Plasma::Applet
#include <Plasma/Applet>
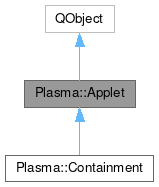
Public Types | |
enum | Constraint { NoConstraint = 0 , FormFactorConstraint = 1 , LocationConstraint = 2 , ScreenConstraint = 4 , ImmutableConstraint = 8 , StartupCompletedConstraint = 16 , UiReadyConstraint = 32 , AllConstraints = FormFactorConstraint | LocationConstraint | ScreenConstraint | ImmutableConstraint } |
enum | ConstraintHint { NoHint = 0 , CanFillArea = 1 , MarginAreasSeparator = CanFillArea | 2 } |
typedef QFlags< ConstraintHint > | ConstraintHints |
typedef QFlags< Constraint > | Constraints |
Public Slots | |
virtual void | configChanged () |
void | destroy () |
void | flushPendingConstraintsEvents () |
virtual void | init () |
void | setImmutability (const Types::ImmutabilityType immutable) |
void | setLaunchErrorMessage (const QString &reason=QString()) |
void | setStatus (const Types::ItemStatus stat) |
Protected Member Functions | |
virtual void | constraintsEvent (Constraints constraints) |
virtual void | saveState (KConfigGroup &config) const |
void | timerEvent (QTimerEvent *event) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
The base Applet class.
Applet provides several important roles for add-ons widgets in Plasma.
First, it is the base class for the plugin system and therefore is the interface to applets for host applications. Background painting (allowing for consistent and complex look and feel in just one line of code for applets), loading and starting of scripting support for each applet, providing access to the associated plasmoid package (if any) and access to configuration data.
See techbase.kde.org for tutorials on writing Applets using this class.
Member Typedef Documentation
◆ ConstraintHints
typedef QFlags< ConstraintHint > Plasma::Applet::ConstraintHints |
◆ Constraints
typedef QFlags< Constraint > Plasma::Applet::Constraints |
Member Enumeration Documentation
◆ Constraint
The Constraint enumeration lists the various constraints that Plasma objects have managed for them and which they may wish to react to, for instance in Applet::constraintsUpdated.
◆ ConstraintHint
This enumeration lists the various hints that an applet can pass to its constraint regarding the way that it is represented.
Enumerator | |
---|---|
CanFillArea | The CompactRepresentation can fill the area and ignore constraint margins. |
MarginAreasSeparator | The applet acts as a separator between the standard and slim panel margin areas. |
Property Documentation
◆ backgroundHints
|
readwrite |
◆ busy
|
readwrite |
◆ configuration
|
read |
A KConfigPropertyMap instance that represents the configuration which is usable from QML to read and write settings like any JavaScript Object.
◆ configurationRequired
|
readwrite |
◆ constraintHints
|
readwrite |
◆ containment
|
read |
The Containment managing this applet.
◆ containmentDisplayHints
|
read |
◆ contextualActions
◆ effectiveBackgroundHints
|
read |
◆ formFactor
|
read |
◆ globalShortcut
|
readwrite |
◆ hasConfigurationInterface
|
readwrite |
◆ icon
|
readwrite |
◆ id
|
read |
◆ immutability
|
readwrite |
◆ immutable
|
read |
◆ isContainment
|
read |
True if this applet is a Containment and is acting as one, such as a desktop or a panel.
◆ location
|
read |
The location of the scene which is displaying applet.
- See also
- Plasma::Types::Location
◆ metaData
|
read |
◆ pluginName
|
read |
◆ status
|
readwrite |
◆ title
|
readwrite |
◆ userBackgroundHints
|
readwrite |
◆ userConfiguring
|
read |
Constructor & Destructor Documentation
◆ Applet()
Plasma::Applet::Applet | ( | QObject * | parentObject, |
const KPluginMetaData & | data, | ||
const QVariantList & | args ) |
This constructor can be used with the KCoreAddons plugin loading system.
The argument list is expected to have contain the KPackage of the applet, the meta data file path (for compatibility) and an applet ID which must be a base 10 number.
- Parameters
-
parent a QObject parent; you probably want to pass in 0 data,KPluginMetaData used to create this plugin args a list of strings containing the applet id @Since 5.86
Definition at line 41 of file applet.cpp.
◆ ~Applet()
|
override |
Definition at line 62 of file applet.cpp.
Member Function Documentation
◆ activated
|
signal |
Emitted when activation is requested due to, for example, a global keyboard shortcut.
By default the widget is given focus.
◆ appletDeleted
|
signal |
Emitted when the applet is deleted.
◆ backgroundHints()
Plasma::Types::BackgroundHints Plasma::Applet::backgroundHints | ( | ) | const |
How the applet wants its background to be drawn.
The containment may chose to ignore this hint.
- Since
- 5.65
Definition at line 345 of file applet.cpp.
◆ backgroundHintsChanged
|
signal |
Emitted when the background hints have changed.
- Since
- 5.65
◆ busyChanged
|
signal |
Emitted when the busy status has changed.
- Since
- 5.21
◆ config()
KConfigGroup Plasma::Applet::config | ( | ) | const |
Returns the KConfigGroup to access the applets configuration.
This config object will write to an instance specific config file named <appletname><instanceid>rc in the Plasma appdata directory.
Definition at line 189 of file applet.cpp.
◆ configChanged
|
virtualslot |
Called when applet configuration values have changed.
Definition at line 832 of file applet.cpp.
◆ configNeedsSaving
|
signal |
Emitted when an applet has changed values in its configuration and wishes for them to be saved at the next save point.
As this implies disk activity, this signal should be used with care.
- Note
- This does not need to be emitted from saveState by individual applets.
◆ configScheme()
KConfigLoader * Plasma::Applet::configScheme | ( | ) | const |
Returns the config skeleton object from this applet's package, if any.
- Returns
- config skeleton object, or 0 if none
Definition at line 248 of file applet.cpp.
◆ configuration()
KConfigPropertyMap * Plasma::Applet::configuration | ( | ) |
- Returns
- a KConfigPropertyMap instance that represents the configuration which is usable from QML to read and write settings like any JavaScript Object
◆ configurationRequired()
bool Plasma::Applet::configurationRequired | ( | ) | const |
- Returns
- true if the applet currently needs to be configured, otherwise, false
Definition at line 477 of file applet.cpp.
◆ configurationRequiredChanged
|
signal |
◆ configurationRequiredReason()
QString Plasma::Applet::configurationRequiredReason | ( | ) | const |
- Returns
- A translated message for the user explaining that the applet needs configuring; this should note what needs to be configured
- See also
- setConfigurationRequired
- Since
- 5.20
Definition at line 482 of file applet.cpp.
◆ constraintHints()
Applet::ConstraintHints Plasma::Applet::constraintHints | ( | ) | const |
- Returns
- The constraint hints such as CanFillArea or MarginAreasSeparator, they can be in bitwise OR
Definition at line 509 of file applet.cpp.
◆ constraintHintsChanged
|
signal |
Emitted when the constraint hints changed.
- See also
- setConstraintHints
◆ constraintsEvent()
|
protectedvirtual |
Definition at line 281 of file applet.cpp.
◆ containment()
Containment * Plasma::Applet::containment | ( | ) | const |
- Returns
- the Containment, if any, this applet belongs to. A containment will return itself if is a first level containment such as a desktop or a panel, or will return the other containment is in if it's a nested containment such a system tray
Definition at line 724 of file applet.cpp.
◆ containmentChanged
|
signal |
Emitted when the containment changes.
◆ containmentDisplayHints()
Types::ContainmentDisplayHints Plasma::Applet::containmentDisplayHints | ( | ) | const |
- Returns
- Display hints that come from the containment that suggest the applet how to look and behave.
- Since
- 5.77
Definition at line 717 of file applet.cpp.
◆ containmentDisplayHintsChanged
|
signal |
Emitted when the containment display hints change.
◆ contextualActions()
Returns a list of context-related QAction instances.
This is used e.g. within the DesktopView to display a contextmenu.
- Returns
- A list of actions. The default implementation returns an empty list.
◆ contextualActionsAboutToShow
|
signal |
Emitted just before the contextual actions are about to show For instance just before the context menu containing the actions added with setAction() is shown.
◆ contextualActionsChanged
Emitted when the list of contextual actions has changed.
◆ destroy
|
slot |
Destroys the applet; it will be removed nicely and deleted.
Its configuration will also be deleted. If you want to remove the Applet configuration, use this, don't just delete the Applet *
Definition at line 222 of file applet.cpp.
◆ destroyed()
bool Plasma::Applet::destroyed | ( | ) | const |
- Returns
- true if destroy() was called; useful for Applets which should avoid certain tasks if they are about to be deleted permanently
Definition at line 243 of file applet.cpp.
◆ destroyedChanged
|
signal |
Emitted when the applet has been scheduled for destruction or the destruction has been undone.
- Since
- 5.4
◆ effectiveBackgroundHints()
Plasma::Types::BackgroundHints Plasma::Applet::effectiveBackgroundHints | ( | ) | const |
The effective background hints the applet will have: it will follow userBackgroundHints only if backgroundHints has the Plasma::Types::ConfigurableBackground flag set.
- Since
- 5.65
Definition at line 366 of file applet.cpp.
◆ effectiveBackgroundHintsChanged
|
signal |
Emitted when the effective background hints have changed.
- Since
- 5.65
◆ failedToLaunch()
bool Plasma::Applet::failedToLaunch | ( | ) | const |
If for some reason, the applet fails to get up on its feet (the library couldn't be loaded, necessary hardware support wasn't found, etc..) this method returns true.
Definition at line 472 of file applet.cpp.
◆ flushPendingConstraintsEvents
|
slot |
Sends all pending constraints updates to the applet.
Will usually be called automatically, but can also be called manually if needed.
Definition at line 543 of file applet.cpp.
◆ formFactor()
Types::FormFactor Plasma::Applet::formFactor | ( | ) | const |
Returns the current form factor the applet is being displayed in.
- See also
- Plasma::FormFactor
Definition at line 696 of file applet.cpp.
◆ formFactorChanged
|
signal |
Emitted when the formfactor changes.
◆ globalConfig()
KConfigGroup Plasma::Applet::globalConfig | ( | ) | const |
Returns a KConfigGroup object to be shared by all applets of this type.
This config object will write to an applet-specific config object named plasma_<appletname>rc in the local config directory.
Definition at line 202 of file applet.cpp.
◆ globalShortcut()
QKeySequence Plasma::Applet::globalShortcut | ( | ) | const |
- Returns
- the global shortcut associated with this widget, or an empty shortcut if no global shortcut is associated.
Definition at line 774 of file applet.cpp.
◆ globalShortcutChanged
|
signal |
Emitted when the global shortcut to activate this applet has chanaged.
◆ hasConfigurationInterface()
bool Plasma::Applet::hasConfigurationInterface | ( | ) | const |
- Returns
- true if this plasmoid provides a GUI configuration
Definition at line 807 of file applet.cpp.
◆ hasConfigurationInterfaceChanged
|
signal |
Emitted when the applet gains or loses the ability to show a configuration interface.
- See also
- hasConfigurationInterface
- Since
- 6.0
◆ icon()
QString Plasma::Applet::icon | ( | ) | const |
- Returns
- The icon name related to this applet By default is the one in the plasmoid desktop file
Definition at line 315 of file applet.cpp.
◆ iconChanged
|
signal |
Emitted when the icon name for the applet has changed.
- Since
- 5.20
◆ id()
uint Plasma::Applet::id | ( | ) | const |
- Returns
- the id of this applet
Definition at line 92 of file applet.cpp.
◆ immutability()
Types::ImmutabilityType Plasma::Applet::immutability | ( | ) | const |
- Returns
- The type of immutability of this applet
Definition at line 411 of file applet.cpp.
◆ immutabilityChanged
|
signal |
Emitted when the immutability changes.
- Since
- 4.4
◆ immutable()
bool Plasma::Applet::immutable | ( | ) | const |
- Returns
- true if immutability() is not Types::Mutable
Definition at line 462 of file applet.cpp.
◆ init
|
virtualslot |
This method is called once the applet is loaded and added to a Corona.
If the applet requires a Scene or has an particularly intensive set of initialization routines to go through, consider implementing it in this method instead of the constructor.
Note: paintInterface may get called before init() depending on initialization order. Painting is managed by the canvas (QGraphisScene), and may schedule a paint event prior to init() being called.
Reimplemented in Plasma::Containment.
Definition at line 87 of file applet.cpp.
◆ internalAction()
- Returns
- the internal action with the given name if available
- Parameters
-
name the unique name of the action we want
Definition at line 672 of file applet.cpp.
◆ internalActions()
- Returns
- All the internal actions such as configure, remove, alternatives etc
Definition at line 691 of file applet.cpp.
◆ internalActionsChanged
Emitted when the list of internal actions has changed.
◆ isBusy()
bool Plasma::Applet::isBusy | ( | ) | const |
- Returns
- true if the applet should show a busy status, for instance doing some network operation
- Since
- 5.21
Definition at line 330 of file applet.cpp.
◆ isContainment()
bool Plasma::Applet::isContainment | ( | ) | const |
- Returns
- true if this Applet is currently being used as a Containment, false otherwise. Normally only first level Containments directly children of Corona can act as containments, except Containments of Type CustomEmbedded which can be containments also when inside another containment, such as a Systray inside a Panel.
Definition at line 897 of file applet.cpp.
◆ isUserConfiguring()
bool Plasma::Applet::isUserConfiguring | ( | ) | const |
- Returns
- true when the configuration interface is being shown
- Since
- 4.5
Definition at line 514 of file applet.cpp.
◆ launchErrorMessage()
QString Plasma::Applet::launchErrorMessage | ( | ) | const |
If for some reason, the applet fails to get up on its feet (the library couldn't be loaded, necessary hardware support wasn't found, etc..) this method returns the reason why, in an user-readable way.
- Since
- 5.0
Definition at line 467 of file applet.cpp.
◆ location()
Types::Location Plasma::Applet::location | ( | ) | const |
Returns the location of the scene which is displaying applet.
- See also
- Plasma::Types::Location
Definition at line 786 of file applet.cpp.
◆ locationChanged
|
signal |
Emitted when the location changes.
◆ pluginMetaData()
KPluginMetaData Plasma::Applet::pluginMetaData | ( | ) | const |
◆ pluginName()
QString Plasma::Applet::pluginName | ( | ) | const |
- Returns
- the plugin name form KPluginMetaData
Definition at line 406 of file applet.cpp.
◆ qmlContextualActions()
QQmlListProperty< QAction > Plasma::Applet::qmlContextualActions | ( | ) |
Definition at line 638 of file applet.cpp.
◆ removeInternalAction()
void Plasma::Applet::removeInternalAction | ( | const QString & | name | ) |
Removes an action from the internal actions.
- Parameters
-
name the action to be removed
Definition at line 677 of file applet.cpp.
◆ restore()
|
virtual |
Restores state information about this applet saved previously in save(KConfigGroup&).
This method does not need to be reimplemented by Applet subclasses, but can be useful for Applet specializations (such as Containment) to do so.
Reimplemented in Plasma::Containment.
Definition at line 135 of file applet.cpp.
◆ save()
|
virtual |
Saves state information about this applet that will be accessed when next instantiated in the restore(KConfigGroup&) method.
This method does not need to be reimplemented by Applet subclasses, but can be useful for Applet specializations (such as Containment) to do so.
Applet subclasses may instead want to reimplement saveState().
Reimplemented in Plasma::Containment.
Definition at line 102 of file applet.cpp.
◆ saveState()
|
protectedvirtual |
When called, the Applet should write any information needed as part of the Applet's running state to the configuration object in config() and/or globalConfig().
Applets that always sync their settings/state with the config objects when these settings/states change do not need to reimplement this method.
Definition at line 179 of file applet.cpp.
◆ secondaryActivated
|
signal |
Emitted when activation is requested due to, for example, middle click.
- Since
- 6.3
◆ setBackgroundHints()
void Plasma::Applet::setBackgroundHints | ( | Plasma::Types::BackgroundHints | hint | ) |
Sets the applet background hints.
Only Applet implementations should write this property
- Since
- 5.65
Definition at line 350 of file applet.cpp.
◆ setBusy()
void Plasma::Applet::setBusy | ( | bool | busy | ) |
Sets the Applet to have a busy status hint, for instance the applet doing some network operation.
The graphical representation of the busy status depends completely from the visualization.
- Parameters
-
busy true if the applet is busy
- Since
- 5.21
Definition at line 335 of file applet.cpp.
◆ setConfigurationRequired()
void Plasma::Applet::setConfigurationRequired | ( | bool | needsConfiguring, |
const QString & | reason = QString() ) |
When the applet needs to be configured before being usable, this method can be called to show a standard interface prompting the user to configure the applet.
- Parameters
-
needsConfiguring true if the applet needs to be configured, or false if it doesn't reason a translated message for the user explaining that the applet needs configuring; this should note what needs to be configured
Definition at line 487 of file applet.cpp.
◆ setConstraintHints()
void Plasma::Applet::setConstraintHints | ( | ConstraintHints | constraintHints | ) |
Sets the constraint hits which give a more granular control over sizing in constrained layouts such as panels.
- Parameters
-
constraintHints such as CanFillArea or MarginAreasSeparator, they can be in bitwise OR
Definition at line 499 of file applet.cpp.
◆ setGlobalShortcut()
void Plasma::Applet::setGlobalShortcut | ( | const QKeySequence & | shortcut = QKeySequence() | ) |
Sets the global shortcut to associate with this widget.
Definition at line 748 of file applet.cpp.
◆ setHasConfigurationInterface()
void Plasma::Applet::setHasConfigurationInterface | ( | bool | hasInterface | ) |
Sets whether or not this applet provides a user interface for configuring the applet.
It defaults to false, and if true is passed in you should also reimplement createConfigurationInterface()
- Parameters
-
hasInterface whether or not there is a user interface available
Definition at line 812 of file applet.cpp.
◆ setIcon()
void Plasma::Applet::setIcon | ( | const QString & | icon | ) |
Sets an icon name for this applet.
- Parameters
-
icon Freedesktop compatible icon name
Definition at line 320 of file applet.cpp.
◆ setImmutability
|
slot |
Sets the immutability type for this applet (not immutable, user immutable or system immutable)
- Parameters
-
immutable the new immutability type of this applet
Definition at line 448 of file applet.cpp.
◆ setInternalAction()
Add a new internal action.
if an internal action with the same name already exists, it will be replaced with this new one. Those are usually actions defined by the system, such as "configure" and "remove"
- Parameters
-
name The unique name for the action action The new QAction to be added
Definition at line 650 of file applet.cpp.
◆ setLaunchErrorMessage
Call this method when the applet fails to launch properly.
An optional reason can be provided.
Not that all children items will be deleted when this method is called. If you have pointers to these items, you will need to reset them after calling this method.
- Parameters
-
failed true when the applet failed, false when it succeeded reason an optional reason to show the user why the applet failed to launch
- Since
- 5.0
Definition at line 169 of file applet.cpp.
◆ setStatus
|
slot |
◆ setTitle()
void Plasma::Applet::setTitle | ( | const QString & | title | ) |
Sets a custom title for this instance of the applet.
E.g. a clock might use the timezone as its name rather than the .desktop file
- Since
- 5.0
- Parameters
-
title the user-visible title for the applet.
Definition at line 305 of file applet.cpp.
◆ setUserBackgroundHints()
void Plasma::Applet::setUserBackgroundHints | ( | Plasma::Types::BackgroundHints | hint | ) |
Sets the hints the user wished the background style for the applet to be.
- Since
- 5.65
Definition at line 380 of file applet.cpp.
◆ setUserConfiguring()
void Plasma::Applet::setUserConfiguring | ( | bool | configuring | ) |
Tells the applet the user is configuring.
- Parameters
-
configuring true if the configuration ui is showing
Definition at line 519 of file applet.cpp.
◆ startupArguments()
QVariantList Plasma::Applet::startupArguments | ( | ) | const |
- Returns
- the arguments this applet was started with. Some applets support arguments, for instance the notes applet supports to be instantiated with a given text already passed as paramenter
Definition at line 97 of file applet.cpp.
◆ status()
Types::ItemStatus Plasma::Applet::status | ( | ) | const |
◆ statusChanged
|
signal |
Emitted when the applet status changes.
- Since
- 4.4
◆ timerEvent()
|
overrideprotectedvirtual |
◆ title()
QString Plasma::Applet::title | ( | ) | const |
Returns the user-visible title for the applet, as specified in the Name field of the .desktop file.
Can be changed with
- See also
- setTitle
- Since
- 5.0
- Returns
- the user-visible title for the applet.
Definition at line 292 of file applet.cpp.
◆ titleChanged
|
signal |
Emitted when the title has changed.
- Since
- 5.20
◆ translationDomain()
QString Plasma::Applet::translationDomain | ( | ) | const |
◆ updateConstraints()
void Plasma::Applet::updateConstraints | ( | Constraints | constraints = AllConstraints | ) |
Called when any of the geometry constraints have been updated.
This method calls constraintsEvent, which may be reimplemented, once the Applet has been prepared for updating the constraints.
- Parameters
-
constraints the type of constraints that were updated
Definition at line 276 of file applet.cpp.
◆ userBackgroundHints()
Plasma::Types::BackgroundHints Plasma::Applet::userBackgroundHints | ( | ) | const |
The containment (and/or the user) may decide to use another kind of background instead if supported by the applet.
In order for an applet to support user configuration of the background, it needs to have the Plasma::Types::ConfigurableBackground flag set in its backgroundHints
- Since
- 5.65
Definition at line 375 of file applet.cpp.
◆ userBackgroundHintsChanged
|
signal |
Emitted when the user background hints have changed.
- Since
- 5.65
◆ userConfiguringChanged
|
signal |
emitted when the config ui appears or disappears
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:56:56 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.