KEditListBox Class Reference
from PyKDE4.kdeui import *
Inherits: QGroupBox → QWidget → QObject
Detailed Description
An editable listbox
This class provides a editable listbox ;-), this means a listbox which is accompanied by a line edit to enter new items into the listbox and pushbuttons to add and remove items from the listbox and two buttons to move items up and down.
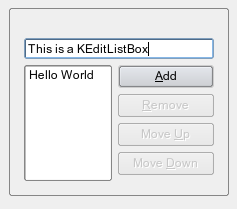
"KDE Edit List Box Widget"
Enumerations | |
Button | { Add, Remove, UpDown, All } Typesafe wrapper: Buttons |
Signals | |
added (QString text) | |
changed () | |
removed (QString text) | |
Methods | |
__init__ (self, QWidget parent=0) | |
__init__ (self, QString title, QWidget parent=0) | |
__init__ (self, QWidget parent, QString name, bool checkAtEntering=0, KEditListBox.Buttons buttons=KEditListBox.All) | |
__init__ (self, QString title, QWidget parent, QString name, bool checkAtEntering=0, KEditListBox.Buttons buttons=KEditListBox.All) | |
__init__ (self, QString title, KEditListBox.CustomEditor customEditor, QWidget parent=0, QString name=0, bool checkAtEntering=0, KEditListBox.Buttons buttons=KEditListBox.All) | |
__init__ (self, KEditListBox a0) | |
QPushButton | addButton (self) |
addItem (self) | |
added (self, QString text) | |
KEditListBox.Buttons | buttons (self) |
changed (self) | |
bool | checkAtEntering (self) |
clear (self) | |
int | count (self) |
int | currentItem (self) |
QString | currentText (self) |
QPushButton | downButton (self) |
enableMoveButtons (self, QModelIndex a0, QModelIndex a1) | |
bool | eventFilter (self, QObject o, QEvent e) |
insertItem (self, QString text, int index=-1) | |
insertStringList (self, QStringList list, int index=-1) | |
QStringList | items (self) |
KLineEdit | lineEdit (self) |
QListView | listView (self) |
moveItemDown (self) | |
moveItemUp (self) | |
QPushButton | removeButton (self) |
removeItem (self) | |
removed (self, QString text) | |
setButtons (self, KEditListBox.Buttons buttons) | |
setCheckAtEntering (self, bool check) | |
setCustomEditor (self, KEditListBox.CustomEditor editor) | |
setItems (self, QStringList items) | |
QString | text (self, int index) |
typedSomething (self, QString text) | |
QPushButton | upButton (self) |
Method Documentation
__init__ | ( | self, | ||
QWidget | parent=0 | |||
) |
Create an editable listbox.
Create an editable listbox.
The same as the other constructor, additionally it takes title, which will be the title of the groupbox around the listbox.
__init__ | ( | self, | ||
QWidget | parent, | |||
QString | name, | |||
bool | checkAtEntering=0, | |||
KEditListBox.Buttons | buttons=KEditListBox.All | |||
) |
Create an editable listbox.
- Deprecated:
If checkAtEntering is true, after every character you type in the line edit KEditListBox will enable or disable the Add-button, depending whether the current content of the line edit is already in the listbox. Maybe this can become a performance hit with large lists on slow machines. If checkAtEntering is false, it will be checked if you press the Add-button. It is not possible to enter items twice into the listbox.
__init__ | ( | self, | ||
QString | title, | |||
QWidget | parent, | |||
QString | name, | |||
bool | checkAtEntering=0, | |||
KEditListBox.Buttons | buttons=KEditListBox.All | |||
) |
Create an editable listbox.
- Deprecated:
The same as the other constructor, additionally it takes title, which will be the title of the frame around the listbox.
__init__ | ( | self, | ||
QString | title, | |||
KEditListBox.CustomEditor | customEditor, | |||
QWidget | parent=0, | |||
QString | name=0, | |||
bool | checkAtEntering=0, | |||
KEditListBox.Buttons | buttons=KEditListBox.All | |||
) |
Another constructor, which allows to use a custom editing widget instead of the standard KLineEdit widget. E.g. you can use a KUrlRequester or a KComboBox as input widget. The custom editor must consist of a lineedit and optionally another widget that is used as representation. A KComboBox or a KUrlRequester have a KLineEdit as child-widget for example, so the KComboBox is used as the representation widget.
- See also:
- KUrlRequester.customEditor(), setCustomEditor
__init__ | ( | self, | ||
KEditListBox | a0 | |||
) |
QPushButton addButton | ( | self ) |
Return a pointer to the Add button
addItem | ( | self ) |
added | ( | self, | ||
QString | text | |||
) |
This signal is emitted when the user adds a new string to the list, the parameter is the added string.
- Signal syntax:
QObject.connect(source, SIGNAL("added(const QString&)"), target_slot)
KEditListBox.Buttons buttons | ( | self ) |
Returns which buttons are visible
changed | ( | self ) |
- Signal syntax:
QObject.connect(source, SIGNAL("changed()"), target_slot)
bool checkAtEntering | ( | self ) |
Returns true if check at entering is enabled.
clear | ( | self ) |
Clears both the listbox and the line edit.
int count | ( | self ) |
See QListBox.count()
int currentItem | ( | self ) |
See QListBox.currentItem()
QString currentText | ( | self ) |
See QListBox.currentText()
QPushButton downButton | ( | self ) |
Return a pointer to the Down button
enableMoveButtons | ( | self, | ||
QModelIndex | a0, | |||
QModelIndex | a1 | |||
) |
Reimplented for interal reasons. The API is not affected.
insertItem | ( | self, | ||
QString | text, | |||
int | index=-1 | |||
) |
See QListBox.insertStrList()
insertStringList | ( | self, | ||
QStringList | list, | |||
int | index=-1 | |||
) |
See QListBox.insertStringList()
QStringList items | ( | self ) |
- Returns:
- a stringlist of all items in the listbox
KLineEdit lineEdit | ( | self ) |
Return a pointer to the embedded KLineEdit.
QListView listView | ( | self ) |
Return a pointer to the embedded QListView.
moveItemDown | ( | self ) |
moveItemUp | ( | self ) |
QPushButton removeButton | ( | self ) |
Return a pointer to the Remove button
removeItem | ( | self ) |
removed | ( | self, | ||
QString | text | |||
) |
This signal is emitted when the user removes a string from the list, the parameter is the removed string.
- Signal syntax:
QObject.connect(source, SIGNAL("removed(const QString&)"), target_slot)
setButtons | ( | self, | ||
KEditListBox.Buttons | buttons | |||
) |
Specifies which buttons should be visible
setCheckAtEntering | ( | self, | ||
bool | check | |||
) |
If check is true, after every character you type in the line edit KEditListBox will enable or disable the Add-button, depending whether the current content of the line edit is already in the listbox. Maybe this can become a performance hit with large lists on slow machines. If check is false, it will be checked if you press the Add-button. It is not possible to enter items twice into the listbox. Default is false.
setCustomEditor | ( | self, | ||
KEditListBox.CustomEditor | editor | |||
) |
Allows to use a custom editing widget instead of the standard KLineEdit widget. E.g. you can use a KUrlRequester or a KComboBox as input widget. The custom editor must consist of a lineedit and optionally another widget that is used as representation. A KComboBox or a KUrlRequester have a KLineEdit as child-widget for example, so the KComboBox is used as the representation widget.
- Since:
- 4.1
setItems | ( | self, | ||
QStringList | items | |||
) |
Clears the listbox and sets the contents to items
QString text | ( | self, | ||
int | index | |||
) |
See QListBox.text()
typedSomething | ( | self, | ||
QString | text | |||
) |
QPushButton upButton | ( | self ) |
Return a pointer to the Up button
Enumeration Documentation
Button |
Enumeration of the buttons, the listbox offers. Specify them in the constructor in the buttons parameter, or in setButtons.
- Note:
- It is necessary to wrap members of this enumeration in a
Buttons
instance when passing them to a method as group of flags. For example:Buttons( Add | Remove)
- Enumerator:
-
Add = 0x0001 Remove = 0x0002 UpDown = 0x0004 All = Add|Remove|UpDown