KConfigLoader
#include <KConfigLoader>
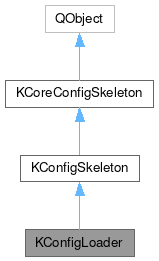
Public Member Functions | |
KConfigLoader (const KConfigGroup &config, QIODevice *xml, QObject *parent=nullptr) | |
KConfigLoader (const QString &configFile, QIODevice *xml, QObject *parent=nullptr) | |
KConfigLoader (KSharedConfigPtr config, QIODevice *xml, QObject *parent=nullptr) | |
KConfigSkeletonItem * | findItem (const QString &group, const QString &key) const |
KConfigSkeletonItem * | findItemByName (const QString &name) const |
QStringList | groupList () const |
bool | hasGroup (const QString &group) const |
QVariant | property (const QString &name) const |
![]() | |
KConfigSkeleton (const QString &configname=QString(), QObject *parent=nullptr) | |
KConfigSkeleton (KSharedConfig::Ptr config, QObject *parent=nullptr) | |
ItemColor * | addItemColor (const QString &name, QColor &reference, const QColor &defaultValue=QColor(128, 128, 128), const QString &key=QString()) |
ItemFont * | addItemFont (const QString &name, QFont &reference, const QFont &defaultValue=QFont(), const QString &key=QString()) |
![]() | |
KCoreConfigSkeleton (const QString &configname=QString(), QObject *parent=nullptr) | |
KCoreConfigSkeleton (KSharedConfig::Ptr config, QObject *parent=nullptr) | |
~KCoreConfigSkeleton () override | |
void | addItem (KConfigSkeletonItem *item, const QString &name=QString()) |
ItemBool * | addItemBool (const QString &name, bool &reference, bool defaultValue=false, const QString &key=QString()) |
ItemDateTime * | addItemDateTime (const QString &name, QDateTime &reference, const QDateTime &defaultValue=QDateTime(), const QString &key=QString()) |
ItemDouble * | addItemDouble (const QString &name, double &reference, double defaultValue=0.0, const QString &key=QString()) |
ItemInt * | addItemInt (const QString &name, qint32 &reference, qint32 defaultValue=0, const QString &key=QString()) |
ItemIntList * | addItemIntList (const QString &name, QList< int > &reference, const QList< int > &defaultValue=QList< int >(), const QString &key=QString()) |
ItemLongLong * | addItemLongLong (const QString &name, qint64 &reference, qint64 defaultValue=0, const QString &key=QString()) |
ItemPassword * | addItemPassword (const QString &name, QString &reference, const QString &defaultValue=QLatin1String(""), const QString &key=QString()) |
ItemPath * | addItemPath (const QString &name, QString &reference, const QString &defaultValue=QLatin1String(""), const QString &key=QString()) |
ItemPoint * | addItemPoint (const QString &name, QPoint &reference, const QPoint &defaultValue=QPoint(), const QString &key=QString()) |
ItemPointF * | addItemPointF (const QString &name, QPointF &reference, const QPointF &defaultValue=QPointF(), const QString &key=QString()) |
ItemProperty * | addItemProperty (const QString &name, QVariant &reference, const QVariant &defaultValue=QVariant(), const QString &key=QString()) |
ItemRect * | addItemRect (const QString &name, QRect &reference, const QRect &defaultValue=QRect(), const QString &key=QString()) |
ItemRectF * | addItemRectF (const QString &name, QRectF &reference, const QRectF &defaultValue=QRectF(), const QString &key=QString()) |
ItemSize * | addItemSize (const QString &name, QSize &reference, const QSize &defaultValue=QSize(), const QString &key=QString()) |
ItemSizeF * | addItemSizeF (const QString &name, QSizeF &reference, const QSizeF &defaultValue=QSizeF(), const QString &key=QString()) |
ItemString * | addItemString (const QString &name, QString &reference, const QString &defaultValue=QLatin1String(""), const QString &key=QString()) |
ItemStringList * | addItemStringList (const QString &name, QStringList &reference, const QStringList &defaultValue=QStringList(), const QString &key=QString()) |
ItemUInt * | addItemUInt (const QString &name, quint32 &reference, quint32 defaultValue=0, const QString &key=QString()) |
ItemULongLong * | addItemULongLong (const QString &name, quint64 &reference, quint64 defaultValue=0, const QString &key=QString()) |
void | clearItems () |
KConfig * | config () |
const KConfig * | config () const |
QString | currentGroup () const |
KConfigSkeletonItem * | findItem (const QString &name) const |
bool | isDefaults () const |
Q_INVOKABLE bool | isImmutable (const QString &name) const |
bool | isSaveNeeded () const |
KConfigSkeletonItem::List | items () const |
void | load () |
void | read () |
void | removeItem (const QString &name) |
void | setCurrentGroup (const QString &group) |
virtual void | setDefaults () |
void | setSharedConfig (KSharedConfig::Ptr pConfig) |
KSharedConfig::Ptr | sharedConfig () const |
virtual bool | useDefaults (bool b) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | usrSave () override |
![]() | |
virtual void | usrRead () |
virtual void | usrSetDefaults () |
virtual bool | usrUseDefaults (bool b) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
void | configChanged () |
![]() | |
bool | save () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
A KConfigSkeleton that populates itself based on KConfigXT XML.
This class allows one to ship an XML file and reconstitute it into a KConfigSkeleton object at runtime. Common usage might look like this:
Alternatively, any QIODevice may be used in place of QFile in the example above.
KConfigLoader is useful if it is not possible to use compiled code and by that the kconfig compiler cannot be used. Common examples are scripted plugins which want to provide a configuration interface. With the help of KConfigLoader a dynamically loaded ui file can be populated with the stored values and also stored back to the config file.
An example for populating a QDialog with a dynamically populated UI with the help of a KConfigDialogManager:
Currently the following data types are supported:
- bools
- colors
- datetimes
- enumerations
- fonts
- ints
- passwords
- paths
- strings
- stringlists
- uints
- urls
- doubles
- int lists
- longlongs
- path lists
- points
- pointfs
- rects
- rectfs
- sizes
- sizefs
- ulonglongs
- url lists
Definition at line 91 of file kconfigloader.h.
Constructor & Destructor Documentation
◆ KConfigLoader() [1/3]
KConfigLoader::KConfigLoader | ( | const QString & | configFile, |
QIODevice * | xml, | ||
QObject * | parent = nullptr ) |
Creates a KConfigSkeleton populated using the definition found in the XML data passed in.
- Parameters
-
configFile path to the configuration file to use xml the xml data; must be valid KConfigXT data parent optional QObject parent
Definition at line 355 of file kconfigloader.cpp.
◆ KConfigLoader() [2/3]
KConfigLoader::KConfigLoader | ( | KSharedConfigPtr | config, |
QIODevice * | xml, | ||
QObject * | parent = nullptr ) |
Creates a KConfigSkeleton populated using the definition found in the XML data passed in.
- Parameters
-
config the configuration object to use xml the xml data; must be valid KConfigXT data parent optional QObject parent
Definition at line 362 of file kconfigloader.cpp.
◆ KConfigLoader() [3/3]
KConfigLoader::KConfigLoader | ( | const KConfigGroup & | config, |
QIODevice * | xml, | ||
QObject * | parent = nullptr ) |
Creates a KConfigSkeleton populated using the definition found in the XML data passed in.
- Parameters
-
config the group to use as the root for configuration items xml the xml data; must be valid KConfigXT data parent optional QObject parent
Definition at line 372 of file kconfigloader.cpp.
◆ ~KConfigLoader()
|
override |
Definition at line 385 of file kconfigloader.cpp.
Member Function Documentation
◆ findItem()
KConfigSkeletonItem * KConfigLoader::findItem | ( | const QString & | group, |
const QString & | key ) const |
Finds the item for the given group and key.
- Parameters
-
group the group in the config file to look in key the configuration key to find
- Returns
- the associated KConfigSkeletonItem, or
nullptr
if none
Definition at line 390 of file kconfigloader.cpp.
◆ findItemByName()
KConfigSkeletonItem * KConfigLoader::findItemByName | ( | const QString & | name | ) | const |
Finds an item by its name.
Definition at line 395 of file kconfigloader.cpp.
◆ groupList()
QStringList KConfigLoader::groupList | ( | ) | const |
- Returns
- the list of groups defined by the XML
Definition at line 416 of file kconfigloader.cpp.
◆ hasGroup()
bool KConfigLoader::hasGroup | ( | const QString & | group | ) | const |
Check to see if a group exists.
- Parameters
-
group the name of the group to check for
- Returns
- true if the group exists, or false if it does not
Definition at line 411 of file kconfigloader.cpp.
◆ property()
Returns the property (variantized value) of the named item.
Definition at line 400 of file kconfigloader.cpp.
◆ usrSave()
|
overrideprotectedvirtual |
Perform the actual writing of the configuration file.
Override in derived classes to write special config values. Called from save()
Reimplemented from KCoreConfigSkeleton.
Definition at line 421 of file kconfigloader.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:52:48 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.