KUrlRequester Class Reference
from PyKDE4.kio import *
Inherits: KHBox → QFrame → QWidget → QObject
Subclasses: KUrlComboRequester
Detailed Description
This class is a widget showing a lineedit and a button, which invokes a filedialog. File name completion is available in the lineedit.
The defaults for the filedialog are to ask for one existing local file, i.e. KFileDialog.setMode( KFile.File | KFile.ExistingOnly | KFile.LocalOnly ) The default filter is "*", i.e. show all files, and the start directory is the current working directory, or the last directory where a file has been selected.
You can change this behavior by using setMode() or setFilter().
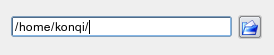
"KDE URL Requester"
A widget to request a filename/url from the user
Method Documentation
__init__ | ( | self, | ||
QWidget | parent=0 | |||
) |
Constructs a KUrlRequester widget.
Constructs a KUrlRequester widget with the initial URL url.
Special constructor, which creates a KUrlRequester widget with a custom edit-widget. The edit-widget can be either a KComboBox or a KLineEdit (or inherited thereof). Note: for geometry management reasons, the edit-widget is reparented to have the KUrlRequester as parent.
__init__ | ( | self, | ||
KUrlRequester | a0 | |||
) |
KPushButton button | ( | self ) |
- Returns:
- a pointer to the pushbutton. It is provided so that you can specify an own pixmap or a text, if you really need to.
changeEvent | ( | self, | ||
QEvent | e | |||
) |
clear | ( | self ) |
Clears the lineedit/combobox.
QString clickMessage | ( | self ) |
- Returns:
- the message set with setClickMessage
- Since:
- 4.2
KComboBox comboBox | ( | self ) |
- Returns:
- a pointer to the combobox, in case you have set one using the special constructor. Returns 0 otherwise.
KUrlCompletion completionObject | ( | self ) |
- Returns:
- the KUrlCompletion object used in the lineedit/combobox.
KEditListBox.CustomEditor customEditor | ( | self ) |
- Returns:
- an object, suitable for use with KEditListBox. It allows you to put this KUrlRequester into a KEditListBox. Basically, do it like this:
KUrlRequester *req = new KUrlRequester( someWidget ); [...] KEditListBox *editListBox = new KEditListBox( i18n("Some Title"), req->customEditor(), someWidget );
KFileDialog fileDialog | ( | self ) |
- Returns:
- a pointer to the filedialog You can use this to customize the dialog, e.g. to specify a filter. Never returns 0.
Remove in KDE4? KUrlRequester should use KDirSelectDialog for (mode & KFile.Directory) && !(mode & KFile.File)
QString filter | ( | self ) |
Returns the current filter for the file dialog.
- See also:
- KFileDialog.filter()
KLineEdit lineEdit | ( | self ) |
- Returns:
- a pointer to the lineedit, either the default one, or the special one, if you used the special constructor.
It is provided so that you can e.g. set an own completion object (e.g. KShellCompletion) into it.
KFile.Modes mode | ( | self ) |
Returns the current mode
- See also:
- KFileDialog.mode()
openFileDialog | ( | self, | ||
KUrlRequester | a0 | |||
) |
Emitted before the filedialog is going to open. Connect to this signal to "configure" the filedialog, e.g. set the filefilter, the mode, a preview-widget, etc. It's usually not necessary to set a URL for the filedialog, as it will get set properly from the editfield contents.
If you use multiple KUrlRequesters, you can connect all of them to the same slot and use the given KUrlRequester pointer to know which one is going to open.
- Signal syntax:
QObject.connect(source, SIGNAL("openFileDialog(KUrlRequester*)"), target_slot)
returnPressed | ( | self ) |
Emitted when return or enter was pressed in the lineedit.
- Signal syntax:
QObject.connect(source, SIGNAL("returnPressed()"), target_slot)
returnPressed | ( | self, | ||
QString | a0 | |||
) |
Emitted when return or enter was pressed in the lineedit. The parameter contains the contents of the lineedit.
- Signal syntax:
QObject.connect(source, SIGNAL("returnPressed(const QString&)"), target_slot)
setClickMessage | ( | self, | ||
QString | msg | |||
) |
Set a click message msg
- Since:
- 4.2
setFilter | ( | self, | ||
QString | filter | |||
) |
Sets the filter for the file dialog.
- See also:
- KFileDialog.setFilter()
setMode | ( | self, | ||
KFile.Modes | m | |||
) |
Sets the mode of the file dialog. Note: you can only select one file with the filedialog, so KFile.Files doesn't make much sense.
- See also:
- KFileDialog.setMode()
setPath | ( | self, | ||
QString | path | |||
) |
Sets the url in the lineedit to KUrl.fromPath(path). This is only for local paths; do not pass a url here. This method is mostly for "local paths only" url requesters, for instance those set up with setMode(KFile.File|KFile.ExistingOnly|KFile.LocalOnly)
setUrl | ( | self, | ||
KUrl | url | |||
) |
Sets the url in the lineedit to url.
QString text | ( | self ) |
- Returns:
- the current text in the lineedit or combobox. This does not do the URL expansion that url() does, it's only provided for cases where KUrlRequester is used to enter URL-or-something-else, like KOpenWithDialog where you can type a full command with arguments.
- Since:
- 4.2
textChanged | ( | self, | ||
QString | a0 | |||
) |
Emitted when the text in the lineedit changes. The parameter contains the contents of the lineedit.
- Signal syntax:
QObject.connect(source, SIGNAL("textChanged(const QString&)"), target_slot)
KUrl url | ( | self ) |
- Returns:
- the current url in the lineedit. May be malformed, if the user entered something weird. ~user or environment variables are substituted for local files.
urlSelected | ( | self, | ||
KUrl | a0 | |||
) |
Emitted when the user changed the URL via the file dialog. The parameter contains the contents of the lineedit.
- Signal syntax:
QObject.connect(source, SIGNAL("urlSelected(const KUrl&)"), target_slot)