PlasmoidItem
#include <plasmoiditem.h>
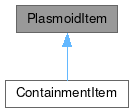
Properties | |
QRect | availableScreenRect |
QVariantList | availableScreenRegion |
bool | hideOnWindowDeactivate |
int | screen |
QRect | screenGeometry |
QQuickItem * | toolTipItem |
QString | toolTipMainText |
QString | toolTipSubText |
int | toolTipTextFormat |
Signals | |
void | availableScreenRectChanged () |
void | availableScreenRegionChanged () |
void | contextualActionsAboutToShow () |
void | contextualActionsChanged () |
void | externalData (const QString &mimetype, const QVariant &data) |
void | hideOnWindowDeactivateChanged () |
void | screenChanged () |
void | screenGeometryChanged () |
void | toolTipItemChanged () |
void | toolTipMainTextChanged () |
void | toolTipSubTextChanged () |
void | toolTipTextFormatChanged () |
Public Member Functions | |
PlasmoidItem (QQuickItem *parent=nullptr) | |
QRect | availableScreenRect () const |
QVariantList | availableScreenRegion () const |
bool | hideOnWindowDeactivate () const |
QString | pluginName () const |
Q_INVOKABLE void | prepareContextualActions () |
int | screen () const |
QRect | screenGeometry () const |
void | setHideOnWindowDeactivate (bool hide) |
void | setToolTipItem (QQuickItem *toolTipItem) |
void | setToolTipMainText (const QString &text) |
void | setToolTipSubText (const QString &text) |
void | setToolTipTextFormat (int format) |
QQuickItem * | toolTipItem () const |
QString | toolTipMainText () const |
QString | toolTipSubText () const |
int | toolTipTextFormat () const |
Protected Member Functions | |
bool | event (QEvent *event) override |
bool | eventFilter (QObject *watched, QEvent *event) override |
void | init () override |
Detailed Description
Import Statement
- Version
- 2.0
Definition at line 40 of file plasmoiditem.h.
Property Documentation
◆ availableScreenRect
|
read |
screen area free of panels: the coordinates are relative to the containment, it's independent from the screen position For more precise available geometry use availableScreenRegion()
Definition at line 104 of file plasmoiditem.h.
◆ availableScreenRegion
|
read |
The available region of this screen, panels excluded.
It's a list of rectanglesO: from containment
Definition at line 109 of file plasmoiditem.h.
◆ hideOnWindowDeactivate
|
readwrite |
Whether the dialog should be hidden when the dialog loses focus.
The default value is false
. TODO KF6: move to Applet? probably not
Definition at line 97 of file plasmoiditem.h.
◆ screen
|
read |
Definition at line 82 of file plasmoiditem.h.
◆ screenGeometry
|
read |
Provides access to the geometry of the applet is in.
Can be useful to figure out what's the absolute position of the applet. TODO: move in containment
Definition at line 89 of file plasmoiditem.h.
◆ toolTipItem
|
readwrite |
This allows to set fully custom QML item as the tooltip.
It will ignore all texts set by setToolTipMainText or setToolTipSubText
- Since
- 5.19
Definition at line 76 of file plasmoiditem.h.
◆ toolTipMainText
|
readwrite |
The QML root object defined in the applet main.qml will be direct child of an PlasmoidItem instance.
Main title for the plasmoid tooltip or other means of quick information: it's the same as the title property by default, but it can be personalized
Definition at line 52 of file plasmoiditem.h.
◆ toolTipSubText
|
readwrite |
Description for the plasmoid tooltip or other means of quick information: it comes from the pluginifo comment by default, but it can be personalized.
Definition at line 58 of file plasmoiditem.h.
◆ toolTipTextFormat
|
readwrite |
how to handle the text format of the tooltip subtext:
- Text.AutoText (default)
- Text.PlainText
- Text.StyledText
- Text.RichText Note: in the default implementation the main text is always plain text
Definition at line 68 of file plasmoiditem.h.
Constructor & Destructor Documentation
◆ PlasmoidItem()
PlasmoidItem::PlasmoidItem | ( | QQuickItem * | parent = nullptr | ) |
Definition at line 33 of file plasmoiditem.cpp.
◆ ~PlasmoidItem()
|
override |
Definition at line 44 of file plasmoiditem.cpp.
Member Function Documentation
◆ availableScreenRect()
QRect PlasmoidItem::availableScreenRect | ( | ) | const |
Definition at line 292 of file plasmoiditem.cpp.
◆ availableScreenRegion()
QVariantList PlasmoidItem::availableScreenRegion | ( | ) | const |
Definition at line 266 of file plasmoiditem.cpp.
◆ contextualActionsAboutToShow
|
signal |
Emitted just before the contextual actions are about to show For instance just before the context menu containing the actions added with setAction() is shown.
◆ event()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 321 of file plasmoiditem.cpp.
◆ eventFilter()
Reimplemented from QObject.
Definition at line 394 of file plasmoiditem.cpp.
◆ externalData
somebody else, usually the containment sent some data to the applet
- Parameters
-
mimetype the mime type of the data such as text/plain data either the actual data or an URL representing it
◆ hideOnWindowDeactivate()
bool PlasmoidItem::hideOnWindowDeactivate | ( | ) | const |
Definition at line 252 of file plasmoiditem.cpp.
◆ init()
|
overrideprotected |
Definition at line 48 of file plasmoiditem.cpp.
◆ prepareContextualActions()
void PlasmoidItem::prepareContextualActions | ( | ) |
Should be called before retrieving any action to ensure contents are up to date.
- See also
- contextualActionsAboutToShow
- Since
- 5.58
Definition at line 389 of file plasmoiditem.cpp.
◆ screen()
int PlasmoidItem::screen | ( | ) | const |
Definition at line 235 of file plasmoiditem.cpp.
◆ screenGeometry()
QRect PlasmoidItem::screenGeometry | ( | ) | const |
Definition at line 257 of file plasmoiditem.cpp.
◆ setHideOnWindowDeactivate()
void PlasmoidItem::setHideOnWindowDeactivate | ( | bool | hide | ) |
Definition at line 244 of file plasmoiditem.cpp.
◆ setToolTipItem()
void PlasmoidItem::setToolTipItem | ( | QQuickItem * | toolTipItem | ) |
Definition at line 223 of file plasmoiditem.cpp.
◆ setToolTipMainText()
void PlasmoidItem::setToolTipMainText | ( | const QString & | text | ) |
Definition at line 160 of file plasmoiditem.cpp.
◆ setToolTipSubText()
void PlasmoidItem::setToolTipSubText | ( | const QString & | text | ) |
Definition at line 187 of file plasmoiditem.cpp.
◆ setToolTipTextFormat()
void PlasmoidItem::setToolTipTextFormat | ( | int | format | ) |
Definition at line 208 of file plasmoiditem.cpp.
◆ toolTipItem()
QQuickItem * PlasmoidItem::toolTipItem | ( | ) | const |
Definition at line 218 of file plasmoiditem.cpp.
◆ toolTipMainText()
QString PlasmoidItem::toolTipMainText | ( | ) | const |
Definition at line 151 of file plasmoiditem.cpp.
◆ toolTipSubText()
QString PlasmoidItem::toolTipSubText | ( | ) | const |
Definition at line 178 of file plasmoiditem.cpp.
◆ toolTipTextFormat()
int PlasmoidItem::toolTipTextFormat | ( | ) | const |
Definition at line 203 of file plasmoiditem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:47 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.