DocumentHandler
#include <documenthandler.h>
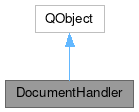
Properties | |
Alerts * | alerts |
Qt::Alignment | alignment |
bool | autoReload |
bool | autoSave |
QColor | backgroundColor |
bool | bold |
int | currentLineIndex |
int | cursorPosition |
QML_ELEMENTQQuickTextDocument * | document |
bool | enableSyntaxHighlighting |
bool | externallyModified |
QVariantMap | fileInfo |
QString | fileName |
QString | fileType |
QUrl | fileUrl |
bool | findCaseSensitively |
bool | findWholeWords |
QString | fontFamily |
int | fontSize |
QString | formatName |
bool | isRich = false |
bool | italic |
int | lineCount |
bool | modified |
int | selectionEnd |
int | selectionStart |
qreal | tabSpace |
QString | text |
QColor | textColor |
QString | theme |
bool | underline |
bool | uppercase |
![]() | |
objectName | |
Signals | |
void | alignmentChanged () |
void | autoReloadChanged () |
void | autoSaveChanged () |
void | backgroundColorChanged () |
void | boldChanged () |
void | currentLineIndexChanged () |
void | cursorPositionChanged () |
void | documentChanged () |
void | enableSyntaxHighlightingChanged () |
void | error (const QString &message) |
void | externallyModifiedChanged () |
void | fileInfoChanged () |
void | fileSaved () |
void | fileUrlChanged () |
void | findCaseSensitivelyChanged () |
void | findWholeWordsChanged () |
void | fontFamilyChanged () |
void | fontSizeChanged () |
void | formatNameChanged () |
void | isRichChanged () |
void | italicChanged () |
void | lineCountChanged () |
void | loaded (const QUrl &url) |
void | loadFile (QUrl url) |
void | modifiedChanged () |
void | searchFound (int start, int end) |
void | selectionEndChanged () |
void | selectionStartChanged () |
void | tabSpaceChanged () |
void | textChanged () |
void | textColorChanged () |
void | themeChanged () |
void | underlineChanged () |
void | uppercaseChanged () |
Public Slots | |
void | find (const QString &query, const bool &forward=true) |
int | getCurrentLineIndex () |
static const QString | getLanguageNameFromFileName (const QUrl &fileName) |
static const QStringList | getLanguageNameList () |
int | goToLine (const int &line) |
bool | isFoldable (const int &line) const |
bool | isFolded (const int &line) const |
int | lineCount () |
int | lineHeight (const int &line) |
void | replace (const QString &query, const QString &value) |
void | replaceAll (const QString &query, const QString &value) |
void | saveAs (const QUrl &url) |
void | toggleFold (const int &line) |
Public Member Functions | |
Qt::Alignment | alignment () const |
bool | autoSave () const |
bool | bold () const |
int | cursorPosition () const |
QQuickTextDocument * | document () const |
bool | enableSyntaxHighlighting () const |
QVariantMap | fileInfo () const |
QString | fileName () const |
QString | fileType () const |
QUrl | fileUrl () const |
QString | fontFamily () const |
int | fontSize () const |
QString | formatName () const |
Alerts * | getAlerts () const |
bool | getAutoReload () const |
QColor | getBackgroundColor () const |
bool | getExternallyModified () const |
bool | getIsRich () const |
bool | getModified () const |
bool | italic () const |
int | selectionEnd () const |
int | selectionStart () const |
void | setAlignment (Qt::Alignment alignment) |
void | setAutoReload (const bool &value) |
void | setAutoSave (const bool &value) |
void | setBackgroundColor (const QColor &color) |
void | setBold (bool bold) |
void | setCursorPosition (int position) |
void | setDocument (QQuickTextDocument *document) |
void | setEnableSyntaxHighlighting (const bool &value) |
void | setExternallyModified (const bool &value) |
void | setFileUrl (const QUrl &url) |
void | setFontFamily (const QString &family) |
void | setFontSize (int size) |
void | setFormatName (const QString &formatName) |
void | setItalic (bool italic) |
void | setSelectionEnd (int position) |
void | setSelectionStart (int position) |
void | setTabSpace (qreal value) |
void | setText (const QString &text) |
void | setTextColor (const QColor &color) |
void | setTheme (const QString &theme) |
void | setUnderline (bool underline) |
void | setUppercase (bool uppercase) |
qreal | tabSpace () const |
QString | text () const |
QColor | textColor () const |
QString | theme () const |
bool | underline () const |
bool | uppercase () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static bool | isDark (const QColor &color) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The DocumentHandler class.
- Note
- This is not part of any public API, and can it is only exposed as part of the TextEditor control.
Definition at line 238 of file documenthandler.h.
Property Documentation
◆ alerts
|
read |
Definition at line 375 of file documenthandler.h.
◆ alignment
|
readwrite |
Definition at line 275 of file documenthandler.h.
◆ autoReload
|
readwrite |
Definition at line 355 of file documenthandler.h.
◆ autoSave
|
readwrite |
Definition at line 360 of file documenthandler.h.
◆ backgroundColor
|
readwrite |
Definition at line 380 of file documenthandler.h.
◆ bold
|
readwrite |
Definition at line 280 of file documenthandler.h.
◆ currentLineIndex
|
read |
Definition at line 370 of file documenthandler.h.
◆ cursorPosition
|
readwrite |
Definition at line 250 of file documenthandler.h.
◆ document
|
readwrite |
Definition at line 245 of file documenthandler.h.
◆ enableSyntaxHighlighting
|
readwrite |
Definition at line 390 of file documenthandler.h.
◆ externallyModified
|
read |
Definition at line 345 of file documenthandler.h.
◆ fileInfo
|
read |
Definition at line 330 of file documenthandler.h.
◆ fileName
|
read |
Definition at line 320 of file documenthandler.h.
◆ fileType
|
read |
Definition at line 325 of file documenthandler.h.
◆ fileUrl
|
readwrite |
Definition at line 335 of file documenthandler.h.
◆ findCaseSensitively
bool DocumentHandler::findCaseSensitively |
Definition at line 400 of file documenthandler.h.
◆ findWholeWords
bool DocumentHandler::findWholeWords |
Definition at line 395 of file documenthandler.h.
◆ fontFamily
|
readwrite |
Definition at line 270 of file documenthandler.h.
◆ fontSize
|
readwrite |
Definition at line 310 of file documenthandler.h.
◆ formatName
|
readwrite |
Definition at line 365 of file documenthandler.h.
◆ isRich
|
read |
Definition at line 300 of file documenthandler.h.
◆ italic
|
readwrite |
Definition at line 290 of file documenthandler.h.
◆ lineCount
|
read |
Definition at line 305 of file documenthandler.h.
◆ modified
|
read |
Definition at line 350 of file documenthandler.h.
◆ selectionEnd
|
readwrite |
Definition at line 260 of file documenthandler.h.
◆ selectionStart
|
readwrite |
Definition at line 255 of file documenthandler.h.
◆ tabSpace
|
readwrite |
Definition at line 315 of file documenthandler.h.
◆ text
|
readwrite |
Definition at line 340 of file documenthandler.h.
◆ textColor
|
readwrite |
Definition at line 265 of file documenthandler.h.
◆ theme
|
readwrite |
Definition at line 385 of file documenthandler.h.
◆ underline
|
readwrite |
Definition at line 295 of file documenthandler.h.
◆ uppercase
|
readwrite |
Definition at line 285 of file documenthandler.h.
Member Function Documentation
◆ alignment()
Qt::Alignment DocumentHandler::alignment | ( | ) | const |
◆ autoSave()
bool DocumentHandler::autoSave | ( | ) | const |
◆ bold()
bool DocumentHandler::bold | ( | ) | const |
◆ cursorPosition()
int DocumentHandler::cursorPosition | ( | ) | const |
◆ document()
QQuickTextDocument * DocumentHandler::document | ( | ) | const |
◆ enableSyntaxHighlighting()
bool DocumentHandler::enableSyntaxHighlighting | ( | ) | const |
◆ fileInfo()
QVariantMap DocumentHandler::fileInfo | ( | ) | const |
◆ fileName()
QString DocumentHandler::fileName | ( | ) | const |
◆ fileType()
QString DocumentHandler::fileType | ( | ) | const |
◆ fileUrl()
QUrl DocumentHandler::fileUrl | ( | ) | const |
◆ find
|
slot |
◆ fontFamily()
QString DocumentHandler::fontFamily | ( | ) | const |
◆ fontSize()
int DocumentHandler::fontSize | ( | ) | const |
◆ formatName()
QString DocumentHandler::formatName | ( | ) | const |
◆ getAlerts()
Alerts * DocumentHandler::getAlerts | ( | ) | const |
◆ getAutoReload()
bool DocumentHandler::getAutoReload | ( | ) | const |
◆ getBackgroundColor()
QColor DocumentHandler::getBackgroundColor | ( | ) | const |
◆ getCurrentLineIndex
|
slot |
◆ getExternallyModified()
bool DocumentHandler::getExternallyModified | ( | ) | const |
◆ getIsRich()
bool DocumentHandler::getIsRich | ( | ) | const |
◆ getLanguageNameFromFileName
getLanguageNameFromFileName
- Parameters
-
fileName
- Returns
Definition at line 809 of file documenthandler.cpp.
◆ getLanguageNameList
|
staticslot |
◆ getModified()
bool DocumentHandler::getModified | ( | ) | const |
◆ goToLine
|
slot |
Definition at line 1104 of file documenthandler.cpp.
◆ isDark()
|
inlinestatic |
◆ isFoldable
|
slot |
Definition at line 1003 of file documenthandler.cpp.
◆ isFolded
|
slot |
Definition at line 1016 of file documenthandler.cpp.
◆ italic()
bool DocumentHandler::italic | ( | ) | const |
◆ lineHeight
|
slot |
◆ replace
Definition at line 935 of file documenthandler.cpp.
◆ replaceAll
Definition at line 958 of file documenthandler.cpp.
◆ saveAs
|
slot |
◆ selectionEnd()
int DocumentHandler::selectionEnd | ( | ) | const |
◆ selectionStart()
int DocumentHandler::selectionStart | ( | ) | const |
◆ setAlignment()
void DocumentHandler::setAlignment | ( | Qt::Alignment | alignment | ) |
◆ setAutoReload()
void DocumentHandler::setAutoReload | ( | const bool & | value | ) |
◆ setAutoSave()
void DocumentHandler::setAutoSave | ( | const bool & | value | ) |
◆ setBackgroundColor()
void DocumentHandler::setBackgroundColor | ( | const QColor & | color | ) |
◆ setBold()
void DocumentHandler::setBold | ( | bool | bold | ) |
◆ setCursorPosition()
void DocumentHandler::setCursorPosition | ( | int | position | ) |
◆ setDocument()
void DocumentHandler::setDocument | ( | QQuickTextDocument * | document | ) |
◆ setEnableSyntaxHighlighting()
void DocumentHandler::setEnableSyntaxHighlighting | ( | const bool & | value | ) |
◆ setExternallyModified()
void DocumentHandler::setExternallyModified | ( | const bool & | value | ) |
◆ setFileUrl()
void DocumentHandler::setFileUrl | ( | const QUrl & | url | ) |
◆ setFontFamily()
void DocumentHandler::setFontFamily | ( | const QString & | family | ) |
◆ setFontSize()
void DocumentHandler::setFontSize | ( | int | size | ) |
◆ setFormatName()
void DocumentHandler::setFormatName | ( | const QString & | formatName | ) |
◆ setItalic()
void DocumentHandler::setItalic | ( | bool | italic | ) |
◆ setSelectionEnd()
void DocumentHandler::setSelectionEnd | ( | int | position | ) |
◆ setSelectionStart()
void DocumentHandler::setSelectionStart | ( | int | position | ) |
◆ setTabSpace()
void DocumentHandler::setTabSpace | ( | qreal | value | ) |
Definition at line 671 of file documenthandler.cpp.
◆ setText()
void DocumentHandler::setText | ( | const QString & | text | ) |
◆ setTextColor()
void DocumentHandler::setTextColor | ( | const QColor & | color | ) |
◆ setTheme()
void DocumentHandler::setTheme | ( | const QString & | theme | ) |
◆ setUnderline()
void DocumentHandler::setUnderline | ( | bool | underline | ) |
◆ setUppercase()
void DocumentHandler::setUppercase | ( | bool | uppercase | ) |
◆ tabSpace()
qreal DocumentHandler::tabSpace | ( | ) | const |
Definition at line 688 of file documenthandler.cpp.
◆ text()
|
inline |
◆ textColor()
QColor DocumentHandler::textColor | ( | ) | const |
◆ theme()
QString DocumentHandler::theme | ( | ) | const |
◆ toggleFold
|
slot |
Definition at line 1039 of file documenthandler.cpp.
◆ underline()
bool DocumentHandler::underline | ( | ) | const |
◆ uppercase()
bool DocumentHandler::uppercase | ( | ) | const |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:33 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.