KColorDialog Class Reference
from PyKDE4.kdeui import *
Inherits: KDialog → QDialog → QWidget → QObject
Detailed Description
A color selection dialog.
Features:\n
In most cases, you will want to use the static method KColorDialog.getColor(). This pops up the dialog (with an initial selection provided by you), lets the user choose a color, and returns.
Example:
QColor myColor; int result = KColorDialog.getColor( myColor ); if ( result == KColorDialog.Accepted ) ...
To react to the color selection as it is being selected, the colorSelected() signal can be used. This can be used still in a modal way, for example:
KColorDialog dialog(this); connect(&dialog, SIGNAL(colorSelected(const QColor &)), this, SLOT(temporarilyChangeColor(const QColor &))); QColor myColor; dialog.setColor(myColor); int result = dialog.exec(); if ( result == KColorDialog.Accepted ) changeColor( dialog.color() ); else temporarilyChangeColor(myColor); //change back to original color
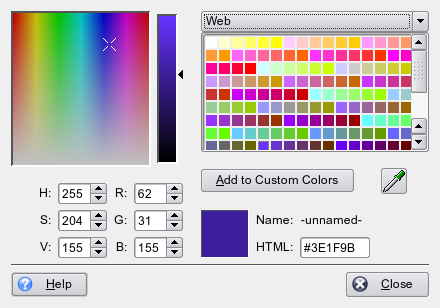
"KDE Color Dialog"
The color dialog is really a collection of several widgets which can you can also use separately: the quadratic plane in the top left of the dialog is a KXYSelector. Right next to it is a KHSSelector for choosing hue/saturation.
On the right side of the dialog you see a KColorTable showing a number of colors with a combo box which offers several predefined palettes or a palette configured by the user. The small field showing the currently selected color is a KColorPatch.
Signals | |
colorSelected (QColor col) | |
Methods | |
__init__ (self, QWidget parent=0, bool modal=0) | |
__init__ (self, KColorDialog a0) | |
QColor | color (self) |
QColor | defaultColor (self) |
bool | eventFilter (self, QObject obj, QEvent ev) |
bool | isAlphaChannelEnabled (self) |
keyPressEvent (self, QKeyEvent a0) | |
mouseMoveEvent (self, QMouseEvent a0) | |
mouseReleaseEvent (self, QMouseEvent a0) | |
setAlphaChannelEnabled (self, bool alpha) | |
setColor (self, QColor col) | |
setDefaultColor (self, QColor defaultCol) | |
Static Methods | |
int | getColor (QColor theColor, QWidget parent=0) |
int | getColor (QColor theColor, QColor defaultColor, QWidget parent=0) |
QColor | grabColor (QPoint p) |
Signal Documentation
colorSelected | ( | QColor | col | |
) |
Emitted when a color is selected. Connect to this to monitor the color as it as selected if you are not running modal.
- Signal syntax:
QObject.connect(source, SIGNAL("colorSelected(const QColor&)"), target_slot)
Method Documentation
__init__ | ( | self, | ||
QWidget | parent=0, | |||
bool | modal=0 | |||
) |
Constructs a color selection dialog.
__init__ | ( | self, | ||
KColorDialog | a0 | |||
) |
QColor color | ( | self ) |
Returns the currently selected color.
QColor defaultColor | ( | self ) |
- Returns:
- the value passed to setDefaultColor
bool eventFilter | ( | self, | ||
QObject | obj, | |||
QEvent | ev | |||
) |
bool isAlphaChannelEnabled | ( | self ) |
Returns true when the user can change the alpha channel.
- Since:
- 4.5
keyPressEvent | ( | self, | ||
QKeyEvent | a0 | |||
) |
mouseMoveEvent | ( | self, | ||
QMouseEvent | a0 | |||
) |
mouseReleaseEvent | ( | self, | ||
QMouseEvent | a0 | |||
) |
setAlphaChannelEnabled | ( | self, | ||
bool | alpha | |||
) |
When set to true, the user is allowed to change the alpha component of the color. The default value is false.
- Since:
- 4.5
setColor | ( | self, | ||
QColor | col | |||
) |
Preselects a color.
setDefaultColor | ( | self, | ||
QColor | defaultCol | |||
) |
Call this to make the dialog show a "Default Color" checkbox. If this checkbox is selected, the dialog will return an "invalid" color (QColor()). This can be used to mean "the default text color", for instance, the one with the KDE text color on screen, but black when printing.
Static Method Documentation
int getColor | ( | QColor | theColor, | |
QWidget | parent=0 | |||
) |
Creates a modal color dialog, lets the user choose a color, and returns when the dialog is closed.
The selected color is returned in the argument theColor.
This version takes a defaultColor argument, which sets the color selected by the "default color" checkbox. When this checkbox is checked, the invalid color (QColor()) is returned into theColor.
- Parameters:
-
theColor if valid, specifies the color to be initially selected. On return, holds the selected color. defaultColor color selected by the "default color" checkbox
- Returns:
- QDialog.result().
int getColor | ( | QColor | theColor, | |
QColor | defaultColor, | |||
QWidget | parent=0 | |||
) |
Creates a modal color dialog, lets the user choose a color, and returns when the dialog is closed.
The selected color is returned in the argument theColor.
This version takes a defaultColor argument, which sets the color selected by the "default color" checkbox. When this checkbox is checked, the invalid color (QColor()) is returned into theColor.
- Parameters:
-
theColor if valid, specifies the color to be initially selected. On return, holds the selected color. defaultColor color selected by the "default color" checkbox
- Returns:
- QDialog.result().
QColor grabColor | ( | QPoint | p | |
) |
Gets the color from the pixel at point p on the screen.