Plasma::Containment
#include <Plasma/Containment>
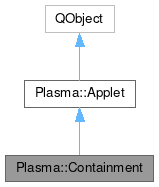
Public Types | |
enum | Type { NoContainment = -1 , Desktop = 0 , Panel , Custom = 127 , CustomPanel = 128 , CustomEmbedded = 129 } |
![]() | |
enum | Constraint { NoConstraint = 0 , FormFactorConstraint = 1 , LocationConstraint = 2 , ScreenConstraint = 4 , ImmutableConstraint = 8 , StartupCompletedConstraint = 16 , UiReadyConstraint = 32 , AllConstraints = FormFactorConstraint | LocationConstraint | ScreenConstraint | ImmutableConstraint } |
enum | ConstraintHint { NoHint = 0 , CanFillArea = 1 , MarginAreasSeparator = CanFillArea | 2 } |
typedef QFlags< ConstraintHint > | ConstraintHints |
typedef QFlags< Constraint > | Constraints |
Public Slots | |
void | reactToScreenChange () |
void | setContainmentDisplayHints (Plasma::Types::ContainmentDisplayHints hints) |
void | setFormFactor (Plasma::Types::FormFactor formFactor) |
void | setLocation (Plasma::Types::Location location) |
![]() | |
virtual void | configChanged () |
void | destroy () |
void | flushPendingConstraintsEvents () |
void | setImmutability (const Types::ImmutabilityType immutable) |
void | setLaunchErrorMessage (const QString &reason=QString()) |
void | setStatus (const Types::ItemStatus stat) |
Protected Member Functions | |
virtual void | restoreContents (KConfigGroup &group) |
virtual void | saveContents (KConfigGroup &group) const |
![]() | |
virtual void | constraintsEvent (Constraints constraints) |
virtual void | saveState (KConfigGroup &config) const |
void | timerEvent (QTimerEvent *event) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
The base class for plugins that provide backgrounds and applet grouping containers.
Containment objects provide the means to group applets into functional sets. They also provide the following:
creation of focusing event
- drawing of the background image (which can be interactive)
- form factors (e.g. panel, desktop, full screen, etc)
- applet layout management
Since containment is actually just a Plasma::Applet, all the techniques used for writing the visual presentation of Applets is applicable to Containtments. Containments are differentiated from Applets by being marked with the ServiceType of Plasma/Containment. Plugins registered with both the Applet and the Containment ServiceTypes can be loaded for us in either situation.
See techbase.kde.org for a tutorial on writing Containments using this class.
Definition at line 46 of file containment.h.
Member Enumeration Documentation
◆ Type
This enumeration describes the type of the Containment.
DesktopContainments represent main containments that will own a screen in a mutually exclusive fashion, while PanelContainments are accessories which can be present multiple per screen.
This value is specified in the "X-Plasma-ContainmentType" JSON-metadata value of containments.
Definition at line 134 of file containment.h.
Property Documentation
◆ activity
|
read |
Activity UID of this containment.
Definition at line 69 of file containment.h.
◆ activityName
|
read |
Activity name of this containment.
Definition at line 74 of file containment.h.
◆ applets
|
read |
List of applets this containment has: the containments KF6: this should be AppletQuickItem *.
Definition at line 54 of file containment.h.
◆ availableScreenRect
|
read |
screen area free of panels: the coordinates are relative to the containment, it's independent from the screen position For more precise available geometry use availableScreenRegion()
Definition at line 94 of file containment.h.
◆ availableScreenRegion
The available region of this screen, panels excluded.
It's a list of rectangles
Definition at line 99 of file containment.h.
◆ containmentDisplayHints
|
readwrite |
Definition at line 76 of file containment.h.
◆ containmentType
|
read |
Type of this containment.
Definition at line 64 of file containment.h.
◆ corona
|
read |
The corona for this contaiment.
Definition at line 59 of file containment.h.
◆ isUiReady
|
read |
Definition at line 82 of file containment.h.
◆ screen
|
read |
The screen number this containment is serving as the desktop for, or -1 if none.
Definition at line 87 of file containment.h.
◆ screenGeometry
|
read |
Provides access to the geometry of the applet is in.
Can be useful to figure out what's the absolute position of the applet.
Definition at line 105 of file containment.h.
◆ wallpaperGraphicsObject
|
readwrite |
Definition at line 80 of file containment.h.
◆ wallpaperPlugin
|
readwrite |
Definition at line 79 of file containment.h.
Constructor & Destructor Documentation
◆ Containment()
|
explicit |
This constructor can be used with the KCoreAddons plugin loading system.
The argument list is expected to have contain the KPackage of the applet, the meta data file path (for compatibility) and an applet ID which must be a base 10 number.
- Parameters
-
parent a QObject parent; you probably want to pass in 0 data,KPluginMetaData used to create this plugin args a list of strings containing the applet id
- Since
- 5.86
Definition at line 43 of file containment.cpp.
◆ ~Containment()
|
override |
Definition at line 61 of file containment.cpp.
Member Function Documentation
◆ activity()
QString Plasma::Containment::activity | ( | ) | const |
- Returns
- the current activity id associated with this containment TODO KF6: this should be available to Appelt as well as a property... virtual?
Definition at line 652 of file containment.cpp.
◆ activityChanged
|
signal |
Emitted when the activity id has changed.
◆ activityName()
QString Plasma::Containment::activityName | ( | ) | const |
- Returns
- Activity name corresponding to the activity UID
- See also
- activity
Definition at line 657 of file containment.cpp.
◆ activityNameChanged
|
signal |
Emitted when the activity name has changed.
◆ addApplet()
Add an existing applet to this Containment.
- Parameters
-
applet the applet that should be added geometryHint an hint to pass to the GUI on the location and size we prefer for the newly created applet; the gui might choose whether to respect or not this hint
Definition at line 352 of file containment.cpp.
◆ appletAboutToBeAdded
|
signal |
This signal is emitted right before appletAdded, it can be used to do a preliminary setup on the applet before the handlers of appletAdded are executed.
Useful for instance to prepare the GUI for the applet
- Parameters
-
applet the applet that is about to be added geometryHint an hint to pass to the GUI on the location and size we prefer for the newly created applet; the gui might choose whether to respect or not this hint
◆ appletAboutToBeRemoved
|
signal |
This signal is emitted right before appletRemoved, it can be used to do a preliminary setup on the applet before the handlers of appletRemoved are executed.
Useful for instance to prepare or teardown the GUI for the applet
◆ appletAdded
|
signal |
This signal is emitted when a new applet is added in the containment It may happen in the following situations:
- The user created the applet
- The applet was moved in from another containment
- The applet got restored at startup
- Parameters
-
applet the applet that has been added geometryHint an hint to pass to the GUI on the location and size we prefer for the newly created applet; the gui might choose whether to respect or not this hint
◆ appletAlternativesRequested
|
signal |
Emitted when the user wants to chose an alternative for this applet or containment.
◆ appletCreated
|
signal |
This signal is emitted when a new applet is created by the containment.
Compared to appletAdded, this gets emitted only when the user explicitly creates a new applet, either via the widget explorer or the scripting environment.
- See also
- appletAdded
- Since
- 5.16
◆ appletRemoved
|
signal |
This signal is emitted when an applet is destroyed.
◆ applets()
- Returns
- the applets currently in this Containment
Definition at line 462 of file containment.cpp.
◆ appletsChanged
|
signal |
Emitted when the list of applets has changed, either added or removed.
◆ availableRelativeScreenRect()
QRectF Plasma::Containment::availableRelativeScreenRect | ( | ) | const |
- Returns
- The available screen rect (excluding panels) for the screen this containment is associated to, empty rectangle if the containment is not active in a screen
Definition at line 487 of file containment.cpp.
◆ availableRelativeScreenRectChanged
|
signal |
Emitted when the available screen rectangle has changed.
◆ availableRelativeScreenRegion()
- Returns
- The available region of this screen, panels excluded. It's a list of rectangles
Definition at line 515 of file containment.cpp.
◆ availableRelativeScreenRegionChanged
|
signal |
Emitted when the available screen rectangle has changed.
◆ configureRequested
|
signal |
Emitted when the user wants to configure/change the containment, or an applet inside it.
◆ containmentActions()
QHash< QString, ContainmentActions * > & Plasma::Containment::containmentActions | ( | ) |
- Returns
- All the loaded containment action plugins, indexed by trigger name
- Since
- 5.0
Definition at line 628 of file containment.cpp.
◆ containmentType()
Plasma::Containment::Type Plasma::Containment::containmentType | ( | ) | const |
Returns the type of containment.
Definition at line 277 of file containment.cpp.
◆ containmentTypeChanged
|
signal |
emitted when the containment type changed
◆ corona()
Corona * Plasma::Containment::corona | ( | ) | const |
Returns the Corona (if any) that this Containment is hosted by.
Definition at line 282 of file containment.cpp.
◆ createApplet()
Applet * Plasma::Containment::createApplet | ( | const QString & | name, |
const QVariantList & | args = QVariantList(), | ||
const QRectF & | geometryHint = QRectF(-1, -1, 0, 0) ) |
Adds an applet to this Containment.
- Parameters
-
name the plugin name for the applet, as given by KPluginInfo::pluginName() args argument list to pass to the plasmoid geometryHint an hint to pass to the GUI on the location and size we prefer for the newly created applet; the gui might choose whether to respect or not this hint. The default position is (-1, -1) and the default size is (0, 0).
- Returns
- a pointer to the applet on success, or 0 on failure
Definition at line 343 of file containment.cpp.
◆ formFactorChanged
|
signal |
Emitted when the formFactor has changed.
- Since
- 5.0
◆ init()
|
overridevirtual |
Reimplemented from Applet.
Reimplemented from Plasma::Applet.
Definition at line 68 of file containment.cpp.
◆ isUiReady()
bool Plasma::Containment::isUiReady | ( | ) | const |
- Returns
- true when the ui of this containment is fully loaded, as well the ui of every applet in it
Definition at line 633 of file containment.cpp.
◆ lastScreen()
int Plasma::Containment::lastScreen | ( | ) | const |
- Returns
- the last screen number this containment had only returns -1 if it's never ever been on a screen
- Since
- 4.5
Definition at line 479 of file containment.cpp.
◆ locationChanged
|
signal |
Emitted when the location has changed.
- Since
- 5.0
◆ reactToScreenChange
|
slot |
Definition at line 665 of file containment.cpp.
◆ restore()
|
overridevirtual |
- Reimplemented from superclass.
- See also
- Applet::restore(KConfigGroup &)
Reimplemented from Plasma::Applet.
Definition at line 135 of file containment.cpp.
◆ restoreContents()
|
protectedvirtual |
Called when the contents of the containment should be loaded.
By default this loads all previously saved Applets
- Parameters
-
group the KConfigGroup to save settings under
Definition at line 230 of file containment.cpp.
◆ save()
|
overridevirtual |
- Reimplemented from superclass.
- See also
- Applet::save(KConfigGroup &)
Reimplemented from Plasma::Applet.
Definition at line 196 of file containment.cpp.
◆ saveContents()
|
protectedvirtual |
Called when the contents of the containment should be saved.
By default this saves all loaded Applets
- Parameters
-
group the KConfigGroup to save settings under
Definition at line 221 of file containment.cpp.
◆ screen()
int Plasma::Containment::screen | ( | ) | const |
- Returns
- the screen number this containment is serving as the desktop for or -1 if none TODO KF6 virtual? this shouldbe available to applet as well
Definition at line 467 of file containment.cpp.
◆ screenChanged
|
signal |
This signal indicates that a containment has been associated (or dissociated) with a physical screen.
- Parameters
-
newScreen the screen it is now associated with
◆ screenGeometry()
QRectF Plasma::Containment::screenGeometry | ( | ) | const |
- Returns
- The geometry of the screen this containment is associated to
Definition at line 541 of file containment.cpp.
◆ screenGeometryChanged
|
signal |
Emitted when the screen geometry has changed.
◆ setActivity()
void Plasma::Containment::setActivity | ( | const QString & | activityId | ) |
Sets the current activity by id.
- Parameters
-
activity the id of the activity
Definition at line 638 of file containment.cpp.
◆ setContainmentActions()
void Plasma::Containment::setContainmentActions | ( | const QString & | trigger, |
const QString & | pluginName ) |
Sets a containmentactions plugin.
- Parameters
-
trigger the mouse button (and optional modifier) to associate the plugin with pluginName the name of the plugin to attempt to load. blank = set no plugin.
- Since
- 4.4
Definition at line 589 of file containment.cpp.
◆ setContainmentDisplayHints
|
slot |
Set Display hints that come from the containment that suggest the applet how to look and behave.
- Parameters
-
hints the new hints, as bitwise OR
- Since
- 5.77
Definition at line 313 of file containment.cpp.
◆ setFormFactor
|
slot |
Sets the form factor for this Containment.
This may cause changes in both the arrangement of Applets as well as the display choices of individual Applets.
Definition at line 296 of file containment.cpp.
◆ setLocation
|
slot |
Informs the Corona as to what position it is in.
This is informational only, as the Corona doesn't change its actual location. This is, however, passed on to Applets that may be managed by this Corona.
- Parameters
-
location the new location of this Corona
Definition at line 323 of file containment.cpp.
◆ setWallpaperPlugin()
void Plasma::Containment::setWallpaperPlugin | ( | const QString & | pluginName | ) |
Sets wallpaper plugin.
- Parameters
-
pluginName the name of the wallpaper to attempt to load
Definition at line 550 of file containment.cpp.
◆ showAddWidgetsInterface
|
signal |
Emitted when the containment requests an add widgets dialog is shown.
Usually only used for desktop containments.
- Parameters
-
pos where in the containment this request was made from, or an invalid position (QPointF()) is not location specific
◆ uiReadyChanged
|
signal |
Emitted when the ui has been fully loaded and is fully working.
- Parameters
-
uiReady true when the ui of the containment is ready, as well the ui of each applet in it
◆ wallpaperGraphicsObjectChanged
|
signal |
Emitted when the root wallpaper item has changed.
◆ wallpaperPlugin()
QString Plasma::Containment::wallpaperPlugin | ( | ) | const |
Return wallpaper plugin.
Definition at line 562 of file containment.cpp.
◆ wallpaperPluginChanged
|
signal |
Emitted when the wallpaper plugin is changed.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:12:57 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.