KConfigGroup
#include <KConfigGroup>
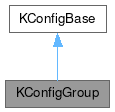
Public Member Functions | |
KConfigGroup () | |
KConfigGroup (const KConfigBase *master, const QString &group) | |
KConfigGroup (const KConfigGroup &) | |
KConfigGroup (const QExplicitlySharedDataPointer< KSharedConfig > &master, const QString &group) | |
KConfigGroup (KConfigBase *master, const QString &group) | |
AccessMode | accessMode () const override |
KConfig * | config () |
const KConfig * | config () const |
void | copyTo (KConfigBase *other, WriteConfigFlags pFlags=Normal) const |
void | deleteEntry (const char *key, WriteConfigFlags pFlags=Normal) |
void | deleteEntry (const QString &pKey, WriteConfigFlags pFlags=Normal) |
void | deleteGroup (const QString &group, WriteConfigFlags flags=Normal) |
void | deleteGroup (WriteConfigFlags pFlags=Normal) |
QMap< QString, QString > | entryMap () const |
bool | exists () const |
QStringList | groupList () const override |
bool | hasDefault (const char *key) const |
bool | hasDefault (const QString &key) const |
bool | hasKey (const char *key) const |
bool | hasKey (const QString &key) const |
bool | isEntryImmutable (const char *key) const |
bool | isEntryImmutable (const QString &key) const |
bool | isImmutable () const override |
bool | isValid () const |
QStringList | keyList () const |
void | markAsClean () override |
void | moveValuesTo (const QList< const char * > &keys, KConfigGroup &other, WriteConfigFlags pFlags=Normal) |
void | moveValuesTo (KConfigGroup &other, WriteConfigFlags pFlags=Normal) |
QString | name () const |
KConfigGroup & | operator= (const KConfigGroup &) |
KConfigGroup | parent () const |
QString | readEntry (const char *key, const char *aDefault=nullptr) const |
template<typename T> | |
QList< T > | readEntry (const char *key, const QList< T > &aDefault) const |
QString | readEntry (const char *key, const QString &aDefault) const |
QStringList | readEntry (const char *key, const QStringList &aDefault) const |
QVariant | readEntry (const char *key, const QVariant &aDefault) const |
QVariantList | readEntry (const char *key, const QVariantList &aDefault) const |
template<typename T> | |
T | readEntry (const char *key, const T &aDefault) const |
QString | readEntry (const QString &key, const char *aDefault=nullptr) const |
template<typename T> | |
QList< T > | readEntry (const QString &key, const QList< T > &aDefault) const |
QString | readEntry (const QString &key, const QString &aDefault) const |
QStringList | readEntry (const QString &key, const QStringList &aDefault) const |
QVariant | readEntry (const QString &key, const QVariant &aDefault) const |
QVariantList | readEntry (const QString &key, const QVariantList &aDefault) const |
template<typename T> | |
T | readEntry (const QString &key, const T &aDefault) const |
QString | readEntryUntranslated (const char *key, const QString &aDefault=QString()) const |
QString | readEntryUntranslated (const QString &pKey, const QString &aDefault=QString()) const |
QString | readPathEntry (const char *key, const QString &aDefault) const |
QStringList | readPathEntry (const char *key, const QStringList &aDefault) const |
QString | readPathEntry (const QString &pKey, const QString &aDefault) const |
QStringList | readPathEntry (const QString &pKey, const QStringList &aDefault) const |
QStringList | readXdgListEntry (const char *key, const QStringList &aDefault=QStringList()) const |
QStringList | readXdgListEntry (const QString &pKey, const QStringList &aDefault=QStringList()) const |
void | reparent (KConfigBase *parent, WriteConfigFlags pFlags=Normal) |
void | revertToDefault (const char *key, WriteConfigFlags pFlag=WriteConfigFlags()) |
void | revertToDefault (const QString &key, WriteConfigFlags pFlag=WriteConfigFlags()) |
bool | sync () override |
void | writeEntry (const char *key, const char *value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const char *key, const QByteArray &value, WriteConfigFlags pFlags=Normal) |
template<typename T> | |
void | writeEntry (const char *key, const QList< T > &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const char *key, const QString &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const char *key, const QStringList &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const char *key, const QVariant &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const char *key, const QVariantList &value, WriteConfigFlags pFlags=Normal) |
template<typename T> | |
void | writeEntry (const char *key, const T &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const QString &key, const char *value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const QString &key, const QByteArray &value, WriteConfigFlags pFlags=Normal) |
template<typename T> | |
void | writeEntry (const QString &key, const QList< T > &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const QString &key, const QString &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const QString &key, const QStringList &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const QString &key, const QVariant &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const QString &key, const QVariantList &value, WriteConfigFlags pFlags=Normal) |
template<typename T> | |
void | writeEntry (const QString &key, const T &value, WriteConfigFlags pFlags=Normal) |
void | writePathEntry (const char *Key, const QString &path, WriteConfigFlags pFlags=Normal) |
void | writePathEntry (const char *key, const QStringList &value, WriteConfigFlags pFlags=Normal) |
void | writePathEntry (const QString &pKey, const QString &path, WriteConfigFlags pFlags=Normal) |
void | writePathEntry (const QString &pKey, const QStringList &value, WriteConfigFlags pFlags=Normal) |
void | writeXdgListEntry (const char *key, const QStringList &value, WriteConfigFlags pFlags=Normal) |
void | writeXdgListEntry (const QString &pKey, const QStringList &value, WriteConfigFlags pFlags=Normal) |
![]() | |
virtual | ~KConfigBase () |
void | deleteGroup (const QString &group, WriteConfigFlags flags=Normal) |
KConfigGroup | group (const QString &group) |
const KConfigGroup | group (const QString &group) const |
bool | hasGroup (const QString &group) const |
bool | isGroupImmutable (const QString &group) const |
Protected Member Functions | |
void | deleteGroupImpl (const QString &groupName, WriteConfigFlags flags) override |
const KConfigGroup | groupImpl (const QString &groupName) const override |
KConfigGroup | groupImpl (const QString &groupName) override |
bool | hasGroupImpl (const QString &groupName) const override |
bool | isGroupImmutableImpl (const QString &groupName) const override |
![]() | |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
enum | AccessMode { NoAccess , ReadOnly , ReadWrite } |
enum | WriteConfigFlag { Persistent = 0x01 , Global = 0x02 , Localized = 0x04 , Notify = 0x08 | Persistent , Normal = Persistent } |
typedef QFlags< WriteConfigFlag > | WriteConfigFlags |
Detailed Description
A class for one specific group in a KConfig object.
If you want to access the top-level entries of a KConfig object, which are not associated with any group, use an empty group name.
A KConfigGroup will be read-only if it is constructed from a const config object or from another read-only group.
Definition at line 38 of file kconfiggroup.h.
Constructor & Destructor Documentation
◆ KConfigGroup() [1/5]
KConfigGroup::KConfigGroup | ( | ) |
◆ KConfigGroup() [2/5]
KConfigGroup::KConfigGroup | ( | KConfigBase * | master, |
const QString & | group ) |
Construct a config group corresponding to group
in master
.
This allows the creation of subgroups by passing another group as master
.
- Parameters
-
group name of group
Definition at line 477 of file kconfiggroup.cpp.
◆ KConfigGroup() [3/5]
KConfigGroup::KConfigGroup | ( | const KConfigBase * | master, |
const QString & | group ) |
Construct a read-only config group.
A read-only group will silently ignore any attempts to write to it.
This allows the creation of subgroups by passing an existing group as master
.
Definition at line 482 of file kconfiggroup.cpp.
◆ KConfigGroup() [4/5]
KConfigGroup::KConfigGroup | ( | const QExplicitlySharedDataPointer< KSharedConfig > & | master, |
const QString & | group ) |
Overload for KConfigGroup(const KConfigBase*,const QString&)
Definition at line 487 of file kconfiggroup.cpp.
◆ KConfigGroup() [5/5]
KConfigGroup::KConfigGroup | ( | const KConfigGroup & | rhs | ) |
Creates a copy of a group.
Definition at line 498 of file kconfiggroup.cpp.
◆ ~KConfigGroup()
|
override |
Definition at line 503 of file kconfiggroup.cpp.
Member Function Documentation
◆ accessMode()
|
overridevirtual |
- Reimplemented from superclass.
Implements KConfigBase.
Definition at line 1172 of file kconfiggroup.cpp.
◆ config() [1/2]
KConfig * KConfigGroup::config | ( | ) |
Return the config object that this group belongs to.
Definition at line 589 of file kconfiggroup.cpp.
◆ config() [2/2]
const KConfig * KConfigGroup::config | ( | ) | const |
Return the config object that this group belongs to.
Definition at line 596 of file kconfiggroup.cpp.
◆ copyTo()
void KConfigGroup::copyTo | ( | KConfigBase * | other, |
WriteConfigFlags | pFlags = Normal ) const |
Copies the entries in this group to another configuration object.
- Note
other
can be either another group or a different file.
- Parameters
-
other the configuration object to copy this group's entries to pFlags the flags to use when writing the entries to the other configuration object
- Since
- 4.1
Definition at line 1205 of file kconfiggroup.cpp.
◆ deleteEntry() [1/2]
void KConfigGroup::deleteEntry | ( | const char * | key, |
WriteConfigFlags | pFlags = Normal ) |
Overload for deleteEntry(const QString&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 1087 of file kconfiggroup.cpp.
◆ deleteEntry() [2/2]
void KConfigGroup::deleteEntry | ( | const QString & | pKey, |
WriteConfigFlags | pFlags = Normal ) |
Deletes the entry specified by pKey
in the current group.
This also hides system wide defaults.
- Parameters
-
pKey the key to delete pFlags the flags to use when deleting this entry
- See also
- deleteGroup(), readEntry(), writeEntry()
Definition at line 1095 of file kconfiggroup.cpp.
◆ deleteGroup() [1/2]
void KConfigBase::deleteGroup | ( | const QString & | group, |
WriteConfigFlags | flags = Normal ) |
Delete group
.
This marks group
as deleted in the config object. This effectively removes any cascaded values from config files earlier in the stack.
Definition at line 108 of file kconfigbase.cpp.
◆ deleteGroup() [2/2]
void KConfigGroup::deleteGroup | ( | WriteConfigFlags | pFlags = Normal | ) |
Delete all entries in the entire group.
- Parameters
-
pFlags flags passed to KConfig::deleteGroup
- See also
- deleteEntry()
Definition at line 549 of file kconfiggroup.cpp.
◆ deleteGroupImpl()
|
overrideprotectedvirtual |
- Parameters
-
groupName name of group
Implements KConfigBase.
Definition at line 1186 of file kconfiggroup.cpp.
◆ entryMap()
Returns a map (tree) of entries for all entries in this group.
Only the actual entry string is returned, none of the other internal data should be included.
- Returns
- a map of entries in this group, indexed by key
Definition at line 582 of file kconfiggroup.cpp.
◆ exists()
bool KConfigGroup::exists | ( | ) | const |
Check whether the containing KConfig object actually contains a group with this name.
Definition at line 564 of file kconfiggroup.cpp.
◆ groupImpl() [1/2]
|
overrideprotectedvirtual |
- Parameters
-
groupName name of group
Implements KConfigBase.
Definition at line 520 of file kconfiggroup.cpp.
◆ groupImpl() [2/2]
|
overrideprotectedvirtual |
- Parameters
-
groupName name of group
Implements KConfigBase.
Definition at line 508 of file kconfiggroup.cpp.
◆ groupList()
|
overridevirtual |
- Reimplemented from superclass.
Implements KConfigBase.
Definition at line 1151 of file kconfiggroup.cpp.
◆ hasDefault() [1/2]
bool KConfigGroup::hasDefault | ( | const char * | key | ) | const |
Overload for hasDefault(const QString&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 1113 of file kconfiggroup.cpp.
◆ hasDefault() [2/2]
bool KConfigGroup::hasDefault | ( | const QString & | key | ) | const |
Whether a default is specified for an entry in either the system wide configuration file or the global KDE config file.
If an application computes a default value at runtime for a certain entry, e.g. like:
then it may wish to make the following check before writing back changes:
This ensures that as long as the entry is not modified to differ from the computed default, the application will keep using the computed default and will follow changes the computed default makes over time.
- Parameters
-
key the key of the entry to check
- Returns
true
if the global or system settings files specify a default forkey
in this group,false
otherwise
Definition at line 1122 of file kconfiggroup.cpp.
◆ hasGroupImpl()
|
overrideprotectedvirtual |
- Parameters
-
groupName name of group
Implements KConfigBase.
Definition at line 1179 of file kconfiggroup.cpp.
◆ hasKey() [1/2]
bool KConfigGroup::hasKey | ( | const char * | key | ) | const |
Overload for hasKey(const QString&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 1127 of file kconfiggroup.cpp.
◆ hasKey() [2/2]
bool KConfigGroup::hasKey | ( | const QString & | key | ) | const |
Checks whether the key has an entry in this group.
Use this to determine if a key is not specified for the current group (hasKey() returns false).
If this returns false
for a key, readEntry() (and its variants) will return the default value passed to them.
- Parameters
-
key the key to search for
- Returns
true
if the key is defined in this group by any of the configuration sources,false
otherwise
- See also
- readEntry()
Definition at line 1139 of file kconfiggroup.cpp.
◆ isEntryImmutable() [1/2]
bool KConfigGroup::isEntryImmutable | ( | const char * | key | ) | const |
Overload for isEntryImmutable(const QString&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 603 of file kconfiggroup.cpp.
◆ isEntryImmutable() [2/2]
bool KConfigGroup::isEntryImmutable | ( | const QString & | key | ) | const |
Checks if it is possible to change the given entry.
If isImmutable() returns true
, then this method will return true
for all inputs.
- Parameters
-
key the key to check
- Returns
false
if the key may be changed using this configuration group object,true
otherwise
Definition at line 610 of file kconfiggroup.cpp.
◆ isGroupImmutableImpl()
|
overrideprotectedvirtual |
- Parameters
-
groupName name of group
Implements KConfigBase.
Definition at line 1194 of file kconfiggroup.cpp.
◆ isImmutable()
|
overridevirtual |
Whether this group may be changed.
- Returns
false
if the group may be changed,true
otherwise
Implements KConfigBase.
Definition at line 1144 of file kconfiggroup.cpp.
◆ isValid()
bool KConfigGroup::isValid | ( | ) | const |
Whether the group is valid.
A group is invalid if it was constructed without arguments.
You should not call any functions on an invalid group.
- Returns
true
if the group is valid,false
if it is invalid.
Definition at line 455 of file kconfiggroup.cpp.
◆ keyList()
QStringList KConfigGroup::keyList | ( | ) | const |
Returns a list of keys this group contains.
Definition at line 1158 of file kconfiggroup.cpp.
◆ markAsClean()
|
overridevirtual |
- Reimplemented from superclass.
Implements KConfigBase.
Definition at line 1165 of file kconfiggroup.cpp.
◆ moveValuesTo() [1/2]
void KConfigGroup::moveValuesTo | ( | const QList< const char * > & | keys, |
KConfigGroup & | other, | ||
WriteConfigFlags | pFlags = Normal ) |
Moves the key-value pairs from one config group to the other.
In case the entries do not exist the key is ignored.
- Since
- 5.88
Definition at line 1255 of file kconfiggroup.cpp.
◆ moveValuesTo() [2/2]
void KConfigGroup::moveValuesTo | ( | KConfigGroup & | other, |
WriteConfigFlags | pFlags = Normal ) |
Moves the key-value pairs from one config group to the other.
- Since
- 6.3
Definition at line 1265 of file kconfiggroup.cpp.
◆ name()
QString KConfigGroup::name | ( | ) | const |
The name of this group.
The root group is named "<default>".
Definition at line 557 of file kconfiggroup.cpp.
◆ operator=()
KConfigGroup & KConfigGroup::operator= | ( | const KConfigGroup & | rhs | ) |
Definition at line 492 of file kconfiggroup.cpp.
◆ parent()
KConfigGroup KConfigGroup::parent | ( | ) | const |
Returns the group that this group belongs to.
- Returns
- the parent group, or an invalid group if this is a top-level group
- Since
- 4.1
Definition at line 532 of file kconfiggroup.cpp.
◆ readEntry() [1/14]
QString KConfigGroup::readEntry | ( | const char * | key, |
const char * | aDefault = nullptr ) const |
Overload for readEntry(const QString&, const QString&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 631 of file kconfiggroup.cpp.
◆ readEntry() [2/14]
QList< T > KConfigGroup::readEntry | ( | const char * | key, |
const QList< T > & | aDefault ) const |
Overload for readEntry<T>(const QString&, const QList<T>&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 769 of file kconfiggroup.h.
◆ readEntry() [3/14]
Overload for readEntry(const QString&, const QString&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 641 of file kconfiggroup.cpp.
◆ readEntry() [4/14]
QStringList KConfigGroup::readEntry | ( | const char * | key, |
const QStringList & | aDefault ) const |
Overload for readEntry(const QString&, const QStringList&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 665 of file kconfiggroup.cpp.
◆ readEntry() [5/14]
Overload for readEntry(const QString&, const QVariant&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 682 of file kconfiggroup.cpp.
◆ readEntry() [6/14]
QVariantList KConfigGroup::readEntry | ( | const char * | key, |
const QVariantList & | aDefault ) const |
Overload for readEntry(const QString&, const QVariantList&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 704 of file kconfiggroup.cpp.
◆ readEntry() [7/14]
T KConfigGroup::readEntry | ( | const char * | key, |
const T & | aDefault ) const |
Overload for readEntry<T>(const QString&, const T&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 762 of file kconfiggroup.h.
◆ readEntry() [8/14]
Overload for readEntry(const QString&, const QString&) const.
Definition at line 636 of file kconfiggroup.cpp.
◆ readEntry() [9/14]
|
inline |
Reads a list of values from the config object.
- Parameters
-
key the key to search for aDefault the default value to use if the key does not exist
- Returns
- the list, or
aDefault
ifkey
does not exist
- See also
- readXdgListEntry(), writeEntry(), deleteEntry(), hasKey()
Definition at line 317 of file kconfiggroup.h.
◆ readEntry() [10/14]
Reads the string value of an entry specified by key
in the current group.
If you want to read a path, please use readPathEntry().
- Parameters
-
key the key to search for aDefault a default value returned if the key was not found
- Returns
- the value for this key, or
aDefault
if the key was not found
- See also
- readPathEntry(), writeEntry(), deleteEntry(), hasKey()
Definition at line 660 of file kconfiggroup.cpp.
◆ readEntry() [11/14]
QStringList KConfigGroup::readEntry | ( | const QString & | key, |
const QStringList & | aDefault ) const |
Reads a list of strings from the config object.
- Parameters
-
key The key to search for aDefault The default value to use if the key does not exist
- Returns
- The list, or
aDefault
ifkey
does not exist
- See also
- readXdgListEntry(), writeEntry(), deleteEntry(), hasKey()
Definition at line 677 of file kconfiggroup.cpp.
◆ readEntry() [12/14]
Reads the value of an entry specified by key
in the current group.
- Parameters
-
key the key to search for aDefault a default value returned if the key was not found
- Returns
- the value for this key, or
aDefault
if the key was not found
- See also
- writeEntry(), deleteEntry(), hasKey()
Definition at line 699 of file kconfiggroup.cpp.
◆ readEntry() [13/14]
QVariantList KConfigGroup::readEntry | ( | const QString & | key, |
const QVariantList & | aDefault ) const |
Overload for readEntry(const QString&, const QStringList&) const.
- Parameters
-
key name of key, encoded in UTF-8
- Warning
- This function doesn't convert the items returned to any type. It's actually a list of QVariant::String's. If you want the items converted to a specific type use readEntry(const char*, const QList<T>&) const
Definition at line 724 of file kconfiggroup.cpp.
◆ readEntry() [14/14]
|
inline |
Reads the value of an entry specified by pKey
in the current group.
This template method makes it possible to write QString foo = readEntry("...", QString("default")); and the same with all other types supported by QVariant.
The return type of the method is simply the same as the type of the default value.
- Note
- readEntry("...", Qt::white) will not compile because Qt::white is an enum. You must turn it into readEntry("...", QColor(Qt::white)).
- Only the following QVariant types are allowed : String, StringList, List, Font, Point, PointF, Rect, RectF, Size, SizeF, Color, Int, UInt, Bool, Double, LongLong, ULongLong, DateTime and Date.
- Parameters
-
key The key to search for aDefault A default value returned if the key was not found
- Returns
- The value for this key, or
aDefault
.
- See also
- writeEntry(), deleteEntry(), hasKey()
Definition at line 223 of file kconfiggroup.h.
◆ readEntryUntranslated() [1/2]
QString KConfigGroup::readEntryUntranslated | ( | const char * | key, |
const QString & | aDefault = QString() ) const |
Overload for readEntryUntranslated(const QString&, const QString&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 620 of file kconfiggroup.cpp.
◆ readEntryUntranslated() [2/2]
QString KConfigGroup::readEntryUntranslated | ( | const QString & | pKey, |
const QString & | aDefault = QString() ) const |
Reads an untranslated string entry.
You should not normally need to use this.
- Parameters
-
pKey the key to search for aDefault a default value returned if the key was not found
- Returns
- the value for this key, or
aDefault
if the key does not exist
Definition at line 615 of file kconfiggroup.cpp.
◆ readPathEntry() [1/4]
Overload for readPathEntry(const QString&, const QString&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 774 of file kconfiggroup.cpp.
◆ readPathEntry() [2/4]
QStringList KConfigGroup::readPathEntry | ( | const char * | key, |
const QStringList & | aDefault ) const |
Overload for readPathEntry(const QString&, const QStringList&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 793 of file kconfiggroup.cpp.
◆ readPathEntry() [3/4]
Reads a path.
Read the value of an entry specified by pKey
in the current group and interpret it as a path. This means, dollar expansion is activated for this value, so that e.g. $HOME gets expanded.
- Parameters
-
pKey The key to search for. aDefault A default value returned if the key was not found.
- Returns
- The value for this key. Can be QString() if
aDefault
is null.
Definition at line 769 of file kconfiggroup.cpp.
◆ readPathEntry() [4/4]
QStringList KConfigGroup::readPathEntry | ( | const QString & | pKey, |
const QStringList & | aDefault ) const |
Reads a list of paths.
Read the value of an entry specified by pKey
in the current group and interpret it as a list of paths. This means, dollar expansion is activated for this value, so that e.g. $HOME gets expanded.
- Parameters
-
pKey the key to search for aDefault a default value returned if the key was not found
- Returns
- the list, or
aDefault
if the key does not exist
Definition at line 788 of file kconfiggroup.cpp.
◆ readXdgListEntry() [1/2]
QStringList KConfigGroup::readXdgListEntry | ( | const char * | key, |
const QStringList & | aDefault = QStringList() ) const |
Overload for readXdgListEntry(const QString&, const QStringList&) const.
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 734 of file kconfiggroup.cpp.
◆ readXdgListEntry() [2/2]
QStringList KConfigGroup::readXdgListEntry | ( | const QString & | pKey, |
const QStringList & | aDefault = QStringList() ) const |
Reads a list of strings from the config object with semicolons separating them (i.e.
following desktop entry spec separator semantics).
- Parameters
-
pKey the key to search for aDefault the default value to use if the key does not exist
- Returns
- the list, or
aDefault
ifpKey
does not exist
Definition at line 729 of file kconfiggroup.cpp.
◆ reparent()
void KConfigGroup::reparent | ( | KConfigBase * | parent, |
WriteConfigFlags | pFlags = Normal ) |
Changes the configuration object that this group belongs to.
- Note
other
can be another group, the top-level KConfig object or a different KConfig object entirely.
If parent
is already the parent of this group, this method will have no effect.
- Parameters
-
parent the config object to place this group under pFlags the flags to use in determining which storage source to write the data to
- Since
- 4.1
Definition at line 1220 of file kconfiggroup.cpp.
◆ revertToDefault() [1/2]
void KConfigGroup::revertToDefault | ( | const char * | key, |
WriteConfigFlags | pFlag = WriteConfigFlags() ) |
Overload for revertToDefault(const QString&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 1100 of file kconfiggroup.cpp.
◆ revertToDefault() [2/2]
void KConfigGroup::revertToDefault | ( | const QString & | key, |
WriteConfigFlags | pFlag = WriteConfigFlags() ) |
Reverts an entry to the default settings.
Reverts the entry with key key
in the current group in the application specific config file to either the system wide (default) value or the value specified in the global KDE config file.
To revert entries in the global KDE config file, the global KDE config file should be opened explicitly in a separate config object.
- Note
- This is not the same as deleting the key, as instead the global setting will be copied to the configuration file that this object manipulates.
- Parameters
-
key The key of the entry to revert.
Definition at line 1108 of file kconfiggroup.cpp.
◆ sync()
|
overridevirtual |
- Reimplemented from superclass.
Syncs the parent config.
Implements KConfigBase.
Definition at line 571 of file kconfiggroup.cpp.
◆ writeEntry() [1/16]
void KConfigGroup::writeEntry | ( | const char * | key, |
const char * | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 826 of file kconfiggroup.cpp.
◆ writeEntry() [2/16]
void KConfigGroup::writeEntry | ( | const char * | key, |
const QByteArray & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 831 of file kconfiggroup.cpp.
◆ writeEntry() [3/16]
void KConfigGroup::writeEntry | ( | const char * | key, |
const QList< T > & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 798 of file kconfiggroup.h.
◆ writeEntry() [4/16]
void KConfigGroup::writeEntry | ( | const char * | key, |
const QString & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 805 of file kconfiggroup.cpp.
◆ writeEntry() [5/16]
void KConfigGroup::writeEntry | ( | const char * | key, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 844 of file kconfiggroup.cpp.
◆ writeEntry() [6/16]
void KConfigGroup::writeEntry | ( | const char * | key, |
const QVariant & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 883 of file kconfiggroup.cpp.
◆ writeEntry() [7/16]
void KConfigGroup::writeEntry | ( | const char * | key, |
const QVariantList & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 864 of file kconfiggroup.cpp.
◆ writeEntry() [8/16]
void KConfigGroup::writeEntry | ( | const char * | key, |
const T & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 791 of file kconfiggroup.h.
◆ writeEntry() [9/16]
void KConfigGroup::writeEntry | ( | const QString & | key, |
const char * | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 818 of file kconfiggroup.cpp.
◆ writeEntry() [10/16]
void KConfigGroup::writeEntry | ( | const QString & | key, |
const QByteArray & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 839 of file kconfiggroup.cpp.
◆ writeEntry() [11/16]
|
inline |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 468 of file kconfiggroup.h.
◆ writeEntry() [12/16]
void KConfigGroup::writeEntry | ( | const QString & | key, |
const QString & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 813 of file kconfiggroup.cpp.
◆ writeEntry() [13/16]
void KConfigGroup::writeEntry | ( | const QString & | key, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 859 of file kconfiggroup.cpp.
◆ writeEntry() [14/16]
void KConfigGroup::writeEntry | ( | const QString & | key, |
const QVariant & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Writes a value to the configuration object.
- Parameters
-
key the key to write to value the value to write pFlags the flags to use when writing this entry
- See also
- readEntry(), writeXdgListEntry(), deleteEntry()
Definition at line 1022 of file kconfiggroup.cpp.
◆ writeEntry() [15/16]
void KConfigGroup::writeEntry | ( | const QString & | key, |
const QVariantList & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 1027 of file kconfiggroup.cpp.
◆ writeEntry() [16/16]
|
inline |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 445 of file kconfiggroup.h.
◆ writePathEntry() [1/4]
void KConfigGroup::writePathEntry | ( | const char * | Key, |
const QString & | path, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writePathEntry(const QString&, const QString&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 1060 of file kconfiggroup.cpp.
◆ writePathEntry() [2/4]
void KConfigGroup::writePathEntry | ( | const char * | key, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writePathEntry(const QString&, const QStringList&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 1073 of file kconfiggroup.cpp.
◆ writePathEntry() [3/4]
void KConfigGroup::writePathEntry | ( | const QString & | pKey, |
const QString & | path, | ||
WriteConfigFlags | pFlags = Normal ) |
Writes a file path to the configuration.
If the path is located under $HOME, the user's home directory is replaced with $HOME in the persistent storage. The path should therefore be read back with readPathEntry()
- Parameters
-
pKey the key to write to path the path to write pFlags the flags to use when writing this entry
- See also
- writeEntry(), readPathEntry()
Definition at line 1055 of file kconfiggroup.cpp.
◆ writePathEntry() [4/4]
void KConfigGroup::writePathEntry | ( | const QString & | pKey, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Writes a list of paths to the configuration.
If any of the paths are located under $HOME, the user's home directory is replaced with $HOME in the persistent storage. The paths should therefore be read back with readPathEntry()
- Parameters
-
pKey the key to write to value the list to write pFlags the flags to use when writing this entry
- See also
- writeEntry(), readPathEntry()
Definition at line 1068 of file kconfiggroup.cpp.
◆ writeXdgListEntry() [1/2]
void KConfigGroup::writeXdgListEntry | ( | const char * | key, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Overload for writeXdgListEntry(const QString&, const QStringList&, WriteConfigFlags)
- Parameters
-
key name of key, encoded in UTF-8
Definition at line 1037 of file kconfiggroup.cpp.
◆ writeXdgListEntry() [2/2]
void KConfigGroup::writeXdgListEntry | ( | const QString & | pKey, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal ) |
Writes a list of strings to the config object, following XDG desktop entry spec separator semantics.
- Parameters
-
pKey the key to write to value the list to write pFlags the flags to use when writing this entry
- See also
- writeEntry(), readXdgListEntry()
Definition at line 1032 of file kconfiggroup.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:04:35 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.