KPluginMetaData
#include <KPluginMetaData>
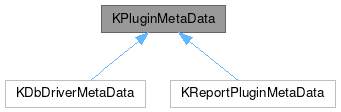
Public Types | |
enum | KPluginMetaDataOption { AllowEmptyMetaData = 1 , CacheMetaData = 2 } |
typedef QFlags< KPluginMetaDataOption > | KPluginMetaDataOptions |
Public Member Functions | |
KPluginMetaData () | |
KPluginMetaData (const KPluginMetaData &) | |
KPluginMetaData (const QJsonObject &metaData, const QString &fileName) | |
KPluginMetaData (const QPluginLoader &loader, KPluginMetaDataOptions options={}) | |
KPluginMetaData (const QString &pluginFile, KPluginMetaDataOptions options={}) | |
~KPluginMetaData () | |
QList< KAboutPerson > | authors () const |
QString | bugReportUrl () const |
QString | category () const |
QString | copyrightText () const |
QString | description () const |
QString | fileName () const |
QStringList | formFactors () const |
QString | iconName () const |
template<typename T > | |
bool | isEnabled (const T &config) const |
bool | isEnabledByDefault () const |
bool | isHidden () const |
bool | isStaticPlugin () const |
bool | isValid () const |
QString | license () const |
QString | licenseText () const |
QStringList | mimeTypes () const |
QString | name () const |
bool | operator!= (const KPluginMetaData &other) const |
KPluginMetaData & | operator= (const KPluginMetaData &) |
bool | operator== (const KPluginMetaData &other) const |
QList< KAboutPerson > | otherContributors () const |
QString | pluginId () const |
QJsonObject | rawData () const |
bool | supportsMimeType (const QString &mimeType) const |
QList< KAboutPerson > | translators () const |
bool | value (const QString &key, bool defaultValue) const |
QString | value (const QString &key, const char *ch) const =delete |
QString | value (const QString &key, const QString &defaultValue=QString()) const |
QStringList | value (const QString &key, const QStringList &defaultValue) const |
int | value (const QString &key, int defaultValue) const |
QString | version () const |
QString | website () const |
Static Public Member Functions | |
static KPluginMetaData | findPluginById (const QString &directory, const QString &pluginId, KPluginMetaDataOptions options={}) |
static QList< KPluginMetaData > | findPlugins (const QString &directory, std::function< bool(const KPluginMetaData &)> filter={}, KPluginMetaDataOptions options={}) |
static KPluginMetaData | fromJsonFile (const QString &jsonFile) |
Detailed Description
This class allows easily accessing some standardized values from the JSON metadata that can be embedded into Qt plugins.
Additional plugin-specific metadata can be retrieved by directly reading from the QJsonObject returned by KPluginMetaData::rawData().
For embedded metadata, you should not specify an id manually. Instead the id will be derived from the file basename.
The following keys will be read from an object "KPlugin" inside the metadata JSON:
Key | Accessor function | JSON Type |
---|---|---|
Name | name() | string |
Description | description() | string |
Icon | iconName() | string |
Authors | authors() | object array (KAboutPerson) |
Category | category() | string |
License | license() | string |
Copyright | copyrightText() | string |
Id | pluginId() | string |
Version | version() | string |
Website | website() | string |
BugReportUrl | bugReportUrl() | string |
EnabledByDefault | isEnabledByDefault() | bool |
MimeTypes | mimeTypes() | string array |
FormFactors | formFactors() | string array |
Translators | translators() | object array (KAboutPerson) |
OtherContributors | otherContributors() | object array (KAboutPerson) |
The Authors, Translators and OtherContributors keys are expected to be list of objects that match the structure expected by KAboutPerson::fromJSON().
An example metadata json file could look like this:
{ "KPlugin": { "Name": "Date and Time", "Description": "Date and time by timezone", "Icon": "preferences-system-time", "Authors": [ { "Name": "Aaron Seigo", "Email": "aseigo@kde.org" } ], "Category": "Date and Time", "EnabledByDefault": "true", "License": "LGPL", "Version": "1.0", "Website": "https://plasma.kde.org/" } }
- See also
- KAboutPerson::fromJSON()
- Since
- 5.1
Definition at line 81 of file kpluginmetadata.h.
Member Typedef Documentation
◆ KPluginMetaDataOptions
Definition at line 119 of file kpluginmetadata.h.
Member Enumeration Documentation
◆ KPluginMetaDataOption
Options for creating a KPluginMetaData object.
- Since
- 5.91
Enumerator | |
---|---|
AllowEmptyMetaData | Plugins with empty metaData are considered valid. |
CacheMetaData | If KCoreAddons should keep metadata in cache. This makes querying the namespace again faster. Consider using this if you need revalidation of plugins
|
Definition at line 111 of file kpluginmetadata.h.
Property Documentation
◆ authors
|
read |
Definition at line 90 of file kpluginmetadata.h.
◆ bugReportUrl
|
read |
Definition at line 101 of file kpluginmetadata.h.
◆ category
|
read |
Definition at line 93 of file kpluginmetadata.h.
◆ copyrightText
|
read |
Definition at line 97 of file kpluginmetadata.h.
◆ description
|
read |
Definition at line 89 of file kpluginmetadata.h.
◆ fileName
|
read |
Definition at line 86 of file kpluginmetadata.h.
◆ formFactors
|
read |
Definition at line 103 of file kpluginmetadata.h.
◆ iconName
|
read |
Definition at line 94 of file kpluginmetadata.h.
◆ isEnabledByDefault
|
read |
Definition at line 104 of file kpluginmetadata.h.
◆ isHidden
|
read |
Definition at line 85 of file kpluginmetadata.h.
◆ isValid
|
read |
Definition at line 84 of file kpluginmetadata.h.
◆ license
|
read |
Definition at line 95 of file kpluginmetadata.h.
◆ licenseText
|
read |
Definition at line 96 of file kpluginmetadata.h.
◆ mimeTypes
|
read |
Definition at line 102 of file kpluginmetadata.h.
◆ name
|
read |
Definition at line 88 of file kpluginmetadata.h.
◆ otherContributors
|
read |
Definition at line 92 of file kpluginmetadata.h.
◆ pluginId
|
read |
Definition at line 98 of file kpluginmetadata.h.
◆ rawData
|
read |
Definition at line 87 of file kpluginmetadata.h.
◆ translators
|
read |
Definition at line 91 of file kpluginmetadata.h.
◆ version
|
read |
Definition at line 99 of file kpluginmetadata.h.
◆ website
|
read |
Definition at line 100 of file kpluginmetadata.h.
Constructor & Destructor Documentation
◆ KPluginMetaData() [1/5]
KPluginMetaData::KPluginMetaData | ( | ) |
Creates an invalid KPluginMetaData instance.
Definition at line 142 of file kpluginmetadata.cpp.
◆ KPluginMetaData() [2/5]
KPluginMetaData::KPluginMetaData | ( | const QPluginLoader & | loader, |
KPluginMetaDataOptions | options = {} ) |
Reads the plugin metadata from a QPluginLoader instance.
You must call QPluginLoader::setFileName() or use the appropriate constructor on loader
before calling this.
- Parameters
-
option Added in 6.0, see enum docs
Definition at line 183 of file kpluginmetadata.cpp.
◆ KPluginMetaData() [3/5]
KPluginMetaData::KPluginMetaData | ( | const QString & | pluginFile, |
KPluginMetaDataOptions | options = {} ) |
Reads the plugin metadata from a plugin which can be loaded from file
.
Platform-specific library suffixes may be omitted since file
will be resolved using the same logic as QPluginLoader.
- See also
- QPluginLoader::setFileName()
Definition at line 160 of file kpluginmetadata.cpp.
◆ KPluginMetaData() [4/5]
KPluginMetaData::KPluginMetaData | ( | const QJsonObject & | metaData, |
const QString & | fileName ) |
Creates a KPluginMetaData from a QJsonObject holding the metadata and a file name This can be used if the data is not retrieved from a Qt C++ plugin library but from some other source.
- Parameters
-
metaData the JSON metadata to use for this object pluginFile the file that the plugin can be loaded from
- Since
- 6.0
Definition at line 191 of file kpluginmetadata.cpp.
◆ KPluginMetaData() [5/5]
KPluginMetaData::KPluginMetaData | ( | const KPluginMetaData & | other | ) |
Copy contructor.
Definition at line 147 of file kpluginmetadata.cpp.
◆ ~KPluginMetaData()
|
default |
Destructor.
Member Function Documentation
◆ authors()
QList< KAboutPerson > KPluginMetaData::authors | ( | ) | const |
- Returns
- the author(s) of this plugin.
Definition at line 345 of file kpluginmetadata.cpp.
◆ bugReportUrl()
QString KPluginMetaData::bugReportUrl | ( | ) | const |
- Returns
- the website where people can report a bug found in this plugin
- Since
- 5.99
Definition at line 410 of file kpluginmetadata.cpp.
◆ category()
QString KPluginMetaData::category | ( | ) | const |
- Returns
- the categories of this plugin (e.g. "playlist/skin").
Definition at line 360 of file kpluginmetadata.cpp.
◆ copyrightText()
QString KPluginMetaData::copyrightText | ( | ) | const |
◆ description()
QString KPluginMetaData::description | ( | ) | const |
- Returns
- a short description of the plugin.
Definition at line 365 of file kpluginmetadata.cpp.
◆ fileName()
QString KPluginMetaData::fileName | ( | ) | const |
- Returns
- the path to the plugin. When the KPluginMetaData(QJsonObject, QString) constructor is used, the string is not modified. Otherwise, the path is resolved using QPluginLoader. For static plugins the fileName is the namespace and pluginId concatenated
- Note
- It is not guaranteed that this is a valid path to a shared library (i.e. loadable by QPluginLoader) since the metadata could also refer to a non-C++ plugin.
Definition at line 245 of file kpluginmetadata.cpp.
◆ findPluginById()
|
static |
- Parameters
-
directory The directory to search for plugins. If a relative path is given for directory
, all entries of QCoreApplication::libraryPaths() will be checked withdirectory
appended as a subdirectory. If an absolute path is given only that directory will be searched.
- Note
- Check if the returned KPluginMetaData is valid before continuing to use it.
- Parameters
-
pluginId The Id of the plugin. The id should be the same as the filename, see KPluginMetaData::pluginId() option Added in 6.0, see enum docs
- Since
- 5.84
Definition at line 203 of file kpluginmetadata.cpp.
◆ findPlugins()
|
static |
Find all plugins inside directory
.
Only plugins which have JSON metadata will be considered.
- Parameters
-
directory The directory to search for plugins. If a relative path is given for directory
, all entries of QCoreApplication::libraryPaths() will be checked withdirectory
appended as a subdirectory. If an absolute path is given only that directory will be searched.filter a callback function that returns true
if the found plugin should be loaded andfalse
if it should be skipped. If this argument is omitted all plugins will be loadedoption Weather or not allow plugins with empty metadata to be considered valid
- Returns
- all plugins found in
directory
that fulfil the constraints offilter
- Since
- 5.86
Definition at line 250 of file kpluginmetadata.cpp.
◆ formFactors()
QStringList KPluginMetaData::formFactors | ( | ) | const |
- Returns
- A string list of formfactors this plugin is useful for, e.g. desktop, handset or mediacenter. The keys for this are not formally defined, though the above-mentioned values should be used when applicable.
- Since
- 5.12
Definition at line 442 of file kpluginmetadata.cpp.
◆ fromJsonFile()
|
static |
Load a KPluginMetaData instance from a .json file.
Unlike the constructor with a single file argument, this ensure that only JSON format plugins are loaded and any other type is rejected.
- Parameters
-
jsonFile the .json file to load
- Since
- 5.91
Definition at line 223 of file kpluginmetadata.cpp.
◆ iconName()
QString KPluginMetaData::iconName | ( | ) | const |
- Returns
- the icon name for this plugin
- See also
- QIcon::fromTheme()
Definition at line 370 of file kpluginmetadata.cpp.
◆ isEnabled()
|
inline |
Returns true
if the plugin is enabled in config
, otherwise returns isEnabledByDefault().
This can be used in conjunction with KPluginWidget.
The config
param should be a KConfigGroup object, because KCoreAddons can not depend on KConfig directly, this parameter is a template.
- Parameters
-
config KConfigGroup where the enabled state is stored
- Since
- 5.89
Definition at line 359 of file kpluginmetadata.h.
◆ isEnabledByDefault()
bool KPluginMetaData::isEnabledByDefault | ( | ) | const |
- Returns
- whether the plugin should be enabled by default. This is only a recommendation, applications can ignore this value if they want to.
Definition at line 447 of file kpluginmetadata.cpp.
◆ isHidden()
bool KPluginMetaData::isHidden | ( | ) | const |
- Returns
- whether this object should be hidden
- Since
- 5.8
Definition at line 313 of file kpluginmetadata.cpp.
◆ isStaticPlugin()
bool KPluginMetaData::isStaticPlugin | ( | ) | const |
- Note
- for loading plugin the plugin independently of it being static/dynamic use KPluginFactory::loadFactory or KPluginFactory::instantiatePlugin.
- Returns
- true if the instance represents a static plugin
- Since
- 5.89
Definition at line 532 of file kpluginmetadata.cpp.
◆ isValid()
bool KPluginMetaData::isValid | ( | ) | const |
- Returns
- whether this object holds valid information about a plugin. If this is
true
pluginId() will return a non-empty string.
Definition at line 306 of file kpluginmetadata.cpp.
◆ license()
QString KPluginMetaData::license | ( | ) | const |
- Returns
- the short license identifier (e.g. LGPL).
- See also
- KAboutLicense::byKeyword() for retrieving the full license information
Definition at line 375 of file kpluginmetadata.cpp.
◆ licenseText()
QString KPluginMetaData::licenseText | ( | ) | const |
- Returns
- the text of the license, equivalent to KAboutLicense::byKeyword(license()).text()
- Since
- 5.73
Definition at line 380 of file kpluginmetadata.cpp.
◆ mimeTypes()
QStringList KPluginMetaData::mimeTypes | ( | ) | const |
- Returns
- a list of MIME types this plugin can handle (e.g. "application/pdf", "image/png", etc.)
- Since
- 5.16
Definition at line 415 of file kpluginmetadata.cpp.
◆ name()
QString KPluginMetaData::name | ( | ) | const |
- Returns
- the user visible name of the plugin.
Definition at line 385 of file kpluginmetadata.cpp.
◆ operator!=()
|
inline |
- Returns
true
if this object is not equal toother
, otherwisefalse
.
Definition at line 401 of file kpluginmetadata.h.
◆ operator=()
KPluginMetaData & KPluginMetaData::operator= | ( | const KPluginMetaData & | other | ) |
Copy assignment.
Definition at line 152 of file kpluginmetadata.cpp.
◆ operator==()
bool KPluginMetaData::operator== | ( | const KPluginMetaData & | other | ) | const |
- Returns
true
if this object is equal toother
, otherwisefalse
Definition at line 527 of file kpluginmetadata.cpp.
◆ otherContributors()
QList< KAboutPerson > KPluginMetaData::otherContributors | ( | ) | const |
- Returns
- a list of people that contributed to this plugin (other than the authors and translators).
- Since
- 5.18
Definition at line 355 of file kpluginmetadata.cpp.
◆ pluginId()
QString KPluginMetaData::pluginId | ( | ) | const |
- Returns
- the unique identifier within the namespace of the plugin
For C++ plugins, this ID is derived from the filename. It should not be set in the metadata explicitly.
When using KPluginMetaData::fromJsonFile or KPluginMetaData(QJsonObject, QString), the "Id" of the "KPlugin" object will be used. If unset, it will be derived from the filename.
Definition at line 395 of file kpluginmetadata.cpp.
◆ rawData()
QJsonObject KPluginMetaData::rawData | ( | ) | const |
- Returns
- the full metadata stored inside the plugin file.
Definition at line 240 of file kpluginmetadata.cpp.
◆ supportsMimeType()
bool KPluginMetaData::supportsMimeType | ( | const QString & | mimeType | ) | const |
- Returns
- true if this plugin can handle the given mimetype This is more accurate than mimeTypes().contains(mimeType) because it also takes MIME type inheritance into account.
- Since
- 5.66
Definition at line 420 of file kpluginmetadata.cpp.
◆ translators()
QList< KAboutPerson > KPluginMetaData::translators | ( | ) | const |
- Returns
- the translator(s) of this plugin.
- Since
- 5.18
Definition at line 350 of file kpluginmetadata.cpp.
◆ value() [1/4]
bool KPluginMetaData::value | ( | const QString & | key, |
bool | defaultValue ) const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Since
- 5.88
Definition at line 475 of file kpluginmetadata.cpp.
◆ value() [2/4]
QString KPluginMetaData::value | ( | const QString & | key, |
const QString & | defaultValue = QString() ) const |
- Returns
- the string value for
key
from the metadata ordefaultValue
if the key does not exist
if QString is not the correct type for key
you should use the other overloads or KPluginMetaData::rawData
Definition at line 460 of file kpluginmetadata.cpp.
◆ value() [3/4]
QStringList KPluginMetaData::value | ( | const QString & | key, |
const QStringList & | defaultValue ) const |
- Returns
- the value for
key
from the metadata ordefaultValue
if the key does not exist. If the type ofkey
is string, a list containing just that string will be returned. If the type is array, the list will contain one entry for each array member. This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Since
- 5.88
Definition at line 506 of file kpluginmetadata.cpp.
◆ value() [4/4]
int KPluginMetaData::value | ( | const QString & | key, |
int | defaultValue ) const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Since
- 5.88
Definition at line 487 of file kpluginmetadata.cpp.
◆ version()
QString KPluginMetaData::version | ( | ) | const |
- Returns
- the version of the plugin.
Definition at line 400 of file kpluginmetadata.cpp.
◆ website()
QString KPluginMetaData::website | ( | ) | const |
- Returns
- the website of the plugin.
Definition at line 405 of file kpluginmetadata.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:56:13 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.