Konsole::ColorScheme
#include <ColorScheme.h>
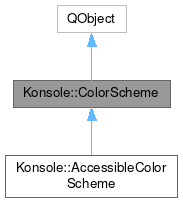
Signals | |
void | colorChanged (int index) |
Public Member Functions | |
ColorScheme (QObject *parent=nullptr) | |
QColor | backgroundColor () const |
ColorEntry | colorEntry (int index, uint randomSeed=0) const |
QString | description () const |
QColor | foregroundColor () const |
std::array< ColorEntry, TABLE_COLORS > | getColorTable (uint randomSeed=0) const |
bool | hasDarkBackground () const |
QString | name () const |
qreal | opacity () const |
bool | randomizedBackgroundColor () const |
void | read (const QString &filename) |
void | setColor (int index, QColor color) |
void | setColorTableEntry (int index, const ColorEntry &entry) |
void | setDescription (const QString &description) |
void | setName (const QString &name) |
void | setOpacity (qreal opacity) |
void | setRandomizedBackgroundColor (bool randomize) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QString | colorNameForIndex (int index) |
static QString | translatedColorNameForIndex (int index) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Represents a color scheme for a terminal display.
The color scheme includes the palette of colors used to draw the text and character backgrounds in the display and the opacity level of the display background.
Definition at line 55 of file ColorScheme.h.
Constructor & Destructor Documentation
◆ ColorScheme()
ColorScheme::ColorScheme | ( | QObject * | parent = nullptr | ) |
Constructs a new color scheme which is initialised to the default color set for Konsole.
Definition at line 113 of file ColorScheme.cpp.
◆ ~ColorScheme()
ColorScheme::~ColorScheme | ( | ) |
Definition at line 120 of file ColorScheme.cpp.
Member Function Documentation
◆ backgroundColor()
QColor ColorScheme::backgroundColor | ( | ) | const |
Convenience method.
Returns the background color for this scheme, this is the primary color used to draw the terminal background in this scheme.
Definition at line 253 of file ColorScheme.cpp.
◆ colorEntry()
ColorEntry ColorScheme::colorEntry | ( | int | index, |
uint | randomSeed = 0 ) const |
Retrieves a single color entry from the table.
See getColorTable()
Definition at line 168 of file ColorScheme.cpp.
◆ colorNameForIndex()
|
static |
Definition at line 323 of file ColorScheme.cpp.
◆ description()
QString ColorScheme::description | ( | ) | const |
Returns the descriptive name of the color scheme.
Definition at line 129 of file ColorScheme.cpp.
◆ foregroundColor()
QColor ColorScheme::foregroundColor | ( | ) | const |
Convenience method.
Returns the foreground color for this scheme, this is the primary color used to draw the text in this scheme.
Definition at line 248 of file ColorScheme.cpp.
◆ getColorTable()
std::array< ColorEntry, TABLE_COLORS > ColorScheme::getColorTable | ( | uint | randomSeed = 0 | ) | const |
Copies the color entries which form the palette for this color scheme into table
.
table
should be an array with TABLE_COLORS entries.
- Parameters
-
table Array into which the color entries for this color scheme are copied. randomSeed Color schemes may allow certain colors in their palette to be randomized. The seed is used to pick the random color.
Definition at line 195 of file ColorScheme.cpp.
◆ hasDarkBackground()
bool ColorScheme::hasDarkBackground | ( | ) | const |
Returns true if this color scheme has a dark background.
The background color is said to be dark if it has a value of less than 127 in the HSV color space.
Definition at line 258 of file ColorScheme.cpp.
◆ name()
QString ColorScheme::name | ( | ) | const |
Returns the name of the color scheme.
Definition at line 139 of file ColorScheme.cpp.
◆ opacity()
qreal ColorScheme::opacity | ( | ) | const |
Returns the opacity level for this color scheme, see setOpacity() TODO: More documentation.
Definition at line 270 of file ColorScheme.cpp.
◆ randomizedBackgroundColor()
bool ColorScheme::randomizedBackgroundColor | ( | ) | const |
Returns true if the background color is randomized.
Definition at line 205 of file ColorScheme.cpp.
◆ read()
void ColorScheme::read | ( | const QString & | filename | ) |
Definition at line 275 of file ColorScheme.cpp.
◆ setColor()
void ColorScheme::setColor | ( | int | index, |
QColor | color ) |
Definition at line 157 of file ColorScheme.cpp.
◆ setColorTableEntry()
void ColorScheme::setColorTableEntry | ( | int | index, |
const ColorEntry & | entry ) |
Sets a single entry within the color palette.
Definition at line 144 of file ColorScheme.cpp.
◆ setDescription()
void ColorScheme::setDescription | ( | const QString & | description | ) |
Sets the descriptive name of the color scheme.
Definition at line 124 of file ColorScheme.cpp.
◆ setName()
void ColorScheme::setName | ( | const QString & | name | ) |
Sets the name of the color scheme.
Definition at line 134 of file ColorScheme.cpp.
◆ setOpacity()
void ColorScheme::setOpacity | ( | qreal | opacity | ) |
Sets the opacity level of the display background.
opacity
ranges between 0 (completely transparent background) and 1 (completely opaque background).
Defaults to 1.
TODO: More documentation
Definition at line 265 of file ColorScheme.cpp.
◆ setRandomizedBackgroundColor()
void ColorScheme::setRandomizedBackgroundColor | ( | bool | randomize | ) |
Enables randomization of the background color.
This will cause the palette returned by getColorTable() and colorEntry() to be adjusted depending on the value of the random seed argument to them.
Definition at line 210 of file ColorScheme.cpp.
◆ translatedColorNameForIndex()
|
static |
Definition at line 329 of file ColorScheme.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:37 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.