KPassivePopup Class Reference
from PyKDE4.kdeui import *
Inherits: QFrame → QWidget → QObject
Detailed Description
A dialog-like popup that displays messages without interrupting the user.
The simplest uses of KPassivePopup are by using the various message() static methods. The position the popup appears at depends on the type of the parent window:
You also have the option of calling show with a QPoint as a parameter that removes the automatic placing of KPassivePopup and shows it in the point you want.
The most basic use of KPassivePopup displays a popup containing a piece of text:
KPassivePopup.message( "This is the message", this );We can create popups with titles and icons too, as this example shows:
QPixmap px; px.load( "hi32-app-logtracker.png" ); KPassivePopup.message( "Some title", "This is the main text", px, this );This screenshot shows a popup with both a caption and a main text which is being displayed next to the toolbar icon of the window that triggered it:
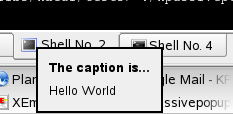
"A passive popup"
For more control over the popup, you can use the setView(QWidget *) method to create a custom popup.
KPassivePopup *pop = new KPassivePopup( parent ); KVBox *vb = new KVBox( pop ); (void) new QLabel( vb, "<b>Isn't this great?</b>" ); KHBox *box = new KHBox( vb ); (void) new QPushButton( "Yes", box ); (void) new QPushButton( "No", box ); pop->setView( vb ); pop->show();
Enumerations | |
PopupStyle | { Boxed, Balloon, CustomStyle } |
Signals | |
clicked () | |
clicked (QPoint pos) | |
Methods | |
__init__ (self, QWidget parent=0, Qt.WFlags f=0) | |
__init__ (self, WId parent) | |
QPoint | anchor (self) |
bool | autoDelete (self) |
QPoint | calculateNearbyPoint (self, QRect target) |
clicked (self) | |
clicked (self, QPoint pos) | |
QRect | defaultArea (self) |
hideEvent (self, QHideEvent a0) | |
mouseReleaseEvent (self, QMouseEvent e) | |
moveNear (self, QRect target) | |
paintEvent (self, QPaintEvent pe) | |
positionSelf (self) | |
setAnchor (self, QPoint anchor) | |
setAutoDelete (self, bool autoDelete) | |
setPopupStyle (self, int popupstyle) | |
setTimeout (self, int delay) | |
setView (self, QWidget child) | |
setView (self, QString caption, QString text=QString()) | |
setView (self, QString caption, QString text, QPixmap icon) | |
setVisible (self, bool visible) | |
show (self) | |
show (self, QPoint p) | |
KVBox | standardView (self, QString caption, QString text, QPixmap icon, QWidget parent=0) |
int | timeout (self) |
updateMask (self) | |
QWidget | view (self) |
Static Methods | |
KPassivePopup | message (QString text, QWidget parent) |
KPassivePopup | message (QString text, QSystemTrayIcon parent) |
KPassivePopup | message (QString caption, QString text, QWidget parent) |
KPassivePopup | message (QString caption, QString text, QSystemTrayIcon parent) |
KPassivePopup | message (QString caption, QString text, QPixmap icon, QWidget parent, int timeout=-1) |
KPassivePopup | message (QString caption, QString text, QPixmap icon, QSystemTrayIcon parent, int timeout=-1) |
KPassivePopup | message (QString caption, QString text, QPixmap icon, WId parent, int timeout=-1) |
KPassivePopup | message (int popupStyle, QString text, QWidget parent) |
KPassivePopup | message (int popupStyle, QString text, QSystemTrayIcon parent) |
KPassivePopup | message (int popupStyle, QString caption, QString text, QSystemTrayIcon parent) |
KPassivePopup | message (int popupStyle, QString caption, QString text, QWidget parent) |
KPassivePopup | message (int popupStyle, QString caption, QString text, QPixmap icon, QWidget parent, int timeout=-1) |
KPassivePopup | message (int popupStyle, QString caption, QString text, QPixmap icon, QSystemTrayIcon parent, int timeout=-1) |
KPassivePopup | message (int popupStyle, QString caption, QString text, QPixmap icon, WId parent, int timeout=-1) |
Method Documentation
__init__ | ( | self, | ||
WId | parent | |||
) |
Creates a popup for the specified window.
QPoint anchor | ( | self ) |
Returns the position to which this popup is anchored.
bool autoDelete | ( | self ) |
- Returns:
- true if the widget auto-deletes itself when the timeout occurs.
- See also:
- setAutoDelete
Calculates the position to place the popup near the specified rectangle.
clicked | ( | self ) |
Emitted when the popup is clicked.
- Signal syntax:
QObject.connect(source, SIGNAL("clicked()"), target_slot)
clicked | ( | self, | ||
QPoint | pos | |||
) |
Emitted when the popup is clicked.
- Signal syntax:
QObject.connect(source, SIGNAL("clicked(const QPoint&)"), target_slot)
QRect defaultArea | ( | self ) |
If no relative window (eg taskbar button, system tray window) is available, use this rectangle (pass it to moveNear()). Basically KWindowSystem.workArea() with width and height set to 0 so that moveNear uses the upper-left position.
- Returns:
- The QRect to be passed to moveNear() if no other is available.
hideEvent | ( | self, | ||
QHideEvent | a0 | |||
) |
Reimplemented to destroy the object when autoDelete() is enabled.
KPassivePopup message | ( | QString | text, | |
QWidget | parent | |||
) |
Convenience method that displays popup with the specified message beside the icon of the specified widget. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | QString | text, | |
QSystemTrayIcon | parent | |||
) |
Convenience method that displays popup with the specified message beside the icon of the specified QSystemTrayIcon. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | QString | caption, | |
QString | text, | |||
QWidget | parent | |||
) |
Convenience method that displays popup with the specified caption and message beside the icon of the specified widget. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | QString | caption, | |
QString | text, | |||
QSystemTrayIcon | parent | |||
) |
Convenience method that displays popup with the specified caption and message beside the icon of the specified QSystemTrayIcon. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | QString | caption, | |
QString | text, | |||
QPixmap | icon, | |||
QWidget | parent, | |||
int | timeout=-1 | |||
) |
Convenience method that displays popup with the specified icon, caption and message beside the icon of the specified widget. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | QString | caption, | |
QString | text, | |||
QPixmap | icon, | |||
QSystemTrayIcon | parent, | |||
int | timeout=-1 | |||
) |
Convenience method that displays popup with the specified icon, caption and message beside the icon of the specified QSystemTrayIcon. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | QString | caption, | |
QString | text, | |||
QPixmap | icon, | |||
WId | parent, | |||
int | timeout=-1 | |||
) |
Convenience method that displays popup with the specified icon, caption and message beside the icon of the specified window. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | int | popupStyle, | |
QString | text, | |||
QWidget | parent | |||
) |
Convenience method that displays popup with the specified popup-style and message beside the icon of the specified widget. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | int | popupStyle, | |
QString | text, | |||
QSystemTrayIcon | parent | |||
) |
Convenience method that displays popup with the specified popup-style and message beside the icon of the specified QSystemTrayIcon. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | int | popupStyle, | |
QString | caption, | |||
QString | text, | |||
QSystemTrayIcon | parent | |||
) |
Convenience method that displays popup with the specified popup-style, caption and message beside the icon of the specified QSystemTrayIcon. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | int | popupStyle, | |
QString | caption, | |||
QString | text, | |||
QWidget | parent | |||
) |
Convenience method that displays popup with the specified popup-style, caption and message beside the icon of the specified widget. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | int | popupStyle, | |
QString | caption, | |||
QString | text, | |||
QPixmap | icon, | |||
QWidget | parent, | |||
int | timeout=-1 | |||
) |
Convenience method that displays popup with the specified popup-style, icon, caption and message beside the icon of the specified widget. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | int | popupStyle, | |
QString | caption, | |||
QString | text, | |||
QPixmap | icon, | |||
QSystemTrayIcon | parent, | |||
int | timeout=-1 | |||
) |
Convenience method that displays popup with the specified popup-style, icon, caption and message beside the icon of the specified QSystemTrayIcon. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
KPassivePopup message | ( | int | popupStyle, | |
QString | caption, | |||
QString | text, | |||
QPixmap | icon, | |||
WId | parent, | |||
int | timeout=-1 | |||
) |
Convenience method that displays popup with the specified popup-style, icon, caption and message beside the icon of the specified window. Note that the returned object is destroyed when it is hidden.
- See also:
- setAutoDelete
mouseReleaseEvent | ( | self, | ||
QMouseEvent | e | |||
) |
Reimplemented to detect mouse clicks.
moveNear | ( | self, | ||
QRect | target | |||
) |
Moves the popup to be adjacent to the icon of the specified rectangle.
paintEvent | ( | self, | ||
QPaintEvent | pe | |||
) |
Overwrite to paint the border when PopupStyle == Balloon. Unused if PopupStyle == Boxed
positionSelf | ( | self ) |
This method positions the popup.
setAnchor | ( | self, | ||
QPoint | anchor | |||
) |
Sets the anchor of this popup. The popup tries automatically to adjust itself somehow around the point.
setAutoDelete | ( | self, | ||
bool | autoDelete | |||
) |
Enables / disables auto-deletion of this widget when the timeout occurs. The default is false. If you use the class-methods message(), auto-deletion is turned on by default.
setPopupStyle | ( | self, | ||
int | popupstyle | |||
) |
Sets the visual appearance of the popup.
- See also:
- PopupStyle
setTimeout | ( | self, | ||
int | delay | |||
) |
Sets the delay for the popup is removed automatically. Setting the delay to 0 disables the timeout, if you're doing this, you may want to connect the clicked() signal to the hide() slot. Setting the delay to -1 makes it use the default value.
- See also:
- timeout
setView | ( | self, | ||
QWidget | child | |||
) |
Sets the main view to be the specified widget (which must be a child of the popup).
Creates a standard view then calls setView(QWidget*) .
Creates a standard view then calls setView(QWidget*) .
setVisible | ( | self, | ||
bool | visible | |||
) |
show | ( | self ) |
Reimplemented to reposition the popup.
show | ( | self, | ||
QPoint | p | |||
) |
Shows the popup in the given point
Returns a widget that is used as standard view if one of the setView() methods taking the QString arguments is used. You can use the returned widget to customize the passivepopup while keeping the look similar to the "standard" passivepopups.
After customizing the widget, pass it to setView( QWidget* )
- Parameters:
-
caption The window caption (title) on the popup text The text for the popup icon The icon to use for the popup parent The parent widget used for the returned KVBox. If left 0, then "this", i.e. the passive popup object will be used.
- Returns:
- a KVBox containing the given arguments, looking like the standard passivepopups.
- See also:
- setView( QWidget * )
- See also:
- setView( const QString&, const QString& )
- See also:
- setView( const QString&, const QString&, const QPixmap& )
int timeout | ( | self ) |
Returns the delay before the popup is removed automatically.
updateMask | ( | self ) |
Updates the transparency mask. Unused if PopupStyle == Boxed
QWidget view | ( | self ) |
Returns the main view.
Enumeration Documentation
PopupStyle |
Styles that a KPassivePopup can have.
- Enumerator:
-
Boxed Balloon CustomStyle = 128