMinimumSize (const QSizeF &size) |
void | setMinimumSize (qreal w, qreal h) |
void | setMinimumWidth (qreal width) |
void | setParentLayoutItem (QGraphicsLayoutItem *parent) |
void | setPreferredHeight (qreal height) |
void | setPreferredSize (const QSizeF &size) |
void | setPreferredSize (qreal w, qreal h) |
void | setPreferredWidth (qreal width) |
void | setSizePolicy (const QSizePolicy &policy) |
void | setSizePolicy (QSizePolicy::Policy hPolicy, QSizePolicy::Policy vPolicy, QSizePolicy::ControlType controlType) |
QSizePolicy | sizePolicy () const |
Additional Inherited Members | |
![]() | |
bool | instantInvalidatePropagation () |
void | setInstantInvalidatePropagation (bool enable) |
![]() | |
void | addChildLayoutItem (QGraphicsLayoutItem *layoutItem) |
![]() | |
void | setGraphicsItem (QGraphicsItem *item) |
void | setOwnedByLayout (bool ownership) |
virtual QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint) const =0 |
Detailed Description
A modified version of Plasma::BorderLayout.
A layout which lays one item per border (left, top, bottom, right) one per corner and one item in center.
Definition at line 38 of file FullBorderLayout.h.
Member Enumeration Documentation
Borders enum.
Enumerator | |
---|---|
TopBorder | |
BottomBorder |
Top border. |
LeftBorder |
Bottom border. |
RightBorder |
Left border. |
Definition at line 43 of file FullBorderLayout.h.
Positions supported by FullBorderLayout.
Enumerator | |
---|---|
Center | |
Top | |
Bottom | |
Left | |
Right | |
TopLeft | |
TopRight | |
BottomLeft | |
BottomRight |
Definition at line 53 of file FullBorderLayout.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new Lancelot::FullBorderLayout.
- Parameters
-
parent parent layout item
Definition at line 112 of file FullBorderLayout.cpp.
|
virtual |
Destroys this Lancelot::FullBorderLayout.
Definition at line 117 of file FullBorderLayout.cpp.
Member Function Documentation
void Lancelot::FullBorderLayout::addItem | ( | QGraphicsLayoutItem * | item | ) |
Adds item in the center.
Equal to: addItem(item, Center);
- Parameters
-
item item to add
Definition at line 230 of file FullBorderLayout.cpp.
void Lancelot::FullBorderLayout::addItem | ( | QGraphicsLayoutItem * | item, |
Place | position | ||
) |
Adds item at the specified position.
- Parameters
-
item item to add position position to which to add
Definition at line 235 of file FullBorderLayout.cpp.
int Lancelot::FullBorderLayout::count | ( | ) | const |
Definition at line 241 of file FullBorderLayout.cpp.
QGraphicsLayoutItem * Lancelot::FullBorderLayout::itemAt | ( | int | i | ) | const |
Definition at line 252 of file FullBorderLayout.cpp.
void Lancelot::FullBorderLayout::removeAt | ( | int | index | ) |
Definition at line 283 of file FullBorderLayout.cpp.
void Lancelot::FullBorderLayout::setAutoSize | ( | Border | border | ) |
Activates the automatic sizing of a border widget, according to it's sizeHint()
- Parameters
-
border border for which the auto size is being specified
Definition at line 272 of file FullBorderLayout.cpp.
|
virtual |
Reimplemented from QGraphicsLayoutItem.
Definition at line 122 of file FullBorderLayout.cpp.
void Lancelot::FullBorderLayout::setSize | ( | qreal | size, |
Border | border | ||
) |
Deactivates the automatic sizing of a border widget, and sets it to the specified size.
For left and right widgets, it sets the width; while for top and bottom ones, it sets the height.
- Parameters
-
size size of the border border border for which the size is being specified
Definition at line 266 of file FullBorderLayout.cpp.
qreal Lancelot::FullBorderLayout::size | ( | Border | border | ) | const |
Returns the size of the specified border widget.
If automatic sizing for that border widget is activated, it will return a value less than zero.
- Parameters
-
border border for which the size is requested
Definition at line 278 of file FullBorderLayout.cpp.
QSizeF Lancelot::FullBorderLayout::sizeHint | ( | Qt::SizeHint | which, |
const QSizeF & | constraint = QSizeF() |
||
) | const |
Definition at line 203 of file FullBorderLayout.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:43:01 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.