KEduVocDocument
#include <keduvocdocument.h>
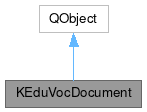
Public Types | |
enum | ErrorCode { NoError = 0 , Unknown , InvalidXml , FileTypeUnknown , FileCannotWrite , FileWriterFailed , FileCannotRead , FileReaderFailed , FileDoesNotExist , FileLocked , FileCannotLock , FileIsReadOnly } |
enum | FileDialogMode { Reading , Writing } |
enum | FileHandlingFlags { FileDefaultHandling = 0x0 , FileIgnoreLock = 0x1 , FileOpenReadOnly = 0x2 } |
enum | FileType { KvdNone , Automatic , Kvtml , Wql , Pauker , Vokabeln , Xdxf , Csv , Kvtml1 } |
enum | LessonDeletion { DeleteEmptyLesson , DeleteEntriesAndLesson } |
Signals | |
void | docModified (bool mod) |
void | progressChanged (KEduVocDocument *, int curr_percent) |
Public Member Functions | |
KEduVocDocument (QObject *parent=nullptr) | |
~KEduVocDocument () override | |
Whole Document Methods | |
Methods related to saving/loading and locating the document | |
ErrorCode | open (const QUrl &url, FileHandlingFlags flags=FileDefaultHandling) |
void | close () |
ErrorCode | saveAs (const QUrl &url, FileType ft, FileHandlingFlags flags=FileDefaultHandling) |
QByteArray | toByteArray (const QString &generator) |
void | merge (KEduVocDocument *docToMerge, bool matchIdentifiers) |
void | setModified (bool dirty=true) |
bool | isModified () const |
void | setUrl (const QUrl &url) |
QUrl | url () const |
General Document Properties | |
void | setTitle (const QString &title) |
QString | title () const |
void | setAuthor (const QString &author) |
QString | author () const |
void | setAuthorContact (const QString &authorContact) |
QString | authorContact () const |
void | setLicense (const QString &license) |
QString | license () const |
void | setDocumentComment (const QString &comment) |
QString | documentComment () const |
void | setCategory (const QString &category) |
QString | category () const |
void | setGenerator (const QString &generator) |
QString | generator () const |
void | setVersion (const QString &ver) |
QString | version () const |
Identifier Methods | |
int | identifierCount () const |
int | appendIdentifier (const KEduVocIdentifier &identifier=KEduVocIdentifier()) |
void | setIdentifier (int index, const KEduVocIdentifier &lang) |
KEduVocIdentifier & | identifier (int index) |
const KEduVocIdentifier & | identifier (int index) const |
void | removeIdentifier (int index) |
int | indexOfIdentifier (const QString &name) const |
Grade Methods | |
KEDUVOCDOCUMENT_DEPRECATED void | queryIdentifier (QString &org, QString &trans) const |
KEDUVOCDOCUMENT_DEPRECATED void | setQueryIdentifier (const QString &org, const QString &trans) |
Lesson Methods | |
KEduVocLesson * | lesson () |
KEduVocWordType * | wordTypeContainer () |
KEduVocLeitnerBox * | leitnerContainer () |
File Format Specific Methods | |
QString | csvDelimiter () const |
void | setCsvDelimiter (const QString &delimiter) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static FileType | detectFileType (const QString &fileName) |
static QString | errorDescription (int errorCode) |
static QString | pattern (FileDialogMode mode) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The primary entry point to the hierarchy of objects describing vocabularies.
This class contains the expressions of your vocabulary as well as other information about the vocabulary.
Definition at line 31 of file keduvocdocument.h.
Member Enumeration Documentation
◆ ErrorCode
the return code when opening/saving
Definition at line 51 of file keduvocdocument.h.
◆ FileDialogMode
used as parameter for pattern
Definition at line 74 of file keduvocdocument.h.
◆ FileHandlingFlags
indicates file open/save status locking or readonly
Enumerator | |
---|---|
FileDefaultHandling | Default status. |
FileIgnoreLock | Ignore the file lock. |
FileOpenReadOnly | Open without any intention to change and save back later. This also implies FileIgnoreLock. |
Definition at line 67 of file keduvocdocument.h.
◆ FileType
known vocabulary file types
Definition at line 38 of file keduvocdocument.h.
◆ LessonDeletion
delete only empty lessons or also if they have entries
Definition at line 80 of file keduvocdocument.h.
Constructor & Destructor Documentation
◆ KEduVocDocument()
|
explicit |
Constructor for a KDEEdu vocabulary document.
- Parameters
-
parent calling object
Definition at line 179 of file keduvocdocument.cpp.
◆ ~KEduVocDocument()
|
override |
Destructor.
Definition at line 185 of file keduvocdocument.cpp.
Member Function Documentation
◆ appendIdentifier()
int KEduVocDocument::appendIdentifier | ( | const KEduVocIdentifier & | identifier = KEduVocIdentifier() | ) |
Appends a new identifier (usually a language)
- Parameters
-
identifier the identifier to append. If empty default names are used.
- Returns
- the identifier number
Definition at line 650 of file keduvocdocument.cpp.
◆ author()
QString KEduVocDocument::author | ( | ) | const |
- Returns
- the author of the file
Definition at line 707 of file keduvocdocument.cpp.
◆ authorContact()
QString KEduVocDocument::authorContact | ( | ) | const |
- Returns
- the author contact information
Definition at line 718 of file keduvocdocument.cpp.
◆ category()
QString KEduVocDocument::category | ( | ) | const |
- Returns
- the category of the file
- Todo
- make writer/reader use this
Definition at line 745 of file keduvocdocument.cpp.
◆ close()
void KEduVocDocument::close | ( | ) |
Close a document file and release the lock on the file.
Definition at line 275 of file keduvocdocument.cpp.
◆ csvDelimiter()
QString KEduVocDocument::csvDelimiter | ( | ) | const |
Returns the delimiter (separator) used for csv import and export.
The default is a single tab character
- Returns
- the delimiter used
Definition at line 798 of file keduvocdocument.cpp.
◆ detectFileType()
|
static |
Detects the file type
- Parameters
-
fileName filename
- Returns
- enum of filetype
Definition at line 196 of file keduvocdocument.cpp.
◆ docModified
|
signal |
Emitted when the document becomes modified or saved.
- Parameters
-
mod the current modified/dirty state
- Returns
- state (true=modified)
◆ documentComment()
QString KEduVocDocument::documentComment | ( | ) | const |
- Returns
- the comment of the file
Definition at line 734 of file keduvocdocument.cpp.
◆ errorDescription()
|
static |
Returns a QString description of the errorCode.
- Parameters
-
errorCode the code
- Returns
- a user useful error string.
Definition at line 838 of file keduvocdocument.cpp.
◆ generator()
QString KEduVocDocument::generator | ( | ) | const |
- Returns
- the generator of the file
Definition at line 782 of file keduvocdocument.cpp.
◆ identifier() [1/2]
KEduVocIdentifier & KEduVocDocument::identifier | ( | int | index | ) |
Returns the identifier of translation index
.
- Parameters
-
index number of translation 0..x
- Returns
- the language identifier: en=english, de=german, ...
Definition at line 607 of file keduvocdocument.cpp.
◆ identifier() [2/2]
const KEduVocIdentifier & KEduVocDocument::identifier | ( | int | index | ) | const |
Const overload of identifier(int);.
- Parameters
-
index of the identifier
- Returns
- reference to the identifier
Definition at line 599 of file keduvocdocument.cpp.
◆ identifierCount()
int KEduVocDocument::identifierCount | ( | ) | const |
- Returns
- the number of different identifiers (usually languages)
Definition at line 645 of file keduvocdocument.cpp.
◆ indexOfIdentifier()
int KEduVocDocument::indexOfIdentifier | ( | const QString & | name | ) | const |
Determines the index of a given identifier.
- Parameters
-
name identifier of language
- Returns
- index of identifier, 0 = original, 1..n = translation, -1 = not found
Definition at line 624 of file keduvocdocument.cpp.
◆ isModified()
bool KEduVocDocument::isModified | ( | ) | const |
- Returns
- the modification state of the doc
Definition at line 640 of file keduvocdocument.cpp.
◆ leitnerContainer()
KEduVocLeitnerBox * KEduVocDocument::leitnerContainer | ( | ) |
Returns the root Leitner container.
- Returns
- pointer to the internal Leitner container object
- Todo
- in new API determine if this is used
Definition at line 676 of file keduvocdocument.cpp.
◆ lesson()
KEduVocLesson * KEduVocDocument::lesson | ( | ) |
Get the lesson root object.
- Returns
- a pointer to the lesson object
Definition at line 666 of file keduvocdocument.cpp.
◆ license()
QString KEduVocDocument::license | ( | ) | const |
- Returns
- the license of the file
Definition at line 729 of file keduvocdocument.cpp.
◆ merge()
void KEduVocDocument::merge | ( | KEduVocDocument * | docToMerge, |
bool | matchIdentifiers ) |
Merges data from another document (UNIMPLEMENTED)
- Parameters
-
docToMerge document containing the data to be merged matchIdentifiers if true only entries having identifiers present in the current document will be mergedurl is empty (or NULL) actual name is preserved
- Todo
- IMPLEMENT ME
Definition at line 383 of file keduvocdocument.cpp.
◆ open()
KEduVocDocument::ErrorCode KEduVocDocument::open | ( | const QUrl & | url, |
FileHandlingFlags | flags = FileDefaultHandling ) |
Opens and locks a document file.
Note: This is intended to be preserve binary compatible with int open(const QUrl&) When the API increments the major version number, then merge them
- Parameters
-
url url to file to open flags How to handle expected unusual conditions (i.e. locking)
- Returns
- ErrorCode
Definition at line 208 of file keduvocdocument.cpp.
◆ pattern()
|
static |
Create a string with the supported document types, that can be used as filter in KFileDialog.
It includes also an entry to match all the supported types.
- Parameters
-
mode the mode for the supported document types
- Returns
- the filter string
Definition at line 809 of file keduvocdocument.cpp.
◆ progressChanged
|
signal |
never used
- Parameters
-
curr_percent
◆ queryIdentifier()
Retrieves the identifiers for the current query not written in the new version!
- Parameters
-
org identifier for original trans identifier for translation
Definition at line 751 of file keduvocdocument.cpp.
◆ removeIdentifier()
void KEduVocDocument::removeIdentifier | ( | int | index | ) |
Removes identifier and the according translation in all entries.
- Parameters
-
index number of translation 0..x
Definition at line 632 of file keduvocdocument.cpp.
◆ saveAs()
KEduVocDocument::ErrorCode KEduVocDocument::saveAs | ( | const QUrl & | url, |
FileType | ft, | ||
FileHandlingFlags | flags = FileDefaultHandling ) |
Saves the data under the given name.
- Precondition
- generator is set.
Note: This is intended to be preserve binary compatible with int saveAs(const QUrl&, FileType ft, const QString & generator ); When the API increments the major version number, then merge them
- Parameters
-
url if url is empty (or NULL) actual name is preserved ft the filetype to be used when saving the document flags How to handle expected unusual conditions (i.e. locking)
- Returns
- ErrorCode
- Todo
- port me
Definition at line 282 of file keduvocdocument.cpp.
◆ setAuthor()
void KEduVocDocument::setAuthor | ( | const QString & | author | ) |
Set the author of the file.
- Parameters
-
author author to set
Definition at line 712 of file keduvocdocument.cpp.
◆ setAuthorContact()
void KEduVocDocument::setAuthorContact | ( | const QString & | authorContact | ) |
Set the author contact info.
- Parameters
-
authorContact contact email/contact info to set
Definition at line 723 of file keduvocdocument.cpp.
◆ setCategory()
void KEduVocDocument::setCategory | ( | const QString & | category | ) |
Set the category of the file.
- Parameters
-
category category to set
Definition at line 739 of file keduvocdocument.cpp.
◆ setCsvDelimiter()
void KEduVocDocument::setCsvDelimiter | ( | const QString & | delimiter | ) |
Sets the delimiter (separator) used for csv import and export.
- Parameters
-
delimiter the delimiter to use
Definition at line 803 of file keduvocdocument.cpp.
◆ setDocumentComment()
void KEduVocDocument::setDocumentComment | ( | const QString & | comment | ) |
Set the comment of the file.
- Parameters
-
comment comment to set
Definition at line 770 of file keduvocdocument.cpp.
◆ setGenerator()
void KEduVocDocument::setGenerator | ( | const QString & | generator | ) |
Sets the generator of the file.
- Parameters
-
generator name of the application generating the file
Definition at line 776 of file keduvocdocument.cpp.
◆ setIdentifier()
void KEduVocDocument::setIdentifier | ( | int | index, |
const KEduVocIdentifier & | lang ) |
Sets the identifier of translation.
- Parameters
-
index number of translation 0..x lang the language identifier: en=english, de=german, ...
Definition at line 615 of file keduvocdocument.cpp.
◆ setLicense()
void KEduVocDocument::setLicense | ( | const QString & | license | ) |
Set the license of the file.
- Parameters
-
license license to set
Definition at line 764 of file keduvocdocument.cpp.
◆ setModified()
void KEduVocDocument::setModified | ( | bool | dirty = true | ) |
Indicates if the document is modified.
- Parameters
-
dirty new state
Definition at line 190 of file keduvocdocument.cpp.
◆ setQueryIdentifier()
Sets the identifiers for the current query not written in the new version!
- Parameters
-
org identifier for original trans identifier for translation
Definition at line 757 of file keduvocdocument.cpp.
◆ setTitle()
void KEduVocDocument::setTitle | ( | const QString & | title | ) |
Set the title of the file.
- Parameters
-
title title to set
- Todo
- decouple document title and root lesson title
Definition at line 699 of file keduvocdocument.cpp.
◆ setUrl()
void KEduVocDocument::setUrl | ( | const QUrl & | url | ) |
◆ setVersion()
void KEduVocDocument::setVersion | ( | const QString & | ver | ) |
Sets version of the loaded file.
- Parameters
-
ver the new version
Definition at line 792 of file keduvocdocument.cpp.
◆ title()
QString KEduVocDocument::title | ( | ) | const |
- Returns
- the title of the file
Definition at line 691 of file keduvocdocument.cpp.
◆ toByteArray()
QByteArray KEduVocDocument::toByteArray | ( | const QString & | generator | ) |
returns a QByteArray KVTML2 representation of this doc
- Parameters
-
generator name of the application creating the QByteArray
- Returns
- KVTML2 XML
- Todo
- in new API if this should be part of save
Definition at line 376 of file keduvocdocument.cpp.
◆ url()
QUrl KEduVocDocument::url | ( | ) | const |
- Returns
- the URL of the XML file
Definition at line 681 of file keduvocdocument.cpp.
◆ version()
QString KEduVocDocument::version | ( | ) | const |
- Returns
- the version of the loaded file
Definition at line 787 of file keduvocdocument.cpp.
◆ wordTypeContainer()
KEduVocWordType * KEduVocDocument::wordTypeContainer | ( | ) |
Returns the root word type object.
- Returns
- pointer to the internal word type object
Definition at line 671 of file keduvocdocument.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:52:05 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.