Plasma::Types
#include <Plasma/Plasma>
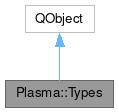
Public Types | |
typedef QFlags< BackgroundHints > | BackgroundFlags |
enum | BackgroundHints { NoBackground = 0 , StandardBackground = 1 , TranslucentBackground = 2 , ShadowBackground = 4 , ConfigurableBackground = 8 , DefaultBackground = StandardBackground } |
enum | ContainmentDisplayHint { NoContainmentDisplayHint = 0 , ContainmentDrawsPlasmoidHeading , ContainmentForcesSquarePlasmoids , ContainmentPrefersOpaqueBackground = 4 , ContainmentPrefersFloatingApplets = 8 } |
typedef QFlags< ContainmentDisplayHint > | ContainmentDisplayHints |
enum | FormFactor { Planar = 0 , MediaCenter , Horizontal , Vertical , Application } |
enum | ImmutabilityType { Mutable = 1 , UserImmutable = 2 , SystemImmutable = 4 } |
enum | ItemStatus { UnknownStatus = 0 , PassiveStatus = 1 , ActiveStatus = 2 , NeedsAttentionStatus = 3 , RequiresAttentionStatus = 4 , AcceptingInputStatus = 5 , HiddenStatus = 6 } |
enum | Location { Floating = 0 , Desktop , FullScreen , TopEdge , BottomEdge , LeftEdge , RightEdge } |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Member Typedef Documentation
◆ BackgroundFlags
typedef QFlags< BackgroundHints > Plasma::Types::BackgroundFlags |
◆ ContainmentDisplayHints
Member Enumeration Documentation
◆ BackgroundHints
Description on how draw a background for the applet.
◆ ContainmentDisplayHint
Display hints that come from the containment that suggest the applet how to look and behave.
- Since
- 5.77
◆ FormFactor
The FormFactor enumeration describes how a Plasma::Applet should arrange itself.
The value is derived from the container managing the Applet (e.g. in Plasma, a Corona on the desktop or on a panel).
Enumerator | |
---|---|
Planar | The applet lives in a plane and has two degrees of freedom to grow. Optimize for desktop, laptop or tablet usage: a high resolution screen 1-3 feet distant from the viewer. |
MediaCenter | As with Planar, the applet lives in a plane but the interface should be optimized for medium-to-high resolution screens that are 5-15 feet distant from the viewer. Sometimes referred to as a "ten foot interface". |
Horizontal | The applet is constrained vertically, but can expand horizontally. |
Vertical | The applet is constrained horizontally, but can expand vertically. |
Application | The Applet lives in a plane and should be optimized to look as a full application, for the desktop or the particular device. |
◆ ImmutabilityType
Defines the immutability of items like applets, corona and containments they can be free to modify, locked down by the user or locked down by the system (e.g.
kiosk setups).
◆ ItemStatus
Status of an applet.
- Since
- 4.3
Enumerator | |
---|---|
UnknownStatus | The status is unknown. |
PassiveStatus | The Item is passive. |
ActiveStatus | The Item is active. |
NeedsAttentionStatus | The Item needs attention. |
RequiresAttentionStatus | The Item needs persistent attention. |
AcceptingInputStatus | The Item is accepting input. |
HiddenStatus | The Item will be hidden totally |
◆ Location
The Location enumeration describes where on screen an element, such as an Applet or its managing container, is positioned on the screen.
Constructor & Destructor Documentation
◆ ~Types()
|
override |
Definition at line 22 of file plasma.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri May 3 2024 11:46:04 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.